Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / SectionXmlInfo.cs / 1305376 / SectionXmlInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.Configuration; using System.Text; using System.Threading; using System.Reflection; using System.Xml; internal sealed class SectionXmlInfo : IConfigErrorInfo { private string _configKey; // configKey private string _definitionConfigPath; // the config path of the configuration record where this directive was defined private string _targetConfigPath; // the full config path this location directive applies to private string _subPath; // the "path" attribute specified in thedirective private string _filename; // filename containg this section definition private int _lineNumber; // line number where definition occurs private object _streamVersion; // version of the filestream containing this section private string _configSource; // the "configSource" attribute private string _configSourceStreamName; // filename of the configSource private object _configSourceStreamVersion; // version of the configSource filestream private bool _skipInChildApps; // skip inheritence by child apps? private string _rawXml; // raw xml input of the section private string _protectionProviderName; // name of the protection provider private OverrideModeSetting _overrideMode; // override mode for child config paths internal SectionXmlInfo( string configKey, string definitionConfigPath, string targetConfigPath, string subPath, string filename, int lineNumber, object streamVersion, string rawXml, string configSource, string configSourceStreamName, object configSourceStreamVersion, string protectionProviderName, OverrideModeSetting overrideMode, bool skipInChildApps) { _configKey = configKey; _definitionConfigPath = definitionConfigPath; _targetConfigPath = targetConfigPath; _subPath = subPath; _filename = filename; _lineNumber = lineNumber; _streamVersion = streamVersion; _rawXml = rawXml; _configSource = configSource; _configSourceStreamName = configSourceStreamName; _configSourceStreamVersion = configSourceStreamVersion; _protectionProviderName = protectionProviderName; _overrideMode = overrideMode; _skipInChildApps = skipInChildApps; } // IConfigErrorInfo interface public string Filename { get {return _filename;} } public int LineNumber { get {return _lineNumber;} set {_lineNumber = value;} } // other access methods internal object StreamVersion { get {return _streamVersion;} set {_streamVersion = value;} } internal string ConfigSource { get {return _configSource;} set {_configSource = value;} } internal string ConfigSourceStreamName { get {return _configSourceStreamName;} set {_configSourceStreamName = value;} } internal object ConfigSourceStreamVersion { #if UNUSED_CODE get {return _configSourceStreamVersion; } #endif set {_configSourceStreamVersion = value; } } internal string ConfigKey { get {return _configKey;} } internal string DefinitionConfigPath { get {return _definitionConfigPath;} } internal string TargetConfigPath { get {return _targetConfigPath;} set {_targetConfigPath = value;} } internal string SubPath { get {return _subPath;} } internal string RawXml { get {return _rawXml;} set {_rawXml = value;} } internal string ProtectionProviderName { get {return _protectionProviderName;} set {_protectionProviderName = value;} } internal OverrideModeSetting OverrideModeSetting { get { return _overrideMode; } set { _overrideMode = value; } } internal bool SkipInChildApps { get {return _skipInChildApps;} set {_skipInChildApps = value;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.Configuration; using System.Text; using System.Threading; using System.Reflection; using System.Xml; internal sealed class SectionXmlInfo : IConfigErrorInfo { private string _configKey; // configKey private string _definitionConfigPath; // the config path of the configuration record where this directive was defined private string _targetConfigPath; // the full config path this location directive applies to private string _subPath; // the "path" attribute specified in thedirective private string _filename; // filename containg this section definition private int _lineNumber; // line number where definition occurs private object _streamVersion; // version of the filestream containing this section private string _configSource; // the "configSource" attribute private string _configSourceStreamName; // filename of the configSource private object _configSourceStreamVersion; // version of the configSource filestream private bool _skipInChildApps; // skip inheritence by child apps? private string _rawXml; // raw xml input of the section private string _protectionProviderName; // name of the protection provider private OverrideModeSetting _overrideMode; // override mode for child config paths internal SectionXmlInfo( string configKey, string definitionConfigPath, string targetConfigPath, string subPath, string filename, int lineNumber, object streamVersion, string rawXml, string configSource, string configSourceStreamName, object configSourceStreamVersion, string protectionProviderName, OverrideModeSetting overrideMode, bool skipInChildApps) { _configKey = configKey; _definitionConfigPath = definitionConfigPath; _targetConfigPath = targetConfigPath; _subPath = subPath; _filename = filename; _lineNumber = lineNumber; _streamVersion = streamVersion; _rawXml = rawXml; _configSource = configSource; _configSourceStreamName = configSourceStreamName; _configSourceStreamVersion = configSourceStreamVersion; _protectionProviderName = protectionProviderName; _overrideMode = overrideMode; _skipInChildApps = skipInChildApps; } // IConfigErrorInfo interface public string Filename { get {return _filename;} } public int LineNumber { get {return _lineNumber;} set {_lineNumber = value;} } // other access methods internal object StreamVersion { get {return _streamVersion;} set {_streamVersion = value;} } internal string ConfigSource { get {return _configSource;} set {_configSource = value;} } internal string ConfigSourceStreamName { get {return _configSourceStreamName;} set {_configSourceStreamName = value;} } internal object ConfigSourceStreamVersion { #if UNUSED_CODE get {return _configSourceStreamVersion; } #endif set {_configSourceStreamVersion = value; } } internal string ConfigKey { get {return _configKey;} } internal string DefinitionConfigPath { get {return _definitionConfigPath;} } internal string TargetConfigPath { get {return _targetConfigPath;} set {_targetConfigPath = value;} } internal string SubPath { get {return _subPath;} } internal string RawXml { get {return _rawXml;} set {_rawXml = value;} } internal string ProtectionProviderName { get {return _protectionProviderName;} set {_protectionProviderName = value;} } internal OverrideModeSetting OverrideModeSetting { get { return _overrideMode; } set { _overrideMode = value; } } internal bool SkipInChildApps { get {return _skipInChildApps;} set {_skipInChildApps = value;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
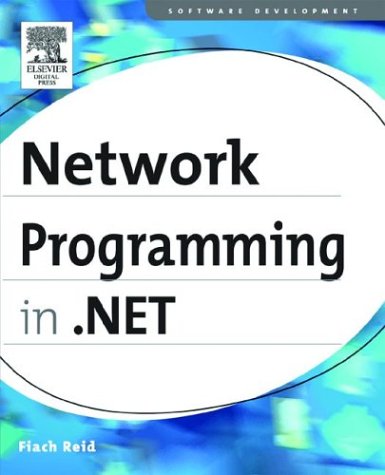
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewEditEventArgs.cs
- AttributeSetAction.cs
- PeerTransportElement.cs
- ParallelEnumerableWrapper.cs
- ProfilePropertySettings.cs
- DataMisalignedException.cs
- HtmlTernaryTree.cs
- TextBox.cs
- ItemAutomationPeer.cs
- OleDbSchemaGuid.cs
- DataProtection.cs
- MobileCategoryAttribute.cs
- elementinformation.cs
- OverrideMode.cs
- InvalidPropValue.cs
- DurationConverter.cs
- ServiceDurableInstanceContextProvider.cs
- OrderToken.cs
- TextReader.cs
- ResXDataNode.cs
- CurrentChangedEventManager.cs
- LogEntryDeserializer.cs
- EFAssociationProvider.cs
- DictionaryContent.cs
- EmptyReadOnlyDictionaryInternal.cs
- DSASignatureFormatter.cs
- DirectoryInfo.cs
- RuntimeConfig.cs
- DynamicValidator.cs
- LinkLabel.cs
- AuthorizationSection.cs
- ContentOperations.cs
- InstanceDataCollection.cs
- DrawItemEvent.cs
- Calendar.cs
- FileUtil.cs
- Wrapper.cs
- DeflateInput.cs
- ClickablePoint.cs
- GridItemPattern.cs
- Compiler.cs
- XmlDocument.cs
- XmlDataDocument.cs
- CapabilitiesUse.cs
- InternalMappingException.cs
- ApplicationProxyInternal.cs
- NotifyCollectionChangedEventArgs.cs
- ServiceRoute.cs
- HttpCapabilitiesSectionHandler.cs
- DetailsViewModeEventArgs.cs
- keycontainerpermission.cs
- BaseTypeViewSchema.cs
- AttachInfo.cs
- ToolStripRendererSwitcher.cs
- ComNativeDescriptor.cs
- ColumnCollection.cs
- DateTimeFormatInfoScanner.cs
- XmlSchemaSimpleContentExtension.cs
- NativeActivityFaultContext.cs
- XpsImage.cs
- FileDataSourceCache.cs
- PackWebRequest.cs
- DbMetaDataCollectionNames.cs
- RoutingChannelExtension.cs
- XmlAttributes.cs
- UnsafeNativeMethodsPenimc.cs
- FrugalList.cs
- XmlSchemaException.cs
- UnaryNode.cs
- FixedSchema.cs
- MissingFieldException.cs
- DecimalAnimationUsingKeyFrames.cs
- SqlRewriteScalarSubqueries.cs
- figurelength.cs
- ProcessHostFactoryHelper.cs
- AnnotationResource.cs
- CatalogZone.cs
- Normalizer.cs
- MachineKeyConverter.cs
- SystemWebExtensionsSectionGroup.cs
- DurationConverter.cs
- ServiceInfo.cs
- QueryCacheManager.cs
- SymLanguageType.cs
- If.cs
- HttpWrapper.cs
- ProcessHostServerConfig.cs
- HtmlControlPersistable.cs
- SecurityException.cs
- IisHelper.cs
- XsltQilFactory.cs
- XNodeValidator.cs
- ScalarConstant.cs
- ListViewGroupItemCollection.cs
- ArrayTypeMismatchException.cs
- AnnotationResourceCollection.cs
- ReflectionPermission.cs
- TypeContext.cs
- ItemTypeToolStripMenuItem.cs
- ListItemCollection.cs