Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / DeflateInput.cs / 1305376 / DeflateInput.cs
namespace System.IO.Compression { using System.Diagnostics; internal class DeflateInput { private byte[] buffer; private int count; private int startIndex; internal byte[] Buffer { get { return buffer; } set { buffer = value; } } internal int Count { get { return count; } set { count = value; } } internal int StartIndex { get { return startIndex; } set { startIndex = value; } } internal void ConsumeBytes(int n) { Debug.Assert(n <= count, "Should use more bytes than what we have in the buffer"); startIndex += n; count -= n; Debug.Assert(startIndex + count <= buffer.Length, "Input buffer is in invalid state!"); } internal InputState DumpState() { InputState savedState; savedState.count = count; savedState.startIndex = startIndex; return savedState; } internal void RestoreState(InputState state) { count = state.count; startIndex = state.startIndex; } internal struct InputState { internal int count; internal int startIndex; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
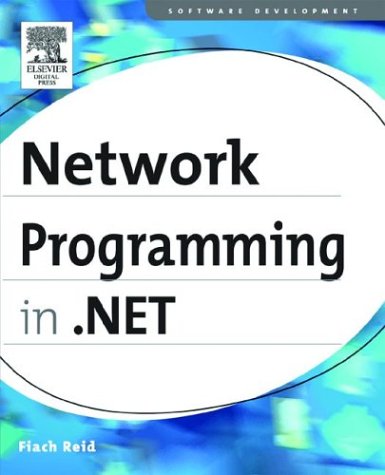
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpPostedFile.cs
- tibetanshape.cs
- FactoryRecord.cs
- TimeStampChecker.cs
- WebControlAdapter.cs
- HMACSHA1.cs
- ResourceExpression.cs
- SspiSafeHandles.cs
- SessionStateItemCollection.cs
- DropSource.cs
- Token.cs
- TextEvent.cs
- ArgIterator.cs
- HttpApplicationFactory.cs
- SecureStringHasher.cs
- EntityDataSourceState.cs
- CompiledRegexRunnerFactory.cs
- ArrayTypeMismatchException.cs
- EntityDataSourceContainerNameConverter.cs
- Math.cs
- BindableTemplateBuilder.cs
- DataConnectionHelper.cs
- DesignerActionPanel.cs
- ScriptModule.cs
- SessionPageStatePersister.cs
- InputScopeManager.cs
- CopyNamespacesAction.cs
- CodeRemoveEventStatement.cs
- CodeDOMUtility.cs
- ThreadAttributes.cs
- SelectionEditor.cs
- DocumentSequenceHighlightLayer.cs
- DigitShape.cs
- IPGlobalProperties.cs
- CommandLineParser.cs
- TabControlEvent.cs
- SingleKeyFrameCollection.cs
- GacUtil.cs
- XmlIgnoreAttribute.cs
- dbenumerator.cs
- SqlUserDefinedTypeAttribute.cs
- EventMappingSettings.cs
- ResourceKey.cs
- ColumnHeaderConverter.cs
- XPathSingletonIterator.cs
- RepeatInfo.cs
- DataService.cs
- WeakReference.cs
- SQLSingleStorage.cs
- Keywords.cs
- DefaultIfEmptyQueryOperator.cs
- HtmlInputPassword.cs
- SafeHGlobalHandleCritical.cs
- EnumMember.cs
- CqlLexerHelpers.cs
- OLEDB_Util.cs
- WebPartCollection.cs
- ListBindingHelper.cs
- BitmapEffectRenderDataResource.cs
- Zone.cs
- FrameworkTextComposition.cs
- InkCollectionBehavior.cs
- XmlnsPrefixAttribute.cs
- FlatButtonAppearance.cs
- ServerValidateEventArgs.cs
- ControlType.cs
- WebResourceUtil.cs
- NetworkCredential.cs
- XmlFormatExtensionPointAttribute.cs
- Asn1IntegerConverter.cs
- SafeSystemMetrics.cs
- QilLiteral.cs
- HttpMethodConstraint.cs
- LocatorManager.cs
- GridViewItemAutomationPeer.cs
- TreeNodeCollection.cs
- TrackingServices.cs
- Vector3DAnimationBase.cs
- MediaPlayer.cs
- HttpCookieCollection.cs
- SymbolTable.cs
- BackgroundWorker.cs
- ToolboxDataAttribute.cs
- SystemIcmpV4Statistics.cs
- ActionFrame.cs
- Events.cs
- RegexWorker.cs
- FilterableAttribute.cs
- PropertyTabAttribute.cs
- CatalogPartChrome.cs
- BufferedStream.cs
- Clock.cs
- CheckBox.cs
- SrgsToken.cs
- SafeIUnknown.cs
- LinearKeyFrames.cs
- SubpageParaClient.cs
- EntitySetBaseCollection.cs
- MimeParameterWriter.cs
- MailAddressCollection.cs