Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / HttpCookieCollection.cs / 2 / HttpCookieCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Collection of Http cookies for request and response intrinsics * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCookieCollection : NameObjectCollectionBase { // Response object to notify about changes in collection private HttpResponse _response; // cached All[] arrays private HttpCookie[] _all; private String[] _allKeys; private bool _changed; internal HttpCookieCollection(HttpResponse response, bool readOnly) : base(StringComparer.OrdinalIgnoreCase) { _response = response; IsReadOnly = readOnly; } ////// Provides a type-safe /// way to manipulate HTTP cookies. /// ////// public HttpCookieCollection(): base(StringComparer.OrdinalIgnoreCase) { } internal bool Changed { get { return _changed; } set { _changed = value; } } internal void AddCookie(HttpCookie cookie, bool append) { _all = null; _allKeys = null; if (append) { // mark cookie as new cookie.Added = true; BaseAdd(cookie.Name, cookie); } else { if (BaseGet(cookie.Name) != null) { // mark the cookie as changed because we are overriding the existing one cookie.Changed = true; } BaseSet(cookie.Name, cookie); } } internal void RemoveCookie(String name) { _all = null; _allKeys = null; BaseRemove(name); _changed = true; } internal void Reset() { _all = null; _allKeys = null; BaseClear(); _changed = true; } // // Public APIs to add / remove // ////// Initializes a new instance of the HttpCookieCollection /// class. /// ////// public void Add(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, true); if (_response != null) _response.OnCookieAdd(cookie); } ////// Adds a cookie to the collection. /// ////// public void CopyTo(Array dest, int index) { if (_all == null) { int n = Count; _all = new HttpCookie[n]; for (int i = 0; i < n; i++) _all[i] = Get(i); } _all.CopyTo(dest, index); } ///[To be supplied.] ////// public void Set(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, false); if (_response != null) _response.OnCookieCollectionChange(); } ///Updates the value of a cookie. ////// public void Remove(String name) { if (_response != null) _response.BeforeCookieCollectionChange(); RemoveCookie(name); if (_response != null) _response.OnCookieCollectionChange(); } ////// Removes a cookie from the collection. /// ////// public void Clear() { Reset(); } // // Access by name // ////// Clears all cookies from the collection. /// ////// public HttpCookie Get(String name) { HttpCookie cookie = (HttpCookie)BaseGet(name); if (cookie == null && _response != null) { // response cookies are created on demand cookie = new HttpCookie(name); AddCookie(cookie, true); _response.OnCookieAdd(cookie); } return cookie; } ///Returns an ///item from the collection. /// public HttpCookie this[String name] { get { return Get(name);} } // // Indexed access // ///Indexed value that enables access to a cookie in the collection. ////// public HttpCookie Get(int index) { return(HttpCookie)BaseGet(index); } ////// Returns an ////// item from the collection. /// /// public String GetKey(int index) { return BaseGetKey(index); } ////// Returns key name from collection. /// ////// public HttpCookie this[int index] { get { return Get(index);} } // // Access to keys and values as arrays // /* * All keys */ ////// Default property. /// Indexed property that enables access to a cookie in the collection. /// ////// public String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } }/// Returns /// an array of all cookie keys in the cookie collection. /// ///
Link Menu
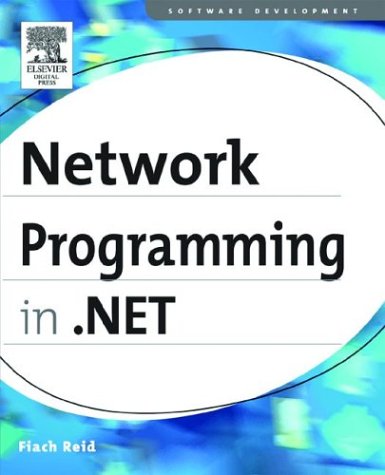
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IFormattable.cs
- _NtlmClient.cs
- ChineseLunisolarCalendar.cs
- EmptyEnumerator.cs
- NameTable.cs
- TypedLocationWrapper.cs
- KeySplineConverter.cs
- _HTTPDateParse.cs
- TimersDescriptionAttribute.cs
- HijriCalendar.cs
- DoubleUtil.cs
- BufferedOutputAsyncStream.cs
- DecimalFormatter.cs
- PageRequestManager.cs
- RemotingConfigParser.cs
- StaticExtension.cs
- Debugger.cs
- SafeProcessHandle.cs
- Site.cs
- X509ClientCertificateCredentialsElement.cs
- DocumentEventArgs.cs
- PairComparer.cs
- ReturnType.cs
- SigningCredentials.cs
- TemplateControl.cs
- PackageRelationshipSelector.cs
- DataGridViewRow.cs
- SeekStoryboard.cs
- FormCollection.cs
- BindingNavigator.cs
- HttpApplication.cs
- PathParser.cs
- EndpointDiscoveryElement.cs
- DataGridTable.cs
- EditorAttribute.cs
- FileLogRecord.cs
- FlowDocumentFormatter.cs
- DeviceSpecific.cs
- ExpressionBindingsDialog.cs
- GroupItem.cs
- ApplicationServiceHelper.cs
- PageContent.cs
- MetadataSource.cs
- HtmlFormParameterReader.cs
- DocumentReferenceCollection.cs
- XmlHierarchicalDataSourceView.cs
- MD5CryptoServiceProvider.cs
- CatalogPart.cs
- ImageField.cs
- TemplateEditingFrame.cs
- TransformConverter.cs
- RoleManagerModule.cs
- XPathPatternBuilder.cs
- ExpressionVisitorHelpers.cs
- HttpHandlersSection.cs
- HotSpot.cs
- EncodingTable.cs
- ParameterCollection.cs
- FileChangesMonitor.cs
- ToolStripItemBehavior.cs
- RecordManager.cs
- CryptoProvider.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ObjectDataSourceMethodEditor.cs
- Triangle.cs
- XmlSchemaExporter.cs
- DataGridViewColumnStateChangedEventArgs.cs
- UpdateTracker.cs
- HttpProfileGroupBase.cs
- ControlType.cs
- UnionCodeGroup.cs
- Quad.cs
- SqlAliasesReferenced.cs
- KoreanCalendar.cs
- UniqueIdentifierService.cs
- ZeroOpNode.cs
- XmlSchemaException.cs
- ParameterBuilder.cs
- DragDeltaEventArgs.cs
- loginstatus.cs
- AlgoModule.cs
- Panel.cs
- AsyncSerializedWorker.cs
- Matrix.cs
- SafeLibraryHandle.cs
- WebPartDescription.cs
- unsafenativemethodsother.cs
- PasswordPropertyTextAttribute.cs
- AsyncCompletedEventArgs.cs
- ScrollEventArgs.cs
- StandardToolWindows.cs
- MimePart.cs
- Stopwatch.cs
- WebPartVerbCollection.cs
- CursorEditor.cs
- CryptoApi.cs
- LoadWorkflowByInstanceKeyCommand.cs
- ServiceHttpModule.cs
- XmlSchemaObjectCollection.cs
- InteropTrackingRecord.cs