Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / DataClasses / RelationshipNavigation.cs / 1305376 / RelationshipNavigation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Objects.DataClasses { ////// This class describes a relationship navigation from the /// navigation property on one entity to another entity. It is /// used throughout the collections and refs system to describe a /// relationship and to connect from the navigation property on /// one end of a relationship to the navigation property on the /// other end. /// [Serializable] internal class RelationshipNavigation { // ------------ // Constructors // ------------ ////// Creates a navigation object with the given relationship /// name, role name for the source and role name for the /// destination. /// /// Canonical-space name of the relationship. /// Name of the role which is the source of the navigation. /// Name of the role which is the destination of the navigation. /// The navigation property which is the source of the navigation. /// The navigation property which is the destination of the navigation. internal RelationshipNavigation(string relationshipName, string from, string to, NavigationPropertyAccessor fromAccessor, NavigationPropertyAccessor toAccessor) { EntityUtil.CheckStringArgument(relationshipName, "relationshipName"); EntityUtil.CheckStringArgument(from, "from"); EntityUtil.CheckStringArgument(to, "to"); _relationshipName = relationshipName; _from = from; _to = to; _fromAccessor = fromAccessor; _toAccessor = toAccessor; } // ------ // Fields // ------ // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private readonly string _relationshipName; private readonly string _from; private readonly string _to; [NonSerialized] private RelationshipNavigation _reverse; [NonSerialized] private NavigationPropertyAccessor _fromAccessor; [NonSerialized] private NavigationPropertyAccessor _toAccessor; // ---------- // Properties // ---------- ////// Canonical-space relationship name. /// internal string RelationshipName { get { return _relationshipName; } } ////// Role name for the source of this navigation. /// internal string From { get { return _from; } } ////// Role name for the destination of this navigation. /// internal string To { get { return _to; } } ////// Navigation property name for the destination of this navigation. /// NOTE: There is not a FromPropertyAccessor property on RelationshipNavigation because it is not currently accessed anywhere /// It is only used to calculate the "reverse" RelationshipNavigation. /// internal NavigationPropertyAccessor ToPropertyAccessor { get { return _toAccessor; } } internal bool IsInitialized { get { return _toAccessor != null && _fromAccessor != null; } } internal void InitializeAccessors(NavigationPropertyAccessor fromAccessor, NavigationPropertyAccessor toAccessor) { _fromAccessor = fromAccessor; _toAccessor = toAccessor; } ////// The "reverse" version of this navigation. /// internal RelationshipNavigation Reverse { get { if (_reverse == null || !_reverse.IsInitialized) { // the reverse relationship is exactly like this // one but from & to are switched _reverse = new RelationshipNavigation(_relationshipName, _to, _from, _toAccessor, _fromAccessor); } return _reverse; } } ////// Compares this instance to a given Navigation by their values. /// public override bool Equals(object obj) { RelationshipNavigation compareTo = obj as RelationshipNavigation; return ((this == compareTo) || ((null != this) && (null != compareTo) && (this.RelationshipName == compareTo.RelationshipName) && (this.From == compareTo.From) && (this.To == compareTo.To))); } ////// Returns a value-based hash code. /// ///the hash value of this Navigation public override int GetHashCode() { return this.RelationshipName.GetHashCode(); } // ------- // Methods // ------- ////// ToString is provided to simplify debugging, etc. /// public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "RelationshipNavigation: ({0},{1},{2})", _relationshipName, _from, _to); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Objects.DataClasses { ////// This class describes a relationship navigation from the /// navigation property on one entity to another entity. It is /// used throughout the collections and refs system to describe a /// relationship and to connect from the navigation property on /// one end of a relationship to the navigation property on the /// other end. /// [Serializable] internal class RelationshipNavigation { // ------------ // Constructors // ------------ ////// Creates a navigation object with the given relationship /// name, role name for the source and role name for the /// destination. /// /// Canonical-space name of the relationship. /// Name of the role which is the source of the navigation. /// Name of the role which is the destination of the navigation. /// The navigation property which is the source of the navigation. /// The navigation property which is the destination of the navigation. internal RelationshipNavigation(string relationshipName, string from, string to, NavigationPropertyAccessor fromAccessor, NavigationPropertyAccessor toAccessor) { EntityUtil.CheckStringArgument(relationshipName, "relationshipName"); EntityUtil.CheckStringArgument(from, "from"); EntityUtil.CheckStringArgument(to, "to"); _relationshipName = relationshipName; _from = from; _to = to; _fromAccessor = fromAccessor; _toAccessor = toAccessor; } // ------ // Fields // ------ // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private readonly string _relationshipName; private readonly string _from; private readonly string _to; [NonSerialized] private RelationshipNavigation _reverse; [NonSerialized] private NavigationPropertyAccessor _fromAccessor; [NonSerialized] private NavigationPropertyAccessor _toAccessor; // ---------- // Properties // ---------- ////// Canonical-space relationship name. /// internal string RelationshipName { get { return _relationshipName; } } ////// Role name for the source of this navigation. /// internal string From { get { return _from; } } ////// Role name for the destination of this navigation. /// internal string To { get { return _to; } } ////// Navigation property name for the destination of this navigation. /// NOTE: There is not a FromPropertyAccessor property on RelationshipNavigation because it is not currently accessed anywhere /// It is only used to calculate the "reverse" RelationshipNavigation. /// internal NavigationPropertyAccessor ToPropertyAccessor { get { return _toAccessor; } } internal bool IsInitialized { get { return _toAccessor != null && _fromAccessor != null; } } internal void InitializeAccessors(NavigationPropertyAccessor fromAccessor, NavigationPropertyAccessor toAccessor) { _fromAccessor = fromAccessor; _toAccessor = toAccessor; } ////// The "reverse" version of this navigation. /// internal RelationshipNavigation Reverse { get { if (_reverse == null || !_reverse.IsInitialized) { // the reverse relationship is exactly like this // one but from & to are switched _reverse = new RelationshipNavigation(_relationshipName, _to, _from, _toAccessor, _fromAccessor); } return _reverse; } } ////// Compares this instance to a given Navigation by their values. /// public override bool Equals(object obj) { RelationshipNavigation compareTo = obj as RelationshipNavigation; return ((this == compareTo) || ((null != this) && (null != compareTo) && (this.RelationshipName == compareTo.RelationshipName) && (this.From == compareTo.From) && (this.To == compareTo.To))); } ////// Returns a value-based hash code. /// ///the hash value of this Navigation public override int GetHashCode() { return this.RelationshipName.GetHashCode(); } // ------- // Methods // ------- ////// ToString is provided to simplify debugging, etc. /// public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "RelationshipNavigation: ({0},{1},{2})", _relationshipName, _from, _to); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
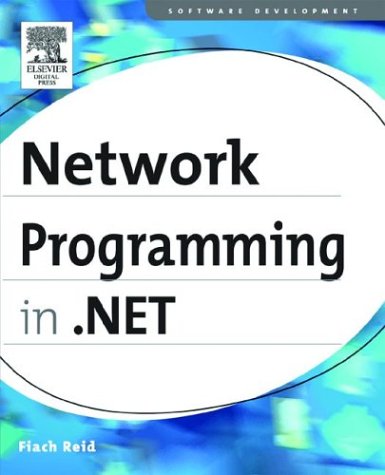
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpNtlmAuthenticationModule.cs
- UnsafeNativeMethodsTablet.cs
- PropertyChangingEventArgs.cs
- ApplicationFileParser.cs
- TriggerActionCollection.cs
- JsonByteArrayDataContract.cs
- UnicodeEncoding.cs
- CompositeDataBoundControl.cs
- UserControl.cs
- UInt32Storage.cs
- ConfigXmlElement.cs
- CodeTypeParameterCollection.cs
- ExportOptions.cs
- ExpressionParser.cs
- BulletedListEventArgs.cs
- SqlExpressionNullability.cs
- ClientScriptManager.cs
- ContextBase.cs
- ImmutableObjectAttribute.cs
- MonthChangedEventArgs.cs
- DoubleMinMaxAggregationOperator.cs
- CodeThrowExceptionStatement.cs
- PrinterResolution.cs
- XmlSchemaNotation.cs
- SetterBaseCollection.cs
- SecurityContext.cs
- SecurityTokenException.cs
- LassoSelectionBehavior.cs
- xml.cs
- EmbeddedObject.cs
- DataGridViewComboBoxColumn.cs
- sitestring.cs
- Literal.cs
- DataTableTypeConverter.cs
- AutomationPatternInfo.cs
- InProcStateClientManager.cs
- MimeMultiPart.cs
- CompositeKey.cs
- StrokeNodeEnumerator.cs
- GAC.cs
- MenuEventArgs.cs
- ResourcePool.cs
- ModelVisual3D.cs
- SmiEventSink_Default.cs
- RSACryptoServiceProvider.cs
- TableLayoutCellPaintEventArgs.cs
- HttpListenerRequest.cs
- DataDesignUtil.cs
- HelpKeywordAttribute.cs
- DoubleAnimationBase.cs
- XmlDataSourceDesigner.cs
- SqlClientPermission.cs
- SeverityFilter.cs
- HttpModule.cs
- DPAPIProtectedConfigurationProvider.cs
- TextEndOfParagraph.cs
- LocalizationParserHooks.cs
- RequiredAttributeAttribute.cs
- DataGridViewMethods.cs
- BasePropertyDescriptor.cs
- X509CertificateClaimSet.cs
- ListViewPagedDataSource.cs
- DetailsViewRow.cs
- TransformerConfigurationWizardBase.cs
- SqlNodeAnnotations.cs
- SqlNodeAnnotation.cs
- QilDataSource.cs
- TrackingExtract.cs
- RefreshResponseInfo.cs
- EdmComplexPropertyAttribute.cs
- EdgeProfileValidation.cs
- FigureParagraph.cs
- NoPersistScope.cs
- UIAgentMonitorHandle.cs
- DrawingVisual.cs
- TrustManagerPromptUI.cs
- DiscoveryEndpointValidator.cs
- SslStream.cs
- Size.cs
- SettingsPropertyCollection.cs
- KeyToListMap.cs
- _NativeSSPI.cs
- XmlILOptimizerVisitor.cs
- DateTimeAutomationPeer.cs
- PackagingUtilities.cs
- SqlRowUpdatedEvent.cs
- EventlogProvider.cs
- SafeCloseHandleCritical.cs
- ScriptMethodAttribute.cs
- JapaneseLunisolarCalendar.cs
- ConnectorRouter.cs
- Matrix3DValueSerializer.cs
- TextSegment.cs
- ResizeBehavior.cs
- EllipseGeometry.cs
- Win32Exception.cs
- ProgressBarAutomationPeer.cs
- BitmapEffectrendercontext.cs
- SettingsPropertyNotFoundException.cs
- AssociatedControlConverter.cs