Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / Win32Exception.cs / 1 / Win32Exception.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; ////// // Code already shipped - safe to place link demand on derived class constructor when base doesn't have it - Suppress message. [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] [Serializable] [SuppressUnmanagedCodeSecurity] public class Win32Exception : ExternalException, ISerializable { ///The exception that is thrown for a Win32 error code. ////// private readonly int nativeErrorCode; ///Represents the Win32 error code associated with this exception. This /// field is read-only. ////// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception() : this(Marshal.GetLastWin32Error()) { } ///Initializes a new instance of the ///class with the last Win32 error /// that occured. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error) : this(error, GetErrorMessage(error)) { } ///Initializes a new instance of the ///class with the specified error. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error, string message) : base(message) { nativeErrorCode = error; } ///Initializes a new instance of the ///class with the specified error and the /// specified detailed description. /// Initializes a new instance of the Exception class with a specified error message. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message ) : this(Marshal.GetLastWin32Error(), message) { } ////// Initializes a new instance of the Exception class with a specified error message and a /// reference to the inner exception that is the cause of this exception. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message, Exception innerException ) : base(message, innerException) { nativeErrorCode = Marshal.GetLastWin32Error(); } protected Win32Exception(SerializationInfo info, StreamingContext context) : base (info, context) { IntSecurity.UnmanagedCode.Demand(); nativeErrorCode = info.GetInt32("NativeErrorCode"); } ////// public int NativeErrorCode { get { return nativeErrorCode; } } private static string GetErrorMessage(int error) { //get the system error message... string errorMsg = ""; StringBuilder sb = new StringBuilder(256); int result = SafeNativeMethods.FormatMessage( SafeNativeMethods.FORMAT_MESSAGE_IGNORE_INSERTS | SafeNativeMethods.FORMAT_MESSAGE_FROM_SYSTEM | SafeNativeMethods.FORMAT_MESSAGE_ARGUMENT_ARRAY, NativeMethods.NullHandleRef, error, 0, sb, sb.Capacity + 1, IntPtr.Zero); if (result != 0) { int i = sb.Length; while (i > 0) { char ch = sb[i - 1]; if (ch > 32 && ch != '.') break; i--; } errorMsg = sb.ToString(0, i); } else { errorMsg ="Unknown error (0x" + Convert.ToString(error, 16) + ")"; } return errorMsg; } // Even though all we're exposing is the nativeErrorCode (which is also available via public property) // it's not a bad idea to have this in place. Later, if more fields are added to this exception, // we won't need to worry about accidentaly exposing them through this interface. [SecurityPermissionAttribute(SecurityAction.Demand,SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("NativeErrorCode", nativeErrorCode); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Represents the Win32 error code associated with this exception. This /// field is read-only. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Text; ////// // Code already shipped - safe to place link demand on derived class constructor when base doesn't have it - Suppress message. [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] [Serializable] [SuppressUnmanagedCodeSecurity] public class Win32Exception : ExternalException, ISerializable { ///The exception that is thrown for a Win32 error code. ////// private readonly int nativeErrorCode; ///Represents the Win32 error code associated with this exception. This /// field is read-only. ////// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception() : this(Marshal.GetLastWin32Error()) { } ///Initializes a new instance of the ///class with the last Win32 error /// that occured. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error) : this(error, GetErrorMessage(error)) { } ///Initializes a new instance of the ///class with the specified error. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception(int error, string message) : base(message) { nativeErrorCode = error; } ///Initializes a new instance of the ///class with the specified error and the /// specified detailed description. /// Initializes a new instance of the Exception class with a specified error message. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message ) : this(Marshal.GetLastWin32Error(), message) { } ////// Initializes a new instance of the Exception class with a specified error message and a /// reference to the inner exception that is the cause of this exception. /// FxCop CA1032: Multiple constructors are required to correctly implement a custom exception. /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public Win32Exception( string message, Exception innerException ) : base(message, innerException) { nativeErrorCode = Marshal.GetLastWin32Error(); } protected Win32Exception(SerializationInfo info, StreamingContext context) : base (info, context) { IntSecurity.UnmanagedCode.Demand(); nativeErrorCode = info.GetInt32("NativeErrorCode"); } ////// public int NativeErrorCode { get { return nativeErrorCode; } } private static string GetErrorMessage(int error) { //get the system error message... string errorMsg = ""; StringBuilder sb = new StringBuilder(256); int result = SafeNativeMethods.FormatMessage( SafeNativeMethods.FORMAT_MESSAGE_IGNORE_INSERTS | SafeNativeMethods.FORMAT_MESSAGE_FROM_SYSTEM | SafeNativeMethods.FORMAT_MESSAGE_ARGUMENT_ARRAY, NativeMethods.NullHandleRef, error, 0, sb, sb.Capacity + 1, IntPtr.Zero); if (result != 0) { int i = sb.Length; while (i > 0) { char ch = sb[i - 1]; if (ch > 32 && ch != '.') break; i--; } errorMsg = sb.ToString(0, i); } else { errorMsg ="Unknown error (0x" + Convert.ToString(error, 16) + ")"; } return errorMsg; } // Even though all we're exposing is the nativeErrorCode (which is also available via public property) // it's not a bad idea to have this in place. Later, if more fields are added to this exception, // we won't need to worry about accidentaly exposing them through this interface. [SecurityPermissionAttribute(SecurityAction.Demand,SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("NativeErrorCode", nativeErrorCode); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Represents the Win32 error code associated with this exception. This /// field is read-only. ///
Link Menu
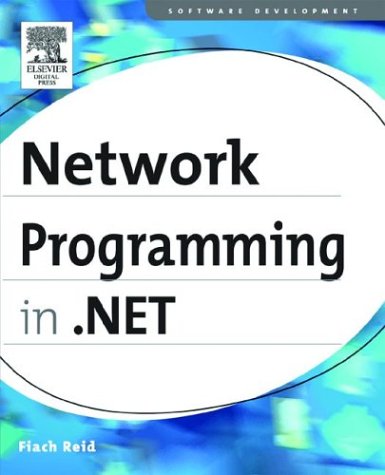
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonXSendMessage.cs
- InstanceData.cs
- NoneExcludedImageIndexConverter.cs
- ToolTipAutomationPeer.cs
- WindowsHyperlink.cs
- BuildProviderAppliesToAttribute.cs
- Binding.cs
- XPathNodeIterator.cs
- TCEAdapterGenerator.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- InkSerializer.cs
- ExpanderAutomationPeer.cs
- PersonalizationState.cs
- Pair.cs
- EventLogLink.cs
- AvTraceDetails.cs
- DataGridViewSortCompareEventArgs.cs
- ColorConverter.cs
- XhtmlBasicCommandAdapter.cs
- BitVector32.cs
- InputBinder.cs
- DataBinder.cs
- SystemIcons.cs
- UnaryQueryOperator.cs
- HierarchicalDataBoundControl.cs
- RNGCryptoServiceProvider.cs
- GeneralTransform3DTo2DTo3D.cs
- DataSourceCacheDurationConverter.cs
- UnsafeNativeMethods.cs
- ObjectStorage.cs
- XmlCodeExporter.cs
- ComponentDispatcher.cs
- ProjectionPruner.cs
- NameValueFileSectionHandler.cs
- XmlDataLoader.cs
- Variant.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- PixelShader.cs
- TreeView.cs
- FontWeights.cs
- ResourceExpression.cs
- Trace.cs
- TrustSection.cs
- DataGridViewLayoutData.cs
- EntityModelSchemaGenerator.cs
- LoginStatusDesigner.cs
- DataGridRow.cs
- Pkcs7Recipient.cs
- LateBoundBitmapDecoder.cs
- XmlNotation.cs
- WindowsListViewItem.cs
- CodeTypeMemberCollection.cs
- ToolStripDropDownItem.cs
- QueryStringParameter.cs
- AssemblyBuilder.cs
- IndexedEnumerable.cs
- RelationshipManager.cs
- SafeRightsManagementQueryHandle.cs
- ProtocolInformationReader.cs
- DataSourceHelper.cs
- ValuePattern.cs
- ActivityXRefConverter.cs
- DebugView.cs
- AccessDataSource.cs
- EnumerableRowCollection.cs
- ExtendedTransformFactory.cs
- HttpWriter.cs
- XmlFormatWriterGenerator.cs
- DataGridViewDataErrorEventArgs.cs
- XsltContext.cs
- Validator.cs
- SizeConverter.cs
- BindingList.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- MulticastOption.cs
- XPathEmptyIterator.cs
- SerialReceived.cs
- DES.cs
- Focus.cs
- HandleValueEditor.cs
- HtmlGenericControl.cs
- SingleStorage.cs
- SoundPlayer.cs
- RegistryKey.cs
- Rectangle.cs
- InitializerFacet.cs
- ConfigurationException.cs
- SafeArrayTypeMismatchException.cs
- ShapeTypeface.cs
- MediaSystem.cs
- SEHException.cs
- FormViewInsertEventArgs.cs
- PersonalizationStateInfoCollection.cs
- SiteMapNodeCollection.cs
- EventTrigger.cs
- ThreadStateException.cs
- XmlUrlResolver.cs
- XmlElement.cs
- BookmarkOptionsHelper.cs
- BindingContext.cs