Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / ProtocolInformationReader.cs / 1 / ProtocolInformationReader.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the class that knows how to deserialize WS-AT protocol information using System; using System.IO; using System.Net; using Microsoft.Transactions.Wsat.Recovery; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Protocol { class ProtocolInformationReader { int httpsPort; string hostName; string nodeName; string basePath; TimeSpan maxTimeout; ProtocolInformationFlags flags; public ProtocolInformationReader(MemoryStream mem) { ReadProtocolInformation(mem); } public int HttpsPort { get { return this.httpsPort; } } public string HostName { get { return this.hostName; } } public string NodeName { get { return this.nodeName; } } public string BasePath { get { return this.basePath; } } public TimeSpan MaxTimeout { get { return this.maxTimeout; } } public bool IssuedTokensEnabled { get { return (flags & ProtocolInformationFlags.IssuedTokensEnabled) != 0; } } public bool NetworkInboundAccess { get { return (flags & ProtocolInformationFlags.NetworkInboundAccess) != 0; } } public bool NetworkOutboundAccess { get { return (flags & ProtocolInformationFlags.NetworkOutboundAccess) != 0; } } public bool NetworkClientAccess { get { return (flags & ProtocolInformationFlags.NetworkClientAccess) != 0; } } public bool IsClustered { get { return (flags & ProtocolInformationFlags.IsClustered) != 0; } } bool isV10Enabled = false; public bool IsV10Enabled { get { return this.isV10Enabled; } } bool isV11Enabled = false; public bool IsV11Enabled { get { return this.isV11Enabled; } } void ReadProtocolInformation(MemoryStream mem) { // We assume that all extended whereabouts emitted under the PluggableProtocol guids for versions // 1.0 or 1.1 start with the following set of data (version 1.1) // { version(major,minor), flags, httpsPort, maxTimeout, hostName, basePath, nodeName } // Read major version ProtocolInformationMajorVersion version = (ProtocolInformationMajorVersion)mem.ReadByte(); if (version != ProtocolInformationMajorVersion.v1) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoUnsupportedVersion, version))); } // Read minor version // We don't assert the minor version. In the future, by incrementing the minor version, we will be able // to maintain compatibility while adding new information onto the end of the stream ProtocolInformationMinorVersion minorVersion = (ProtocolInformationMinorVersion)mem.ReadByte(); // Begin reading protocol info version 1.1 // Flags this.flags = (ProtocolInformationFlags)mem.ReadByte(); CheckFlags(this.flags); // Https port this.httpsPort = SerializationUtils.ReadInt(mem); if (this.httpsPort < IPEndPoint.MinPort || this.httpsPort > IPEndPoint.MaxPort) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidHttpsPort, this.httpsPort))); } // Max timeout this.maxTimeout = SerializationUtils.ReadTimeout(mem); if (this.maxTimeout < TimeSpan.Zero) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidMaxTimeout, this.maxTimeout))); } // HostName this.hostName = SerializationUtils.ReadString(mem); if (string.IsNullOrEmpty(this.hostName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidHostName))); } // BasePath this.basePath = SerializationUtils.ReadString(mem); if (string.IsNullOrEmpty(this.basePath)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidBasePath))); } // NodeName this.nodeName = SerializationUtils.ReadString(mem); if (string.IsNullOrEmpty(this.nodeName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidNodeName))); } // End of version 1.1 // We assume that all minor versions >= than 2 whereabouts have a short field describing the // supported protocol versions byte minMinorVersion = 2; if((byte)minorVersion >= minMinorVersion) { ProtocolVersion supportedProtocols = (ProtocolVersion)SerializationUtils.ReadUShort(mem); // check if coordinator supports WSAT 1.0 if ((supportedProtocols & ProtocolVersion.Version10) != 0) { this.isV10Enabled = true; } // check if coordinator supports WSAT 1.1 if ((supportedProtocols & ProtocolVersion.Version11) != 0) { this.isV11Enabled = true; } } else { this.isV10Enabled = true; } } void CheckFlags(ProtocolInformationFlags flags) { const ProtocolInformationFlags allFlags = ProtocolInformationFlags.IssuedTokensEnabled | ProtocolInformationFlags.NetworkClientAccess | ProtocolInformationFlags.NetworkInboundAccess | ProtocolInformationFlags.NetworkOutboundAccess | ProtocolInformationFlags.IsClustered; if ((flags | allFlags) != allFlags) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidFlags, flags))); } // Either NetworkInboundAccess or NetworkOutboundAccess must be set const ProtocolInformationFlags inOutFlags = ProtocolInformationFlags.NetworkInboundAccess | ProtocolInformationFlags.NetworkOutboundAccess; if ((flags & inOutFlags) == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.ProtocolInfoInvalidFlags, flags))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
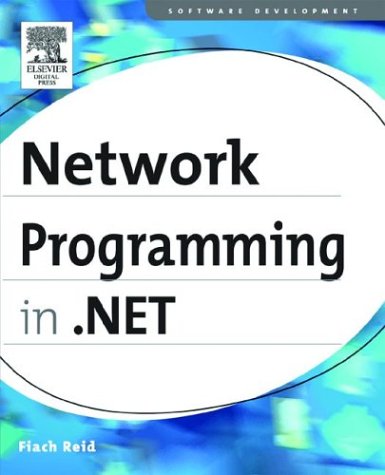
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStrip.cs
- IDataContractSurrogate.cs
- BinaryObjectInfo.cs
- NTAccount.cs
- WsiProfilesElement.cs
- XmlLoader.cs
- MessagePropertyDescription.cs
- TextEditorCharacters.cs
- CanExpandCollapseAllConverter.cs
- DefaultTypeArgumentAttribute.cs
- SectionXmlInfo.cs
- MarkupWriter.cs
- WebConfigurationHostFileChange.cs
- DiscardableAttribute.cs
- TabletCollection.cs
- StringBlob.cs
- AVElementHelper.cs
- ReceiveActivityValidator.cs
- EdmToObjectNamespaceMap.cs
- GroupBox.cs
- SectionVisual.cs
- LinqDataView.cs
- RepeaterItemEventArgs.cs
- RefreshPropertiesAttribute.cs
- RegisteredArrayDeclaration.cs
- OleDbCommandBuilder.cs
- ObjectCacheHost.cs
- MenuBindingsEditor.cs
- UrlPath.cs
- Assembly.cs
- DataPagerFieldItem.cs
- CodeExporter.cs
- DefaultPrintController.cs
- AnonymousIdentificationModule.cs
- ForEachDesigner.xaml.cs
- RoleGroup.cs
- BinaryKeyIdentifierClause.cs
- Enum.cs
- Container.cs
- TextPenaltyModule.cs
- ColumnResizeAdorner.cs
- ElementUtil.cs
- SchemaManager.cs
- KernelTypeValidation.cs
- DetailsViewRow.cs
- UserControlBuildProvider.cs
- LinkButton.cs
- XmlSchemaSequence.cs
- EdmSchemaAttribute.cs
- ReadOnlyState.cs
- ArrayHelper.cs
- DesignerSerializationOptionsAttribute.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- WebEventTraceProvider.cs
- ObservableDictionary.cs
- XDRSchema.cs
- DoubleAnimationUsingPath.cs
- DeleteIndexBinder.cs
- TemplateBindingExpression.cs
- ProxyElement.cs
- GifBitmapDecoder.cs
- CodeIdentifiers.cs
- Rect3DValueSerializer.cs
- SparseMemoryStream.cs
- RefreshEventArgs.cs
- CompositeTypefaceMetrics.cs
- SubstitutionDesigner.cs
- SimpleType.cs
- FontStyles.cs
- MemberPath.cs
- PropertyGridEditorPart.cs
- ConnectionConsumerAttribute.cs
- ListenerElementsCollection.cs
- CommandCollectionEditor.cs
- CodeDomLoader.cs
- TextEvent.cs
- listviewsubitemcollectioneditor.cs
- ProxyManager.cs
- SortKey.cs
- RuntimeHelpers.cs
- XPathNode.cs
- StreamSecurityUpgradeInitiatorAsyncResult.cs
- DynamicEntity.cs
- RootAction.cs
- securitycriticaldataClass.cs
- COM2ExtendedTypeConverter.cs
- SqlConnectionString.cs
- CustomAttribute.cs
- TimeoutValidationAttribute.cs
- PreviewKeyDownEventArgs.cs
- ValidationRuleCollection.cs
- StyleBamlRecordReader.cs
- ExportOptions.cs
- Scene3D.cs
- ConnectionConsumerAttribute.cs
- SessionEndedEventArgs.cs
- XmlParser.cs
- UrlMappingsSection.cs
- DataStreamFromComStream.cs
- Attributes.cs