Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / ColumnResizeAdorner.cs / 1 / ColumnResizeAdorner.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Adorner for column resize. // // History: // 08/19/2004 : ghermann - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Controls; using System.Windows.Controls.Primitives; using MS.Win32; using MS.Internal; namespace System.Windows.Documents.Internal { internal class ColumnResizeAdorner : Adorner { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// C'tor for adorner /// /// /// FramwerokElement with TextView to which this element is attached /// as adorner. /// internal ColumnResizeAdorner(UIElement scope) : base(scope) { Debug.Assert(scope != null); // position _pen = new Pen(new SolidColorBrush(Colors.LightSlateGray), 2.0); _x = Double.NaN; _top = Double.NaN; _height = Double.NaN; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Add a transform so that the adorner is in right spot. /// /// /// The transform applied to the object the adorner adorns /// ////// Transform to apply to the adorner /// public override GeneralTransform GetDesiredTransform(GeneralTransform transform) { GeneralTransformGroup group; TranslateTransform translation; group = new GeneralTransformGroup(); translation = new TranslateTransform(_x, _top); group.Children.Add(translation); if (transform != null) { group.Children.Add(transform); } return group; } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods protected override void OnRender(DrawingContext drawingContext) { // Render as a 2 pixel wide rect, one pixel in each bordering char bounding box. drawingContext.DrawLine(_pen, new Point(0, 0), new Point(0, _height)); } #endregion Protected Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Updates position for adorner. /// /// /// internal void Update(double newX) { if(_x != newX) { _x = newX; AdornerLayer adornerLayer; adornerLayer = VisualTreeHelper.GetParent(this) as AdornerLayer; if (adornerLayer != null) { // It may be null when TextBox is detached from a tree adornerLayer.Update(AdornedElement); adornerLayer.InvalidateVisual(); } } } internal void Initialize(UIElement renderScope, double xPos, double yPos, double height) { Debug.Assert(_adornerLayer == null, "Attempt to overwrite existing AdornerLayer!"); _adornerLayer = AdornerLayer.GetAdornerLayer(renderScope); if (_adornerLayer != null) { _adornerLayer.Add(this); } _x = xPos; _top = yPos; _height = height; } internal void Uninitialize() { if (_adornerLayer != null) { _adornerLayer.Remove(this); _adornerLayer = null; } } #endregion Internal methods //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods #endregion Private methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // x position private double _x; // top position private double _top; // height private double _height; private Pen _pen; // Cached adornerlayer private AdornerLayer _adornerLayer; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Adorner for column resize. // // History: // 08/19/2004 : ghermann - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Controls; using System.Windows.Controls.Primitives; using MS.Win32; using MS.Internal; namespace System.Windows.Documents.Internal { internal class ColumnResizeAdorner : Adorner { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// C'tor for adorner /// /// /// FramwerokElement with TextView to which this element is attached /// as adorner. /// internal ColumnResizeAdorner(UIElement scope) : base(scope) { Debug.Assert(scope != null); // position _pen = new Pen(new SolidColorBrush(Colors.LightSlateGray), 2.0); _x = Double.NaN; _top = Double.NaN; _height = Double.NaN; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Add a transform so that the adorner is in right spot. /// /// /// The transform applied to the object the adorner adorns /// ////// Transform to apply to the adorner /// public override GeneralTransform GetDesiredTransform(GeneralTransform transform) { GeneralTransformGroup group; TranslateTransform translation; group = new GeneralTransformGroup(); translation = new TranslateTransform(_x, _top); group.Children.Add(translation); if (transform != null) { group.Children.Add(transform); } return group; } #endregion Public Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods protected override void OnRender(DrawingContext drawingContext) { // Render as a 2 pixel wide rect, one pixel in each bordering char bounding box. drawingContext.DrawLine(_pen, new Point(0, 0), new Point(0, _height)); } #endregion Protected Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Updates position for adorner. /// /// /// internal void Update(double newX) { if(_x != newX) { _x = newX; AdornerLayer adornerLayer; adornerLayer = VisualTreeHelper.GetParent(this) as AdornerLayer; if (adornerLayer != null) { // It may be null when TextBox is detached from a tree adornerLayer.Update(AdornedElement); adornerLayer.InvalidateVisual(); } } } internal void Initialize(UIElement renderScope, double xPos, double yPos, double height) { Debug.Assert(_adornerLayer == null, "Attempt to overwrite existing AdornerLayer!"); _adornerLayer = AdornerLayer.GetAdornerLayer(renderScope); if (_adornerLayer != null) { _adornerLayer.Add(this); } _x = xPos; _top = yPos; _height = height; } internal void Uninitialize() { if (_adornerLayer != null) { _adornerLayer.Remove(this); _adornerLayer = null; } } #endregion Internal methods //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods #endregion Private methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // x position private double _x; // top position private double _top; // height private double _height; private Pen _pen; // Cached adornerlayer private AdornerLayer _adornerLayer; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
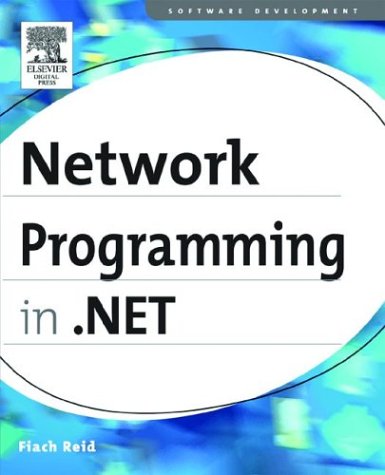
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlEncodedRawTextWriter.cs
- WeakKeyDictionary.cs
- AssociationType.cs
- CodeTypeParameterCollection.cs
- Vector3DAnimationUsingKeyFrames.cs
- TextServicesDisplayAttribute.cs
- ContentValidator.cs
- ColorPalette.cs
- regiisutil.cs
- EventEntry.cs
- DynamicRendererThreadManager.cs
- RectangleConverter.cs
- RequestTimeoutManager.cs
- ColorConvertedBitmap.cs
- RewritingValidator.cs
- CodeTypeReferenceCollection.cs
- OperationResponse.cs
- WebPartAddingEventArgs.cs
- DataGridViewRowPostPaintEventArgs.cs
- CompositeScriptReferenceEventArgs.cs
- TableAdapterManagerNameHandler.cs
- ClientScriptItemCollection.cs
- ToolStripSeparatorRenderEventArgs.cs
- EntityDataSourceDesigner.cs
- XsltException.cs
- MultiSelectRootGridEntry.cs
- ExpressionBuilder.cs
- DataGridPreparingCellForEditEventArgs.cs
- InlinedAggregationOperatorEnumerator.cs
- HttpVersion.cs
- KeySplineConverter.cs
- PagesChangedEventArgs.cs
- ClientSettingsProvider.cs
- ServiceManager.cs
- TypeSystem.cs
- AppDomainFactory.cs
- InitializerFacet.cs
- DebuggerAttributes.cs
- FillBehavior.cs
- TreeNodeBindingCollection.cs
- AbsoluteQuery.cs
- MarshalDirectiveException.cs
- TemplateParser.cs
- VariableQuery.cs
- SecuritySessionClientSettings.cs
- BlurBitmapEffect.cs
- NotifyIcon.cs
- CodeIdentifier.cs
- ElementHost.cs
- SchemaDeclBase.cs
- SqlEnums.cs
- DecoratedNameAttribute.cs
- _AutoWebProxyScriptWrapper.cs
- XmlWrappingReader.cs
- HitTestResult.cs
- SoapSchemaImporter.cs
- SQLSingle.cs
- clipboard.cs
- XmlSchemaComplexContentRestriction.cs
- WebPartPersonalization.cs
- SevenBitStream.cs
- PropertyConverter.cs
- PartitionResolver.cs
- CharConverter.cs
- DependencyPropertyKey.cs
- MetadataHelper.cs
- EventLogPermissionEntryCollection.cs
- SqlCacheDependencyDatabase.cs
- OverrideMode.cs
- ResXResourceSet.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ToolStripDropDownItem.cs
- IResourceProvider.cs
- PropertyTabChangedEvent.cs
- JsonQueryStringConverter.cs
- DebuggerService.cs
- DataReceivedEventArgs.cs
- DataRecord.cs
- ConfigXmlReader.cs
- WizardForm.cs
- CustomGrammar.cs
- FunctionNode.cs
- SerializationInfoEnumerator.cs
- EntityDataSourceChangingEventArgs.cs
- CodeActivityContext.cs
- DesignerAutoFormatCollection.cs
- WhileDesigner.cs
- HiddenField.cs
- WindowsProgressbar.cs
- PanelStyle.cs
- NgenServicingAttributes.cs
- LayoutTableCell.cs
- PersonalizableAttribute.cs
- ItemCheckEvent.cs
- Utils.cs
- SqlDataSourceStatusEventArgs.cs
- ListDictionary.cs
- ExceptionRoutedEventArgs.cs
- WebConvert.cs
- FilteredAttributeCollection.cs