Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Media / XamlSerializationHelper.cs / 1 / XamlSerializationHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Utilities for converting types to custom Binary format // // History: // 11/09/05 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Globalization; // CultureInfo #if PRESENTATION_CORE using MS.Internal.PresentationCore; // FriendAccessAllowed #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #endif #if PBTCOMPILER using System.Collections.Generic; namespace MS.Internal.Markup #else using System.Windows; using System.Windows.Media ; using System.Windows.Media.Media3D; namespace MS.Internal.Media #endif { #if PBTCOMPILER // // Internal class used during serialization of Point3D, or Vectors. // // We define this struct so that we can create a collection of types during serialization. // If we used defined avalon types, like Point & Vector, we'd have to bring these into PBT // with a compiler change everytime these types are changed. // // The type is called "ThreeDoubles" to make clear what it is and what it's for. // // If either Vector3D or Point3D changes - we will need to change this code. // internal class ThreeDoublesMarkup { internal ThreeDoublesMarkup( double X, double Y, double Z) { _x = X; _y = Y; _z = Z; } internal double X { get { return _x; } } internal double Y { get { return _y; } } internal double Z { get { return _z; } } double _x ; double _y ; double _z ; } internal struct Point { internal Point(double x, double y) { _x = x; _y = y; } internal double X { set { _x = value; } get { return _x; } } internal double Y { set { _y = value; } get { return _y; } } private double _x; private double _y; } internal struct Size { internal Size(double width, double height) { _width = width; _height = height; } internal double Width { set { _width = value; } get { return _width; } } internal double Height { set { _height = value; } get { return _height; } } private double _width; private double _height; } #endif internal static class XamlSerializationHelper { // ===================================================== // // All PBT specific types and methods go here. // // ===================================================== internal enum SerializationFloatType : byte { Unknown = 0, Zero = 1, One = 2, MinusOne = 3, ScaledInteger = 4, Double = 5, Other } ////// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// #if !PBTCOMPILER [FriendAccessAllowed] // Built into Core, also used by Framework. #endif internal static bool SerializePoint3D(BinaryWriter writer, string stringValues) { #if PBTCOMPILER Listpoint3Ds = ParseThreeDoublesCollection( stringValues, CultureInfo.GetCultureInfo("en-us") ) ; ThreeDoublesMarkup curPoint; #else Point3DCollection point3Ds = Point3DCollection.Parse( stringValues ) ; Point3D curPoint ; #endif // Write out the size. writer.Write( ( uint ) point3Ds.Count ) ; // Write out the doubles. for ( int i = 0; i < point3Ds.Count ; i ++ ) { curPoint = point3Ds[i] ; WriteDouble( writer, curPoint.X); WriteDouble( writer, curPoint.Y); WriteDouble( writer, curPoint.Z); } return true ; } /// /// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// #if !PBTCOMPILER [FriendAccessAllowed] // Built into Core, also used by Framework. #endif internal static bool SerializeVector3D(BinaryWriter writer, string stringValues) { #if PBTCOMPILER Listpoints = ParseThreeDoublesCollection( stringValues, CultureInfo.GetCultureInfo("en-us") ) ; ThreeDoublesMarkup curPoint; #else Vector3DCollection points = Vector3DCollection.Parse( stringValues ) ; Vector3D curPoint ; #endif // Write out the size. writer.Write( ( uint ) points.Count ) ; // Write out the doubles. for ( int i = 0; i < points.Count ; i ++ ) { curPoint = points[ i ] ; WriteDouble( writer, curPoint.X); WriteDouble( writer, curPoint.Y); WriteDouble( writer, curPoint.Z); } return true ; } /// /// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// #if !PBTCOMPILER [FriendAccessAllowed] // Built into Core, also used by Framework. #endif internal static bool SerializePoint(BinaryWriter writer, string stringValue) { #if PBTCOMPILER Listpoints = ParsePointCollection( stringValue, CultureInfo.GetCultureInfo("en-us") ) ; Point curPoint; #else PointCollection points = PointCollection.Parse( stringValue ) ; Point curPoint ; #endif // Write out the size. writer.Write( ( uint ) points.Count ) ; // Write out the doubles. for ( int i = 0; i < points.Count ; i ++ ) { curPoint = points[ i ] ; WriteDouble( writer, curPoint.X); WriteDouble( writer, curPoint.Y); } return true ; } private const double scaleFactor = 1000000 ; // approx == 2^20 private const double inverseScaleFactor = 0.000001 ; // approx = 1 / 2^20 // // Write a double into our internal binary format // // // The format is : // ( <4 bytes for scaledinteger> | < 8 bytes for double> ) // internal static void WriteDouble( BinaryWriter writer, Double value ) { if ( value == 0.0 ) { writer.Write( (byte) SerializationFloatType.Zero ) ; } else if ( value == 1.0 ) { writer.Write( (byte) SerializationFloatType.One ) ; } else if ( value == -1.0 ) { writer.Write( (byte) SerializationFloatType.MinusOne ) ; } else { int intValue = 0 ; if ( CanConvertToInteger( value, ref intValue ) ) { writer.Write( (byte) SerializationFloatType.ScaledInteger ) ; writer.Write( intValue ) ; } else { writer.Write( (byte) SerializationFloatType.Double ) ; writer.Write( value ) ; } } } #if !PBTCOMPILER // // Read a double from our internal binary format. // We assume that the binary reader is at the start of a byte. // internal static double ReadDouble( BinaryReader reader ) { SerializationFloatType type = ( SerializationFloatType ) reader.ReadByte(); switch( type ) { case SerializationFloatType.Zero : return 0.0 ; case SerializationFloatType.One : return 1.0 ; case SerializationFloatType.MinusOne : return -1.0 ; case SerializationFloatType.ScaledInteger : return ReadScaledInteger( reader ); case SerializationFloatType.Double : return reader.ReadDouble(); default: throw new ArgumentException(SR.Get(SRID.FloatUnknownBamlType)); } } internal static double ReadScaledInteger(BinaryReader reader ) { double value = (double) reader.ReadInt32(); value = value * inverseScaleFactor ; return value ; } #endif #if PBTCOMPILER /// /// Parse - returns an instance converted from the provided string. /// string with Point3DCollection data /// IFormatprovider for processing string /// private static ListParseThreeDoublesCollection(string source, IFormatProvider formatProvider) { TokenizerHelper th = new TokenizerHelper(source, formatProvider); List resource = new List ( source.Length/ 8 ) ; // SWAG the length of the collection. ThreeDoublesMarkup value; while (th.NextToken()) { value = new ThreeDoublesMarkup( Convert.ToDouble(th.GetCurrentToken(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); resource.Add(value); } return resource; } /// /// Parse - returns an instance converted from the provided string. /// string with Point3DCollection data /// IFormatprovider for processing string /// private static ListParsePointCollection(string source, IFormatProvider formatProvider) { TokenizerHelper th = new TokenizerHelper(source, formatProvider); List resource = new List (source.Length/ 8 ); // SWAG the length of the collection. Point value; while (th.NextToken()) { value = new Point( Convert.ToDouble(th.GetCurrentToken(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider) ); resource.Add(value); } return resource; } #endif // // Can we convert this double to a "scaled integer" // // We multiply by approx 2^20 - see if the result is either // - greater than maxInt // - if there is a non-numeric integer remaining // // as a result this routine will convert doubles with six-digits precision between +/- 2048 // internal static bool CanConvertToInteger( Double doubleValue , ref int intValue ) { double scaledValue ; double scaledInteger ; scaledValue = doubleValue * scaleFactor ; scaledInteger = Math.Floor( scaledValue ) ; if ( !( scaledInteger <= Int32.MaxValue ) // equivalent to scaledInteger > MaxValue, but take care of NaN. || !( scaledInteger >= Int32.MinValue ) ) // equivalent to scaledInteger < Minvalue but take care of NaN. { return false ; } else if ( ( scaledValue - scaledInteger ) > Double.Epsilon ) { return false ; } else { intValue = (int) scaledValue ; return true ; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
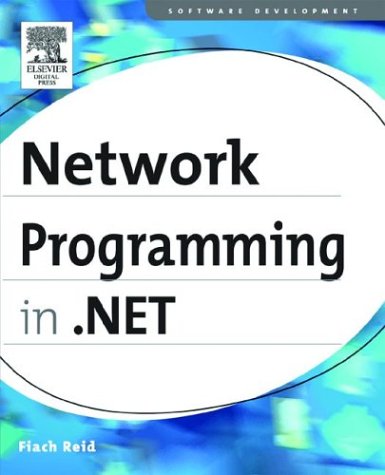
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpHeaderCollection.cs
- HelpProvider.cs
- SqlDelegatedTransaction.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- ExtensionFile.cs
- TypedDatasetGenerator.cs
- ListSortDescription.cs
- PersonalizationEntry.cs
- PathSegment.cs
- ElementsClipboardData.cs
- MsmqBindingBase.cs
- SqlProcedureAttribute.cs
- PeerCollaborationPermission.cs
- ShutDownListener.cs
- WebPartEditVerb.cs
- XPathNodeInfoAtom.cs
- ProviderConnectionPointCollection.cs
- SqlTypesSchemaImporter.cs
- InternalEnumValidatorAttribute.cs
- FunctionDescription.cs
- AssemblyHelper.cs
- AssertSection.cs
- X509PeerCertificateAuthentication.cs
- DesignerCategoryAttribute.cs
- OrderedHashRepartitionEnumerator.cs
- BindingExpression.cs
- XamlReaderHelper.cs
- XmlDataImplementation.cs
- StructuralCache.cs
- ImportContext.cs
- RenamedEventArgs.cs
- LicenseException.cs
- SqlInfoMessageEvent.cs
- WindowsTab.cs
- QueryCacheManager.cs
- METAHEADER.cs
- ApplicationId.cs
- SocketPermission.cs
- sitestring.cs
- Vector3DAnimation.cs
- RotateTransform.cs
- AnnotationAdorner.cs
- WebServiceData.cs
- ScrollEventArgs.cs
- DocumentOrderQuery.cs
- DesignerTransaction.cs
- MetadataItemCollectionFactory.cs
- ScriptingJsonSerializationSection.cs
- ExclusiveTcpListener.cs
- SiteMapNodeCollection.cs
- XPathNodePointer.cs
- PerformanceCounterManager.cs
- PointConverter.cs
- SpellerInterop.cs
- DragEvent.cs
- SystemResources.cs
- GACIdentityPermission.cs
- WebPartTransformerCollection.cs
- ScopeCompiler.cs
- StorageRoot.cs
- RootProjectionNode.cs
- GeneralTransform3DTo2DTo3D.cs
- MatrixTransform3D.cs
- FreezableOperations.cs
- AbandonedMutexException.cs
- AddingNewEventArgs.cs
- BindingListCollectionView.cs
- oledbmetadatacollectionnames.cs
- IERequestCache.cs
- _ConnectOverlappedAsyncResult.cs
- DesignerAdapterUtil.cs
- TemplateColumn.cs
- CodeSnippetCompileUnit.cs
- ContentValidator.cs
- XmlWriterSettings.cs
- VersionUtil.cs
- DbModificationCommandTree.cs
- WebBrowserEvent.cs
- GZipDecoder.cs
- SafeRegistryHandle.cs
- WorkflowDesigner.cs
- Point4DConverter.cs
- ReadWriteSpinLock.cs
- UpnEndpointIdentityExtension.cs
- ConstNode.cs
- SessionStateUtil.cs
- PolicyChain.cs
- BookmarkUndoUnit.cs
- OrderedDictionary.cs
- PointConverter.cs
- TokenizerHelper.cs
- ScriptManagerProxy.cs
- BamlReader.cs
- ChangeBlockUndoRecord.cs
- GridViewColumn.cs
- Flattener.cs
- StateChangeEvent.cs
- RNGCryptoServiceProvider.cs
- SelectorAutomationPeer.cs
- Helper.cs