Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusEventArgs.cs / 1 / StylusEventArgs.cs
using System; using System.Collections; using System.Windows.Media; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The StylusEventArgs class provides access to the logical /// Stylus device for all derived event args. /// public class StylusEventArgs : InputEventArgs { ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// public StylusEventArgs(StylusDevice stylus, int timestamp) : base(stylus, timestamp) { if( stylus == null ) { throw new System.ArgumentNullException("stylus"); } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the stylus device associated with this /// event. /// public StylusDevice StylusDevice { get { return (StylusDevice)this.Device; } } ///////////////////////////////////////////////////////////////////// ////// Calculates the position of the stylus relative to a particular element. /// public Point GetPosition(IInputElement relativeTo) { return StylusDevice.GetPosition(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Indicates the stylus is not touching the surface. /// public bool InAir { get { return StylusDevice.InAir; } } ///////////////////////////////////////////////////////////////////// ////// Indicates stylusDevice is in the inverted state. /// public bool Inverted { get { return StylusDevice.Inverted; } } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo) { return StylusDevice.GetStylusPoints(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo, StylusPointDescription subsetToReformatTo) { return StylusDevice.GetStylusPoints(relativeTo, subsetToReformatTo); } ///////////////////////////////////////////////////////////////////// ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { StylusEventHandler handler = (StylusEventHandler) genericHandler; handler(genericTarget, this); } ///////////////////////////////////////////////////////////////////// internal RawStylusInputReport InputReport { get { return _inputReport; } set { _inputReport = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _inputReport; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Windows.Media; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The StylusEventArgs class provides access to the logical /// Stylus device for all derived event args. /// public class StylusEventArgs : InputEventArgs { ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// public StylusEventArgs(StylusDevice stylus, int timestamp) : base(stylus, timestamp) { if( stylus == null ) { throw new System.ArgumentNullException("stylus"); } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the stylus device associated with this /// event. /// public StylusDevice StylusDevice { get { return (StylusDevice)this.Device; } } ///////////////////////////////////////////////////////////////////// ////// Calculates the position of the stylus relative to a particular element. /// public Point GetPosition(IInputElement relativeTo) { return StylusDevice.GetPosition(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Indicates the stylus is not touching the surface. /// public bool InAir { get { return StylusDevice.InAir; } } ///////////////////////////////////////////////////////////////////// ////// Indicates stylusDevice is in the inverted state. /// public bool Inverted { get { return StylusDevice.Inverted; } } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo) { return StylusDevice.GetStylusPoints(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo, StylusPointDescription subsetToReformatTo) { return StylusDevice.GetStylusPoints(relativeTo, subsetToReformatTo); } ///////////////////////////////////////////////////////////////////// ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { StylusEventHandler handler = (StylusEventHandler) genericHandler; handler(genericTarget, this); } ///////////////////////////////////////////////////////////////////// internal RawStylusInputReport InputReport { get { return _inputReport; } set { _inputReport = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _inputReport; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
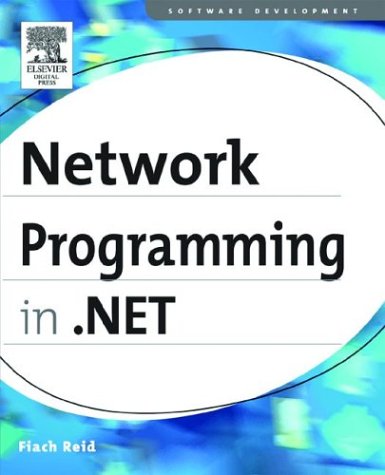
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiscoveryMessageSequence11.cs
- AnimationClock.cs
- EntityDataSourceReferenceGroup.cs
- WinFormsSpinner.cs
- SoapExtensionReflector.cs
- DBSchemaTable.cs
- WsdlInspector.cs
- NetworkInformationException.cs
- HttpStaticObjectsCollectionBase.cs
- StateValidator.cs
- XmlToDatasetMap.cs
- XmlSchemaSimpleTypeList.cs
- documentation.cs
- NumberAction.cs
- DocumentPageHost.cs
- DesignerForm.cs
- Brush.cs
- compensatingcollection.cs
- EncoderBestFitFallback.cs
- CodeSubDirectory.cs
- UDPClient.cs
- WebUtility.cs
- HttpRuntimeSection.cs
- WindowsTitleBar.cs
- DeferredElementTreeState.cs
- ConfigurationValidatorAttribute.cs
- TcpClientChannel.cs
- DiagnosticTraceRecords.cs
- PolicyFactory.cs
- AppDomainUnloadedException.cs
- Emitter.cs
- WindowsListViewGroup.cs
- XPathDocumentNavigator.cs
- ToolStripItemClickedEventArgs.cs
- SoapCodeExporter.cs
- ConfigurationCollectionAttribute.cs
- AtomContentProperty.cs
- ListViewSelectEventArgs.cs
- COM2ComponentEditor.cs
- RegionIterator.cs
- SafeArrayTypeMismatchException.cs
- KeyPullup.cs
- _AuthenticationState.cs
- Group.cs
- XmlDataSourceNodeDescriptor.cs
- WebDisplayNameAttribute.cs
- CurrencyManager.cs
- ImageClickEventArgs.cs
- RenderData.cs
- LoginView.cs
- TextServicesProperty.cs
- httpapplicationstate.cs
- IssuedTokensHeader.cs
- IsolatedStorageFile.cs
- CacheDependency.cs
- GridViewRowPresenter.cs
- QueryExtender.cs
- TimeSpanStorage.cs
- OdbcCommandBuilder.cs
- CustomCategoryAttribute.cs
- SubqueryRules.cs
- CommentAction.cs
- XmlDesigner.cs
- OleDbInfoMessageEvent.cs
- ByteStack.cs
- XmlExpressionDumper.cs
- MailHeaderInfo.cs
- BrushConverter.cs
- HTTPNotFoundHandler.cs
- X509Certificate2.cs
- XmlSerializationReader.cs
- PeerMessageDispatcher.cs
- DataGridItem.cs
- QilGeneratorEnv.cs
- TogglePattern.cs
- COAUTHINFO.cs
- TemplatedMailWebEventProvider.cs
- TypedRowHandler.cs
- DataShape.cs
- MbpInfo.cs
- DependencyPropertyValueSerializer.cs
- ViewManager.cs
- TextEditorParagraphs.cs
- PtsCache.cs
- ContractComponent.cs
- UriSectionData.cs
- ListViewGroup.cs
- PropertyChangedEventArgs.cs
- sqlstateclientmanager.cs
- XNodeNavigator.cs
- WebPartConnectionsEventArgs.cs
- ExpressionPrefixAttribute.cs
- GatewayIPAddressInformationCollection.cs
- TextServicesLoader.cs
- CodeTypeMember.cs
- ControlIdConverter.cs
- RuleSettings.cs
- BitStream.cs
- XmlReaderSettings.cs
- AssemblyBuilder.cs