Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Instrumentation / ManagementInstaller.cs / 1305376 / ManagementInstaller.cs
namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Configuration.Install; using System.Text; using System.IO; using System.Runtime.Versioning; using System.Globalization; using System.Security.Permissions; ////// ///Installs instrumented assemblies. Include an instance of this installer class in the project installer for /// an assembly that includes instrumentation. ////// ///If this is the only installer for your application, you may use the helper class ////// provided in this namespace. /// public class ManagementInstaller : Installer { // private static bool helpPrinted = false; ///If you have a master project installer for your /// project, add the following code to your project installers constructor: ///// Instantiate installer for assembly. /// ManagementInstaller managementInstaller = new ManagementInstaller(); /// /// // Add installer to collection. /// Installers.Add(managementInstaller); ///
///'Instantiate installer for assembly. /// Dim managementInstaller As New ManagementInstaller() /// /// 'Add installer to collection. /// Installers.Add(managementInstaller) ///
////// ///Gets or sets installer options for this class. ////// public override string HelpText { get { if (helpPrinted) return base.HelpText; else { helpPrinted = true; // return Res.GetString(Res.HelpText) + "\r\n" + base.HelpText; // StringBuilder help = new StringBuilder(); help.Append("/MOF=[filename]\r\n"); help.Append(" " + RC.GetString("FILETOWRITE_MOF")+"\r\n\r\n"); // // [RAID: 123895] // If the force parameter is present, we update registration information independent if it already // exists. // help.Append ( "/Force or /F\r\n" ) ; help.Append(" " + RC.GetString("FORCE_UPDATE")); return help.ToString() + base.HelpText; } } } ///The help text for all the installers in the installer collection, including /// the description of what each installer does and the command-line options (for /// the installation program) that can be passed to and understood by each /// installer. ////// /// The state of the assembly. [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public override void Install(IDictionary savedState) { // Demand IO permission required for LoadFrom FXCop 191096 FileIOPermission ioPermission = new FileIOPermission(FileIOPermissionAccess.Read, (string)Context.Parameters["assemblypath"]); ioPermission.Demand(); base.Install(savedState); // Context.LogMessage(RC.GetString("WMISCHEMA_INSTALLATIONSTART")); string assemblyPath = Context.Parameters["assemblypath"]; Assembly assembly = Assembly.LoadFrom(assemblyPath); SchemaNaming naming = SchemaNaming.GetSchemaNaming(assembly); // // We always use the full version number for Whidbey. // naming.DecoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyFullVersion(assembly); // See if this assembly provides instrumentation if(null == naming) return; // // [RAID: 123895] // If the force parameter is present, we update registration information independent if it already // exists. // if( ( naming.IsAssemblyRegistered() == false ) || ( Context.Parameters.ContainsKey ( "force" ) ) || ( Context.Parameters.ContainsKey ( "f" ) ) ) { Context.LogMessage(RC.GetString("REGESTRING_ASSEMBLY") + " " + naming.DecoupledProviderInstanceName); naming.RegisterNonAssemblySpecificSchema(Context); naming.RegisterAssemblySpecificSchema(); } mof = naming.Mof; Context.LogMessage(RC.GetString("WMISCHEMA_INSTALLATIONEND")); } string mof; ///Installs the assembly. ////// /// The state of the assembly. [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public override void Commit(IDictionary savedState) { base.Commit(savedState); // See if we were asked to generate a MOF file if(Context.Parameters.ContainsKey("mof")) { string mofFile = Context.Parameters["mof"]; // bug#62252 - Pick a default MOF file name if(mofFile == null || mofFile.Length == 0) { mofFile = Context.Parameters["assemblypath"]; if(mofFile == null || mofFile.Length == 0) mofFile = "defaultmoffile"; else mofFile = Path.GetFileName(mofFile); } // Append '.mof' in necessary if(mofFile.Length<4) mofFile += ".mof"; else { if(String.Compare(mofFile.Substring(mofFile.Length-4,4),".mof",StringComparison.OrdinalIgnoreCase)!=0) mofFile += ".mof"; } Context.LogMessage(RC.GetString("MOFFILE_GENERATING") + " " + mofFile); using(StreamWriter log = new StreamWriter(mofFile, false, Encoding.Unicode)) { log.WriteLine("//**************************************************************************"); log.WriteLine("//* {0}", mofFile); log.WriteLine("//**************************************************************************"); log.WriteLine(mof); } } } ///Commits the assembly to the operation. ////// /// The state of the assembly. public override void Rollback(IDictionary savedState) { base.Rollback(savedState); } ///Rolls back the state of the assembly. ////// /// The state of the assembly. public override void Uninstall(IDictionary savedState) { base.Uninstall(savedState); } } ///Uninstalls the assembly. ////// ///Installs an instrumented assembly. This class is a default project installer for assemblies that contain /// management instrumentation and do not use other installers (such as services, or message /// queues). To use this default project installer, simply derive a class from /// ///inside the assembly. No methods need /// to be overridden. /// ///If your project has a master project /// installer, use the ///class instead. /// public class DefaultManagementProjectInstaller : Installer { ///Add the following code to your instrumented assembly to enable the installation step: ///[System.ComponentModel.RunInstaller(true)] /// public class MyInstaller : DefaultManagementProjectInstaller {} ///
///<System.ComponentModel.RunInstaller(true)> /// public class MyInstaller /// Inherits DefaultManagementProjectInstaller ///
////// public DefaultManagementProjectInstaller() { // Instantiate installer for assembly. ManagementInstaller managementInstaller = new ManagementInstaller(); // Add installers to collection. Order is not important. Installers.Add(managementInstaller); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Management.Instrumentation { using System; using System.Reflection; using System.Collections; using System.Configuration.Install; using System.Text; using System.IO; using System.Runtime.Versioning; using System.Globalization; using System.Security.Permissions; ///Initializes a new instance of the /// ///class. This is the default constructor. /// ///Installs instrumented assemblies. Include an instance of this installer class in the project installer for /// an assembly that includes instrumentation. ////// ///If this is the only installer for your application, you may use the helper class ////// provided in this namespace. /// public class ManagementInstaller : Installer { // private static bool helpPrinted = false; ///If you have a master project installer for your /// project, add the following code to your project installers constructor: ///// Instantiate installer for assembly. /// ManagementInstaller managementInstaller = new ManagementInstaller(); /// /// // Add installer to collection. /// Installers.Add(managementInstaller); ///
///'Instantiate installer for assembly. /// Dim managementInstaller As New ManagementInstaller() /// /// 'Add installer to collection. /// Installers.Add(managementInstaller) ///
////// ///Gets or sets installer options for this class. ////// public override string HelpText { get { if (helpPrinted) return base.HelpText; else { helpPrinted = true; // return Res.GetString(Res.HelpText) + "\r\n" + base.HelpText; // StringBuilder help = new StringBuilder(); help.Append("/MOF=[filename]\r\n"); help.Append(" " + RC.GetString("FILETOWRITE_MOF")+"\r\n\r\n"); // // [RAID: 123895] // If the force parameter is present, we update registration information independent if it already // exists. // help.Append ( "/Force or /F\r\n" ) ; help.Append(" " + RC.GetString("FORCE_UPDATE")); return help.ToString() + base.HelpText; } } } ///The help text for all the installers in the installer collection, including /// the description of what each installer does and the command-line options (for /// the installation program) that can be passed to and understood by each /// installer. ////// /// The state of the assembly. [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public override void Install(IDictionary savedState) { // Demand IO permission required for LoadFrom FXCop 191096 FileIOPermission ioPermission = new FileIOPermission(FileIOPermissionAccess.Read, (string)Context.Parameters["assemblypath"]); ioPermission.Demand(); base.Install(savedState); // Context.LogMessage(RC.GetString("WMISCHEMA_INSTALLATIONSTART")); string assemblyPath = Context.Parameters["assemblypath"]; Assembly assembly = Assembly.LoadFrom(assemblyPath); SchemaNaming naming = SchemaNaming.GetSchemaNaming(assembly); // // We always use the full version number for Whidbey. // naming.DecoupledProviderInstanceName = AssemblyNameUtility.UniqueToAssemblyFullVersion(assembly); // See if this assembly provides instrumentation if(null == naming) return; // // [RAID: 123895] // If the force parameter is present, we update registration information independent if it already // exists. // if( ( naming.IsAssemblyRegistered() == false ) || ( Context.Parameters.ContainsKey ( "force" ) ) || ( Context.Parameters.ContainsKey ( "f" ) ) ) { Context.LogMessage(RC.GetString("REGESTRING_ASSEMBLY") + " " + naming.DecoupledProviderInstanceName); naming.RegisterNonAssemblySpecificSchema(Context); naming.RegisterAssemblySpecificSchema(); } mof = naming.Mof; Context.LogMessage(RC.GetString("WMISCHEMA_INSTALLATIONEND")); } string mof; ///Installs the assembly. ////// /// The state of the assembly. [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public override void Commit(IDictionary savedState) { base.Commit(savedState); // See if we were asked to generate a MOF file if(Context.Parameters.ContainsKey("mof")) { string mofFile = Context.Parameters["mof"]; // bug#62252 - Pick a default MOF file name if(mofFile == null || mofFile.Length == 0) { mofFile = Context.Parameters["assemblypath"]; if(mofFile == null || mofFile.Length == 0) mofFile = "defaultmoffile"; else mofFile = Path.GetFileName(mofFile); } // Append '.mof' in necessary if(mofFile.Length<4) mofFile += ".mof"; else { if(String.Compare(mofFile.Substring(mofFile.Length-4,4),".mof",StringComparison.OrdinalIgnoreCase)!=0) mofFile += ".mof"; } Context.LogMessage(RC.GetString("MOFFILE_GENERATING") + " " + mofFile); using(StreamWriter log = new StreamWriter(mofFile, false, Encoding.Unicode)) { log.WriteLine("//**************************************************************************"); log.WriteLine("//* {0}", mofFile); log.WriteLine("//**************************************************************************"); log.WriteLine(mof); } } } ///Commits the assembly to the operation. ////// /// The state of the assembly. public override void Rollback(IDictionary savedState) { base.Rollback(savedState); } ///Rolls back the state of the assembly. ////// /// The state of the assembly. public override void Uninstall(IDictionary savedState) { base.Uninstall(savedState); } } ///Uninstalls the assembly. ////// ///Installs an instrumented assembly. This class is a default project installer for assemblies that contain /// management instrumentation and do not use other installers (such as services, or message /// queues). To use this default project installer, simply derive a class from /// ///inside the assembly. No methods need /// to be overridden. /// ///If your project has a master project /// installer, use the ///class instead. /// public class DefaultManagementProjectInstaller : Installer { ///Add the following code to your instrumented assembly to enable the installation step: ///[System.ComponentModel.RunInstaller(true)] /// public class MyInstaller : DefaultManagementProjectInstaller {} ///
///<System.ComponentModel.RunInstaller(true)> /// public class MyInstaller /// Inherits DefaultManagementProjectInstaller ///
////// public DefaultManagementProjectInstaller() { // Instantiate installer for assembly. ManagementInstaller managementInstaller = new ManagementInstaller(); // Add installers to collection. Order is not important. Installers.Add(managementInstaller); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Initializes a new instance of the /// ///class. This is the default constructor.
Link Menu
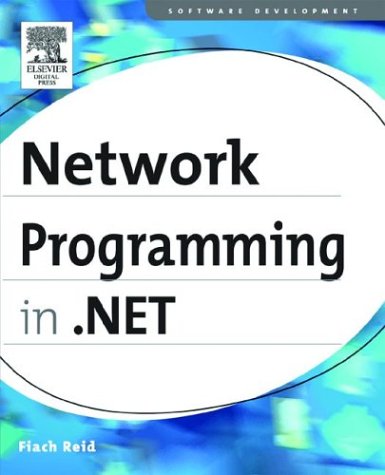
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationSection.cs
- SchemaCompiler.cs
- XamlTreeBuilder.cs
- GlyphTypeface.cs
- XmlSerializerVersionAttribute.cs
- DataGridViewAddColumnDialog.cs
- DropShadowEffect.cs
- TreeNodeEventArgs.cs
- EditorPart.cs
- DBNull.cs
- OciHandle.cs
- ButtonChrome.cs
- HandledMouseEvent.cs
- StorageEntityTypeMapping.cs
- AuthenticationServiceManager.cs
- MessageHeaderAttribute.cs
- KeyFrames.cs
- PrinterSettings.cs
- GC.cs
- StateDesigner.CommentLayoutGlyph.cs
- ProviderSettingsCollection.cs
- DataGridViewCellStyle.cs
- Point4D.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- XpsFixedPageReaderWriter.cs
- ImageFormatConverter.cs
- TextTreeRootNode.cs
- ImportCatalogPart.cs
- MouseOverProperty.cs
- BitmapDecoder.cs
- XmlNodeReader.cs
- ClrProviderManifest.cs
- TypeDependencyAttribute.cs
- FixedSOMTextRun.cs
- DataKeyCollection.cs
- NCryptSafeHandles.cs
- HandledMouseEvent.cs
- XmlSchemaSimpleTypeRestriction.cs
- SmiXetterAccessMap.cs
- TableLayoutPanel.cs
- ContractTypeNameCollection.cs
- BaseDataBoundControl.cs
- Vector3DKeyFrameCollection.cs
- Keywords.cs
- TypeDescriptorContext.cs
- ComponentSerializationService.cs
- DataPagerFieldCommandEventArgs.cs
- StyleXamlParser.cs
- DataGridViewTextBoxCell.cs
- LocatorManager.cs
- AppSettingsExpressionBuilder.cs
- ValidatorCollection.cs
- DBSchemaTable.cs
- ResourceIDHelper.cs
- XmlEntity.cs
- TextEffect.cs
- DiagnosticsConfiguration.cs
- UInt32Converter.cs
- HttpConfigurationContext.cs
- PropertyMapper.cs
- _NestedMultipleAsyncResult.cs
- XmlSchemaProviderAttribute.cs
- PassportAuthenticationEventArgs.cs
- updatecommandorderer.cs
- DataGridAddNewRow.cs
- EventOpcode.cs
- AuthorizationSection.cs
- StoreItemCollection.Loader.cs
- XmlStreamNodeWriter.cs
- WinEventTracker.cs
- InternalResources.cs
- NTAccount.cs
- DetailsViewCommandEventArgs.cs
- SHA1CryptoServiceProvider.cs
- HtmlTableRowCollection.cs
- Substitution.cs
- RichTextBoxAutomationPeer.cs
- ToolStripItemRenderEventArgs.cs
- ExtensionQuery.cs
- PrintPreviewControl.cs
- HttpChannelFactory.cs
- SerializationFieldInfo.cs
- HttpConfigurationContext.cs
- Msec.cs
- PagesSection.cs
- DataBoundControlAdapter.cs
- FontFamily.cs
- ExportOptions.cs
- RemotingServices.cs
- ParsedAttributeCollection.cs
- PlatformCulture.cs
- newinstructionaction.cs
- Menu.cs
- CodeTypeConstructor.cs
- DataGridViewLayoutData.cs
- XPathNavigatorReader.cs
- NetTcpBindingElement.cs
- MergeExecutor.cs
- HttpModuleActionCollection.cs
- ReadOnlyHierarchicalDataSource.cs