Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / SerializationHelper.cs / 1 / SerializationHelper.cs
using MS.Utility; using System; using System.IO; using System.Runtime.InteropServices; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// Summary description for HelperMethods. /// internal static class SerializationHelper { ////// returns the no of byte it requires to mutlibyte encode a uint value /// /// ///public static uint VarSize(uint Value) { if (Value < 0x80) return 1; else if (Value < 0x4000) return 2; else if (Value < 0x200000) return 3; else if (Value < 0x10000000) return 4; else return 5; } /// /// MultiByte Encodes an uint Value into the stream /// /// /// ///public static uint Encode(Stream strm, uint Value) { ulong ib = 0; for (; ; ) { if (Value < 128) { strm.WriteByte((byte)Value); return (uint)(ib + 1); } strm.WriteByte((byte)(0x0080 | (Value & 0x7f))); Value >>= 7; ib++; } } /// /// Multibyte encodes a unsinged long value in the stream /// /// /// ///public static uint EncodeLarge(Stream strm, ulong ulValue) { uint ib = 0; for (; ; ) { if (ulValue < 128) { strm.WriteByte((byte)ulValue); return ib + 1; } strm.WriteByte((byte)(0x0080 | (ulValue & 0x7f))); ulValue >>= 7; ib++; } } /// /// Multibyte encodes a signed integer value into a stream. Use 1's complement to /// store signed values. This means both 00 and 01 are actually 0, but we don't /// need to encode all negative numbers as 64 bit values. /// /// /// ///// Use 1's complement to store signed values. This means both 00 and 01 are // actually 0, but we don't need to encode all negative numbers as 64 bit values. public static uint SignEncode(Stream strm, int Value) { ulong ull = 0; // special case LONG_MIN if (-2147483648 == Value) { ull = 0x0000000100000001; } else { ull = (ulong)Math.Abs(Value); // multiply by 2 ull <<= 1; // For -ve nos, add 1 if (Value < 0) ull |= 1; } return EncodeLarge(strm, ull); } /// /// Decodes a multi byte encoded unsigned integer from the stream /// /// /// ///public static uint Decode(Stream strm, out uint dw) { int shift = 0; byte b = 0; uint cb = 0; dw = 0; do { b = (byte)strm.ReadByte(); cb++; dw += (uint)((int)(b & 0x7f) << shift); shift += 7; } while (((b & 0x80) > 0) && (shift < 29)); return cb; } /// /// Decodes a multibyte encoded unsigned long from the stream /// /// /// ///public static uint DecodeLarge(Stream strm, out ulong ull) { long ull1; int shift = 0; byte b = 0, a = 0; uint cb = 0; ull = 0; do { b = (byte)strm.ReadByte(); cb++; a = (byte)(b & 0x7f); ull1 = a; ull |= (ulong)(ull1 << shift); shift += 7; } while (((b & 0x80) > 0) && (shift < 57)); return cb; } /// /// Decodes a multibyte encoded signed integer from the stream /// /// /// ///public static uint SignDecode(Stream strm, out int i) { i = 0; ulong ull = 0; uint cb = DecodeLarge(strm, out ull); if (cb > 0) { bool fneg = false; if ((ull & 0x0001) > 0) fneg = true; ull = ull >> 1; long l = (long)ull; i = (int)(fneg ? -l : l); } return cb; } /// /// Converts the CLR type information into a COM-compatible type enumeration /// /// The CLR type information of the object to convert /// Throw an exception if unknown type is used ///The COM-compatible type enumeration ///Only supports the types of data that are supported in ISF ExtendedProperties public static VarEnum ConvertToVarEnum(Type type, bool throwOnError) { if (typeof(char) == type) { return VarEnum.VT_I1; } else if (typeof(char[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I1); } else if (typeof(byte) == type) { return VarEnum.VT_UI1; } else if (typeof(byte[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI1); } else if (typeof(Int16) == type) { return VarEnum.VT_I2; } else if (typeof(Int16[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I2); } else if (typeof(UInt16) == type) { return VarEnum.VT_UI2; } else if (typeof(UInt16[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI2); } else if (typeof(Int32) == type) { return VarEnum.VT_I4; } else if (typeof(Int32[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I4); } else if (typeof(UInt32) == type) { return VarEnum.VT_UI4; } else if (typeof(UInt32[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI4); } else if (typeof(Int64) == type) { return VarEnum.VT_I8; } else if (typeof(Int64[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I8); } else if (typeof(UInt64) == type) { return VarEnum.VT_UI8; } else if (typeof(UInt64[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI8); } else if (typeof(Single) == type) { return VarEnum.VT_R4; } else if (typeof(Single[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_R4); } else if (typeof(Double) == type) { return VarEnum.VT_R8; } else if (typeof(Double[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_R8); } else if (typeof(DateTime) == type) { return VarEnum.VT_DATE; } else if (typeof(DateTime[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_DATE); } else if (typeof(Boolean) == type) { return VarEnum.VT_BOOL; } else if (typeof(Boolean[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_BOOL); } else if (typeof(String) == type) { return VarEnum.VT_BSTR; } else if (typeof(Decimal) == type) { return VarEnum.VT_DECIMAL; } else if (typeof(Decimal[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_DECIMAL); } else { if (throwOnError) { throw new ArgumentException(SR.Get(SRID.InvalidDataTypeForExtendedProperty)); } else { return VarEnum.VT_UNKNOWN; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.IO; using System.Runtime.InteropServices; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// Summary description for HelperMethods. /// internal static class SerializationHelper { ////// returns the no of byte it requires to mutlibyte encode a uint value /// /// ///public static uint VarSize(uint Value) { if (Value < 0x80) return 1; else if (Value < 0x4000) return 2; else if (Value < 0x200000) return 3; else if (Value < 0x10000000) return 4; else return 5; } /// /// MultiByte Encodes an uint Value into the stream /// /// /// ///public static uint Encode(Stream strm, uint Value) { ulong ib = 0; for (; ; ) { if (Value < 128) { strm.WriteByte((byte)Value); return (uint)(ib + 1); } strm.WriteByte((byte)(0x0080 | (Value & 0x7f))); Value >>= 7; ib++; } } /// /// Multibyte encodes a unsinged long value in the stream /// /// /// ///public static uint EncodeLarge(Stream strm, ulong ulValue) { uint ib = 0; for (; ; ) { if (ulValue < 128) { strm.WriteByte((byte)ulValue); return ib + 1; } strm.WriteByte((byte)(0x0080 | (ulValue & 0x7f))); ulValue >>= 7; ib++; } } /// /// Multibyte encodes a signed integer value into a stream. Use 1's complement to /// store signed values. This means both 00 and 01 are actually 0, but we don't /// need to encode all negative numbers as 64 bit values. /// /// /// ///// Use 1's complement to store signed values. This means both 00 and 01 are // actually 0, but we don't need to encode all negative numbers as 64 bit values. public static uint SignEncode(Stream strm, int Value) { ulong ull = 0; // special case LONG_MIN if (-2147483648 == Value) { ull = 0x0000000100000001; } else { ull = (ulong)Math.Abs(Value); // multiply by 2 ull <<= 1; // For -ve nos, add 1 if (Value < 0) ull |= 1; } return EncodeLarge(strm, ull); } /// /// Decodes a multi byte encoded unsigned integer from the stream /// /// /// ///public static uint Decode(Stream strm, out uint dw) { int shift = 0; byte b = 0; uint cb = 0; dw = 0; do { b = (byte)strm.ReadByte(); cb++; dw += (uint)((int)(b & 0x7f) << shift); shift += 7; } while (((b & 0x80) > 0) && (shift < 29)); return cb; } /// /// Decodes a multibyte encoded unsigned long from the stream /// /// /// ///public static uint DecodeLarge(Stream strm, out ulong ull) { long ull1; int shift = 0; byte b = 0, a = 0; uint cb = 0; ull = 0; do { b = (byte)strm.ReadByte(); cb++; a = (byte)(b & 0x7f); ull1 = a; ull |= (ulong)(ull1 << shift); shift += 7; } while (((b & 0x80) > 0) && (shift < 57)); return cb; } /// /// Decodes a multibyte encoded signed integer from the stream /// /// /// ///public static uint SignDecode(Stream strm, out int i) { i = 0; ulong ull = 0; uint cb = DecodeLarge(strm, out ull); if (cb > 0) { bool fneg = false; if ((ull & 0x0001) > 0) fneg = true; ull = ull >> 1; long l = (long)ull; i = (int)(fneg ? -l : l); } return cb; } /// /// Converts the CLR type information into a COM-compatible type enumeration /// /// The CLR type information of the object to convert /// Throw an exception if unknown type is used ///The COM-compatible type enumeration ///Only supports the types of data that are supported in ISF ExtendedProperties public static VarEnum ConvertToVarEnum(Type type, bool throwOnError) { if (typeof(char) == type) { return VarEnum.VT_I1; } else if (typeof(char[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I1); } else if (typeof(byte) == type) { return VarEnum.VT_UI1; } else if (typeof(byte[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI1); } else if (typeof(Int16) == type) { return VarEnum.VT_I2; } else if (typeof(Int16[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I2); } else if (typeof(UInt16) == type) { return VarEnum.VT_UI2; } else if (typeof(UInt16[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI2); } else if (typeof(Int32) == type) { return VarEnum.VT_I4; } else if (typeof(Int32[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I4); } else if (typeof(UInt32) == type) { return VarEnum.VT_UI4; } else if (typeof(UInt32[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI4); } else if (typeof(Int64) == type) { return VarEnum.VT_I8; } else if (typeof(Int64[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_I8); } else if (typeof(UInt64) == type) { return VarEnum.VT_UI8; } else if (typeof(UInt64[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_UI8); } else if (typeof(Single) == type) { return VarEnum.VT_R4; } else if (typeof(Single[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_R4); } else if (typeof(Double) == type) { return VarEnum.VT_R8; } else if (typeof(Double[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_R8); } else if (typeof(DateTime) == type) { return VarEnum.VT_DATE; } else if (typeof(DateTime[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_DATE); } else if (typeof(Boolean) == type) { return VarEnum.VT_BOOL; } else if (typeof(Boolean[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_BOOL); } else if (typeof(String) == type) { return VarEnum.VT_BSTR; } else if (typeof(Decimal) == type) { return VarEnum.VT_DECIMAL; } else if (typeof(Decimal[]) == type) { return (VarEnum.VT_ARRAY | VarEnum.VT_DECIMAL); } else { if (throwOnError) { throw new ArgumentException(SR.Get(SRID.InvalidDataTypeForExtendedProperty)); } else { return VarEnum.VT_UNKNOWN; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
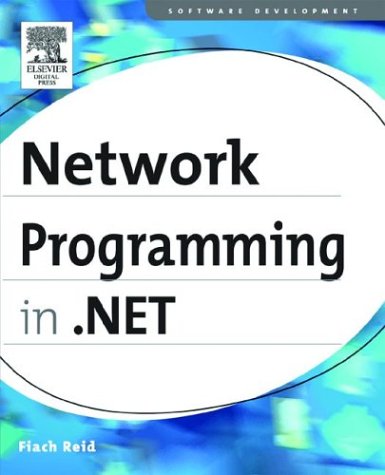
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlArrayItemAttribute.cs
- AppLevelCompilationSectionCache.cs
- Cell.cs
- EventHandlerList.cs
- DbDataSourceEnumerator.cs
- TextModifierScope.cs
- ToolStripItemClickedEventArgs.cs
- SchemaTypeEmitter.cs
- Condition.cs
- WebPartConnectionCollection.cs
- TextFormatter.cs
- FrameworkTemplate.cs
- EncoderParameters.cs
- Compilation.cs
- XmlSchemaException.cs
- UriTemplateVariablePathSegment.cs
- SqlDataSourceCommandEventArgs.cs
- BitmapEffectRenderDataResource.cs
- ExecutionContext.cs
- DesignerExtenders.cs
- ObjectDataSourceMethodEventArgs.cs
- COM2PropertyDescriptor.cs
- VisualBrush.cs
- ResourcesChangeInfo.cs
- SecurityCriticalDataForSet.cs
- DynamicValidator.cs
- storepermissionattribute.cs
- Brush.cs
- EmbossBitmapEffect.cs
- NullRuntimeConfig.cs
- WebControlsSection.cs
- FilterException.cs
- ApplicationInterop.cs
- Padding.cs
- AnnotationResource.cs
- StringFunctions.cs
- GZipUtils.cs
- MatcherBuilder.cs
- DeviceContext.cs
- MulticastDelegate.cs
- RepeaterItemEventArgs.cs
- SqlUnionizer.cs
- ForEachAction.cs
- XmlSchemaAttributeGroupRef.cs
- SqlDuplicator.cs
- GenericWebPart.cs
- Animatable.cs
- UnionCodeGroup.cs
- DataGridViewCheckBoxCell.cs
- DependencyPropertyConverter.cs
- PointValueSerializer.cs
- BaseTemplateCodeDomTreeGenerator.cs
- RegexStringValidator.cs
- ConfigurationValue.cs
- SymbolEqualComparer.cs
- SqlOuterApplyReducer.cs
- ActionFrame.cs
- ConfigurationProperty.cs
- XmlSiteMapProvider.cs
- ApplicationFileCodeDomTreeGenerator.cs
- ClientRolePrincipal.cs
- DatatypeImplementation.cs
- DataServiceRequestOfT.cs
- ListSourceHelper.cs
- FormViewInsertEventArgs.cs
- DefaultValidator.cs
- ChtmlTextWriter.cs
- DataTablePropertyDescriptor.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- DataBindingCollectionEditor.cs
- sortedlist.cs
- TextChange.cs
- ControlPropertyNameConverter.cs
- SelfIssuedAuthRSACryptoProvider.cs
- RegexWriter.cs
- WmlListAdapter.cs
- WorkflowMarkupSerializerMapping.cs
- MultipleViewPatternIdentifiers.cs
- NetworkInformationPermission.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- TextDocumentView.cs
- nulltextnavigator.cs
- CheckBoxBaseAdapter.cs
- RequestValidator.cs
- NavigationCommands.cs
- DrawListViewSubItemEventArgs.cs
- EventlogProvider.cs
- CacheAxisQuery.cs
- OracleRowUpdatedEventArgs.cs
- PerformanceCounterPermissionEntryCollection.cs
- ClientSideProviderDescription.cs
- EventManager.cs
- EarlyBoundInfo.cs
- StrokeRenderer.cs
- ZipArchive.cs
- InputLanguageCollection.cs
- TransformGroup.cs
- HtmlForm.cs
- CommentAction.cs
- PathTooLongException.cs