Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / TextSerializer.cs / 1 / TextSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for text content. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System.Diagnostics; using System.IO; using System.Text; using System.Xml; #endregion Namespaces. ////// Provides support for serializing responses in text format. /// internal struct TextSerializer : IExceptionWriter { ///Writer to which output is sent. private readonly TextWriter writer; ///Initializes a new /// Stream to which output should be sent. /// Encoding to be used to write the result. internal TextSerializer(Stream output, Encoding encoding) { Debug.Assert(output != null, "output != null"); Debug.Assert(encoding != null, "encoding != null"); this.writer = new StreamWriter(output, encoding); } ///for the specified stream. Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { XmlWriter xmlWriter = XmlWriter.Create(this.writer); ErrorHandler.SerializeXmlError(args, xmlWriter); this.writer.Flush(); } ///Handles the complete serialization for the specified content. /// Single Content to write.. ///internal void WriteRequest(object content) { Debug.Assert(content != null, "content != null"); string contentAsText; if (!System.Data.Services.Parsing.WebConvert.TryXmlPrimitiveToString(content, out contentAsText)) { throw new InvalidOperationException(Strings.Serializer_CannotConvertValue(content)); } Debug.Assert(contentAsText != null, "contentAsText != null"); this.writer.Write(contentAsText); this.writer.Flush(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // should be a byte array. // Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for text content. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System.Diagnostics; using System.IO; using System.Text; using System.Xml; #endregion Namespaces. ////// Provides support for serializing responses in text format. /// internal struct TextSerializer : IExceptionWriter { ///Writer to which output is sent. private readonly TextWriter writer; ///Initializes a new /// Stream to which output should be sent. /// Encoding to be used to write the result. internal TextSerializer(Stream output, Encoding encoding) { Debug.Assert(output != null, "output != null"); Debug.Assert(encoding != null, "encoding != null"); this.writer = new StreamWriter(output, encoding); } ///for the specified stream. Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { XmlWriter xmlWriter = XmlWriter.Create(this.writer); ErrorHandler.SerializeXmlError(args, xmlWriter); this.writer.Flush(); } ///Handles the complete serialization for the specified content. /// Single Content to write.. ///internal void WriteRequest(object content) { Debug.Assert(content != null, "content != null"); string contentAsText; if (!System.Data.Services.Parsing.WebConvert.TryXmlPrimitiveToString(content, out contentAsText)) { throw new InvalidOperationException(Strings.Serializer_CannotConvertValue(content)); } Debug.Assert(contentAsText != null, "contentAsText != null"); this.writer.Write(contentAsText); this.writer.Flush(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. should be a byte array.
Link Menu
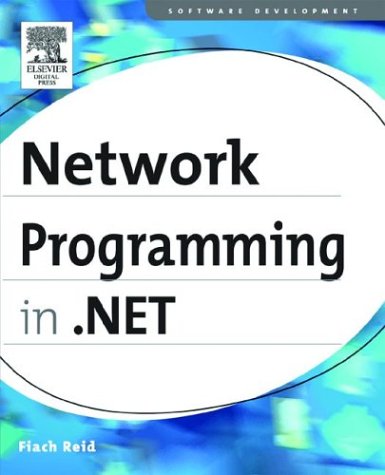
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakReferenceEnumerator.cs
- DesignerGenericWebPart.cs
- CollectionView.cs
- Memoizer.cs
- HtmlInputCheckBox.cs
- MailSettingsSection.cs
- MenuEventArgs.cs
- UnsafeNativeMethods.cs
- PkcsUtils.cs
- _ConnectionGroup.cs
- MimePart.cs
- assemblycache.cs
- RecordBuilder.cs
- RegistrationServices.cs
- XmlSchemaExternal.cs
- DbConnectionPoolGroup.cs
- UnknownBitmapEncoder.cs
- Transactions.cs
- AssemblyInfo.cs
- DbDeleteCommandTree.cs
- TextMessageEncodingBindingElement.cs
- wmiprovider.cs
- SubclassTypeValidator.cs
- TransformedBitmap.cs
- basenumberconverter.cs
- CodeTryCatchFinallyStatement.cs
- DataGridRowClipboardEventArgs.cs
- ProfileGroupSettingsCollection.cs
- CfgParser.cs
- Utils.cs
- Point3D.cs
- BaseTemplateCodeDomTreeGenerator.cs
- Imaging.cs
- Rect3DValueSerializer.cs
- TrackBarDesigner.cs
- LoadedOrUnloadedOperation.cs
- wgx_exports.cs
- XPathDocumentIterator.cs
- InternalResources.cs
- DisplayNameAttribute.cs
- FieldBuilder.cs
- MatrixTransform.cs
- NavigationPropertySingletonExpression.cs
- BulletedList.cs
- DataTableNewRowEvent.cs
- GroupDescription.cs
- FlowDocumentPaginator.cs
- SrgsText.cs
- SwitchElementsCollection.cs
- BitmapEffectGroup.cs
- Scripts.cs
- InvokeSchedule.cs
- JobInputBins.cs
- PrePrepareMethodAttribute.cs
- Knowncolors.cs
- ProgramNode.cs
- DataTableTypeConverter.cs
- Shape.cs
- TableStyle.cs
- TextBox.cs
- TrackPointCollection.cs
- ContractNamespaceAttribute.cs
- StatusBarAutomationPeer.cs
- jithelpers.cs
- IsolatedStorage.cs
- DiagnosticStrings.cs
- PixelFormat.cs
- SecUtil.cs
- DataServiceClientException.cs
- EmptyEnumerable.cs
- SqlMethods.cs
- StateMachineWorkflowDesigner.cs
- BinaryFormatterSinks.cs
- WpfGeneratedKnownProperties.cs
- SystemIPInterfaceProperties.cs
- SqlDataSourceSelectingEventArgs.cs
- OperationCanceledException.cs
- MembershipAdapter.cs
- ByteConverter.cs
- XmlCustomFormatter.cs
- ConvertersCollection.cs
- DataGrid.cs
- PassportAuthenticationEventArgs.cs
- ScopeElement.cs
- ImageMapEventArgs.cs
- SystemFonts.cs
- ScopelessEnumAttribute.cs
- bidPrivateBase.cs
- WebMessageBodyStyleHelper.cs
- DataGridColumnReorderingEventArgs.cs
- PasswordTextContainer.cs
- DataContractJsonSerializerOperationBehavior.cs
- CustomAttribute.cs
- CheckBoxPopupAdapter.cs
- ElementFactory.cs
- FocusTracker.cs
- GCHandleCookieTable.cs
- ArglessEventHandlerProxy.cs
- ComplexType.cs
- FixedSOMLineCollection.cs