Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / SwitchElementsCollection.cs / 1 / SwitchElementsCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; using System.Collections; using System.Collections.Specialized; namespace System.Diagnostics { [ConfigurationCollection(typeof(SwitchElement))] internal class SwitchElementsCollection : ConfigurationElementCollection { new public SwitchElement this[string name] { get { return (SwitchElement) BaseGet(name); } } public override ConfigurationElementCollectionType CollectionType { get { return ConfigurationElementCollectionType.AddRemoveClearMap; } } protected override ConfigurationElement CreateNewElement() { return new SwitchElement(); } protected override Object GetElementKey(ConfigurationElement element) { return ((SwitchElement) element).Name; } } internal class SwitchElement : ConfigurationElement { private static readonly ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), "", ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propValue = new ConfigurationProperty("value", typeof(string), null, ConfigurationPropertyOptions.IsRequired); private Hashtable _attributes; static SwitchElement(){ _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propValue); } public Hashtable Attributes { get { if (_attributes == null) _attributes = new Hashtable(StringComparer.OrdinalIgnoreCase); return _attributes; } } [ConfigurationProperty("name", DefaultValue = "", IsRequired = true, IsKey = true)] public string Name { get { return (string) this[_propName]; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("value", IsRequired = true)] public string Value { get { return (string) this[_propValue]; } } protected override bool OnDeserializeUnrecognizedAttribute(String name, String value) { ConfigurationProperty _propDynamic = new ConfigurationProperty(name, typeof(string), value); _properties.Add(_propDynamic); base[_propDynamic] = value; // Add them to the property bag Attributes.Add(name, value); return true; } internal void ResetProperties() { // blow away any UnrecognizedAttributes that we have deserialized earlier if (_attributes != null) { _attributes.Clear(); _properties.Clear(); _properties.Add(_propName); _properties.Add(_propValue); } } } }
Link Menu
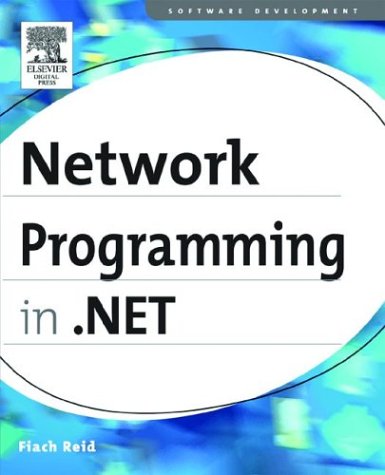
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StrokeRenderer.cs
- SqlCacheDependencyDatabase.cs
- CSharpCodeProvider.cs
- EmbeddedMailObject.cs
- UriTemplateEquivalenceComparer.cs
- LoaderAllocator.cs
- PlaceHolder.cs
- HWStack.cs
- ButtonField.cs
- XmlBaseReader.cs
- FixedHyperLink.cs
- SevenBitStream.cs
- HashMembershipCondition.cs
- PathFigure.cs
- Group.cs
- PowerModeChangedEventArgs.cs
- OSFeature.cs
- DataKeyArray.cs
- XmlNodeChangedEventArgs.cs
- PartialCachingControl.cs
- BamlLocalizabilityResolver.cs
- DataGridLength.cs
- ResourceAssociationSetEnd.cs
- TreeView.cs
- InvokeHandlers.cs
- BaseTemplateBuildProvider.cs
- AnnotationHighlightLayer.cs
- ScriptRef.cs
- DataServiceRequestOfT.cs
- Vector3DAnimationUsingKeyFrames.cs
- DataContractJsonSerializer.cs
- WebBrowserEvent.cs
- FontStretchConverter.cs
- QueryTaskGroupState.cs
- Pair.cs
- DeferredReference.cs
- NestedContainer.cs
- AnnotationHighlightLayer.cs
- ThreadAbortException.cs
- Column.cs
- CommunicationException.cs
- TypeSource.cs
- TextEditorLists.cs
- PropertyDescriptorCollection.cs
- StructuredTypeEmitter.cs
- WindowsFormsSectionHandler.cs
- ResolvePPIDRequest.cs
- QuaternionAnimationUsingKeyFrames.cs
- TextEditorThreadLocalStore.cs
- SelectionItemPattern.cs
- OleDbWrapper.cs
- AppLevelCompilationSectionCache.cs
- ResizeGrip.cs
- CharacterBufferReference.cs
- DynamicResourceExtensionConverter.cs
- SerialReceived.cs
- MsmqHostedTransportConfiguration.cs
- HandlerFactoryWrapper.cs
- contentDescriptor.cs
- ObjectDataProvider.cs
- UserControlCodeDomTreeGenerator.cs
- PreservationFileReader.cs
- EntityUtil.cs
- WebConvert.cs
- Column.cs
- TypeConverterHelper.cs
- ReflectionPermission.cs
- NativeMethodsOther.cs
- RemoteWebConfigurationHostStream.cs
- _BufferOffsetSize.cs
- Style.cs
- ImageList.cs
- ADRoleFactoryConfiguration.cs
- ReflectionUtil.cs
- RemoteWebConfigurationHostStream.cs
- CompilerScopeManager.cs
- ErrorFormatter.cs
- EntitySet.cs
- Function.cs
- GraphicsState.cs
- ToolStripHighContrastRenderer.cs
- ObjectToIdCache.cs
- CodeDirectionExpression.cs
- StylusPlugin.cs
- OleDbTransaction.cs
- SectionRecord.cs
- CompilationUtil.cs
- XmlElementCollection.cs
- AttachmentCollection.cs
- TextCollapsingProperties.cs
- XmlNamedNodeMap.cs
- IISMapPath.cs
- Geometry.cs
- CodeMemberMethod.cs
- SmtpLoginAuthenticationModule.cs
- SafeReversePInvokeHandle.cs
- DeliveryStrategy.cs
- SqlReferenceCollection.cs
- OleDbSchemaGuid.cs
- CultureInfoConverter.cs