Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / DeferredReference.cs / 1 / DeferredReference.cs
//---------------------------------------------------------------------------- // // File: DeferredReference.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Proxy object passed to the property system to delay load values. // //--------------------------------------------------------------------------- namespace System.Windows { using MS.Internal.WindowsBase; // FriendAccessAllowed // Proxy object passed to the property system to delay load values. // // The property system will make a GetValue callback (dereferencing the // reference) inside DependencyProperty.GetValue calls, or before // coercion callbacks to derived classes. // // DeferredReference instances are passed directly to ValidateValue // callbacks (which always go to the DependencyProperty owner class), // and also to CoerceValue callbacks on the owner class only. THEREFORE, // IT IS [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal abstract class DeferredReference { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Deferences a property value on demand. internal abstract object GetValue(BaseValueSourceInternal valueSource); // Gets the type of the value it represents internal abstract Type GetValueType(); #endregion Internal Methods } internal class DeferredMutableDefaultReference : DeferredReference { #region Constructor internal DeferredMutableDefaultReference(PropertyMetadata metadata, DependencyObject d, DependencyProperty dp) { _sourceObject = d; _sourceProperty = dp; _sourceMetadata = metadata; } #endregion Constructor #region Methods internal override object GetValue(BaseValueSourceInternal valueSource) { return _sourceMetadata.GetDefaultValue(_sourceObject, _sourceProperty); } // Gets the type of the value it represents internal override Type GetValueType() { return _sourceProperty.PropertyType; } #endregion Methods #region Properties internal PropertyMetadata SourceMetadata { get { return _sourceMetadata; } } protected DependencyObject SourceObject { get { return _sourceObject; } } protected DependencyProperty SourceProperty { get { return _sourceProperty; } } #endregion Properties #region Data private readonly PropertyMetadata _sourceMetadata; private readonly DependencyObject _sourceObject; private readonly DependencyProperty _sourceProperty; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: DeferredReference.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Proxy object passed to the property system to delay load values. // //--------------------------------------------------------------------------- namespace System.Windows { using MS.Internal.WindowsBase; // FriendAccessAllowed // Proxy object passed to the property system to delay load values. // // The property system will make a GetValue callback (dereferencing the // reference) inside DependencyProperty.GetValue calls, or before // coercion callbacks to derived classes. // // DeferredReference instances are passed directly to ValidateValue // callbacks (which always go to the DependencyProperty owner class), // and also to CoerceValue callbacks on the owner class only. THEREFORE, // IT IS [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal abstract class DeferredReference { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Deferences a property value on demand. internal abstract object GetValue(BaseValueSourceInternal valueSource); // Gets the type of the value it represents internal abstract Type GetValueType(); #endregion Internal Methods } internal class DeferredMutableDefaultReference : DeferredReference { #region Constructor internal DeferredMutableDefaultReference(PropertyMetadata metadata, DependencyObject d, DependencyProperty dp) { _sourceObject = d; _sourceProperty = dp; _sourceMetadata = metadata; } #endregion Constructor #region Methods internal override object GetValue(BaseValueSourceInternal valueSource) { return _sourceMetadata.GetDefaultValue(_sourceObject, _sourceProperty); } // Gets the type of the value it represents internal override Type GetValueType() { return _sourceProperty.PropertyType; } #endregion Methods #region Properties internal PropertyMetadata SourceMetadata { get { return _sourceMetadata; } } protected DependencyObject SourceObject { get { return _sourceObject; } } protected DependencyProperty SourceProperty { get { return _sourceProperty; } } #endregion Properties #region Data private readonly PropertyMetadata _sourceMetadata; private readonly DependencyObject _sourceObject; private readonly DependencyProperty _sourceProperty; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
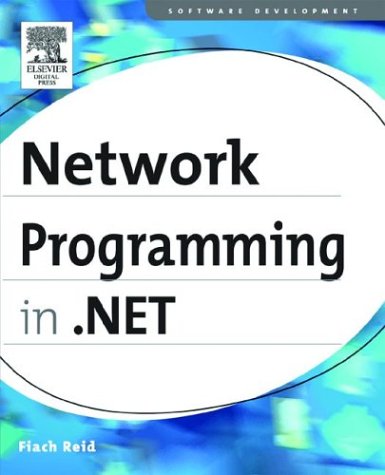
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceContainerNameItem.cs
- CodeSnippetCompileUnit.cs
- JsonReader.cs
- ObjectTokenCategory.cs
- TableParaClient.cs
- SafeNativeMethods.cs
- columnmapfactory.cs
- MemberBinding.cs
- TextContainerChangedEventArgs.cs
- RawUIStateInputReport.cs
- FloaterBaseParaClient.cs
- SerializationHelper.cs
- ContextProperty.cs
- PtsCache.cs
- HtmlInputReset.cs
- DeferredElementTreeState.cs
- DataPagerFieldItem.cs
- XmlCharacterData.cs
- NotificationContext.cs
- Main.cs
- ClaimTypes.cs
- XsdDateTime.cs
- CharUnicodeInfo.cs
- CodePropertyReferenceExpression.cs
- JavaScriptString.cs
- PageWrapper.cs
- PropertyChangedEventManager.cs
- SafeHandles.cs
- XmlIlTypeHelper.cs
- LookupBindingPropertiesAttribute.cs
- MetadataAssemblyHelper.cs
- MemberJoinTreeNode.cs
- ConnectionPointCookie.cs
- PassportAuthenticationEventArgs.cs
- ContextMenuAutomationPeer.cs
- MatrixValueSerializer.cs
- BuildManagerHost.cs
- SchemaImporterExtensionsSection.cs
- ToolStripRendererSwitcher.cs
- SmtpFailedRecipientException.cs
- StateInitializationDesigner.cs
- CssClassPropertyAttribute.cs
- ServiceEndpoint.cs
- GenericAuthenticationEventArgs.cs
- WindowsStatic.cs
- TextTreeTextNode.cs
- AssemblyBuilder.cs
- CompilationUtil.cs
- TextParagraph.cs
- precedingsibling.cs
- Switch.cs
- OracleSqlParser.cs
- GlyphTypeface.cs
- BaseDataListDesigner.cs
- X509SecurityToken.cs
- DropSource.cs
- CodeExpressionRuleDeclaration.cs
- OdbcParameter.cs
- MediaSystem.cs
- DataError.cs
- AutomationPattern.cs
- SharedConnectionInfo.cs
- EntityWithChangeTrackerStrategy.cs
- SslStream.cs
- DomNameTable.cs
- IdnElement.cs
- KnownTypes.cs
- PreservationFileWriter.cs
- WebConfigurationFileMap.cs
- GroupBoxRenderer.cs
- FormClosedEvent.cs
- InputProcessorProfiles.cs
- Glyph.cs
- Timer.cs
- ControlPersister.cs
- SchemaCollectionCompiler.cs
- OleDbException.cs
- TakeQueryOptionExpression.cs
- ClientSettingsProvider.cs
- Monitor.cs
- FontNamesConverter.cs
- ItemMap.cs
- MaskDescriptors.cs
- SignedInfo.cs
- recordstate.cs
- Operator.cs
- BreadCrumbTextConverter.cs
- GeometryCombineModeValidation.cs
- CustomError.cs
- ScrollData.cs
- HandleCollector.cs
- HttpProfileBase.cs
- RelativeSource.cs
- HitTestDrawingContextWalker.cs
- ParseChildrenAsPropertiesAttribute.cs
- FontUnitConverter.cs
- XmlHierarchicalEnumerable.cs
- FolderBrowserDialog.cs
- StrokeNodeData.cs
- WebServiceTypeData.cs