Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Description / ServiceEndpoint.cs / 1 / ServiceEndpoint.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System; using System.Globalization; using System.ServiceModel; using System.ServiceModel.Dispatcher; using System.Transactions; using System.ServiceModel.Channels; using System.Collections.Generic; using System.Diagnostics; [DebuggerDisplay("Address={address}")] [DebuggerDisplay("Name={name}")] public class ServiceEndpoint { EndpointAddress address; Binding binding; ContractDescription contract; Uri listenUri; ListenUriMode listenUriMode = ListenUriMode.Explicit; KeyedByTypeCollectionbehaviors; string id; XmlName name; public ServiceEndpoint(ContractDescription contract) { if (contract == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contract"); this.contract = contract; } public ServiceEndpoint(ContractDescription contract, Binding binding, EndpointAddress address) { if (contract == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contract"); this.contract = contract; this.binding = binding; this.address = address; } public EndpointAddress Address { get { return this.address; } set { this.address = value; } } public KeyedByTypeCollection Behaviors { get { if (this.behaviors == null) this.behaviors = new KeyedByTypeCollection (); return this.behaviors; } } public Binding Binding { get { return this.binding; } set { this.binding = value; } } public ContractDescription Contract { get { return this.contract; } } public string Name { get { if (!XmlName.IsNullOrEmpty(name)) { return name.EncodedName; } else if (binding != null) { // [....]: composing names have potential problem of generating name that looks like an encoded name, consider avoiding '_' return String.Format(CultureInfo.InvariantCulture, "{0}_{1}", new XmlName(Binding.Name).EncodedName, Contract.Name); } else { return Contract.Name; } } set { name = new XmlName(value, true /*isEncoded*/); } } public Uri ListenUri { get { if (this.listenUri == null) { if (this.address == null) { return null; } else { return this.address.Uri; } } else { return this.listenUri; } } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } if (!value.IsAbsoluteUri) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("value", SR.GetString(SR.UriMustBeAbsolute)); } this.listenUri = value; } } public ListenUriMode ListenUriMode { get { return this.listenUriMode; } set { if (!ListenUriModeHelper.IsDefined(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } this.listenUriMode = value; } } internal string Id { get { if (id == null) id = Guid.NewGuid().ToString(); return id; } } // This method ensures that the description object graph is structurally sound and that none // of the fundamental SFx framework assumptions have been violated. internal void EnsureInvariants() { if (Binding == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.AChannelServiceEndpointSBindingIsNull0))); } if (Contract == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.AChannelServiceEndpointSContractIsNull0))); } this.Contract.EnsureInvariants(); this.Binding.EnsureInvariants(this.Contract.Name); } internal void ValidateForClient() { Validate(true, false); } internal void ValidateForService(bool runOperationValidators) { Validate(runOperationValidators, true); } // This method runs validators (both builtin and ones in description). // Precondition: EnsureInvariants() should already have been called. void Validate(bool runOperationValidators, bool isForService) { // contract behaviors ContractDescription contract = this.Contract; for (int j = 0; j < contract.Behaviors.Count; j++) { IContractBehavior iContractBehavior = contract.Behaviors[j]; iContractBehavior.Validate(contract, this); } // endpoint behaviors if (!isForService) { (PartialTrustValidationBehavior.Instance as IEndpointBehavior).Validate(this); (PeerValidationBehavior.Instance as IEndpointBehavior).Validate(this); (TransactionValidationBehavior.Instance as IEndpointBehavior).Validate(this); (SecurityValidationBehavior.Instance as IEndpointBehavior).Validate(this); (System.ServiceModel.MsmqIntegration.MsmqIntegrationValidationBehavior.Instance as IEndpointBehavior).Validate(this); } for (int j = 0; j < this.Behaviors.Count; j++) { IEndpointBehavior ieb = this.Behaviors[j]; ieb.Validate(this); } // operation behaviors if (runOperationValidators) { for (int j = 0; j < contract.Operations.Count; j++) { OperationDescription op = contract.Operations[j]; for (int k = 0; k < op.Behaviors.Count; k++) { IOperationBehavior iob = op.Behaviors[k]; iob.Validate(op); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
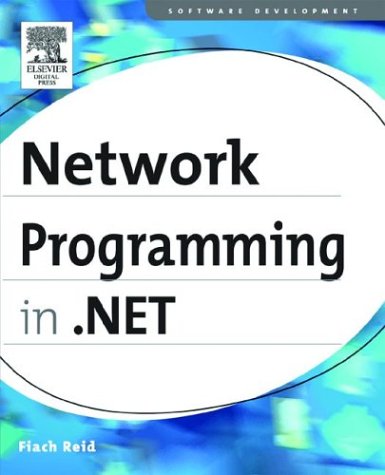
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlyphCollection.cs
- CustomSignedXml.cs
- EventLog.cs
- ModifierKeysConverter.cs
- Encoding.cs
- PrintingPermission.cs
- HttpCookie.cs
- EasingFunctionBase.cs
- SchemaImporterExtensionsSection.cs
- StorageMappingItemLoader.cs
- DynamicFilter.cs
- CodePageEncoding.cs
- IteratorFilter.cs
- TextServicesCompartmentEventSink.cs
- OleDbRowUpdatingEvent.cs
- Exceptions.cs
- NullableLongAverageAggregationOperator.cs
- Win32.cs
- ExpressionBinding.cs
- DataControlCommands.cs
- HtmlTableRow.cs
- EntityClassGenerator.cs
- XmlAnyElementAttribute.cs
- Common.cs
- XmlSerializerNamespaces.cs
- MessageQueue.cs
- Regex.cs
- XPathAncestorQuery.cs
- SqlDuplicator.cs
- DataBindingCollection.cs
- MobileContainerDesigner.cs
- MethodBuilder.cs
- EnumerableCollectionView.cs
- ButtonStandardAdapter.cs
- AutomationElement.cs
- PartitionedStream.cs
- GZipStream.cs
- ValidatingPropertiesEventArgs.cs
- CompilerGeneratedAttribute.cs
- SetStateDesigner.cs
- HostDesigntimeLicenseContext.cs
- ColumnMapTranslator.cs
- XmlDocumentSerializer.cs
- WebConfigurationFileMap.cs
- CodeAccessSecurityEngine.cs
- LoginCancelEventArgs.cs
- Message.cs
- BCryptSafeHandles.cs
- ImageBrush.cs
- UpdateCommand.cs
- List.cs
- RtfToXamlReader.cs
- GiveFeedbackEventArgs.cs
- DbProviderConfigurationHandler.cs
- HtmlElementCollection.cs
- SafeFileHandle.cs
- FormViewInsertEventArgs.cs
- XmlTextReader.cs
- IERequestCache.cs
- CharConverter.cs
- SimpleExpression.cs
- Ipv6Element.cs
- GridViewRowEventArgs.cs
- TableRowCollection.cs
- EncryptedPackage.cs
- SettingsSavedEventArgs.cs
- Tablet.cs
- LinqDataSourceEditData.cs
- TraceHandler.cs
- ParserOptions.cs
- UnsafeNativeMethods.cs
- IndexerNameAttribute.cs
- WriteFileContext.cs
- FileInfo.cs
- SamlDelegatingWriter.cs
- OracleMonthSpan.cs
- OracleDateTime.cs
- AppSettingsReader.cs
- LongTypeConverter.cs
- RuntimeConfig.cs
- AsymmetricCryptoHandle.cs
- ConfigurationElementProperty.cs
- AutomationElement.cs
- ClosableStream.cs
- AutomationElementIdentifiers.cs
- HttpHandlerActionCollection.cs
- BreadCrumbTextConverter.cs
- FieldReference.cs
- FlowNode.cs
- Expressions.cs
- UpdateEventArgs.cs
- Renderer.cs
- XmlILAnnotation.cs
- RequestCachePolicy.cs
- DataGridViewCellMouseEventArgs.cs
- Part.cs
- BufferAllocator.cs
- CrossContextChannel.cs
- ExtenderControl.cs
- XmlMtomReader.cs