Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationTypes / System / Windows / Automation / AutomationElementIdentifiers.cs / 1 / AutomationElementIdentifiers.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: All the Constants that used to be on AutomationElement // // History: // 04/15/2005 : BrendanM Created // //--------------------------------------------------------------------------- using System.Windows.Automation; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using MS.Internal.Automation; #if EVENT_TRACING_PROPERTY using Microsoft.Win32.Diagnostics; #endif // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace System.Windows.Automation { ////// Represents an element in the UIAutomation tree. /// #if (INTERNAL_COMPILE) internal sealed class AutomationElementIdentifiers #else public static class AutomationElementIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ////// Indicates that a element does not support the requested value /// public static readonly object NotSupported = UiaCoreTypesApi.UiaGetReservedNotSupportedValue(); ///Property ID: Indicates that this element should be included in the Control view of the tree public static readonly AutomationProperty IsControlElementProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsControlElement_Property, "AutomationElementIdentifiers.IsControlElementProperty"); ///Property ID: The ControlType of this Element public static readonly AutomationProperty ControlTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.ControlType_Property, "AutomationElementIdentifiers.ControlTypeProperty"); ///Property ID: NativeWindowHandle - Window Handle, if the underlying control is a Window public static readonly AutomationProperty IsContentElementProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsContentElement_Property, "AutomationElementIdentifiers.IsContentElementProperty"); ///Property ID: The AutomationElement that labels this element public static readonly AutomationProperty LabeledByProperty = AutomationProperty.Register(AutomationIdentifierGuids.LabeledBy_Property, "AutomationElementIdentifiers.LabeledByProperty"); ///Property ID: NativeWindowHandle - Window Handle, if the underlying control is a Window public static readonly AutomationProperty NativeWindowHandleProperty = AutomationProperty.Register(AutomationIdentifierGuids.NewNativeWindowHandle_Property, "AutomationElementIdentifiers.NativeWindowHandleProperty"); ///Property ID: AutomationId - An identifier for an element that is unique within its containing element. public static readonly AutomationProperty AutomationIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.AutomationId_Property, "AutomationElementIdentifiers.AutomationIdProperty"); ///Property ID: ItemType - An application-level property used to indicate what the items in a list represent. public static readonly AutomationProperty ItemTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.ItemType_Property, "AutomationElementIdentifiers.ItemTypeProperty"); ///Property ID: True if the control is a password protected field. public static readonly AutomationProperty IsPasswordProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsPassword_Property, "AutomationElementIdentifiers.IsPasswordProperty"); ///Property ID: Localized control type description (eg. "Button") public static readonly AutomationProperty LocalizedControlTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.LocalizedControlType_Property, "AutomationElementIdentifiers.LocalizedControlTypeProperty"); ///Property ID: name of this instance of control public static readonly AutomationProperty NameProperty = AutomationProperty.Register(AutomationIdentifierGuids.Name_Property, "AutomationElementIdentifiers.NameProperty"); ///Property ID: Hot-key equivalent for this command item. (eg. Ctrl-P for Print) public static readonly AutomationProperty AcceleratorKeyProperty = AutomationProperty.Register(AutomationIdentifierGuids.AcceleratorKey_Property, "AutomationElementIdentifiers.AcceleratorKeyProperty"); ///Property ID: Keys used to move focus to this control public static readonly AutomationProperty AccessKeyProperty = AutomationProperty.Register(AutomationIdentifierGuids.AccessKey_Property, "AutomationElementIdentifiers.AccessKeyProperty"); ///Property ID: HasKeyboardFocus public static readonly AutomationProperty HasKeyboardFocusProperty = AutomationProperty.Register(AutomationIdentifierGuids.HasKeyboardFocus_Property, "AutomationElementIdentifiers.HasKeyboardFocusProperty"); ///Property ID: IsKeyboardFocusable public static readonly AutomationProperty IsKeyboardFocusableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsKeyboardFocusable_Property, "AutomationElementIdentifiers.IsKeyboardFocusableProperty"); ///Property ID: Enabled public static readonly AutomationProperty IsEnabledProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsEnabled_Property, "AutomationElementIdentifiers.IsEnabledProperty"); ///Property ID: BoundingRectangle - bounding rectangle public static readonly AutomationProperty BoundingRectangleProperty = AutomationProperty.Register(AutomationIdentifierGuids.BoundingRectangle_Property, "AutomationElementIdentifiers.BoundingRectangleProperty"); ///Property ID: id of process that this element lives in public static readonly AutomationProperty ProcessIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.ProcessId_Property, "AutomationElementIdentifiers.ProcessIdProperty"); ///Property ID: RuntimeId - runtime unique ID public static readonly AutomationProperty RuntimeIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.RuntimeId_Property, "AutomationElementIdentifiers.RuntimeIdProperty"); ///Property ID: ClassName - name of underlying class - implementation dependant, but useful for test public static readonly AutomationProperty ClassNameProperty = AutomationProperty.Register(AutomationIdentifierGuids.ClassName_Property, "AutomationElementIdentifiers.ClassNameProperty"); ///Property ID: HelpText - brief description of what this control does public static readonly AutomationProperty HelpTextProperty = AutomationProperty.Register(AutomationIdentifierGuids.HelpText_Property, "AutomationElementIdentifiers.HelpTextProperty"); ///Property ID: ClickablePoint - Set by provider, used internally for GetClickablePoint public static readonly AutomationProperty ClickablePointProperty = AutomationProperty.Register(AutomationIdentifierGuids.ClickablePoint_Property, "AutomationElementIdentifiers.ClickablePointProperty"); ///Property ID: Culture - Returns the culture that provides information about the control's content. public static readonly AutomationProperty CultureProperty = AutomationProperty.Register(AutomationIdentifierGuids.Culture_Property, "AutomationElementIdentifiers.CultureProperty"); ///Property ID: Offscreen - Determined to be not-visible to the sighted user public static readonly AutomationProperty IsOffscreenProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsOffscreen_Property, "AutomationElementIdentifiers.IsOffscreenProperty"); ///Property ID: Orientation - Identifies whether a control is positioned in a specfic direction public static readonly AutomationProperty OrientationProperty = AutomationProperty.Register(AutomationIdentifierGuids.Orientation_Property, "AutomationElementIdentifiers.OrientationProperty"); ///Property ID: FrameworkId - Identifies the underlying UI framework's name for the element being accessed public static readonly AutomationProperty FrameworkIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.FrameworkId_Property, "AutomationElementIdentifiers.FrameworkIdProperty"); ///Property ID: IsRequiredForForm - Identifies weather an edit field is required to be filled out on a form public static readonly AutomationProperty IsRequiredForFormProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsRequiredForForm_Property, "AutomationElementIdentifiers.IsRequiredForFormProperty"); ///Property ID: ItemStatus - Identifies the status of the visual representation of a complex item public static readonly AutomationProperty ItemStatusProperty = AutomationProperty.Register(AutomationIdentifierGuids.ItemStatus_Property, "AutomationElementIdentifiers.ItemStatusProperty"); #region IsNnnnPatternAvailable properties ///Property that indicates whether the DockPattern is available for this AutomationElement public static readonly AutomationProperty IsDockPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsDockPatternAvailable_Property, "AutomationElementIdentifiers.IsDockPatternAvailableProperty"); ///Property that indicates whether the ExpandCollapsePattern is available for this AutomationElement public static readonly AutomationProperty IsExpandCollapsePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsExpandCollapsePatternAvailable_Property, "AutomationElementIdentifiers.IsExpandCollapsePatternAvailableProperty"); ///Property that indicates whether the GridItemPattern is available for this AutomationElement public static readonly AutomationProperty IsGridItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsGridItemPatternAvailable_Property, "AutomationElementIdentifiers.IsGridItemPatternAvailableProperty"); ///Property that indicates whether the GridPattern is available for this AutomationElement public static readonly AutomationProperty IsGridPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsGridPatternAvailable_Property, "AutomationElementIdentifiers.IsGridPatternAvailableProperty"); ///Property that indicates whether the InvokePattern is available for this AutomationElement public static readonly AutomationProperty IsInvokePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsInvokePatternAvailable_Property, "AutomationElementIdentifiers.IsInvokePatternAvailableProperty"); ///Property that indicates whether the MultipleViewPattern is available for this AutomationElement public static readonly AutomationProperty IsMultipleViewPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsMultipleViewPatternAvailable_Property, "AutomationElementIdentifiers.IsMultipleViewPatternAvailableProperty"); ///Property that indicates whether the RangeValuePattern is available for this AutomationElement public static readonly AutomationProperty IsRangeValuePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsRangeValuePatternAvailable_Property, "AutomationElementIdentifiers.IsRangeValuePatternAvailableProperty"); ///Property that indicates whether the SelectionItemPattern is available for this AutomationElement public static readonly AutomationProperty IsSelectionItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsSelectionItemPatternAvailable_Property, "AutomationElementIdentifiers.IsSelectionItemPatternAvailableProperty"); ///Property that indicates whether the SelectionPattern is available for this AutomationElement public static readonly AutomationProperty IsSelectionPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsSelectionPatternAvailable_Property, "AutomationElementIdentifiers.IsSelectionPatternAvailableProperty"); ///Property that indicates whether the ScrollPattern is available for this AutomationElement public static readonly AutomationProperty IsScrollPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsScrollPatternAvailable_Property, "AutomationElementIdentifiers.IsScrollPatternAvailableProperty"); ///Property that indicates whether the ScrollItemPattern is available for this AutomationElement public static readonly AutomationProperty IsScrollItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsScrollItemPatternAvailable_Property, "AutomationElementIdentifiers.IsScrollItemPatternAvailableProperty"); ///Property that indicates whether the TablePattern is available for this AutomationElement public static readonly AutomationProperty IsTablePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTablePatternAvailable_Property, "AutomationElementIdentifiers.IsTablePatternAvailableProperty"); ///Property that indicates whether the TableItemPattern is available for this AutomationElement public static readonly AutomationProperty IsTableItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTableItemPatternAvailable_Property, "AutomationElementIdentifiers.IsTableItemPatternAvailableProperty"); ///Property that indicates whether the TextPattern is available for this AutomationElement public static readonly AutomationProperty IsTextPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTextPatternAvailable_Property, "AutomationElementIdentifiers.IsTextPatternAvailableProperty"); ///Property that indicates whether the TogglePattern is available for this AutomationElement public static readonly AutomationProperty IsTogglePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTogglePatternAvailable_Property, "AutomationElementIdentifiers.IsTogglePatternAvailableProperty"); ///Property that indicates whether the TransformPattern is available for this AutomationElement public static readonly AutomationProperty IsTransformPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTransformPatternAvailable_Property, "AutomationElementIdentifiers.IsTransformPatternAvailableProperty"); ///Property that indicates whether the ValuePattern is available for this AutomationElement public static readonly AutomationProperty IsValuePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsValuePatternAvailable_Property, "AutomationElementIdentifiers.IsValuePatternAvailableProperty"); ///Property that indicates whether the WindowPattern is available for this AutomationElement public static readonly AutomationProperty IsWindowPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsWindowPatternAvailable_Property, "AutomationElementIdentifiers.IsWindowPatternAvailableProperty"); #endregion IsNnnnPatternAvailable properties #region Events ///Event ID: ToolTipOpenedEvent - indicates a tooltip has appeared public static readonly AutomationEvent ToolTipOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.ToolTipOpened_Event, "AutomationElementIdentifiers.ToolTipOpenedEvent"); ///Event ID: ToolTipClosedEvent - indicates a tooltip has closed. public static readonly AutomationEvent ToolTipClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.ToolTipClosed_Event, "AutomationElementIdentifiers.ToolTipClosedEvent"); ///Event ID: StructureChangedEvent - used mainly by servers to notify of structure changed events. Clients use AddStructureChangedHandler. public static readonly AutomationEvent StructureChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.StructureChanged_Event, "AutomationElementIdentifiers.StructureChangedEvent"); ///Event ID: MenuOpened - Indicates an a menu has opened. public static readonly AutomationEvent MenuOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.MenuOpened_Event, "AutomationElementIdentifiers.MenuOpenedEvent"); ///Event ID: AutomationPropertyChangedEvent - used mainly by servers to notify of property changes. Clients use AddPropertyChangedListener. public static readonly AutomationEvent AutomationPropertyChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AutomationPropertyChanged_Event, "AutomationElementIdentifiers.AutomationPropertyChangedEvent"); ///Event ID: AutomationFocusChangedEvent - used mainly by servers to notify of focus changed events. Clients use AddAutomationFocusChangedListener. public static readonly AutomationEvent AutomationFocusChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AutomationFocusChanged_Event, "AutomationElementIdentifiers.AutomationFocusChangedEvent"); ///Event ID: AsyncContentLoadedEvent - indicates an async content loaded event. public static readonly AutomationEvent AsyncContentLoadedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AsyncContentLoaded_Event, "AutomationElementIdentifiers.AsyncContentLoadedEvent"); ///Event ID: MenuClosed - Indicates an a menu has closed. public static readonly AutomationEvent MenuClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.MenuClosed_Event, "AutomationElementIdentifiers.MenuClosedEvent"); ///Event ID: LayoutInvalidated - Indicates that many element locations/extents/offscreenedness have changed. public static readonly AutomationEvent LayoutInvalidatedEvent = AutomationEvent.Register(AutomationIdentifierGuids.LayoutInvalidated_Event, "AutomationElementIdentifiers.LayoutInvalidatedEvent"); #endregion Events #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: All the Constants that used to be on AutomationElement // // History: // 04/15/2005 : BrendanM Created // //--------------------------------------------------------------------------- using System.Windows.Automation; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using MS.Internal.Automation; #if EVENT_TRACING_PROPERTY using Microsoft.Win32.Diagnostics; #endif // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace System.Windows.Automation { ////// Represents an element in the UIAutomation tree. /// #if (INTERNAL_COMPILE) internal sealed class AutomationElementIdentifiers #else public static class AutomationElementIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ////// Indicates that a element does not support the requested value /// public static readonly object NotSupported = UiaCoreTypesApi.UiaGetReservedNotSupportedValue(); ///Property ID: Indicates that this element should be included in the Control view of the tree public static readonly AutomationProperty IsControlElementProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsControlElement_Property, "AutomationElementIdentifiers.IsControlElementProperty"); ///Property ID: The ControlType of this Element public static readonly AutomationProperty ControlTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.ControlType_Property, "AutomationElementIdentifiers.ControlTypeProperty"); ///Property ID: NativeWindowHandle - Window Handle, if the underlying control is a Window public static readonly AutomationProperty IsContentElementProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsContentElement_Property, "AutomationElementIdentifiers.IsContentElementProperty"); ///Property ID: The AutomationElement that labels this element public static readonly AutomationProperty LabeledByProperty = AutomationProperty.Register(AutomationIdentifierGuids.LabeledBy_Property, "AutomationElementIdentifiers.LabeledByProperty"); ///Property ID: NativeWindowHandle - Window Handle, if the underlying control is a Window public static readonly AutomationProperty NativeWindowHandleProperty = AutomationProperty.Register(AutomationIdentifierGuids.NewNativeWindowHandle_Property, "AutomationElementIdentifiers.NativeWindowHandleProperty"); ///Property ID: AutomationId - An identifier for an element that is unique within its containing element. public static readonly AutomationProperty AutomationIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.AutomationId_Property, "AutomationElementIdentifiers.AutomationIdProperty"); ///Property ID: ItemType - An application-level property used to indicate what the items in a list represent. public static readonly AutomationProperty ItemTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.ItemType_Property, "AutomationElementIdentifiers.ItemTypeProperty"); ///Property ID: True if the control is a password protected field. public static readonly AutomationProperty IsPasswordProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsPassword_Property, "AutomationElementIdentifiers.IsPasswordProperty"); ///Property ID: Localized control type description (eg. "Button") public static readonly AutomationProperty LocalizedControlTypeProperty = AutomationProperty.Register(AutomationIdentifierGuids.LocalizedControlType_Property, "AutomationElementIdentifiers.LocalizedControlTypeProperty"); ///Property ID: name of this instance of control public static readonly AutomationProperty NameProperty = AutomationProperty.Register(AutomationIdentifierGuids.Name_Property, "AutomationElementIdentifiers.NameProperty"); ///Property ID: Hot-key equivalent for this command item. (eg. Ctrl-P for Print) public static readonly AutomationProperty AcceleratorKeyProperty = AutomationProperty.Register(AutomationIdentifierGuids.AcceleratorKey_Property, "AutomationElementIdentifiers.AcceleratorKeyProperty"); ///Property ID: Keys used to move focus to this control public static readonly AutomationProperty AccessKeyProperty = AutomationProperty.Register(AutomationIdentifierGuids.AccessKey_Property, "AutomationElementIdentifiers.AccessKeyProperty"); ///Property ID: HasKeyboardFocus public static readonly AutomationProperty HasKeyboardFocusProperty = AutomationProperty.Register(AutomationIdentifierGuids.HasKeyboardFocus_Property, "AutomationElementIdentifiers.HasKeyboardFocusProperty"); ///Property ID: IsKeyboardFocusable public static readonly AutomationProperty IsKeyboardFocusableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsKeyboardFocusable_Property, "AutomationElementIdentifiers.IsKeyboardFocusableProperty"); ///Property ID: Enabled public static readonly AutomationProperty IsEnabledProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsEnabled_Property, "AutomationElementIdentifiers.IsEnabledProperty"); ///Property ID: BoundingRectangle - bounding rectangle public static readonly AutomationProperty BoundingRectangleProperty = AutomationProperty.Register(AutomationIdentifierGuids.BoundingRectangle_Property, "AutomationElementIdentifiers.BoundingRectangleProperty"); ///Property ID: id of process that this element lives in public static readonly AutomationProperty ProcessIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.ProcessId_Property, "AutomationElementIdentifiers.ProcessIdProperty"); ///Property ID: RuntimeId - runtime unique ID public static readonly AutomationProperty RuntimeIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.RuntimeId_Property, "AutomationElementIdentifiers.RuntimeIdProperty"); ///Property ID: ClassName - name of underlying class - implementation dependant, but useful for test public static readonly AutomationProperty ClassNameProperty = AutomationProperty.Register(AutomationIdentifierGuids.ClassName_Property, "AutomationElementIdentifiers.ClassNameProperty"); ///Property ID: HelpText - brief description of what this control does public static readonly AutomationProperty HelpTextProperty = AutomationProperty.Register(AutomationIdentifierGuids.HelpText_Property, "AutomationElementIdentifiers.HelpTextProperty"); ///Property ID: ClickablePoint - Set by provider, used internally for GetClickablePoint public static readonly AutomationProperty ClickablePointProperty = AutomationProperty.Register(AutomationIdentifierGuids.ClickablePoint_Property, "AutomationElementIdentifiers.ClickablePointProperty"); ///Property ID: Culture - Returns the culture that provides information about the control's content. public static readonly AutomationProperty CultureProperty = AutomationProperty.Register(AutomationIdentifierGuids.Culture_Property, "AutomationElementIdentifiers.CultureProperty"); ///Property ID: Offscreen - Determined to be not-visible to the sighted user public static readonly AutomationProperty IsOffscreenProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsOffscreen_Property, "AutomationElementIdentifiers.IsOffscreenProperty"); ///Property ID: Orientation - Identifies whether a control is positioned in a specfic direction public static readonly AutomationProperty OrientationProperty = AutomationProperty.Register(AutomationIdentifierGuids.Orientation_Property, "AutomationElementIdentifiers.OrientationProperty"); ///Property ID: FrameworkId - Identifies the underlying UI framework's name for the element being accessed public static readonly AutomationProperty FrameworkIdProperty = AutomationProperty.Register(AutomationIdentifierGuids.FrameworkId_Property, "AutomationElementIdentifiers.FrameworkIdProperty"); ///Property ID: IsRequiredForForm - Identifies weather an edit field is required to be filled out on a form public static readonly AutomationProperty IsRequiredForFormProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsRequiredForForm_Property, "AutomationElementIdentifiers.IsRequiredForFormProperty"); ///Property ID: ItemStatus - Identifies the status of the visual representation of a complex item public static readonly AutomationProperty ItemStatusProperty = AutomationProperty.Register(AutomationIdentifierGuids.ItemStatus_Property, "AutomationElementIdentifiers.ItemStatusProperty"); #region IsNnnnPatternAvailable properties ///Property that indicates whether the DockPattern is available for this AutomationElement public static readonly AutomationProperty IsDockPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsDockPatternAvailable_Property, "AutomationElementIdentifiers.IsDockPatternAvailableProperty"); ///Property that indicates whether the ExpandCollapsePattern is available for this AutomationElement public static readonly AutomationProperty IsExpandCollapsePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsExpandCollapsePatternAvailable_Property, "AutomationElementIdentifiers.IsExpandCollapsePatternAvailableProperty"); ///Property that indicates whether the GridItemPattern is available for this AutomationElement public static readonly AutomationProperty IsGridItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsGridItemPatternAvailable_Property, "AutomationElementIdentifiers.IsGridItemPatternAvailableProperty"); ///Property that indicates whether the GridPattern is available for this AutomationElement public static readonly AutomationProperty IsGridPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsGridPatternAvailable_Property, "AutomationElementIdentifiers.IsGridPatternAvailableProperty"); ///Property that indicates whether the InvokePattern is available for this AutomationElement public static readonly AutomationProperty IsInvokePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsInvokePatternAvailable_Property, "AutomationElementIdentifiers.IsInvokePatternAvailableProperty"); ///Property that indicates whether the MultipleViewPattern is available for this AutomationElement public static readonly AutomationProperty IsMultipleViewPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsMultipleViewPatternAvailable_Property, "AutomationElementIdentifiers.IsMultipleViewPatternAvailableProperty"); ///Property that indicates whether the RangeValuePattern is available for this AutomationElement public static readonly AutomationProperty IsRangeValuePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsRangeValuePatternAvailable_Property, "AutomationElementIdentifiers.IsRangeValuePatternAvailableProperty"); ///Property that indicates whether the SelectionItemPattern is available for this AutomationElement public static readonly AutomationProperty IsSelectionItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsSelectionItemPatternAvailable_Property, "AutomationElementIdentifiers.IsSelectionItemPatternAvailableProperty"); ///Property that indicates whether the SelectionPattern is available for this AutomationElement public static readonly AutomationProperty IsSelectionPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsSelectionPatternAvailable_Property, "AutomationElementIdentifiers.IsSelectionPatternAvailableProperty"); ///Property that indicates whether the ScrollPattern is available for this AutomationElement public static readonly AutomationProperty IsScrollPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsScrollPatternAvailable_Property, "AutomationElementIdentifiers.IsScrollPatternAvailableProperty"); ///Property that indicates whether the ScrollItemPattern is available for this AutomationElement public static readonly AutomationProperty IsScrollItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsScrollItemPatternAvailable_Property, "AutomationElementIdentifiers.IsScrollItemPatternAvailableProperty"); ///Property that indicates whether the TablePattern is available for this AutomationElement public static readonly AutomationProperty IsTablePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTablePatternAvailable_Property, "AutomationElementIdentifiers.IsTablePatternAvailableProperty"); ///Property that indicates whether the TableItemPattern is available for this AutomationElement public static readonly AutomationProperty IsTableItemPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTableItemPatternAvailable_Property, "AutomationElementIdentifiers.IsTableItemPatternAvailableProperty"); ///Property that indicates whether the TextPattern is available for this AutomationElement public static readonly AutomationProperty IsTextPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTextPatternAvailable_Property, "AutomationElementIdentifiers.IsTextPatternAvailableProperty"); ///Property that indicates whether the TogglePattern is available for this AutomationElement public static readonly AutomationProperty IsTogglePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTogglePatternAvailable_Property, "AutomationElementIdentifiers.IsTogglePatternAvailableProperty"); ///Property that indicates whether the TransformPattern is available for this AutomationElement public static readonly AutomationProperty IsTransformPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsTransformPatternAvailable_Property, "AutomationElementIdentifiers.IsTransformPatternAvailableProperty"); ///Property that indicates whether the ValuePattern is available for this AutomationElement public static readonly AutomationProperty IsValuePatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsValuePatternAvailable_Property, "AutomationElementIdentifiers.IsValuePatternAvailableProperty"); ///Property that indicates whether the WindowPattern is available for this AutomationElement public static readonly AutomationProperty IsWindowPatternAvailableProperty = AutomationProperty.Register(AutomationIdentifierGuids.IsWindowPatternAvailable_Property, "AutomationElementIdentifiers.IsWindowPatternAvailableProperty"); #endregion IsNnnnPatternAvailable properties #region Events ///Event ID: ToolTipOpenedEvent - indicates a tooltip has appeared public static readonly AutomationEvent ToolTipOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.ToolTipOpened_Event, "AutomationElementIdentifiers.ToolTipOpenedEvent"); ///Event ID: ToolTipClosedEvent - indicates a tooltip has closed. public static readonly AutomationEvent ToolTipClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.ToolTipClosed_Event, "AutomationElementIdentifiers.ToolTipClosedEvent"); ///Event ID: StructureChangedEvent - used mainly by servers to notify of structure changed events. Clients use AddStructureChangedHandler. public static readonly AutomationEvent StructureChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.StructureChanged_Event, "AutomationElementIdentifiers.StructureChangedEvent"); ///Event ID: MenuOpened - Indicates an a menu has opened. public static readonly AutomationEvent MenuOpenedEvent = AutomationEvent.Register(AutomationIdentifierGuids.MenuOpened_Event, "AutomationElementIdentifiers.MenuOpenedEvent"); ///Event ID: AutomationPropertyChangedEvent - used mainly by servers to notify of property changes. Clients use AddPropertyChangedListener. public static readonly AutomationEvent AutomationPropertyChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AutomationPropertyChanged_Event, "AutomationElementIdentifiers.AutomationPropertyChangedEvent"); ///Event ID: AutomationFocusChangedEvent - used mainly by servers to notify of focus changed events. Clients use AddAutomationFocusChangedListener. public static readonly AutomationEvent AutomationFocusChangedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AutomationFocusChanged_Event, "AutomationElementIdentifiers.AutomationFocusChangedEvent"); ///Event ID: AsyncContentLoadedEvent - indicates an async content loaded event. public static readonly AutomationEvent AsyncContentLoadedEvent = AutomationEvent.Register(AutomationIdentifierGuids.AsyncContentLoaded_Event, "AutomationElementIdentifiers.AsyncContentLoadedEvent"); ///Event ID: MenuClosed - Indicates an a menu has closed. public static readonly AutomationEvent MenuClosedEvent = AutomationEvent.Register(AutomationIdentifierGuids.MenuClosed_Event, "AutomationElementIdentifiers.MenuClosedEvent"); ///Event ID: LayoutInvalidated - Indicates that many element locations/extents/offscreenedness have changed. public static readonly AutomationEvent LayoutInvalidatedEvent = AutomationEvent.Register(AutomationIdentifierGuids.LayoutInvalidated_Event, "AutomationElementIdentifiers.LayoutInvalidatedEvent"); #endregion Events #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
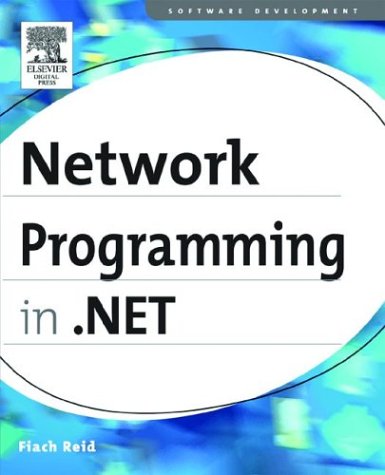
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExceptionAggregator.cs
- WebSysDescriptionAttribute.cs
- Enum.cs
- ComponentEditorForm.cs
- Msec.cs
- QueryExtender.cs
- ExpressionBinding.cs
- ConstNode.cs
- NamespaceCollection.cs
- TreeNodeCollection.cs
- BaseTemplateBuildProvider.cs
- StorageFunctionMapping.cs
- AutomationAttributeInfo.cs
- MergePropertyDescriptor.cs
- ListControlDataBindingHandler.cs
- StorageEntitySetMapping.cs
- COM2TypeInfoProcessor.cs
- DataServiceHostFactory.cs
- CellLabel.cs
- Cursor.cs
- FixedSOMTextRun.cs
- Panel.cs
- StyleSheetDesigner.cs
- SeverityFilter.cs
- SourceInterpreter.cs
- LineBreak.cs
- RectConverter.cs
- mactripleDES.cs
- StylesEditorDialog.cs
- EntityException.cs
- SpecularMaterial.cs
- JsonReader.cs
- TransactionInformation.cs
- TypedElement.cs
- WindowsScroll.cs
- Int16Converter.cs
- DataException.cs
- WebPartEditorCancelVerb.cs
- PartialClassGenerationTaskInternal.cs
- InstanceData.cs
- MimePart.cs
- DateRangeEvent.cs
- LoginUtil.cs
- XPathNodeInfoAtom.cs
- SiteMapDataSource.cs
- TypeResolver.cs
- ByteFacetDescriptionElement.cs
- DynamicExpression.cs
- HotSpot.cs
- WebPartPersonalization.cs
- ExecutionContext.cs
- CommonProperties.cs
- DBProviderConfigurationHandler.cs
- TabItem.cs
- WorkflowControlEndpoint.cs
- RemotingException.cs
- XPathDescendantIterator.cs
- SQLByteStorage.cs
- X509Utils.cs
- DataPagerField.cs
- NavigationEventArgs.cs
- SoapEnvelopeProcessingElement.cs
- DiscoveryClientReferences.cs
- QueryExpression.cs
- WebUtil.cs
- ObjectViewListener.cs
- MimeBasePart.cs
- CalendarDataBindingHandler.cs
- ServerIdentity.cs
- RelationshipConverter.cs
- CultureTable.cs
- ElementUtil.cs
- TokenBasedSetEnumerator.cs
- ExclusiveCanonicalizationTransform.cs
- KnowledgeBase.cs
- PaperSource.cs
- WebRequestModuleElement.cs
- TabItemWrapperAutomationPeer.cs
- HandlerBase.cs
- DigestTraceRecordHelper.cs
- RelationshipConverter.cs
- UpdatePanel.cs
- SchemaImporterExtensionsSection.cs
- arabicshape.cs
- JapaneseCalendar.cs
- AnonymousIdentificationSection.cs
- TableRowGroupCollection.cs
- SqlCacheDependencyDatabase.cs
- DllNotFoundException.cs
- ActivationWorker.cs
- WebPartDisplayModeCancelEventArgs.cs
- ServicesUtilities.cs
- StatusBarDrawItemEvent.cs
- AuthenticationModuleElementCollection.cs
- AttachedAnnotationChangedEventArgs.cs
- GridViewEditEventArgs.cs
- SystemIPv6InterfaceProperties.cs
- RoleManagerEventArgs.cs
- PropertyEntry.cs
- TextPenaltyModule.cs