Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageFunctionMapping.cs / 1407647 / StorageFunctionMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; using System.Linq; namespace System.Data.Mapping { ////// Describes function mappings for an association set. /// internal sealed class StorageAssociationSetFunctionMapping { internal StorageAssociationSetFunctionMapping( AssociationSet associationSet, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping) { this.AssociationSet = EntityUtil.CheckArgumentNull(associationSet, "associationSet"); this.DeleteFunctionMapping = deleteFunctionMapping; this.InsertFunctionMapping = insertFunctionMapping; } ////// Association set these functions handles. /// internal readonly AssociationSet AssociationSet; ////// Delete function for this association set. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Insert function for this association set. /// internal readonly StorageFunctionMapping InsertFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "AS{{{0}}}:{3}DFunc={{{1}}},{3}IFunc={{{2}}}", AssociationSet, DeleteFunctionMapping, InsertFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Association Set Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function mappings for an entity type within an entity set. /// internal sealed class StorageEntityTypeFunctionMapping { internal StorageEntityTypeFunctionMapping( EntityType entityType, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping, StorageFunctionMapping updateFunctionMapping) { this.EntityType = EntityUtil.CheckArgumentNull(entityType, "entityType"); this.DeleteFunctionMapping = deleteFunctionMapping; this.InsertFunctionMapping = insertFunctionMapping; this.UpdateFunctionMapping = updateFunctionMapping; } ////// Gets (specific) entity type these functions handle. /// internal readonly EntityType EntityType; ////// Gets delete function for the current entity type. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Gets insert function for the current entity type. /// internal readonly StorageFunctionMapping InsertFunctionMapping; ////// Gets update function for the current entity type. /// internal readonly StorageFunctionMapping UpdateFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "ET{{{0}}}:{4}DFunc={{{1}}},{4}IFunc={{{2}}},{4}UFunc={{{3}}}", EntityType, DeleteFunctionMapping, InsertFunctionMapping, UpdateFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Entity Type Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function binding for change processing of entities or associations. /// internal sealed class StorageFunctionMapping { internal StorageFunctionMapping(EntitySetBase entitySet, EntityTypeBase entityType, EdmFunction function, IEnumerableparameterBindings, FunctionParameter rowsAffectedParameter, IEnumerable resultBindings) { EntityUtil.CheckArgumentNull(entitySet, "entitySet"); this.Function = EntityUtil.CheckArgumentNull(function, "function"); this.RowsAffectedParameter = rowsAffectedParameter; this.ParameterBindings = EntityUtil.CheckArgumentNull(parameterBindings, "parameterBindings") .ToList().AsReadOnly(); if (null != resultBindings) { List bindings = resultBindings.ToList(); if (0 < bindings.Count) { ResultBindings = bindings.AsReadOnly(); } } this.CollocatedAssociationSetEnds = GetReferencedAssociationSetEnds(entitySet as EntitySet, entityType as EntityType, parameterBindings) .ToList() .AsReadOnly(); } /// /// Gets output parameter producing number of rows affected. May be null. /// internal readonly FunctionParameter RowsAffectedParameter; ////// Gets Metadata of function to which we should bind. /// internal readonly EdmFunction Function; ////// Gets bindings for function parameters. /// internal readonly ReadOnlyCollectionParameterBindings; /// /// Gets all association set ends collocated in this mapping. /// internal readonly ReadOnlyCollectionCollocatedAssociationSetEnds; /// /// Gets bindings for the results of function evaluation. /// internal readonly ReadOnlyCollectionResultBindings; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "Func{{{0}}}: Prm={{{1}}}, Result={{{2}}}", Function, StringUtil.ToCommaSeparatedStringSorted(ParameterBindings), StringUtil.ToCommaSeparatedStringSorted(ResultBindings)); } // requires: entitySet must not be null // Yields all referenced association set ends in this mapping. private static IEnumerable GetReferencedAssociationSetEnds(EntitySet entitySet, EntityType entityType, IEnumerable parameterBindings) { HashSet ends = new HashSet (); if (null != entitySet && null != entityType) { foreach (StorageFunctionParameterBinding parameterBinding in parameterBindings) { AssociationSetEnd end = parameterBinding.MemberPath.AssociationSetEnd; if (null != end) { ends.Add(end); } } // If there is a referential constraint, it counts as an implicit mapping of // the association set foreach (AssociationSet assocationSet in MetadataHelper.GetAssociationsForEntitySet(entitySet)) { ReadOnlyMetadataCollection constraints = assocationSet.ElementType.ReferentialConstraints; if (null != constraints) { foreach (ReferentialConstraint constraint in constraints) { if ( (assocationSet.AssociationSetEnds[constraint.ToRole.Name].EntitySet == entitySet) && (constraint.ToRole.GetEntityType().IsAssignableFrom(entityType))) { ends.Add(assocationSet.AssociationSetEnds[constraint.FromRole.Name]); } } } } } return ends; } } /// /// Defines a binding from a named result set column to a member taking the value. /// internal sealed class StorageFunctionResultBinding { internal StorageFunctionResultBinding(string columnName, EdmProperty property) { this.ColumnName = EntityUtil.CheckArgumentNull(columnName, "columnName"); this.Property = EntityUtil.CheckArgumentNull(property, "property"); } ////// Gets the name of the column to bind from the function result set. We use a string /// value rather than EdmMember, since there is no metadata for function result sets. /// internal readonly string ColumnName; ////// Gets the property to be set on the entity. /// internal readonly EdmProperty Property; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}->{1}", ColumnName, Property); } } ////// Binds a function parameter to a member of the entity or association being modified. /// internal sealed class StorageFunctionParameterBinding { internal StorageFunctionParameterBinding(FunctionParameter parameter, StorageFunctionMemberPath memberPath, bool isCurrent) { this.Parameter = EntityUtil.CheckArgumentNull(parameter, "parameter"); this.MemberPath = EntityUtil.CheckArgumentNull(memberPath, "memberPath"); this.IsCurrent = isCurrent; } ////// Gets the parameter taking the value. /// internal readonly FunctionParameter Parameter; ////// Gets the path to the entity or association member defining the value. /// internal readonly StorageFunctionMemberPath MemberPath; ////// Gets a value indicating whether the current or original /// member value is being bound. /// internal readonly bool IsCurrent; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "@{0}->{1}{2}", Parameter, IsCurrent ? "+" : "-", MemberPath); } } ////// Describes the location of a member within an entity or association type structure. /// internal sealed class StorageFunctionMemberPath { internal StorageFunctionMemberPath(IEnumerablemembers, AssociationSet associationSetNavigation) { this.Members = new ReadOnlyCollection (new List ( EntityUtil.CheckArgumentNull(members, "members"))); if (null != associationSetNavigation) { Debug.Assert(2 == this.Members.Count, "Association bindings must always consist of the end and the key"); // find the association set end this.AssociationSetEnd = associationSetNavigation.AssociationSetEnds[this.Members[1].Name]; } } /// /// Gets the members in the path from the leaf (the member being bound) /// to the Root of the structure. /// internal readonly ReadOnlyCollectionMembers; /// /// Gets the association set to which we are navigating via this member. If the value /// is null, this is not a navigation member path. /// internal readonly AssociationSetEnd AssociationSetEnd; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}{1}", null == AssociationSetEnd ? String.Empty : "[" + AssociationSetEnd.ParentAssociationSet.ToString() + "]", StringUtil.BuildDelimitedList(Members, null, ".")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; using System.Linq; namespace System.Data.Mapping { ////// Describes function mappings for an association set. /// internal sealed class StorageAssociationSetFunctionMapping { internal StorageAssociationSetFunctionMapping( AssociationSet associationSet, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping) { this.AssociationSet = EntityUtil.CheckArgumentNull(associationSet, "associationSet"); this.DeleteFunctionMapping = deleteFunctionMapping; this.InsertFunctionMapping = insertFunctionMapping; } ////// Association set these functions handles. /// internal readonly AssociationSet AssociationSet; ////// Delete function for this association set. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Insert function for this association set. /// internal readonly StorageFunctionMapping InsertFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "AS{{{0}}}:{3}DFunc={{{1}}},{3}IFunc={{{2}}}", AssociationSet, DeleteFunctionMapping, InsertFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Association Set Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function mappings for an entity type within an entity set. /// internal sealed class StorageEntityTypeFunctionMapping { internal StorageEntityTypeFunctionMapping( EntityType entityType, StorageFunctionMapping deleteFunctionMapping, StorageFunctionMapping insertFunctionMapping, StorageFunctionMapping updateFunctionMapping) { this.EntityType = EntityUtil.CheckArgumentNull(entityType, "entityType"); this.DeleteFunctionMapping = deleteFunctionMapping; this.InsertFunctionMapping = insertFunctionMapping; this.UpdateFunctionMapping = updateFunctionMapping; } ////// Gets (specific) entity type these functions handle. /// internal readonly EntityType EntityType; ////// Gets delete function for the current entity type. /// internal readonly StorageFunctionMapping DeleteFunctionMapping; ////// Gets insert function for the current entity type. /// internal readonly StorageFunctionMapping InsertFunctionMapping; ////// Gets update function for the current entity type. /// internal readonly StorageFunctionMapping UpdateFunctionMapping; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "ET{{{0}}}:{4}DFunc={{{1}}},{4}IFunc={{{2}}},{4}UFunc={{{3}}}", EntityType, DeleteFunctionMapping, InsertFunctionMapping, UpdateFunctionMapping, Environment.NewLine + " "); } internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("Entity Type Function Mapping"); sb.Append(" "); sb.Append(this.ToString()); Console.WriteLine(sb.ToString()); } } ////// Describes function binding for change processing of entities or associations. /// internal sealed class StorageFunctionMapping { internal StorageFunctionMapping(EntitySetBase entitySet, EntityTypeBase entityType, EdmFunction function, IEnumerableparameterBindings, FunctionParameter rowsAffectedParameter, IEnumerable resultBindings) { EntityUtil.CheckArgumentNull(entitySet, "entitySet"); this.Function = EntityUtil.CheckArgumentNull(function, "function"); this.RowsAffectedParameter = rowsAffectedParameter; this.ParameterBindings = EntityUtil.CheckArgumentNull(parameterBindings, "parameterBindings") .ToList().AsReadOnly(); if (null != resultBindings) { List bindings = resultBindings.ToList(); if (0 < bindings.Count) { ResultBindings = bindings.AsReadOnly(); } } this.CollocatedAssociationSetEnds = GetReferencedAssociationSetEnds(entitySet as EntitySet, entityType as EntityType, parameterBindings) .ToList() .AsReadOnly(); } /// /// Gets output parameter producing number of rows affected. May be null. /// internal readonly FunctionParameter RowsAffectedParameter; ////// Gets Metadata of function to which we should bind. /// internal readonly EdmFunction Function; ////// Gets bindings for function parameters. /// internal readonly ReadOnlyCollectionParameterBindings; /// /// Gets all association set ends collocated in this mapping. /// internal readonly ReadOnlyCollectionCollocatedAssociationSetEnds; /// /// Gets bindings for the results of function evaluation. /// internal readonly ReadOnlyCollectionResultBindings; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "Func{{{0}}}: Prm={{{1}}}, Result={{{2}}}", Function, StringUtil.ToCommaSeparatedStringSorted(ParameterBindings), StringUtil.ToCommaSeparatedStringSorted(ResultBindings)); } // requires: entitySet must not be null // Yields all referenced association set ends in this mapping. private static IEnumerable GetReferencedAssociationSetEnds(EntitySet entitySet, EntityType entityType, IEnumerable parameterBindings) { HashSet ends = new HashSet (); if (null != entitySet && null != entityType) { foreach (StorageFunctionParameterBinding parameterBinding in parameterBindings) { AssociationSetEnd end = parameterBinding.MemberPath.AssociationSetEnd; if (null != end) { ends.Add(end); } } // If there is a referential constraint, it counts as an implicit mapping of // the association set foreach (AssociationSet assocationSet in MetadataHelper.GetAssociationsForEntitySet(entitySet)) { ReadOnlyMetadataCollection constraints = assocationSet.ElementType.ReferentialConstraints; if (null != constraints) { foreach (ReferentialConstraint constraint in constraints) { if ( (assocationSet.AssociationSetEnds[constraint.ToRole.Name].EntitySet == entitySet) && (constraint.ToRole.GetEntityType().IsAssignableFrom(entityType))) { ends.Add(assocationSet.AssociationSetEnds[constraint.FromRole.Name]); } } } } } return ends; } } /// /// Defines a binding from a named result set column to a member taking the value. /// internal sealed class StorageFunctionResultBinding { internal StorageFunctionResultBinding(string columnName, EdmProperty property) { this.ColumnName = EntityUtil.CheckArgumentNull(columnName, "columnName"); this.Property = EntityUtil.CheckArgumentNull(property, "property"); } ////// Gets the name of the column to bind from the function result set. We use a string /// value rather than EdmMember, since there is no metadata for function result sets. /// internal readonly string ColumnName; ////// Gets the property to be set on the entity. /// internal readonly EdmProperty Property; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}->{1}", ColumnName, Property); } } ////// Binds a function parameter to a member of the entity or association being modified. /// internal sealed class StorageFunctionParameterBinding { internal StorageFunctionParameterBinding(FunctionParameter parameter, StorageFunctionMemberPath memberPath, bool isCurrent) { this.Parameter = EntityUtil.CheckArgumentNull(parameter, "parameter"); this.MemberPath = EntityUtil.CheckArgumentNull(memberPath, "memberPath"); this.IsCurrent = isCurrent; } ////// Gets the parameter taking the value. /// internal readonly FunctionParameter Parameter; ////// Gets the path to the entity or association member defining the value. /// internal readonly StorageFunctionMemberPath MemberPath; ////// Gets a value indicating whether the current or original /// member value is being bound. /// internal readonly bool IsCurrent; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "@{0}->{1}{2}", Parameter, IsCurrent ? "+" : "-", MemberPath); } } ////// Describes the location of a member within an entity or association type structure. /// internal sealed class StorageFunctionMemberPath { internal StorageFunctionMemberPath(IEnumerablemembers, AssociationSet associationSetNavigation) { this.Members = new ReadOnlyCollection (new List ( EntityUtil.CheckArgumentNull(members, "members"))); if (null != associationSetNavigation) { Debug.Assert(2 == this.Members.Count, "Association bindings must always consist of the end and the key"); // find the association set end this.AssociationSetEnd = associationSetNavigation.AssociationSetEnds[this.Members[1].Name]; } } /// /// Gets the members in the path from the leaf (the member being bound) /// to the Root of the structure. /// internal readonly ReadOnlyCollectionMembers; /// /// Gets the association set to which we are navigating via this member. If the value /// is null, this is not a navigation member path. /// internal readonly AssociationSetEnd AssociationSetEnd; public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}{1}", null == AssociationSetEnd ? String.Empty : "[" + AssociationSetEnd.ParentAssociationSet.ToString() + "]", StringUtil.BuildDelimitedList(Members, null, ".")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
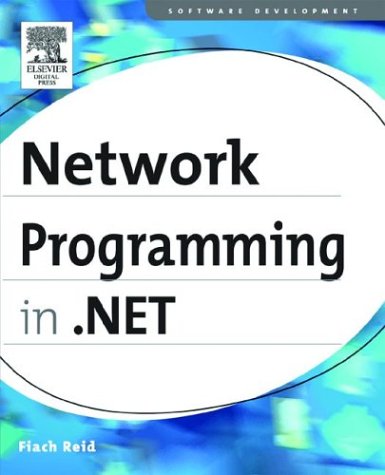
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionContext.cs
- AudioStateChangedEventArgs.cs
- KeyMatchBuilder.cs
- Win32PrintDialog.cs
- HtmlTableCellCollection.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- HttpDictionary.cs
- Mapping.cs
- RowToParametersTransformer.cs
- SubMenuStyle.cs
- Itemizer.cs
- DataTemplateKey.cs
- HandlerMappingMemo.cs
- ResXResourceWriter.cs
- BidirectionalDictionary.cs
- DrawingAttributes.cs
- ProxyRpc.cs
- CustomSignedXml.cs
- DesignerTextViewAdapter.cs
- ContainerControl.cs
- SignedXml.cs
- SqlUserDefinedAggregateAttribute.cs
- PenContext.cs
- TypeAccessException.cs
- _Win32.cs
- CharAnimationUsingKeyFrames.cs
- CommonDialog.cs
- RelatedCurrencyManager.cs
- ServiceDiscoveryBehavior.cs
- IFlowDocumentViewer.cs
- XmlStreamStore.cs
- MultiSelector.cs
- UnsafeNativeMethods.cs
- Vector3D.cs
- RoleGroup.cs
- IgnorePropertiesAttribute.cs
- SchemaImporterExtensionElementCollection.cs
- UIElement3D.cs
- Compress.cs
- EncryptedKeyHashIdentifierClause.cs
- LogRecordSequence.cs
- EditCommandColumn.cs
- DiffuseMaterial.cs
- OracleInternalConnection.cs
- CodePrimitiveExpression.cs
- TabControlToolboxItem.cs
- XmlSchemaSimpleContent.cs
- DbConnectionPoolGroup.cs
- DataGridViewLinkCell.cs
- EmptyControlCollection.cs
- DataGridViewBand.cs
- XmlSchemaSequence.cs
- XPathSingletonIterator.cs
- StaticExtensionConverter.cs
- DataSet.cs
- UniqueIdentifierService.cs
- PropertyGridCommands.cs
- Track.cs
- DeferredRunTextReference.cs
- WorkflowApplicationException.cs
- Identity.cs
- ResourceReferenceKeyNotFoundException.cs
- MetadataItem_Static.cs
- NCryptNative.cs
- TabItemWrapperAutomationPeer.cs
- RightNameExpirationInfoPair.cs
- AdPostCacheSubstitution.cs
- RuntimeComponentFilter.cs
- DiscoveryDocument.cs
- ImageCodecInfoPrivate.cs
- MenuItem.cs
- HttpListenerPrefixCollection.cs
- CounterSampleCalculator.cs
- DoubleAverageAggregationOperator.cs
- OracleCommand.cs
- SmtpReplyReader.cs
- XmlSchemaComplexContentRestriction.cs
- PermissionToken.cs
- GroupItem.cs
- RIPEMD160Managed.cs
- GroupBox.cs
- XPathMessageFilterElementCollection.cs
- ViewLoader.cs
- StylusButtonCollection.cs
- AnnotationStore.cs
- CodeDesigner.cs
- LoginCancelEventArgs.cs
- Transform.cs
- Shared.cs
- EventSource.cs
- DesignerVerbToolStripMenuItem.cs
- FixedSOMSemanticBox.cs
- indexingfiltermarshaler.cs
- SourceFilter.cs
- TextTrailingCharacterEllipsis.cs
- ProtocolsConfigurationEntry.cs
- PageTheme.cs
- DeclarativeCatalogPart.cs
- ChoiceConverter.cs
- SystemIPGlobalProperties.cs