Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / ExpressionContext.cs / 1 / ExpressionContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.ComponentModel.Design.Serialization; using System.Diagnostics; ////// /// An expression context is an object that is placed on the context stack and contains /// the most relevant expression during serialization. For example, take the following /// statement: /// /// button1.Text = "Hello"; /// /// During serialization several serializers will be responsible for creating this single /// statement. One of those serializers will be responsible for writing "Hello". There /// are times when that serializer may need to know the context in which it is creating /// its expression. In the above example, this isn't needed, but take this slightly /// modified example: /// /// button1.Text = rm.GetString("button1_Text"); /// /// Here, the serializer responsible for writing the resource expression needs to /// know the names of the target objects. The ExpressionContext class can be used /// for this. As each serializer creates an expression and invokes a serializer /// to handle a smaller part of the statement as a whole, the serializer pushes /// an expression context on the context stack. Each expression context has /// a parent property that locates the next expression context on the stack, which /// provides a way for easy traversal. /// public sealed class ExpressionContext { private CodeExpression _expression; private Type _expressionType; private object _owner; private object _presetValue; ////// /// Creates a new expression context. /// public ExpressionContext(CodeExpression expression, Type expressionType, object owner, object presetValue) { // To make this public, we cannot have random special cases for what the args mean. Debug.Assert(expression != null && expressionType != null && owner != null, "Obsolete use of expression context."); if (expression == null) throw new ArgumentNullException("expression"); if (expressionType == null) throw new ArgumentNullException("expressionType"); if (owner == null) throw new ArgumentNullException("owner"); _expression = expression; _expressionType = expressionType; _owner = owner; _presetValue = presetValue; } ////// /// Creates a new expression context. /// public ExpressionContext(CodeExpression expression, Type expressionType, object owner) : this(expression, expressionType, owner, null) { } ////// /// The expression this context represents. /// public CodeExpression Expression { get { return _expression; } } ////// /// The type of the expression. This can be used to determine if a cast is needed when assigning /// to the expression. /// public Type ExpressionType { get { return _expressionType; } } ////// /// The object owning this expression. For example, if the expression was a property reference /// to button1's Text property, Owner would return button1. /// public object Owner { get { return _owner; } } ////// /// Contains the preset value of an expression, should one exist. For example, if the /// expression is a property reference expression referring to the Controls property of /// a button, PresetValue will contain the instance of Controls property because the property /// is read-only and preset by the object to contain a value. On the other hand, a property /// such as Text or Visible does not have a preset value and therefore the PresetValue property /// will return null. /// /// Serializers can use this information to guide serialization. For example, take the /// following two snippts of code: /// /// Padding p = new Padding(); /// p.Left = 5; /// button1.Padding = p; /// /// button1.Padding.Left = 5; /// /// The serializer of the Padding class needs to know if it should generate the /// first or second form. The first form would be generated by default. The /// second form will only be generated if there is an ExpressionContext on the /// stack that contains a PresetValue equal to the value of the Padding object /// currently being serialized. /// public object PresetValue { get { return _presetValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
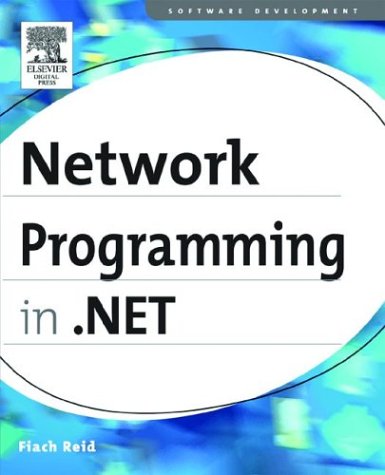
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionPageStatePersister.cs
- TableColumn.cs
- EdmScalarPropertyAttribute.cs
- PeerReferralPolicy.cs
- TreeViewAutomationPeer.cs
- codemethodreferenceexpression.cs
- StrokeNode.cs
- FilteredAttributeCollection.cs
- BindingValueChangedEventArgs.cs
- SelectorItemAutomationPeer.cs
- BufferModeSettings.cs
- RegexStringValidatorAttribute.cs
- ExpressionEditorAttribute.cs
- DataGridViewSelectedRowCollection.cs
- NotImplementedException.cs
- InputGestureCollection.cs
- Pair.cs
- SerializationHelper.cs
- AttachedPropertyDescriptor.cs
- HttpProxyCredentialType.cs
- RestHandlerFactory.cs
- AlgoModule.cs
- SectionVisual.cs
- SqlDataSourceView.cs
- SecuritySessionSecurityTokenProvider.cs
- DependencyPropertyChangedEventArgs.cs
- CacheOutputQuery.cs
- InteropBitmapSource.cs
- IIS7UserPrincipal.cs
- AuthenticatedStream.cs
- SiteMap.cs
- Control.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- DataGridViewDataConnection.cs
- RegexWriter.cs
- ColorConvertedBitmapExtension.cs
- PositiveTimeSpanValidator.cs
- DataBoundControlHelper.cs
- SessionState.cs
- Error.cs
- FileDialogCustomPlacesCollection.cs
- SerializerDescriptor.cs
- SafeFileMapViewHandle.cs
- MethodImplAttribute.cs
- sqlmetadatafactory.cs
- TabControlCancelEvent.cs
- ToolStripDropDownClosingEventArgs.cs
- HttpRequestCacheValidator.cs
- ByteStack.cs
- ListChunk.cs
- BrowserCapabilitiesFactoryBase.cs
- SqlCommandBuilder.cs
- ReadWriteControlDesigner.cs
- TabItemAutomationPeer.cs
- XmlChildNodes.cs
- ContentOperations.cs
- HttpResponseInternalWrapper.cs
- NetCodeGroup.cs
- WebBrowsableAttribute.cs
- securestring.cs
- GridViewUpdateEventArgs.cs
- ToolStripMenuItemDesigner.cs
- RawStylusInput.cs
- ScriptReference.cs
- ExtensionWindowHeader.cs
- LayoutEditorPart.cs
- WorkflowDesignerMessageFilter.cs
- ReplyChannel.cs
- PriorityBinding.cs
- ScriptControlDescriptor.cs
- EtwTrackingBehavior.cs
- ValueUnavailableException.cs
- TextEditorMouse.cs
- BrowsableAttribute.cs
- PatternMatcher.cs
- ResXFileRef.cs
- X509Certificate2Collection.cs
- ScrollEventArgs.cs
- ColorComboBox.cs
- CannotUnloadAppDomainException.cs
- InstanceDataCollection.cs
- Int32CollectionValueSerializer.cs
- UserNamePasswordValidator.cs
- DataSourceGeneratorException.cs
- MetadataUtil.cs
- FormParameter.cs
- DefaultValueAttribute.cs
- TextViewDesigner.cs
- BoundColumn.cs
- ScriptingSectionGroup.cs
- TypedRowHandler.cs
- XamlFigureLengthSerializer.cs
- MenuItemStyleCollection.cs
- MethodAccessException.cs
- NamedPermissionSet.cs
- EDesignUtil.cs
- HashRepartitionEnumerator.cs
- Contracts.cs
- AccessKeyManager.cs
- EncryptedReference.cs