Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewSelectedRowCollection.cs / 1 / DataGridViewSelectedRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IListRepresents a collection of selected ///objects in the /// control. implementation ] public class DataGridViewSelectedRowCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedRowCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewRow this[int index] { get { return (DataGridViewRow) this.items[index]; } } /// /// /// internal int Add(DataGridViewRow dataGridViewRow) { return this.items.Add(dataGridViewRow); } /* Unused at this point internal void AddRange(DataGridViewRow[] dataGridViewRows) { Debug.Assert(dataGridViewRows != null); foreach(DataGridViewRow dataGridViewRow in dataGridViewRows) { this.items.Add(dataGridViewRow); } } internal void AddRowCollection(DataGridViewRowCollection dataGridViewRows) { Debug.Assert(dataGridViewRows != null); foreach(DataGridViewRow dataGridViewRow in dataGridViewRows) { this.items.Add(dataGridViewRow); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewRow dataGridViewRow) { return this.items.IndexOf(dataGridViewRow) != -1; } ///public void CopyTo(DataGridViewRow[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewRow dataGridViewRow) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IListRepresents a collection of selected ///objects in the /// control. implementation ] public class DataGridViewSelectedRowCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedRowCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewRow this[int index] { get { return (DataGridViewRow) this.items[index]; } } /// /// /// internal int Add(DataGridViewRow dataGridViewRow) { return this.items.Add(dataGridViewRow); } /* Unused at this point internal void AddRange(DataGridViewRow[] dataGridViewRows) { Debug.Assert(dataGridViewRows != null); foreach(DataGridViewRow dataGridViewRow in dataGridViewRows) { this.items.Add(dataGridViewRow); } } internal void AddRowCollection(DataGridViewRowCollection dataGridViewRows) { Debug.Assert(dataGridViewRows != null); foreach(DataGridViewRow dataGridViewRow in dataGridViewRows) { this.items.Add(dataGridViewRow); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewRow dataGridViewRow) { return this.items.IndexOf(dataGridViewRow) != -1; } ///public void CopyTo(DataGridViewRow[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewRow dataGridViewRow) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
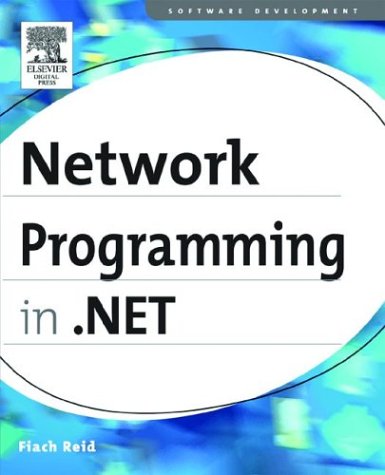
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextBoxLine.cs
- HwndSourceParameters.cs
- ClickablePoint.cs
- XPathNodePointer.cs
- MaterialCollection.cs
- Optimizer.cs
- XmlDataCollection.cs
- SamlAudienceRestrictionCondition.cs
- HttpModuleActionCollection.cs
- DetailsViewUpdateEventArgs.cs
- EditCommandColumn.cs
- StringUtil.cs
- FontStretchConverter.cs
- PropertyPathConverter.cs
- FormParameter.cs
- CompleteWizardStep.cs
- NamespaceMapping.cs
- PropertyNames.cs
- ExpressionDumper.cs
- AssemblySettingAttributes.cs
- TextChange.cs
- TagMapCollection.cs
- PerfCounters.cs
- PhysicalAddress.cs
- AdCreatedEventArgs.cs
- AndMessageFilterTable.cs
- TableProviderWrapper.cs
- StretchValidation.cs
- ScriptManager.cs
- AspCompat.cs
- UInt64Converter.cs
- FlowDecisionLabelFeature.cs
- ElementFactory.cs
- filewebrequest.cs
- MessagingActivityHelper.cs
- MulticastDelegate.cs
- WebBrowser.cs
- WebPartDisplayModeCancelEventArgs.cs
- HtmlProps.cs
- AutomationProperty.cs
- _SingleItemRequestCache.cs
- TailCallAnalyzer.cs
- ISCIIEncoding.cs
- CultureInfoConverter.cs
- ShapingWorkspace.cs
- Region.cs
- BevelBitmapEffect.cs
- Action.cs
- SizeKeyFrameCollection.cs
- HashSetDebugView.cs
- EpmContentSerializerBase.cs
- DataGridViewColumnConverter.cs
- XmlMtomWriter.cs
- ListViewUpdatedEventArgs.cs
- MemberRelationshipService.cs
- InputDevice.cs
- MustUnderstandBehavior.cs
- GeometryDrawing.cs
- MutableAssemblyCacheEntry.cs
- WebPartManagerInternals.cs
- RouteValueDictionary.cs
- FontFaceLayoutInfo.cs
- Polygon.cs
- SessionStateSection.cs
- UnauthorizedWebPart.cs
- SizeKeyFrameCollection.cs
- BinaryCommonClasses.cs
- CaseStatementSlot.cs
- UIElement3D.cs
- ComponentFactoryHelpers.cs
- SecurityHeaderLayout.cs
- ScriptControlManager.cs
- RotateTransform3D.cs
- HatchBrush.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- MenuItemStyleCollectionEditor.cs
- util.cs
- SectionInformation.cs
- SHA256.cs
- SettingsContext.cs
- CollectionContainer.cs
- SizeLimitedCache.cs
- DisplayMemberTemplateSelector.cs
- keycontainerpermission.cs
- AuthenticationException.cs
- TypeGeneratedEventArgs.cs
- ADMembershipUser.cs
- Schema.cs
- WindowsToolbarAsMenu.cs
- AuthStoreRoleProvider.cs
- TextElementAutomationPeer.cs
- TextComposition.cs
- ByteKeyFrameCollection.cs
- VarInfo.cs
- FillBehavior.cs
- TimersDescriptionAttribute.cs
- PersonalizationProviderHelper.cs
- SQlBooleanStorage.cs
- ScriptingWebServicesSectionGroup.cs
- Application.cs