Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / TableRowCollection.cs / 1 / TableRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } }
Link Menu
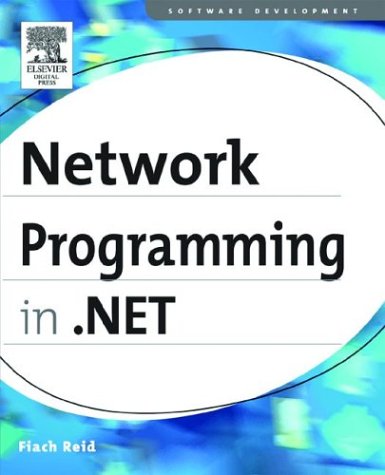
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceExpression.cs
- CodeArrayIndexerExpression.cs
- Renderer.cs
- XmlnsPrefixAttribute.cs
- StateChangeEvent.cs
- _AuthenticationState.cs
- RequestCachePolicyConverter.cs
- Opcode.cs
- StickyNoteHelper.cs
- ScriptDescriptor.cs
- MetadataUtilsSmi.cs
- Pens.cs
- ThreadInterruptedException.cs
- RootBrowserWindow.cs
- RepeatBehavior.cs
- DataAccessor.cs
- MorphHelpers.cs
- LinkButton.cs
- InputEventArgs.cs
- ElementMarkupObject.cs
- ImageSource.cs
- NamespaceDecl.cs
- MarginCollapsingState.cs
- CallInfo.cs
- XsltFunctions.cs
- PeerNode.cs
- HttpContextServiceHost.cs
- UpdateRecord.cs
- UrlPath.cs
- BinaryKeyIdentifierClause.cs
- LoginView.cs
- BufferedResponseStream.cs
- UserControl.cs
- ChannelSinkStacks.cs
- DisplayInformation.cs
- ListViewInsertEventArgs.cs
- SynchronizationFilter.cs
- BookmarkManager.cs
- MediaPlayerState.cs
- TypeToken.cs
- SR.cs
- Predicate.cs
- EntityDataSourceEntitySetNameItem.cs
- ConstraintCollection.cs
- Window.cs
- ApplicationTrust.cs
- TypeGeneratedEventArgs.cs
- SettingsAttributes.cs
- ServiceThrottlingBehavior.cs
- ToolZone.cs
- MenuItem.cs
- SqlResolver.cs
- ConditionalExpression.cs
- WrapperEqualityComparer.cs
- PrimitiveOperationFormatter.cs
- DrawingServices.cs
- unsafeIndexingFilterStream.cs
- CompilationPass2TaskInternal.cs
- ChtmlLinkAdapter.cs
- CurrentTimeZone.cs
- exports.cs
- UnescapedXmlDiagnosticData.cs
- Buffer.cs
- SemanticTag.cs
- SqlAliaser.cs
- BitmapEffectGroup.cs
- StandardToolWindows.cs
- StreamReader.cs
- ScrollItemPattern.cs
- CodeSubDirectory.cs
- Transform3DGroup.cs
- TokenFactoryFactory.cs
- Bold.cs
- ButtonChrome.cs
- EntityCommandDefinition.cs
- OutOfMemoryException.cs
- CqlGenerator.cs
- FontCacheUtil.cs
- assertwrapper.cs
- DiscoveryMessageSequenceGenerator.cs
- ServiceOperation.cs
- GenericTypeParameterBuilder.cs
- Size.cs
- IncomingWebResponseContext.cs
- EmptyEnumerator.cs
- DataExchangeServiceBinder.cs
- KerberosRequestorSecurityToken.cs
- WebControlParameterProxy.cs
- CompilerTypeWithParams.cs
- OdbcPermission.cs
- PieceDirectory.cs
- IOThreadTimer.cs
- TextSearch.cs
- Bits.cs
- CanonicalFontFamilyReference.cs
- ToolboxSnapDragDropEventArgs.cs
- InteropAutomationProvider.cs
- PngBitmapDecoder.cs
- SqlPersonalizationProvider.cs
- NotEqual.cs