Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / UpdateRecord.cs / 1 / UpdateRecord.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: UpdateRecord.cs // // Description: Contains information about current state of upate process // in the current container paragraph. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Contains information about current state of upate process in the // current container paragraph. // --------------------------------------------------------------------- internal sealed class UpdateRecord { // ------------------------------------------------------------------ // Constructor // ----------------------------------------------------------------- internal UpdateRecord() { Dtr = new DirtyTextRange(0,0,0); FirstPara = SyncPara = null; ChangeType = PTS.FSKCHANGE.fskchNone; Next = null; InProcessing = false; } // ------------------------------------------------------------------ // Merge with next update record. // ------------------------------------------------------------------ internal void MergeWithNext() { Debug.Assert(Next != null); // This is the last UR, cannot merge with next. // Merge DTRs int delta = Next.Dtr.StartIndex - Dtr.StartIndex; // Dtr.StartIndex is not changing Dtr.PositionsAdded += delta + Next.Dtr.PositionsAdded; Dtr.PositionsRemoved += delta + Next.Dtr.PositionsRemoved; // Reasign [....] point and next UpdateRecord SyncPara = Next.SyncPara; Next = Next.Next; } // ----------------------------------------------------------------- // Dirty text range. // ------------------------------------------------------------------ internal DirtyTextRange Dtr; // ----------------------------------------------------------------- // The first paragraph affected by the change. // ----------------------------------------------------------------- internal BaseParagraph FirstPara; // ----------------------------------------------------------------- // The first paragraph not affected by DTR, synchronization point for // update process. // ------------------------------------------------------------------ internal BaseParagraph SyncPara; // ----------------------------------------------------------------- // Type of the change (none, new, inside). // ------------------------------------------------------------------ internal PTS.FSKCHANGE ChangeType; // ------------------------------------------------------------------ // Next UpdateRecord. // ----------------------------------------------------------------- internal UpdateRecord Next; // ------------------------------------------------------------------ // Update record is in processing mode? // ----------------------------------------------------------------- internal bool InProcessing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: UpdateRecord.cs // // Description: Contains information about current state of upate process // in the current container paragraph. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Contains information about current state of upate process in the // current container paragraph. // --------------------------------------------------------------------- internal sealed class UpdateRecord { // ------------------------------------------------------------------ // Constructor // ----------------------------------------------------------------- internal UpdateRecord() { Dtr = new DirtyTextRange(0,0,0); FirstPara = SyncPara = null; ChangeType = PTS.FSKCHANGE.fskchNone; Next = null; InProcessing = false; } // ------------------------------------------------------------------ // Merge with next update record. // ------------------------------------------------------------------ internal void MergeWithNext() { Debug.Assert(Next != null); // This is the last UR, cannot merge with next. // Merge DTRs int delta = Next.Dtr.StartIndex - Dtr.StartIndex; // Dtr.StartIndex is not changing Dtr.PositionsAdded += delta + Next.Dtr.PositionsAdded; Dtr.PositionsRemoved += delta + Next.Dtr.PositionsRemoved; // Reasign [....] point and next UpdateRecord SyncPara = Next.SyncPara; Next = Next.Next; } // ----------------------------------------------------------------- // Dirty text range. // ------------------------------------------------------------------ internal DirtyTextRange Dtr; // ----------------------------------------------------------------- // The first paragraph affected by the change. // ----------------------------------------------------------------- internal BaseParagraph FirstPara; // ----------------------------------------------------------------- // The first paragraph not affected by DTR, synchronization point for // update process. // ------------------------------------------------------------------ internal BaseParagraph SyncPara; // ----------------------------------------------------------------- // Type of the change (none, new, inside). // ------------------------------------------------------------------ internal PTS.FSKCHANGE ChangeType; // ------------------------------------------------------------------ // Next UpdateRecord. // ----------------------------------------------------------------- internal UpdateRecord Next; // ------------------------------------------------------------------ // Update record is in processing mode? // ----------------------------------------------------------------- internal bool InProcessing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
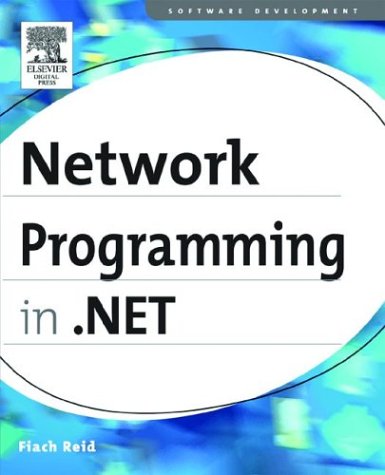
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlILAnnotation.cs
- Command.cs
- XamlPathDataSerializer.cs
- SessionParameter.cs
- CqlLexer.cs
- XmlDigitalSignatureProcessor.cs
- Console.cs
- Guid.cs
- ResXResourceWriter.cs
- embossbitmapeffect.cs
- MulticastDelegate.cs
- WebPartEditorApplyVerb.cs
- MediaEntryAttribute.cs
- COM2PictureConverter.cs
- GrammarBuilderRuleRef.cs
- FileSecurity.cs
- StylusTouchDevice.cs
- ViewValidator.cs
- Base64Encoder.cs
- OracleDataAdapter.cs
- SoapWriter.cs
- DataPagerFieldItem.cs
- PropertyDescriptorCollection.cs
- CodeLinePragma.cs
- PolyBezierSegmentFigureLogic.cs
- ToolStripProgressBar.cs
- AttachedPropertiesService.cs
- ReliableInputConnection.cs
- TextTreeTextNode.cs
- DefaultWorkflowTransactionService.cs
- ActiveDocumentEvent.cs
- AvTraceDetails.cs
- DbParameterCollection.cs
- GridViewRow.cs
- WinEventTracker.cs
- InputMethod.cs
- WebPartEditVerb.cs
- BadImageFormatException.cs
- TimeSpanValidator.cs
- PagePropertiesChangingEventArgs.cs
- UserThread.cs
- HttpRequestTraceRecord.cs
- StandardTransformFactory.cs
- SettingsPropertyNotFoundException.cs
- DataGridViewDataConnection.cs
- ListViewGroupConverter.cs
- ToolStripGripRenderEventArgs.cs
- shaper.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- BooleanFacetDescriptionElement.cs
- Int32Animation.cs
- _LoggingObject.cs
- DataGridItemCollection.cs
- CommentEmitter.cs
- InputMethodStateChangeEventArgs.cs
- DataGridViewBand.cs
- ParseElement.cs
- Metadata.cs
- XPathDescendantIterator.cs
- PtsCache.cs
- ListBindingHelper.cs
- UserNameSecurityTokenProvider.cs
- LinkDescriptor.cs
- Point3DConverter.cs
- PropertyNames.cs
- XmlJsonWriter.cs
- AmbientValueAttribute.cs
- ListItemCollection.cs
- DataGridView.cs
- DrawListViewColumnHeaderEventArgs.cs
- MappingMetadataHelper.cs
- IsolatedStorageFileStream.cs
- TextEditorCharacters.cs
- SafeFreeMibTable.cs
- HitTestResult.cs
- LineServices.cs
- GetWinFXPath.cs
- BmpBitmapDecoder.cs
- LineInfo.cs
- CompositeDesignerAccessibleObject.cs
- Padding.cs
- Int32Storage.cs
- AssertUtility.cs
- CorrelationTokenTypeConvertor.cs
- MetadataPropertyvalue.cs
- ResourceSetExpression.cs
- GeneralTransform2DTo3DTo2D.cs
- TileModeValidation.cs
- FixedDocumentSequencePaginator.cs
- DocumentOrderQuery.cs
- StylusPointProperty.cs
- EntityContainerRelationshipSetEnd.cs
- CultureInfoConverter.cs
- HttpListenerContext.cs
- BitmapScalingModeValidation.cs
- LambdaExpression.cs
- Transactions.cs
- XamlParser.cs
- CheckableControlBaseAdapter.cs
- Storyboard.cs