Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / ResourceSetExpression.cs / 1305376 / ResourceSetExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a resource set in resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; #endregion Namespaces. ///ResourceSet Expression [DebuggerDisplay("ResourceSetExpression {Source}.{MemberExpression}")] internal class ResourceSetExpression : ResourceExpression { #region Private fields. ////// The (static) type of the resources in this resource set. /// The resource type can differ from this.Type if this expression represents a transparent scope. /// For example, in TransparentScope{Category, Product}, the true element type is Product. /// private readonly Type resourceType; ///property member name private readonly Expression member; ///key predicate private DictionarykeyFilter; /// sequence query options private ListsequenceQueryOptions; /// enclosing transparent scope private TransparentAccessors transparentScope; #endregion Private fields. ////// Creates a ResourceSet expression /// /// the return type of the expression /// the source expression /// property member name /// the element type of the resource set /// expand paths for resource set /// count query option for the resource set /// custom query options for resourcse set internal ResourceSetExpression(Type type, Expression source, Expression memberExpression, Type resourceType, ListexpandPaths, CountOption countOption, Dictionary customQueryOptions, ProjectionQueryOptionExpression projection) : base(source, source != null ? (ExpressionType)ResourceExpressionType.ResourceNavigationProperty : (ExpressionType)ResourceExpressionType.RootResourceSet, type, expandPaths, countOption, customQueryOptions, projection) { Debug.Assert(type != null, "type != null"); Debug.Assert(memberExpression != null, "memberExpression != null"); Debug.Assert(resourceType != null, "resourceType != null"); Debug.Assert( (source == null && memberExpression is ConstantExpression) || (source != null && memberExpression is MemberExpression), "source is null with constant entity set name, or not null with member expression"); this.member = memberExpression; this.resourceType = resourceType; this.sequenceQueryOptions = new List (); } #region Internal properties. /// /// Member for ResourceSet /// internal Expression MemberExpression { get { return this.member; } } ////// Type of resources contained in this ResourceSet - it's element type. /// internal override Type ResourceType { get { return this.resourceType; } } ////// Is this ResourceSet enclosed in an anonymously-typed transparent scope produced by a SelectMany operation? /// Applies to navigation ResourceSets. /// internal bool HasTransparentScope { get { return this.transparentScope != null; } } ////// The property accesses required to reference this ResourceSet and its source ResourceSet if a transparent scope is present. /// May be null. Use internal TransparentAccessors TransparentScope { get { return this.transparentScope; } set { this.transparentScope = value; } } ///to test for the presence of a value. /// /// Has a key predicate restriction been applied to this ResourceSet? /// internal bool HasKeyPredicate { get { return this.keyFilter != null; } } ////// The property name/required value pairs that comprise the key predicate (if any) applied to this ResourceSet. /// May be null. Use internal Dictionaryto test for the presence of a value. /// KeyPredicate { get { return this.keyFilter; } set { this.keyFilter = value; } } /// /// A resource set produces at most 1 result if constrained by a key predicate /// internal override bool IsSingleton { get { return this.HasKeyPredicate; } } ////// Have sequence query options (filter, orderby, skip, take), expand paths, projection /// or custom query options been applied to this resource set? /// internal override bool HasQueryOptions { get { return this.sequenceQueryOptions.Count > 0 || this.ExpandPaths.Count > 0 || this.CountOption == CountOption.InlineAll || // value only count is not an option this.CustomQueryOptions.Count > 0 || this.Projection != null; } } ////// Filter query option for ResourceSet /// internal FilterQueryOptionExpression Filter { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// OrderBy query option for ResourceSet /// internal OrderByQueryOptionExpression OrderBy { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Skip query option for ResourceSet /// internal SkipQueryOptionExpression Skip { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Take query option for ResourceSet /// internal TakeQueryOptionExpression Take { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Gets sequence query options for ResourcSet /// internal IEnumerableSequenceQueryOptions { get { return this.sequenceQueryOptions.ToList(); } } /// Whether there are any query options for the sequence. internal bool HasSequenceQueryOptions { get { return this.sequenceQueryOptions.Count > 0; } } #endregion Internal properties. #region Internal methods. ////// Cast ResourceSetExpression to new type /// internal override ResourceExpression CreateCloneWithNewType(Type type) { ResourceSetExpression rse = new ResourceSetExpression( type, this.source, this.MemberExpression, TypeSystem.GetElementType(type), this.ExpandPaths.ToList(), this.CountOption, this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value), this.Projection); rse.keyFilter = this.keyFilter; rse.sequenceQueryOptions = this.sequenceQueryOptions; rse.transparentScope = this.transparentScope; return rse; } ////// Add query option to resource expression /// internal void AddSequenceQueryOption(QueryOptionExpression qoe) { Debug.Assert(qoe != null, "qoe != null"); QueryOptionExpression old = this.sequenceQueryOptions.Where(o => o.GetType() == qoe.GetType()).FirstOrDefault(); if (old != null) { qoe = qoe.ComposeMultipleSpecification(old); this.sequenceQueryOptions.Remove(old); } this.sequenceQueryOptions.Add(qoe); } ////// Instructs this resource set expression to use the input reference expression from /// The resource set expression from which to take the input reference. ///as it's /// own input reference, and to retarget the input reference from to this resource set expression. /// Used exclusively by internal void OverrideInputReference(ResourceSetExpression newInput) { Debug.Assert(newInput != null, "Original resource set cannot be null"); Debug.Assert(this.inputRef == null, "OverrideInputReference cannot be called if the target has already been referenced"); InputReferenceExpression inputRef = newInput.inputRef; if (inputRef != null) { this.inputRef = inputRef; inputRef.OverrideTarget(this); } } #endregion Internal methods. ///. /// Represents the property accesses required to access both /// this resource set and its source resource/set (for navigations). /// /// These accesses are required to reference resource sets enclosed /// in transparent scopes introduced by use of SelectMany. /// ////// For example, this query: /// from c in Custs where c.id == 1 /// from o in c.Orders from od in o.OrderDetails select od /// /// Translates to: /// c.Where(c => c.id == 1) /// .SelectMany(c => o, (c, o) => new $(c=c, o=o)) /// .SelectMany($ => $.o, ($, od) => od) /// /// PatternRules.MatchPropertyProjectionSet identifies Orders as the target of the collector. /// PatternRules.MatchTransparentScopeSelector identifies the introduction of a transparent identifer. /// /// A transparent accessor is associated with Orders, with 'c' being the source accesor, /// and 'o' being the (introduced) accessor. /// [DebuggerDisplay("{ToString()}")] internal class TransparentAccessors { #region Internal fields. ////// The property reference that must be applied to reference this resource set /// internal readonly string Accessor; ////// The property reference that must be applied to reference the source resource set. /// Note that this set's Accessor is NOT required to access the source set, but the /// source set MAY impose it's own Transparent Accessors /// internal readonly DictionarySourceAccessors; #endregion Internal fields. /// /// Constructs a new transparent scope with the specified set and source set accessors /// /// The name of the property required to access the resource set /// The names of the property required to access the resource set's sources. internal TransparentAccessors(string acc, DictionarysourceAccesors) { Debug.Assert(!string.IsNullOrEmpty(acc), "Set accessor cannot be null or empty"); Debug.Assert(sourceAccesors != null, "sourceAccesors != null"); this.Accessor = acc; this.SourceAccessors = sourceAccesors; } /// Provides a string representation of this accessor. ///The text represntation of this accessor. public override string ToString() { string result = "SourceAccessors=[" + string.Join(",", this.SourceAccessors.Keys.ToArray()); result += "] ->* Accessor=" + this.Accessor; return result; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
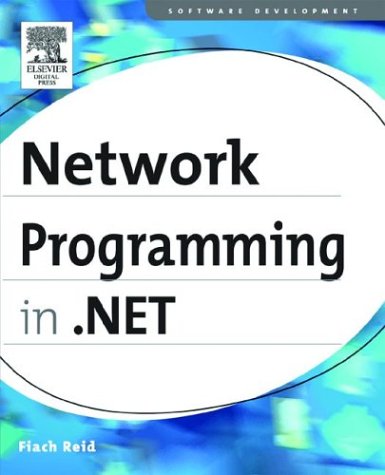
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SdlChannelSink.cs
- Crypto.cs
- XmlDataImplementation.cs
- PackagingUtilities.cs
- SiteMapNodeItem.cs
- TagPrefixAttribute.cs
- SettingsProviderCollection.cs
- NameHandler.cs
- PlatformCulture.cs
- CommandEventArgs.cs
- DiscoveryMessageSequenceGenerator.cs
- CompoundFileIOPermission.cs
- DesignerProperties.cs
- CreateUserWizardStep.cs
- AssemblyAttributes.cs
- EncryptedPackage.cs
- unsafenativemethodstextservices.cs
- TraceInternal.cs
- CTreeGenerator.cs
- RoleManagerSection.cs
- SoapTypeAttribute.cs
- BindingNavigator.cs
- BrowserCapabilitiesFactory.cs
- BuildProviderAppliesToAttribute.cs
- ToolStripDropTargetManager.cs
- PrimitiveXmlSerializers.cs
- HttpPostedFile.cs
- ColumnHeaderConverter.cs
- RayHitTestParameters.cs
- ProtocolElement.cs
- XmlName.cs
- ContentWrapperAttribute.cs
- BlurBitmapEffect.cs
- StateDesigner.Layouts.cs
- ObjectCacheHost.cs
- XmlArrayAttribute.cs
- LassoSelectionBehavior.cs
- DiscoveryUtility.cs
- Header.cs
- XmlRootAttribute.cs
- SystemPens.cs
- RuleProcessor.cs
- InteropBitmapSource.cs
- DataKeyCollection.cs
- DataKeyCollection.cs
- WindowsStreamSecurityBindingElement.cs
- latinshape.cs
- TypeValidationEventArgs.cs
- WebPartDisplayModeEventArgs.cs
- OleDbSchemaGuid.cs
- UiaCoreTypesApi.cs
- FigureParaClient.cs
- StrongNameIdentityPermission.cs
- PartialList.cs
- HttpRequestCacheValidator.cs
- MDIClient.cs
- WindowsContainer.cs
- EntityProxyTypeInfo.cs
- PEFileEvidenceFactory.cs
- QilParameter.cs
- InstancePersistence.cs
- WebPartRestoreVerb.cs
- MenuItemStyle.cs
- ProfileProvider.cs
- HttpGetClientProtocol.cs
- View.cs
- Point3DValueSerializer.cs
- Range.cs
- MenuItemStyleCollectionEditor.cs
- ClientSettings.cs
- SiteMapNodeItemEventArgs.cs
- PaginationProgressEventArgs.cs
- TypeForwardedToAttribute.cs
- NavigationHelper.cs
- X509CertificateStore.cs
- CompressEmulationStream.cs
- WindowsListViewItemCheckBox.cs
- HtmlInputControl.cs
- BuilderPropertyEntry.cs
- DataGridViewDesigner.cs
- NamedObjectList.cs
- MessageVersion.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- ConfigurationFileMap.cs
- XmlDataSourceView.cs
- EventSource.cs
- MarkupProperty.cs
- XmlIncludeAttribute.cs
- HttpClientProtocol.cs
- WebGetAttribute.cs
- StylusPlugInCollection.cs
- SqlBuffer.cs
- CookieProtection.cs
- OdbcStatementHandle.cs
- ContentHostHelper.cs
- CodePrimitiveExpression.cs
- Int64AnimationBase.cs
- CommonDialog.cs
- SecurityException.cs
- CodeMethodReturnStatement.cs