Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / documents / ContentHostHelper.cs / 1 / ContentHostHelper.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentHostHelper.cs // // Description: The ContentHostHelper class contains static methods that are // useful for performing common tasks with IContentHost. // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // Listusing System.Windows; // IContentHost using System.Windows.Controls; // TextBlock using System.Windows.Controls.Primitives; // DocumentPageView using System.Windows.Documents; // FlowDocument using System.Windows.Media; // Visual using MS.Internal.PtsHost; // FlowDocumentPage namespace MS.Internal.Documents { /// /// Static helper functions for dealing with IContentHost. /// internal static class ContentHostHelper { //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Given a ContentElement searches for associated IContentHost, if one exists. /// /// Content element ///Associated IContentHost with ContentElement. internal static IContentHost FindContentHost(ContentElement contentElement) { IContentHost ich = null; DependencyObject parent; TextContainer textContainer; if (contentElement == null) { return null; } // If the ContentElement is a TextElement, retrieve IContentHost form the owner // of TextContainer. if (contentElement is TextElement) { textContainer = ((TextElement)contentElement).TextContainer; parent = textContainer.Parent; if (parent is IContentHost) // TextBlock { ich = (IContentHost)parent; } else if (parent is FlowDocument) // Viewers { ich = GetICHFromFlowDocument((TextElement)contentElement, (FlowDocument)parent); } else if (textContainer.TextView != null && textContainer.TextView.RenderScope is IContentHost) { // TextBlock hosted in ControlTemplate ich = (IContentHost)textContainer.TextView.RenderScope; } } // else; cannot retrive IContentHost return ich; } #endregion Internal Methods //-------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Given a ContentElement within FlowDocument searches for associated IContentHost. /// /// Content element /// FlowDocument hosting ContentElement. ///Associated IContentHost with ContentElement. private static IContentHost GetICHFromFlowDocument(TextElement contentElement, FlowDocument flowDocument) { IContentHost ich = null; ListpageViews; ITextView textView = flowDocument.StructuralCache.TextContainer.TextView; if (textView != null) { // If FlowDocument is hosted by FlowDocumentScrollViewer, the RenderScope // is FlowDocumentView object which hosts PageVisual representing the content. // This PageVisual is also IContentHost for the entire content of DocumentPage. if (textView.RenderScope is FlowDocumentView) // FlowDocumentScrollViewer { if (VisualTreeHelper.GetChildrenCount(textView.RenderScope) > 0) { ich = VisualTreeHelper.GetChild(textView.RenderScope, 0) as IContentHost; } } // Our best guess is that FlowDocument is hosted by DocumentViewerBase. // In this case search the style for all DocumentPageViews. // Having collection of DocumentPageViews, find for the one which hosts TextElement. else if (textView.RenderScope is FrameworkElement) { pageViews = new List (); FindDocumentPageViews(textView.RenderScope, pageViews); for (int i = 0; i < pageViews.Count; i++) { if (pageViews[i].DocumentPage is FlowDocumentPage) { textView = (ITextView)((IServiceProvider)pageViews[i].DocumentPage).GetService(typeof(ITextView)); if (textView != null && textView.IsValid) { // Check if the page contains ContentElement. Check Start and End // position, which will give desired results in most of the cases. // Having hyperlink spanning more than 2 pages is not very common, // and this code will not work with it correctly. if (textView.Contains(contentElement.ContentStart) || textView.Contains(contentElement.ContentEnd)) { ich = pageViews[i].DocumentPage.Visual as IContentHost; } } } } } } return ich; } /// /// Does deep Visual tree walk to retrieve all DocumentPageViews. /// It stops recursing down into visual tree in following situations: /// a) Visual is UIElement and it is not part of Contol Template, /// b) Visual is DocumentPageView. /// /// FrameworkElement that is part of Control Template. /// Collection of DocumentPageViews; found elements are appended here. ///Whether collection of DocumentPageViews has been updated. private static void FindDocumentPageViews(Visual root, ListpageViews) { Invariant.Assert(root != null); Invariant.Assert(pageViews != null); if (root is DocumentPageView) { pageViews.Add((DocumentPageView)root); } else { FrameworkElement fe; // Do deep tree walk to retrieve all DocumentPageViews. // It stops recursing down into visual tree in following situations: // a) Visual is UIElement and it is not part of Contol Template, // b) Visual is DocumentPageView. // Add to collection any DocumentPageViews found in the Control Template. int count = root.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual child = root.InternalGetVisualChild(i); fe = child as FrameworkElement; if (fe != null) { if (fe.TemplatedParent != null) { if (fe is DocumentPageView) { pageViews.Add(fe as DocumentPageView); } else { FindDocumentPageViews(fe, pageViews); } } } else { FindDocumentPageViews(child, pageViews); } } } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ContentHostHelper.cs // // Description: The ContentHostHelper class contains static methods that are // useful for performing common tasks with IContentHost. // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // List using System.Windows; // IContentHost using System.Windows.Controls; // TextBlock using System.Windows.Controls.Primitives; // DocumentPageView using System.Windows.Documents; // FlowDocument using System.Windows.Media; // Visual using MS.Internal.PtsHost; // FlowDocumentPage namespace MS.Internal.Documents { /// /// Static helper functions for dealing with IContentHost. /// internal static class ContentHostHelper { //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Given a ContentElement searches for associated IContentHost, if one exists. /// /// Content element ///Associated IContentHost with ContentElement. internal static IContentHost FindContentHost(ContentElement contentElement) { IContentHost ich = null; DependencyObject parent; TextContainer textContainer; if (contentElement == null) { return null; } // If the ContentElement is a TextElement, retrieve IContentHost form the owner // of TextContainer. if (contentElement is TextElement) { textContainer = ((TextElement)contentElement).TextContainer; parent = textContainer.Parent; if (parent is IContentHost) // TextBlock { ich = (IContentHost)parent; } else if (parent is FlowDocument) // Viewers { ich = GetICHFromFlowDocument((TextElement)contentElement, (FlowDocument)parent); } else if (textContainer.TextView != null && textContainer.TextView.RenderScope is IContentHost) { // TextBlock hosted in ControlTemplate ich = (IContentHost)textContainer.TextView.RenderScope; } } // else; cannot retrive IContentHost return ich; } #endregion Internal Methods //-------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Given a ContentElement within FlowDocument searches for associated IContentHost. /// /// Content element /// FlowDocument hosting ContentElement. ///Associated IContentHost with ContentElement. private static IContentHost GetICHFromFlowDocument(TextElement contentElement, FlowDocument flowDocument) { IContentHost ich = null; ListpageViews; ITextView textView = flowDocument.StructuralCache.TextContainer.TextView; if (textView != null) { // If FlowDocument is hosted by FlowDocumentScrollViewer, the RenderScope // is FlowDocumentView object which hosts PageVisual representing the content. // This PageVisual is also IContentHost for the entire content of DocumentPage. if (textView.RenderScope is FlowDocumentView) // FlowDocumentScrollViewer { if (VisualTreeHelper.GetChildrenCount(textView.RenderScope) > 0) { ich = VisualTreeHelper.GetChild(textView.RenderScope, 0) as IContentHost; } } // Our best guess is that FlowDocument is hosted by DocumentViewerBase. // In this case search the style for all DocumentPageViews. // Having collection of DocumentPageViews, find for the one which hosts TextElement. else if (textView.RenderScope is FrameworkElement) { pageViews = new List (); FindDocumentPageViews(textView.RenderScope, pageViews); for (int i = 0; i < pageViews.Count; i++) { if (pageViews[i].DocumentPage is FlowDocumentPage) { textView = (ITextView)((IServiceProvider)pageViews[i].DocumentPage).GetService(typeof(ITextView)); if (textView != null && textView.IsValid) { // Check if the page contains ContentElement. Check Start and End // position, which will give desired results in most of the cases. // Having hyperlink spanning more than 2 pages is not very common, // and this code will not work with it correctly. if (textView.Contains(contentElement.ContentStart) || textView.Contains(contentElement.ContentEnd)) { ich = pageViews[i].DocumentPage.Visual as IContentHost; } } } } } } return ich; } /// /// Does deep Visual tree walk to retrieve all DocumentPageViews. /// It stops recursing down into visual tree in following situations: /// a) Visual is UIElement and it is not part of Contol Template, /// b) Visual is DocumentPageView. /// /// FrameworkElement that is part of Control Template. /// Collection of DocumentPageViews; found elements are appended here. ///Whether collection of DocumentPageViews has been updated. private static void FindDocumentPageViews(Visual root, ListpageViews) { Invariant.Assert(root != null); Invariant.Assert(pageViews != null); if (root is DocumentPageView) { pageViews.Add((DocumentPageView)root); } else { FrameworkElement fe; // Do deep tree walk to retrieve all DocumentPageViews. // It stops recursing down into visual tree in following situations: // a) Visual is UIElement and it is not part of Contol Template, // b) Visual is DocumentPageView. // Add to collection any DocumentPageViews found in the Control Template. int count = root.InternalVisualChildrenCount; for (int i = 0; i < count; i++) { Visual child = root.InternalGetVisualChild(i); fe = child as FrameworkElement; if (fe != null) { if (fe.TemplatedParent != null) { if (fe is DocumentPageView) { pageViews.Add(fe as DocumentPageView); } else { FindDocumentPageViews(fe, pageViews); } } } else { FindDocumentPageViews(child, pageViews); } } } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
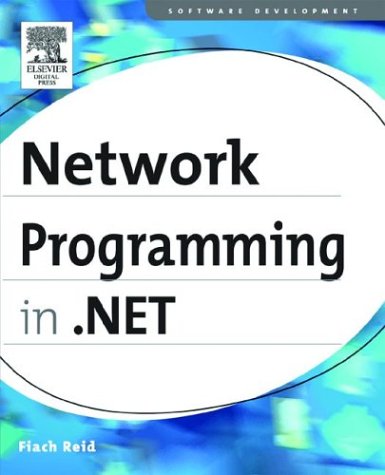
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusPointProperties.cs
- Helper.cs
- PointIndependentAnimationStorage.cs
- SiteMapSection.cs
- IdentifierService.cs
- NotifyCollectionChangedEventArgs.cs
- _ShellExpression.cs
- OdbcConnectionHandle.cs
- SoapMessage.cs
- PointConverter.cs
- RoleManagerModule.cs
- FormsAuthenticationTicket.cs
- BufferedGraphicsManager.cs
- FormViewInsertEventArgs.cs
- CannotUnloadAppDomainException.cs
- X509Certificate2Collection.cs
- SafeSecurityHandles.cs
- OleDbRowUpdatedEvent.cs
- SecureUICommand.cs
- PrivilegedConfigurationManager.cs
- CodeCastExpression.cs
- XmlSchemaSimpleContentRestriction.cs
- HiddenFieldDesigner.cs
- CharUnicodeInfo.cs
- HttpCapabilitiesSectionHandler.cs
- WebBrowserSiteBase.cs
- UndoEngine.cs
- RtfControlWordInfo.cs
- Parsers.cs
- ConnectionPoint.cs
- EntityConnection.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- EntityDesignPluralizationHandler.cs
- GeneralTransform.cs
- ConvertEvent.cs
- HMAC.cs
- MultiBindingExpression.cs
- SoapEnumAttribute.cs
- TiffBitmapDecoder.cs
- ContextCorrelationInitializer.cs
- SHA256CryptoServiceProvider.cs
- ObjectAnimationBase.cs
- PropertyKey.cs
- StrongNameKeyPair.cs
- VarRemapper.cs
- ObfuscationAttribute.cs
- sqlstateclientmanager.cs
- SqlWriter.cs
- ReferenceService.cs
- ComponentConverter.cs
- WebBrowserNavigatingEventHandler.cs
- EmptyStringExpandableObjectConverter.cs
- Cursors.cs
- TextMarkerSource.cs
- CompilerHelpers.cs
- FontUnit.cs
- UpdateManifestForBrowserApplication.cs
- ConnectionStringsExpressionBuilder.cs
- InputLanguageEventArgs.cs
- DataGrid.cs
- IndependentAnimationStorage.cs
- SelectionEditor.cs
- Parallel.cs
- SmtpNetworkElement.cs
- XsltLibrary.cs
- GcSettings.cs
- ForwardPositionQuery.cs
- HttpSysSettings.cs
- XmlMtomWriter.cs
- TextEditorCharacters.cs
- RequestCacheManager.cs
- SpotLight.cs
- CompleteWizardStep.cs
- Pointer.cs
- Wildcard.cs
- MaskInputRejectedEventArgs.cs
- COAUTHINFO.cs
- BitmapCodecInfoInternal.cs
- DependencySource.cs
- PhonemeEventArgs.cs
- COM2ExtendedBrowsingHandler.cs
- FileResponseElement.cs
- LinqDataSourceInsertEventArgs.cs
- TrackingStringDictionary.cs
- WorkflowInstanceAbortedRecord.cs
- SocketInformation.cs
- PlaceHolder.cs
- VirtualPathProvider.cs
- HttpClientChannel.cs
- URLAttribute.cs
- ProcessThreadCollection.cs
- ReadOnlyPermissionSet.cs
- StreamWriter.cs
- WeakReferenceKey.cs
- CheckPair.cs
- DataGridColumnCollection.cs
- TCPListener.cs
- COAUTHIDENTITY.cs
- ResourceAssociationSetEnd.cs
- StringValidatorAttribute.cs