Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataSet / System / Data / EnumerableRowCollection.cs / 1 / EnumerableRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections; using System.Text; using System.Data; using System.Linq; using System.Diagnostics; using System.Linq.Expressions; using System.Collections.ObjectModel; using System.Data.DataSetExtensions; namespace System.Data { ////// Provides an entry point so that Cast operator call can be intercepted within an extension method. /// public abstract class EnumerableRowCollection : IEnumerable { internal abstract Type ElementType { get; } internal abstract DataTable Table { get; } internal EnumerableRowCollection() { } IEnumerator IEnumerable.GetEnumerator() { return null; } } ////// This class provides a wrapper for DataTables to allow for querying via LINQ. /// public class EnumerableRowCollection: EnumerableRowCollection, IEnumerable { private readonly DataTable _table; private readonly IEnumerable _enumerableRows; private readonly List > _listOfPredicates; // Stores list of sort expression in the order provided by user. E.g. order by, thenby, thenby descending.. private readonly SortExpressionBuilder _sortExpression; private readonly Func _selector; #region Properties internal override Type ElementType { get { return typeof(TRow); } } internal IEnumerable EnumerableRows { get { return _enumerableRows; } } internal override DataTable Table { get { return _table; } } #endregion Properties #region Constructors /// /// This constructor is used when Select operator is called with output Type other than input row Type. /// Basically fail on GetLDV(), but other LINQ operators must work. /// internal EnumerableRowCollection(IEnumerableenumerableRows, bool isDataViewable, DataTable table) { Debug.Assert(!isDataViewable || table != null, "isDataViewable bug table is null"); _enumerableRows = enumerableRows; if (isDataViewable) { _table = table; } _listOfPredicates = new List >(); _sortExpression = new SortExpressionBuilder (); } /// /// Basic Constructor /// internal EnumerableRowCollection(DataTable table) { _table = table; _enumerableRows = table.Rows.Cast(); _listOfPredicates = new List >(); _sortExpression = new SortExpressionBuilder (); } /// /// Copy Constructor that sets the input IEnumerable as enumerableRows /// Used to maintain IEnumerable that has linq operators executed in the same order as the user /// internal EnumerableRowCollection(EnumerableRowCollectionsource, IEnumerable enumerableRows, Func selector) { Debug.Assert(null != enumerableRows, "null enumerableRows"); _enumerableRows = enumerableRows; _selector = selector; if (null != source) { if (null == source._selector) { _table = source._table; } _listOfPredicates = new List >(source._listOfPredicates); _sortExpression = source._sortExpression.Clone(); //deep copy the List } else { _listOfPredicates = new List >(); _sortExpression = new SortExpressionBuilder (); } } #endregion Constructors #region PublicInterface IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// This method returns an strongly typed iterator /// for the underlying DataRow collection. /// ////// A strongly typed iterator. /// public IEnumeratorGetEnumerator() { return _enumerableRows.GetEnumerator(); } #endregion PublicInterface /// /// Evaluates filter and sort if necessary and returns /// a LinqDataView representing the LINQ query this class has collected. /// ///LinqDataView repesenting the LINQ query internal LinqDataView GetLinqDataView() //Called by AsLinqDataView { if ((null == _table) || !typeof(DataRow).IsAssignableFrom(typeof(TRow))) { throw DataSetUtil.NotSupported(Strings.ToLDVUnsupported); } LinqDataView view = null; #region BuildSinglePredicate FuncfinalPredicate = null; //Conjunction of all .Where(..) predicates if ((null != _selector) && (0 < _listOfPredicates.Count)) { // Hook up all individual predicates into one predicate // This delegate is a conjunction of multiple predicates set by the user // Note: This is a Short-Circuit Conjunction finalPredicate = delegate(DataRow row) { if (!Object.ReferenceEquals(row, _selector((TRow)(object)row))) { throw DataSetUtil.NotSupported(Strings.ToLDVUnsupported); } foreach (Func pred in _listOfPredicates) { if (!pred((TRow)(object)row)) { return false; } } return true; }; } else if (null != _selector) { finalPredicate = delegate(DataRow row) { if (!Object.ReferenceEquals(row, _selector((TRow)(object)row))) { throw DataSetUtil.NotSupported(Strings.ToLDVUnsupported); } return true; }; } else if (0 < _listOfPredicates.Count) { finalPredicate = delegate(DataRow row) { foreach (Func pred in _listOfPredicates) { if (!pred((TRow)(object)row)) { return false; } } return true; }; } #endregion BuildSinglePredicate #region Evaluate Filter/Sort // All of this mess below is because we want to create index only once. // // If we only have filter, we set _view.Predicate - 1 index creation // If we only have sort, we set _view.SortExpression() - 1 index creation // If we have BOTH, we set them through the constructor - 1 index creation // // Filter AND Sort if ((null != finalPredicate) && (0 < _sortExpression.Count)) { // A lot more work here because constructor does not know type K, // so the responsibility to create appropriate delegate comparers // is outside of the constructor. view = new LinqDataView( _table, finalPredicate, //Func() Predicate delegate(DataRow row) //System.Predicate { return finalPredicate(row); }, delegate(DataRow a, DataRow b) //Comparison for DV for Index creation { return _sortExpression.Compare( _sortExpression.Select((TRow)(object)a), _sortExpression.Select((TRow)(object)b) ); }, delegate(object key, DataRow row) //Comparison_K_T for DV's Find() { return _sortExpression.Compare( (List
Link Menu
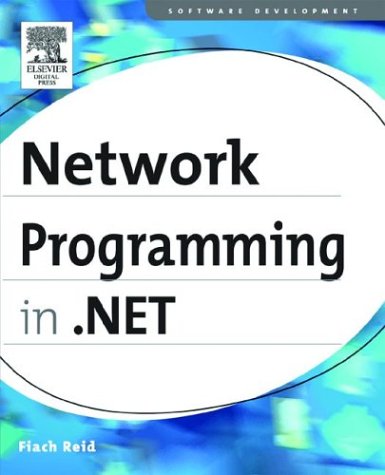
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VerificationAttribute.cs
- StorageAssociationTypeMapping.cs
- SQLCharsStorage.cs
- WebPartMenuStyle.cs
- DynamicPhysicalDiscoSearcher.cs
- LayoutSettings.cs
- DecimalAnimation.cs
- Viewport3DAutomationPeer.cs
- PathSegmentCollection.cs
- TemplatedWizardStep.cs
- MergeFailedEvent.cs
- TagNameToTypeMapper.cs
- AssemblyCache.cs
- IntranetCredentialPolicy.cs
- ArgumentNullException.cs
- OdbcDataReader.cs
- Camera.cs
- SchemaMapping.cs
- baseshape.cs
- ValuePattern.cs
- XamlRtfConverter.cs
- ListViewInsertedEventArgs.cs
- Roles.cs
- RestClientProxyHandler.cs
- ExtensionQuery.cs
- RulePatternOps.cs
- ImageDrawing.cs
- WebControlsSection.cs
- MenuItemAutomationPeer.cs
- TextTreeRootNode.cs
- SimpleParser.cs
- ExpressionEditorAttribute.cs
- TypeLoadException.cs
- odbcmetadatafactory.cs
- ProfileManager.cs
- WebEncodingValidatorAttribute.cs
- ToolStripHighContrastRenderer.cs
- Configuration.cs
- ViewSimplifier.cs
- ColorConverter.cs
- XmlLoader.cs
- DropShadowEffect.cs
- SerializationHelper.cs
- TabItemWrapperAutomationPeer.cs
- MessageTraceRecord.cs
- EventMappingSettingsCollection.cs
- TrustLevel.cs
- MimePart.cs
- MbpInfo.cs
- BitmapPalette.cs
- BaseCAMarshaler.cs
- ErrorEventArgs.cs
- PropertyValueUIItem.cs
- ManagementEventWatcher.cs
- RuleCache.cs
- ToolStripCustomTypeDescriptor.cs
- SafeHandle.cs
- RNGCryptoServiceProvider.cs
- TextRange.cs
- TextFormatter.cs
- ExtensionSurface.cs
- _SslSessionsCache.cs
- TextFormatterHost.cs
- PathBox.cs
- SchemaAttDef.cs
- BoolExpression.cs
- PermissionSet.cs
- DynamicDiscoveryDocument.cs
- VisualStyleElement.cs
- RecognizedWordUnit.cs
- AnnotationAdorner.cs
- StrongNameUtility.cs
- InvokeHandlers.cs
- PackageRelationship.cs
- DesignerDataView.cs
- Byte.cs
- ScrollBar.cs
- EventLogPropertySelector.cs
- loginstatus.cs
- EnumConverter.cs
- Size.cs
- EventLogEntry.cs
- RtfControls.cs
- StrokeIntersection.cs
- AsyncCompletedEventArgs.cs
- Column.cs
- CookieProtection.cs
- XmlSerializationReader.cs
- PasswordBox.cs
- SHA1.cs
- StrongNameIdentityPermission.cs
- RectangleHotSpot.cs
- X509CertificateTokenFactoryCredential.cs
- storepermissionattribute.cs
- TimeIntervalCollection.cs
- XmlDownloadManager.cs
- CancellationHandlerDesigner.cs
- TextTreeInsertUndoUnit.cs
- altserialization.cs
- MenuItem.cs