Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / IO / Packaging / PackageRelationship.cs / 1 / PackageRelationship.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationship. This is a part of the // MMCF Packaging Layer. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation or PackageRelationship methods // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Xml; using System.Windows; // For Exception strings - SRID using System.Text; // for StringBuilder using System.Diagnostics; // for Debug.Assert using MS.Internal; // for Invariant.Assert namespace System.IO.Packaging { ////// This class is used to express a relationship between a source and a target part. /// The only way to create a PackageRelationship, is to call the PackagePart.CreateRelationship() /// or Package.CreateRelationship(). A relationship is owned by a part or by the package itself. /// If the source part is deleted all the relationships it owns are also deleted. /// A target of the relationship need not be present. public class PackageRelationship { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// This is a reference to the parent PackagePart to which this relationship belongs. /// ///Uri public Uri SourceUri { get { if (_source == null) return PackUriHelper.PackageRootUri; else return _source.Uri; } } ////// Uri of the TargetPart, that this relationship points to. /// ///public Uri TargetUri { get { return _targetUri; } } /// /// Type of the relationship - used to uniquely define the role of the relationship /// ///public string RelationshipType { get { return _relationshipType; } } /// /// Enumeration value indicating the interpretations of the "base" of the target uri. /// ///public TargetMode TargetMode { get { return _targetMode; } } /// /// PackageRelationship's identifier. Unique across relationships for the given source. /// ///String public String Id { get { return _id; } } ////// PackageRelationship's owning Package object. /// ///Package public Package Package { get { return _package; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Internal Constructor ////// PackageRelationship constructor /// /// Owning Package object for this relationship /// owning part - will be null if the owner is the container /// target of relationship /// enum specifying the interpretation of the base uri for the target uri /// type name /// unique identifier internal PackageRelationship(Package package, PackagePart sourcePart, Uri targetUri, TargetMode targetMode, string relationshipType, string id) { //sourcePart can be null to represent that the relationships are at the package level if (package == null) throw new ArgumentNullException("package"); if (targetUri == null) throw new ArgumentNullException("targetUri"); if (relationshipType == null) throw new ArgumentNullException("relationshipType"); if (id == null) throw new ArgumentNullException("id"); // The ID is guaranteed to be an XML ID by the caller (InternalRelationshipCollection). // The following check is a precaution against future bug introductions. #if DEBUG try { // An XSD ID is an NCName that is unique. We can't check uniqueness at this level. XmlConvert.VerifyNCName(id); } catch(XmlException exception) { throw new XmlException(SR.Get(SRID.NotAValidXmlIdString, id), exception); } #endif // Additional check - don't accept absolute Uri's if targetMode is Internal. Debug.Assert((targetMode == TargetMode.External || !targetUri.IsAbsoluteUri), "PackageRelationship target must be relative if the TargetMode is Internal"); // Additional check - Verify if the Enum value is valid Debug.Assert ((targetMode >= TargetMode.Internal || targetMode <= TargetMode.External), "TargetMode enum value is out of Range"); // Look for empty string or string with just spaces Debug.Assert(relationshipType.Trim() != String.Empty, "RelationshipType cannot be empty string or a string with just spaces"); _package = package; _source = sourcePart; _targetUri = targetUri; _relationshipType = relationshipType; _targetMode = targetMode; _id = id; } #endregion Internal Constructor //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal static Uri ContainerRelationshipPartName { get { return _containerRelationshipPartName; } } // Property used in streaming production to be able to keep the set // of all properties that have been created in memory while knowing // which remain to be written to XML. internal bool Saved { get { return _saved; } set { _saved = value; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members private Package _package; private PackagePart _source; private Uri _targetUri; private string _relationshipType; private TargetMode _targetMode; private String _id; private bool _saved = false; private static readonly Uri _containerRelationshipPartName = PackUriHelper.CreatePartUri(new Uri("/_rels/.rels", UriKind.Relative)); #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationship. This is a part of the // MMCF Packaging Layer. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation or PackageRelationship methods // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Xml; using System.Windows; // For Exception strings - SRID using System.Text; // for StringBuilder using System.Diagnostics; // for Debug.Assert using MS.Internal; // for Invariant.Assert namespace System.IO.Packaging { ////// This class is used to express a relationship between a source and a target part. /// The only way to create a PackageRelationship, is to call the PackagePart.CreateRelationship() /// or Package.CreateRelationship(). A relationship is owned by a part or by the package itself. /// If the source part is deleted all the relationships it owns are also deleted. /// A target of the relationship need not be present. public class PackageRelationship { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// This is a reference to the parent PackagePart to which this relationship belongs. /// ///Uri public Uri SourceUri { get { if (_source == null) return PackUriHelper.PackageRootUri; else return _source.Uri; } } ////// Uri of the TargetPart, that this relationship points to. /// ///public Uri TargetUri { get { return _targetUri; } } /// /// Type of the relationship - used to uniquely define the role of the relationship /// ///public string RelationshipType { get { return _relationshipType; } } /// /// Enumeration value indicating the interpretations of the "base" of the target uri. /// ///public TargetMode TargetMode { get { return _targetMode; } } /// /// PackageRelationship's identifier. Unique across relationships for the given source. /// ///String public String Id { get { return _id; } } ////// PackageRelationship's owning Package object. /// ///Package public Package Package { get { return _package; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Internal Constructor ////// PackageRelationship constructor /// /// Owning Package object for this relationship /// owning part - will be null if the owner is the container /// target of relationship /// enum specifying the interpretation of the base uri for the target uri /// type name /// unique identifier internal PackageRelationship(Package package, PackagePart sourcePart, Uri targetUri, TargetMode targetMode, string relationshipType, string id) { //sourcePart can be null to represent that the relationships are at the package level if (package == null) throw new ArgumentNullException("package"); if (targetUri == null) throw new ArgumentNullException("targetUri"); if (relationshipType == null) throw new ArgumentNullException("relationshipType"); if (id == null) throw new ArgumentNullException("id"); // The ID is guaranteed to be an XML ID by the caller (InternalRelationshipCollection). // The following check is a precaution against future bug introductions. #if DEBUG try { // An XSD ID is an NCName that is unique. We can't check uniqueness at this level. XmlConvert.VerifyNCName(id); } catch(XmlException exception) { throw new XmlException(SR.Get(SRID.NotAValidXmlIdString, id), exception); } #endif // Additional check - don't accept absolute Uri's if targetMode is Internal. Debug.Assert((targetMode == TargetMode.External || !targetUri.IsAbsoluteUri), "PackageRelationship target must be relative if the TargetMode is Internal"); // Additional check - Verify if the Enum value is valid Debug.Assert ((targetMode >= TargetMode.Internal || targetMode <= TargetMode.External), "TargetMode enum value is out of Range"); // Look for empty string or string with just spaces Debug.Assert(relationshipType.Trim() != String.Empty, "RelationshipType cannot be empty string or a string with just spaces"); _package = package; _source = sourcePart; _targetUri = targetUri; _relationshipType = relationshipType; _targetMode = targetMode; _id = id; } #endregion Internal Constructor //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal static Uri ContainerRelationshipPartName { get { return _containerRelationshipPartName; } } // Property used in streaming production to be able to keep the set // of all properties that have been created in memory while knowing // which remain to be written to XML. internal bool Saved { get { return _saved; } set { _saved = value; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members private Package _package; private PackagePart _source; private Uri _targetUri; private string _relationshipType; private TargetMode _targetMode; private String _id; private bool _saved = false; private static readonly Uri _containerRelationshipPartName = PackUriHelper.CreatePartUri(new Uri("/_rels/.rels", UriKind.Relative)); #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
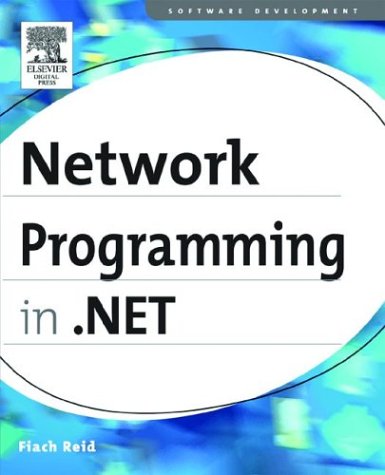
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BamlTreeUpdater.cs
- Label.cs
- Figure.cs
- OleDbError.cs
- SHA1.cs
- XmlSchemaSimpleContent.cs
- StringExpressionSet.cs
- Size.cs
- MenuItemStyleCollection.cs
- HostDesigntimeLicenseContext.cs
- XmlSchemaAny.cs
- WorkflowFileItem.cs
- StorageEndPropertyMapping.cs
- GlyphRun.cs
- COM2ExtendedTypeConverter.cs
- MimeMapping.cs
- DrawingContextWalker.cs
- ImageSourceConverter.cs
- HiddenField.cs
- BindingMemberInfo.cs
- HttpHostedTransportConfiguration.cs
- PersianCalendar.cs
- TreeWalker.cs
- FileChangesMonitor.cs
- ELinqQueryState.cs
- SystemIPInterfaceProperties.cs
- WebPartEventArgs.cs
- SqlProvider.cs
- BaseParser.cs
- PasswordBoxAutomationPeer.cs
- AsyncOperationManager.cs
- SkinBuilder.cs
- PageHandlerFactory.cs
- Interlocked.cs
- Span.cs
- MediaPlayerState.cs
- StringSorter.cs
- PropertyReferenceSerializer.cs
- TextRangeProviderWrapper.cs
- PrintDialog.cs
- OperationGenerator.cs
- EndPoint.cs
- XmlComment.cs
- ClientSession.cs
- HttpPostClientProtocol.cs
- SessionParameter.cs
- KeyTime.cs
- SqlCaseSimplifier.cs
- ResourcesGenerator.cs
- ByteArrayHelperWithString.cs
- NavigationExpr.cs
- _IPv4Address.cs
- RemotingException.cs
- DataGridViewSelectedCellCollection.cs
- XmlLanguageConverter.cs
- ContravarianceAdapter.cs
- GeneratedCodeAttribute.cs
- EntityDataSourceWrapper.cs
- ThicknessAnimation.cs
- XmlElementAttribute.cs
- AppLevelCompilationSectionCache.cs
- SqlInternalConnectionTds.cs
- ProtectedProviderSettings.cs
- IntegerValidator.cs
- WebPartCollection.cs
- ButtonFieldBase.cs
- CombinedHttpChannel.cs
- CodeBlockBuilder.cs
- RuntimeConfig.cs
- RSAOAEPKeyExchangeFormatter.cs
- StandardBindingImporter.cs
- DataGridViewLinkCell.cs
- WindowsUpDown.cs
- TextSpan.cs
- PrinterSettings.cs
- HTTPNotFoundHandler.cs
- StateRuntime.cs
- TraceLevelStore.cs
- NamespaceCollection.cs
- DataRowView.cs
- RijndaelManaged.cs
- HttpSessionStateWrapper.cs
- TargetInvocationException.cs
- ErrorEventArgs.cs
- FlowDocumentPage.cs
- EntityWithKeyStrategy.cs
- OracleConnectionFactory.cs
- IndexerNameAttribute.cs
- DesignUtil.cs
- VarRefManager.cs
- MetaTable.cs
- SiteMapHierarchicalDataSourceView.cs
- HandleExceptionArgs.cs
- ILGenerator.cs
- TextDataBindingHandler.cs
- SecurityPermission.cs
- CodeGenerator.cs
- FullTextState.cs
- PasswordRecovery.cs
- RemotingAttributes.cs