Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Markup / XmlLanguageConverter.cs / 1 / XmlLanguageConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: XmlLanguageConverter.cs // // Description: Contains the XmlLanuageConverter: TypeConverter for the CultureInfo class. // // History: // 11/21/2005 : jerryd - Initial implementation // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Markup { ////// XmlLanuageConverter - Converter class for converting instances of other types to and from XmlLanguage /// in a way that does not depend on the current user's language settings. /// public class XmlLanguageConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string ietfLanguageTag = source as string; if (ietfLanguageTag != null) { return XmlLanguage.GetLanguage(ietfLanguageTag); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The XmlLanguage to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for XmlLanguage.IetfLanguageTag, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } XmlLanguage xmlLanguage = value as XmlLanguage; if (xmlLanguage != null) { if (destinationType == typeof(string)) { return xmlLanguage.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(XmlLanguage).GetMethod( "GetLanguage", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { xmlLanguage.IetfLanguageTag }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: XmlLanguageConverter.cs // // Description: Contains the XmlLanuageConverter: TypeConverter for the CultureInfo class. // // History: // 11/21/2005 : jerryd - Initial implementation // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Markup { ////// XmlLanuageConverter - Converter class for converting instances of other types to and from XmlLanguage /// in a way that does not depend on the current user's language settings. /// public class XmlLanguageConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string ietfLanguageTag = source as string; if (ietfLanguageTag != null) { return XmlLanguage.GetLanguage(ietfLanguageTag); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The XmlLanguage to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for XmlLanguage.IetfLanguageTag, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } XmlLanguage xmlLanguage = value as XmlLanguage; if (xmlLanguage != null) { if (destinationType == typeof(string)) { return xmlLanguage.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(XmlLanguage).GetMethod( "GetLanguage", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { xmlLanguage.IetfLanguageTag }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
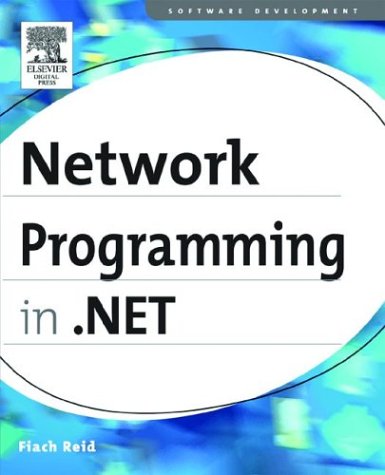
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameSpaceExtractor.cs
- BasicHttpMessageCredentialType.cs
- DataServiceRequestArgs.cs
- basenumberconverter.cs
- OperatingSystem.cs
- WithStatement.cs
- DateTimeEditor.cs
- BCLDebug.cs
- GlyphInfoList.cs
- XomlCompilerError.cs
- MethodImplAttribute.cs
- PolyLineSegment.cs
- TextViewBase.cs
- XMLSchema.cs
- HtmlInputHidden.cs
- ConfigXmlWhitespace.cs
- ProcessInputEventArgs.cs
- SimpleWebHandlerParser.cs
- DataGridLinkButton.cs
- CacheVirtualItemsEvent.cs
- DrawingContext.cs
- streamingZipPartStream.cs
- NullableConverter.cs
- JulianCalendar.cs
- GeometryHitTestResult.cs
- OrderPreservingPipeliningMergeHelper.cs
- ValidateNames.cs
- RemoteHelper.cs
- DeploymentSectionCache.cs
- ManipulationVelocities.cs
- ViewStateException.cs
- TempFiles.cs
- XmlSchemaException.cs
- HierarchicalDataTemplate.cs
- HybridDictionary.cs
- DiscoveryExceptionDictionary.cs
- LogSwitch.cs
- DataProtection.cs
- Logging.cs
- FullTextState.cs
- FloaterParaClient.cs
- Point3DKeyFrameCollection.cs
- Thread.cs
- RegexStringValidatorAttribute.cs
- HandlerFactoryCache.cs
- SystemIcons.cs
- Domain.cs
- LinkConverter.cs
- AppearanceEditorPart.cs
- SettingsPropertyValue.cs
- PropertyBuilder.cs
- WindowsToolbar.cs
- ValidationErrorCollection.cs
- BamlResourceContent.cs
- IsolationInterop.cs
- UriScheme.cs
- LoaderAllocator.cs
- Bezier.cs
- SqlConnectionPoolGroupProviderInfo.cs
- DocumentXPathNavigator.cs
- NonVisualControlAttribute.cs
- BamlRecordHelper.cs
- IconHelper.cs
- WizardStepBase.cs
- Cursors.cs
- fixedPageContentExtractor.cs
- ProxyFragment.cs
- JulianCalendar.cs
- EntityClassGenerator.cs
- Coordinator.cs
- dbdatarecord.cs
- MachineSettingsSection.cs
- DummyDataSource.cs
- TableLayoutCellPaintEventArgs.cs
- NetCodeGroup.cs
- PersonalizationState.cs
- GenericsNotImplementedException.cs
- StateBag.cs
- FixedSOMTable.cs
- ExeContext.cs
- XmlAttributeCollection.cs
- MediaContextNotificationWindow.cs
- ActivityExecutionContext.cs
- XmlEntity.cs
- CompModHelpers.cs
- ProfileSettings.cs
- LogEntrySerialization.cs
- StrongName.cs
- MruCache.cs
- NativeCompoundFileAPIs.cs
- MemoryFailPoint.cs
- PermissionToken.cs
- StrokeIntersection.cs
- HexParser.cs
- DataSetViewSchema.cs
- RuntimeConfig.cs
- ApplicationGesture.cs
- CodeAttributeDeclaration.cs
- DispatcherExceptionFilterEventArgs.cs
- CompModSwitches.cs