Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Xml / XmlAtomErrorReader.cs / 1407647 / XmlAtomErrorReader.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a wrapping XmlReader that can detect in-line errors. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client.Xml { #region Namespaces. using System.Diagnostics; using System.Xml; #endregion Namespaces. ///Use this class to wrap an existing [DebuggerDisplay("XmlAtomErrorReader {NodeType} {Name} {Value}")] internal class XmlAtomErrorReader : XmlWrappingReader { ///. Initializes a new /// Reader to wrap. internal XmlAtomErrorReader(XmlReader baseReader) : base(baseReader) { Debug.Assert(baseReader != null, "baseReader != null"); this.Reader = baseReader; } #region Methods. ///instance. Reads the next node from the stream. ///true if the next node was read successfully; false if there are no more nodes to read. public override bool Read() { bool result = base.Read(); if (this.NodeType == XmlNodeType.Element && Util.AreSame(this.Reader, XmlConstants.XmlErrorElementName, XmlConstants.DataWebMetadataNamespace)) { string message = ReadErrorMessage(this.Reader); // In case of instream errors, the status code should be 500 (which is the default) throw new DataServiceClientException(Strings.Deserialize_ServerException(message)); } return result; } ///Reads an element string from the specified /// Reader to get value from. /// Whether a null attribute marker should be checked on the element. ///. The text value within the element, possibly null. ////// Simple values only are expected - mixed content will throw an error. /// Interspersed comments are ignored. /// internal static string ReadElementString(XmlReader reader, bool checkNullAttribute) { Debug.Assert(reader != null, "reader != null"); Debug.Assert(XmlNodeType.Element == reader.NodeType, "not positioned on Element"); string result = null; bool empty = checkNullAttribute && !Util.DoesNullAttributeSayTrue(reader); if (reader.IsEmptyElement) { return (empty ? String.Empty : null); } while (reader.Read()) { switch (reader.NodeType) { case XmlNodeType.EndElement: return result ?? (empty ? String.Empty : null); case XmlNodeType.CDATA: case XmlNodeType.Text: case XmlNodeType.SignificantWhitespace: if (null != result) { throw Error.InvalidOperation(Strings.Deserialize_MixedTextWithComment); } result = reader.Value; break; case XmlNodeType.Comment: case XmlNodeType.Whitespace: break; case XmlNodeType.Element: default: throw Error.InvalidOperation(Strings.Deserialize_ExpectingSimpleValue); } } // xml ended before EndElement? throw Error.InvalidOperation(Strings.Deserialize_ExpectingSimpleValue); } ///With the reader positioned on an 'error' element, reads the text of the 'message' child. ///from which to read a WCF Data Service inline error message. /// The text of the 'message' child element, empty if not found. private static string ReadErrorMessage(XmlReader reader) { Debug.Assert(reader != null, "reader != null"); Debug.Assert(reader.NodeType == XmlNodeType.Element, "reader.NodeType == XmlNodeType.Element"); Debug.Assert(reader.LocalName == XmlConstants.XmlErrorElementName, "reader.LocalName == XmlConstants.XmlErrorElementName"); int depth = 1; while (depth > 0 && reader.Read()) { if (reader.NodeType == XmlNodeType.Element) { if (!reader.IsEmptyElement) { depth++; } if (depth == 2 && Util.AreSame(reader, XmlConstants.XmlErrorMessageElementName, XmlConstants.DataWebMetadataNamespace)) { return ReadElementString(reader, false); } } else if (reader.NodeType == XmlNodeType.EndElement) { depth--; } } return String.Empty; } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
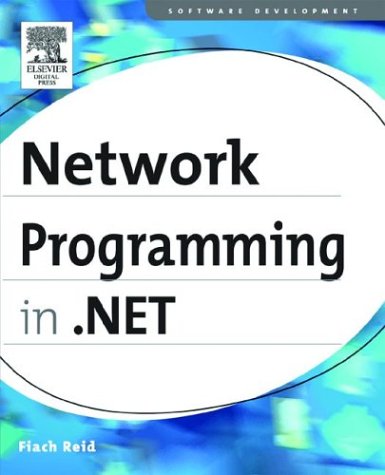
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeMember.cs
- SystemIPGlobalProperties.cs
- CombinedGeometry.cs
- OleDbConnection.cs
- Validator.cs
- StoreItemCollection.Loader.cs
- RecognizeCompletedEventArgs.cs
- MemberAccessException.cs
- ControlPropertyNameConverter.cs
- ModulesEntry.cs
- FieldNameLookup.cs
- BitStream.cs
- BitmapMetadataEnumerator.cs
- DataGridViewCellStyleConverter.cs
- Rect3DValueSerializer.cs
- metadatamappinghashervisitor.cs
- DoubleAnimationUsingPath.cs
- BehaviorEditorPart.cs
- OracleConnectionStringBuilder.cs
- BamlResourceContent.cs
- TextTreePropertyUndoUnit.cs
- ContainerControlDesigner.cs
- PageAsyncTask.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- Rotation3DKeyFrameCollection.cs
- TypeGenericEnumerableViewSchema.cs
- InternalBase.cs
- SHA1CryptoServiceProvider.cs
- DecimalConstantAttribute.cs
- SHA384Managed.cs
- SqlDependency.cs
- ArithmeticException.cs
- ScrollProperties.cs
- HealthMonitoringSectionHelper.cs
- CollectionViewGroupInternal.cs
- HtmlInputReset.cs
- ILGenerator.cs
- WebPartConnectionCollection.cs
- Rect3DConverter.cs
- ReachDocumentReferenceSerializerAsync.cs
- SafeLibraryHandle.cs
- CqlParserHelpers.cs
- DataTableMapping.cs
- LicenseProviderAttribute.cs
- TextStore.cs
- ReadWriteObjectLock.cs
- BooleanAnimationUsingKeyFrames.cs
- GroupItemAutomationPeer.cs
- PersonalizableAttribute.cs
- DataContractAttribute.cs
- SmtpNtlmAuthenticationModule.cs
- DictionaryGlobals.cs
- BitmapFrameEncode.cs
- TextServicesCompartment.cs
- BasicBrowserDialog.cs
- ConditionCollection.cs
- BuildProvider.cs
- XmlReader.cs
- SizeChangedEventArgs.cs
- HeaderedContentControl.cs
- CredentialSelector.cs
- BasePropertyDescriptor.cs
- ControlCollection.cs
- IntranetCredentialPolicy.cs
- RawStylusSystemGestureInputReport.cs
- Label.cs
- CodeTypeMemberCollection.cs
- SmiConnection.cs
- CompilerGlobalScopeAttribute.cs
- WebEventTraceProvider.cs
- HtmlTextArea.cs
- SplineQuaternionKeyFrame.cs
- TextEndOfSegment.cs
- EncryptedPackage.cs
- CellParagraph.cs
- FixedSOMTable.cs
- LogLogRecord.cs
- KeyedQueue.cs
- BamlResourceSerializer.cs
- OuterGlowBitmapEffect.cs
- MetadataPropertyCollection.cs
- OdbcConnectionPoolProviderInfo.cs
- DataPagerFieldCollection.cs
- SetterBaseCollection.cs
- AssemblyBuilder.cs
- EventlogProvider.cs
- ThreadStateException.cs
- FontWeightConverter.cs
- EntityViewGenerationConstants.cs
- ProvidersHelper.cs
- SizeValueSerializer.cs
- CodeMethodReturnStatement.cs
- Compiler.cs
- UnsafeNativeMethods.cs
- TextTreeUndoUnit.cs
- PartialArray.cs
- Point3DCollection.cs
- ManipulationInertiaStartingEventArgs.cs
- Point3DAnimation.cs
- SrgsOneOf.cs