Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / FixedSOMTable.cs / 1 / FixedSOMTable.cs
/*++ File: FixedSOMTable.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: This class reprsents a table on the page History: 05/17/2005: eleese - Created --*/ namespace System.Windows.Documents { using System.Windows.Shapes; using System.Windows.Media; using System.Diagnostics; using System.Windows; using System.Globalization; internal sealed class FixedSOMTable : FixedSOMPageElement { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors public FixedSOMTable(FixedSOMPage page) : base(page) { _numCols = 0; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //--------------------------------------------------------------------- #region Public Methods #if DEBUG public override void Render(DrawingContext dc, string label, DrawDebugVisual debugVisual) { Pen pen = new Pen(Brushes.Green, 5); Rect rect = _boundingRect; dc.DrawRectangle(null, pen , rect); CultureInfo EnglishCulture = CultureInfo.GetCultureInfoByIetfLanguageTag("en-US"); FormattedText ft = new FormattedText(label, EnglishCulture, FlowDirection.LeftToRight, new Typeface("Courier New"), 20, Brushes.Blue); Point labelLocation = new Point(rect.Left-25, (rect.Bottom + rect.Top)/2 - 10); // dc.DrawText(ft, labelLocation); for (int i = 0; i < _semanticBoxes.Count; i++) { _semanticBoxes[i].Render(dc, label + " " + i.ToString(),debugVisual); } } #endif public void AddRow(FixedSOMTableRow row) { base.Add(row); int colCount = row.SemanticBoxes.Count; if (colCount > _numCols) { _numCols = colCount; } } public bool AddContainer(FixedSOMContainer container) { Rect bounds = container.BoundingRect; //Allow couple pixels margin double verticalOverlap = bounds.Height * 0.2; double horizontalOverlap = bounds.Width * 0.2; bounds.Inflate(-horizontalOverlap,-verticalOverlap); if (this.BoundingRect.Contains(bounds)) { foreach (FixedSOMTableRow row in this.SemanticBoxes) { if (row.BoundingRect.Contains(bounds)) { foreach (FixedSOMTableCell cell in row.SemanticBoxes) { if (cell.BoundingRect.Contains(bounds)) { cell.AddContainer(container); FixedSOMFixedBlock block = container as FixedSOMFixedBlock; if (block != null) { if (block.IsRTL) { _RTLCount++; } else { _LTRCount++; } } return true; } } } } } return false; } public override void SetRTFProperties(FixedElement element) { if (element.Type == typeof(Table)) { element.SetValue(Table.CellSpacingProperty, 0.0); } } #endregion Public Methods #region Public Properties public override bool IsRTL { get { return _RTLCount > _LTRCount; } } #endregion Public Properties #region Internal Properties internal override FixedElement.ElementType[] ElementTypes { get { return new FixedElement.ElementType[2] { FixedElement.ElementType.Table, FixedElement.ElementType.TableRowGroup }; } } internal bool IsEmpty { get { foreach (FixedSOMTableRow row in this.SemanticBoxes) { if (!row.IsEmpty) { return false; } } return true; } } internal bool IsSingleCelled { get { if (this.SemanticBoxes.Count == 1) { FixedSOMTableRow row = this.SemanticBoxes[0] as FixedSOMTableRow; Debug.Assert(row != null); return (row.SemanticBoxes.Count == 1); } return false; } } #endregion Internal Properties #region Internal methods internal void DeleteEmptyRows() { for (int i=0; inextCol) { deleteCol = false; } } } if (deleteCol) { for (r = 0; r < nRows; r++) { FixedSOMTableRow row = (FixedSOMTableRow)(this.SemanticBoxes[r]); row.SemanticBoxes.RemoveAt(indexInRow[r]); } if (nextCol == double.MaxValue) { break; } else { continue; } } // are we done? if (nextCol == double.MaxValue) { break; } // increment ColumnSpans, indexInRow for (r = 0; r < nRows; r++) { FixedSOMTableRow row = (FixedSOMTableRow)(this.SemanticBoxes[r]); int idx = indexInRow[r]; if (idx + 1 < row.SemanticBoxes.Count && row.SemanticBoxes[idx + 1].BoundingRect.Left == nextCol) { indexInRow[r] = idx + 1; } else { ((FixedSOMTableCell)row.SemanticBoxes[idx]).ColumnSpan++; } } } } #endregion Internal methods //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields const double _minColumnWidth = 5; // empty columns narrower than this will be deleted const double _minRowHeight = 10; //empty rows smaller than this will be deleted private int _RTLCount; private int _LTRCount; int _numCols; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /*++ File: FixedSOMTable.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: This class reprsents a table on the page History: 05/17/2005: eleese - Created --*/ namespace System.Windows.Documents { using System.Windows.Shapes; using System.Windows.Media; using System.Diagnostics; using System.Windows; using System.Globalization; internal sealed class FixedSOMTable : FixedSOMPageElement { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors public FixedSOMTable(FixedSOMPage page) : base(page) { _numCols = 0; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //--------------------------------------------------------------------- #region Public Methods #if DEBUG public override void Render(DrawingContext dc, string label, DrawDebugVisual debugVisual) { Pen pen = new Pen(Brushes.Green, 5); Rect rect = _boundingRect; dc.DrawRectangle(null, pen , rect); CultureInfo EnglishCulture = CultureInfo.GetCultureInfoByIetfLanguageTag("en-US"); FormattedText ft = new FormattedText(label, EnglishCulture, FlowDirection.LeftToRight, new Typeface("Courier New"), 20, Brushes.Blue); Point labelLocation = new Point(rect.Left-25, (rect.Bottom + rect.Top)/2 - 10); // dc.DrawText(ft, labelLocation); for (int i = 0; i < _semanticBoxes.Count; i++) { _semanticBoxes[i].Render(dc, label + " " + i.ToString(),debugVisual); } } #endif public void AddRow(FixedSOMTableRow row) { base.Add(row); int colCount = row.SemanticBoxes.Count; if (colCount > _numCols) { _numCols = colCount; } } public bool AddContainer(FixedSOMContainer container) { Rect bounds = container.BoundingRect; //Allow couple pixels margin double verticalOverlap = bounds.Height * 0.2; double horizontalOverlap = bounds.Width * 0.2; bounds.Inflate(-horizontalOverlap,-verticalOverlap); if (this.BoundingRect.Contains(bounds)) { foreach (FixedSOMTableRow row in this.SemanticBoxes) { if (row.BoundingRect.Contains(bounds)) { foreach (FixedSOMTableCell cell in row.SemanticBoxes) { if (cell.BoundingRect.Contains(bounds)) { cell.AddContainer(container); FixedSOMFixedBlock block = container as FixedSOMFixedBlock; if (block != null) { if (block.IsRTL) { _RTLCount++; } else { _LTRCount++; } } return true; } } } } } return false; } public override void SetRTFProperties(FixedElement element) { if (element.Type == typeof(Table)) { element.SetValue(Table.CellSpacingProperty, 0.0); } } #endregion Public Methods #region Public Properties public override bool IsRTL { get { return _RTLCount > _LTRCount; } } #endregion Public Properties #region Internal Properties internal override FixedElement.ElementType[] ElementTypes { get { return new FixedElement.ElementType[2] { FixedElement.ElementType.Table, FixedElement.ElementType.TableRowGroup }; } } internal bool IsEmpty { get { foreach (FixedSOMTableRow row in this.SemanticBoxes) { if (!row.IsEmpty) { return false; } } return true; } } internal bool IsSingleCelled { get { if (this.SemanticBoxes.Count == 1) { FixedSOMTableRow row = this.SemanticBoxes[0] as FixedSOMTableRow; Debug.Assert(row != null); return (row.SemanticBoxes.Count == 1); } return false; } } #endregion Internal Properties #region Internal methods internal void DeleteEmptyRows() { for (int i=0; i nextCol) { deleteCol = false; } } } if (deleteCol) { for (r = 0; r < nRows; r++) { FixedSOMTableRow row = (FixedSOMTableRow)(this.SemanticBoxes[r]); row.SemanticBoxes.RemoveAt(indexInRow[r]); } if (nextCol == double.MaxValue) { break; } else { continue; } } // are we done? if (nextCol == double.MaxValue) { break; } // increment ColumnSpans, indexInRow for (r = 0; r < nRows; r++) { FixedSOMTableRow row = (FixedSOMTableRow)(this.SemanticBoxes[r]); int idx = indexInRow[r]; if (idx + 1 < row.SemanticBoxes.Count && row.SemanticBoxes[idx + 1].BoundingRect.Left == nextCol) { indexInRow[r] = idx + 1; } else { ((FixedSOMTableCell)row.SemanticBoxes[idx]).ColumnSpan++; } } } } #endregion Internal methods //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields const double _minColumnWidth = 5; // empty columns narrower than this will be deleted const double _minRowHeight = 10; //empty rows smaller than this will be deleted private int _RTLCount; private int _LTRCount; int _numCols; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
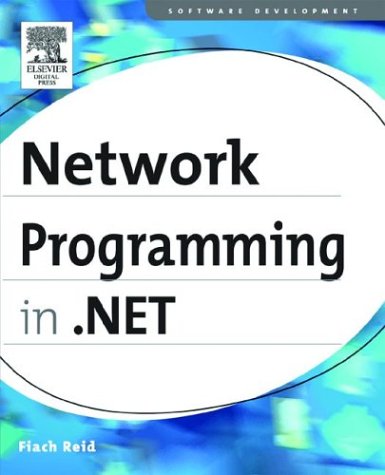
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameValuePermission.cs
- SafeLibraryHandle.cs
- VarInfo.cs
- RadioButtonList.cs
- TextTreeDeleteContentUndoUnit.cs
- SelectionItemProviderWrapper.cs
- ExpandCollapseProviderWrapper.cs
- EntityDataSourceContainerNameItem.cs
- MailWriter.cs
- KnownBoxes.cs
- BinaryConverter.cs
- RIPEMD160.cs
- XmlSerializer.cs
- PrePrepareMethodAttribute.cs
- GlobalizationAssembly.cs
- SimplePropertyEntry.cs
- StringFreezingAttribute.cs
- InteropAutomationProvider.cs
- HashHelper.cs
- TreeIterators.cs
- SimpleApplicationHost.cs
- GlyphRunDrawing.cs
- ButtonBase.cs
- CaseInsensitiveComparer.cs
- SmiXetterAccessMap.cs
- Types.cs
- HttpCacheVary.cs
- figurelengthconverter.cs
- _ShellExpression.cs
- Oci.cs
- TrustManagerMoreInformation.cs
- GenericArgumentsUpdater.cs
- WsdlBuildProvider.cs
- Win32Native.cs
- Style.cs
- MetadataWorkspace.cs
- ServiceActivationException.cs
- MenuItemStyle.cs
- UnsafeNativeMethodsMilCoreApi.cs
- Slider.cs
- BitmapMetadataEnumerator.cs
- XmlParserContext.cs
- OptimizedTemplateContentHelper.cs
- SqlCrossApplyToCrossJoin.cs
- SupportedAddressingMode.cs
- TimerEventSubscriptionCollection.cs
- StorageSetMapping.cs
- InputLanguage.cs
- DispatcherHookEventArgs.cs
- ProfileBuildProvider.cs
- RuleSettings.cs
- SqlRowUpdatingEvent.cs
- SoapFault.cs
- Variant.cs
- XsltOutput.cs
- Walker.cs
- ApplicationDirectory.cs
- DiagnosticTrace.cs
- Relationship.cs
- Events.cs
- CompiledQuery.cs
- StringArrayEditor.cs
- SemanticBasicElement.cs
- EntityContainerRelationshipSetEnd.cs
- NativeMethods.cs
- IdentitySection.cs
- ParallelEnumerable.cs
- MeasureItemEvent.cs
- GreenMethods.cs
- CollectionType.cs
- TableLayoutPanelDesigner.cs
- ListControl.cs
- DoubleAnimationBase.cs
- HttpGetServerProtocol.cs
- ChameleonKey.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- ResponseStream.cs
- MenuItemCollection.cs
- InstanceLockedException.cs
- InputScope.cs
- Sql8ExpressionRewriter.cs
- Suspend.cs
- ProfileElement.cs
- TcpProcessProtocolHandler.cs
- ProfessionalColors.cs
- SafeLibraryHandle.cs
- _NegotiateClient.cs
- ItemsPresenter.cs
- MeasurementDCInfo.cs
- ProfileInfo.cs
- DataStorage.cs
- ToolStripItemRenderEventArgs.cs
- BindingListCollectionView.cs
- HScrollProperties.cs
- SqlBooleanMismatchVisitor.cs
- CharUnicodeInfo.cs
- ComplexTypeEmitter.cs
- DataSourceCache.cs
- CaseInsensitiveComparer.cs
- WebScriptEnablingElement.cs