Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Profile / ProfileInfo.cs / 1 / ProfileInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ProfileInfo * * Copyright (c) 2002 Microsoft Corporation */ namespace System.Web.Profile { using System.Security.Principal; using System.Security.Permissions; using System.Collections; using System.Collections.Specialized; using System.Web.Configuration; using System.Web.Util; using System.Web.Security; using System.Web.Compilation; using System.Configuration; using System.Configuration.Provider; using System.Reflection; using System.CodeDom; [Serializable] [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ProfileInfo { public ProfileInfo(string username, bool isAnonymous, DateTime lastActivityDate, DateTime lastUpdatedDate, int size) { if( username != null ) { username = username.Trim(); } _UserName = username; if (lastActivityDate.Kind == DateTimeKind.Local) { lastActivityDate = lastActivityDate.ToUniversalTime(); } _LastActivityDate = lastActivityDate; if (lastUpdatedDate.Kind == DateTimeKind.Local) { lastUpdatedDate = lastUpdatedDate.ToUniversalTime(); } _LastUpdatedDate = lastUpdatedDate; _IsAnonymous = isAnonymous; _Size = size; } protected ProfileInfo() { } public virtual string UserName { get { return _UserName;} } public virtual DateTime LastActivityDate { get { return _LastActivityDate.ToLocalTime();} } public virtual DateTime LastUpdatedDate { get { return _LastUpdatedDate.ToLocalTime(); } } public virtual bool IsAnonymous { get { return _IsAnonymous;} } public virtual int Size { get { return _Size; } } private string _UserName; private DateTime _LastActivityDate; private DateTime _LastUpdatedDate; private bool _IsAnonymous; private int _Size; } [Serializable] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ProfileInfoCollection : IEnumerable, ICollection { private Hashtable _Hashtable = null; private ArrayList _ArrayList = null; private bool _ReadOnly = false; private int _CurPos = 0; private int _NumBlanks = 0; public ProfileInfoCollection() { _Hashtable = new Hashtable(10, StringComparer.CurrentCultureIgnoreCase); _ArrayList = new ArrayList(); } public void Add(ProfileInfo profileInfo) { if (_ReadOnly) throw new NotSupportedException(); if (profileInfo == null || profileInfo.UserName == null) throw new ArgumentNullException( "profileInfo" ); _Hashtable.Add(profileInfo.UserName, _CurPos); _ArrayList.Add(profileInfo); _CurPos++; } public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); object pos = _Hashtable[name]; if (pos == null) return; _Hashtable.Remove(name); _ArrayList[(int)pos] = null; _NumBlanks++; } public ProfileInfo this[string name] { get { object pos = _Hashtable[name]; if (pos == null) return null; return _ArrayList[(int)pos] as ProfileInfo; } } public IEnumerator GetEnumerator() { DoCompact(); return _ArrayList.GetEnumerator(); } public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; } public void Clear() { if (_ReadOnly) throw new NotSupportedException(); _Hashtable.Clear(); _ArrayList.Clear(); _CurPos = 0; _NumBlanks = 0; } public int Count { get { return _Hashtable.Count; } } public bool IsSynchronized {get { return false;}} public object SyncRoot {get { return this;}} public void CopyTo(Array array, int index) { DoCompact(); _ArrayList.CopyTo(array, index); } public void CopyTo(ProfileInfo [] array, int index) { DoCompact(); _ArrayList.CopyTo(array, index); } private void DoCompact() { if (_NumBlanks < 1) return; ArrayList al = new ArrayList(_CurPos - _NumBlanks); int firstBlankPos = -1; for (int iter = 0; iter < _CurPos; iter++) { if (_ArrayList[iter] != null) al.Add(_ArrayList[iter]); else if (firstBlankPos == -1) firstBlankPos = iter; } _NumBlanks = 0; _ArrayList = al; _CurPos = _ArrayList.Count; for (int iter = firstBlankPos; iter < _CurPos; iter++) { ProfileInfo profileInfo = _ArrayList[iter] as ProfileInfo; _Hashtable[profileInfo.UserName] = iter; } } } }
Link Menu
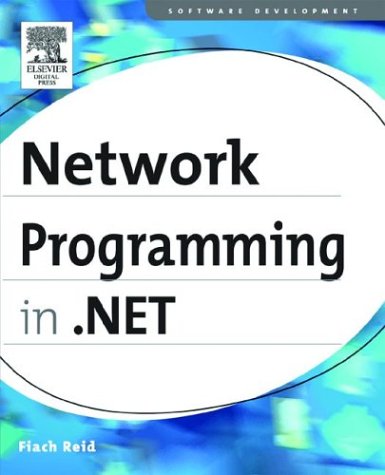
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementIsland.cs
- CapabilitiesSection.cs
- EntityCommandDefinition.cs
- Hash.cs
- QueryResults.cs
- PreloadHost.cs
- XomlCompilerError.cs
- UrlPath.cs
- WebScriptServiceHost.cs
- WindowsAuthenticationEventArgs.cs
- ImageBrush.cs
- NotSupportedException.cs
- RequestCacheEntry.cs
- Padding.cs
- ButtonFieldBase.cs
- SystemEvents.cs
- FileSystemEventArgs.cs
- Decorator.cs
- Nullable.cs
- NativeMethods.cs
- SocketAddress.cs
- FormClosedEvent.cs
- InfoCardHelper.cs
- PolicyLevel.cs
- UriTemplateVariablePathSegment.cs
- XmlAnyElementAttribute.cs
- QilList.cs
- ReferenceConverter.cs
- TextTreeDeleteContentUndoUnit.cs
- PanelStyle.cs
- BitmapData.cs
- SecureConversationSecurityTokenParameters.cs
- XmlIncludeAttribute.cs
- GuidelineCollection.cs
- TextOutput.cs
- RequiredAttributeAttribute.cs
- UserControlAutomationPeer.cs
- ILGenerator.cs
- Span.cs
- Compensate.cs
- AdministrationHelpers.cs
- NavigationHelper.cs
- CompletedAsyncResult.cs
- util.cs
- NullableLongMinMaxAggregationOperator.cs
- Baml2006ReaderSettings.cs
- CharacterMetricsDictionary.cs
- EntityViewContainer.cs
- EventSourceCreationData.cs
- SafeNativeMethods.cs
- PrimarySelectionAdorner.cs
- OleStrCAMarshaler.cs
- ThreadStaticAttribute.cs
- ReadOnlyHierarchicalDataSourceView.cs
- FixedDocumentSequencePaginator.cs
- TreeNodeCollectionEditor.cs
- SqlUserDefinedTypeAttribute.cs
- DataPager.cs
- SerializationException.cs
- Int32EqualityComparer.cs
- DataGridCell.cs
- RepeaterCommandEventArgs.cs
- SQLSingle.cs
- Invariant.cs
- ToolStripPanelSelectionBehavior.cs
- SqlProfileProvider.cs
- SQLInt32.cs
- VisualStyleInformation.cs
- MethodExpression.cs
- ScriptReferenceBase.cs
- TypedOperationInfo.cs
- DetailsViewCommandEventArgs.cs
- Context.cs
- AutomationPattern.cs
- UIElementParagraph.cs
- CodeExpressionRuleDeclaration.cs
- SqlMetaData.cs
- ArraySegment.cs
- SubstitutionList.cs
- XdrBuilder.cs
- DocumentXmlWriter.cs
- RTLAwareMessageBox.cs
- Metadata.cs
- WpfMemberInvoker.cs
- MultilineStringConverter.cs
- PropertyIDSet.cs
- RenderData.cs
- ConnectorDragDropGlyph.cs
- DataControlFieldHeaderCell.cs
- AsymmetricSignatureFormatter.cs
- MultitargetUtil.cs
- InvokeGenerator.cs
- DrawingBrush.cs
- RijndaelManaged.cs
- Helper.cs
- XmlNotation.cs
- ExternalCalls.cs
- TimeEnumHelper.cs
- TypeUnloadedException.cs
- HttpHeaderCollection.cs