Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / SubstitutionList.cs / 1 / SubstitutionList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// Data structure for use in CloneAndReplace /// ///Isolates the many QilNode classes from changes in /// the underlying data structure. internal sealed class SubstitutionList { // private ArrayList s; public SubstitutionList() { this.s = new ArrayList(4); } ////// Add a substituion pair /// /// a node to be replaced /// its replacement public void AddSubstitutionPair(QilNode find, QilNode replace) { s.Add(find); s.Add(replace); } ////// Remove the last a substituion pair /// public void RemoveLastSubstitutionPair() { s.RemoveRange(s.Count - 2, 2); } ////// Remove the last N substitution pairs /// public void RemoveLastNSubstitutionPairs(int n) { Debug.Assert(n >= 0, "n must be nonnegative"); if (n > 0) { n *= 2; s.RemoveRange(s.Count - n, n); } } ////// Find the replacement for a node /// /// the node to replace ///null if no replacement is found public QilNode FindReplacement(QilNode n) { Debug.Assert(s.Count % 2 == 0); for (int i = s.Count-2; i >= 0; i-=2) if (s[i] == n) return (QilNode)s[i+1]; return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// Data structure for use in CloneAndReplace /// ///Isolates the many QilNode classes from changes in /// the underlying data structure. internal sealed class SubstitutionList { // private ArrayList s; public SubstitutionList() { this.s = new ArrayList(4); } ////// Add a substituion pair /// /// a node to be replaced /// its replacement public void AddSubstitutionPair(QilNode find, QilNode replace) { s.Add(find); s.Add(replace); } ////// Remove the last a substituion pair /// public void RemoveLastSubstitutionPair() { s.RemoveRange(s.Count - 2, 2); } ////// Remove the last N substitution pairs /// public void RemoveLastNSubstitutionPairs(int n) { Debug.Assert(n >= 0, "n must be nonnegative"); if (n > 0) { n *= 2; s.RemoveRange(s.Count - n, n); } } ////// Find the replacement for a node /// /// the node to replace ///null if no replacement is found public QilNode FindReplacement(QilNode n) { Debug.Assert(s.Count % 2 == 0); for (int i = s.Count-2; i >= 0; i-=2) if (s[i] == n) return (QilNode)s[i+1]; return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
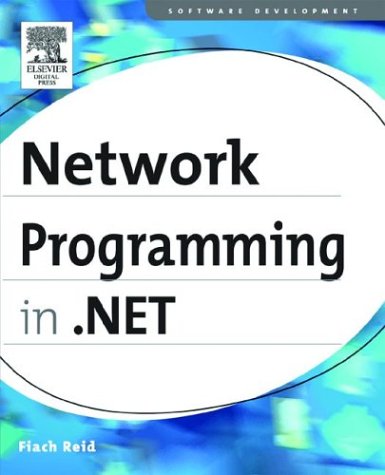
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleStrCAMarshaler.cs
- XmlSchemaObjectCollection.cs
- CalendarButton.cs
- RightsManagementSuppressedStream.cs
- RequestedSignatureDialog.cs
- WebPartVerb.cs
- ProbeMatches11.cs
- AssociationType.cs
- GlyphRunDrawing.cs
- ManagementEventWatcher.cs
- SID.cs
- BatchServiceHost.cs
- Bitmap.cs
- IntAverageAggregationOperator.cs
- HttpChannelBindingToken.cs
- MimeTypeAttribute.cs
- XmlAttributeCache.cs
- RecordConverter.cs
- TextRangeEditTables.cs
- Mapping.cs
- SMSvcHost.cs
- MaterialCollection.cs
- ObjectPersistData.cs
- FormParameter.cs
- ApplicationSecurityManager.cs
- SplitterEvent.cs
- Blend.cs
- mongolianshape.cs
- IconHelper.cs
- TraceEventCache.cs
- ButtonBase.cs
- ContextMenuAutomationPeer.cs
- AnimationClock.cs
- OneWayChannelFactory.cs
- ConnectionProviderAttribute.cs
- Comparer.cs
- ProcessHostServerConfig.cs
- WebResourceAttribute.cs
- FixedDocumentPaginator.cs
- CheckBox.cs
- XNameTypeConverter.cs
- MessageSecurityException.cs
- ToolStripSplitStackLayout.cs
- EncodingInfo.cs
- CellLabel.cs
- safelink.cs
- CatalogZone.cs
- __ConsoleStream.cs
- PerformanceCounter.cs
- SqlNode.cs
- WpfMemberInvoker.cs
- CompositionAdorner.cs
- DependencyStoreSurrogate.cs
- ConfigurationValidatorAttribute.cs
- ProxyElement.cs
- DefaultMemberAttribute.cs
- RTLAwareMessageBox.cs
- Int32CollectionConverter.cs
- XsdDateTime.cs
- TextContainerChangedEventArgs.cs
- TargetException.cs
- Int32.cs
- SharedPerformanceCounter.cs
- RuntimeConfig.cs
- ColumnMapProcessor.cs
- assertwrapper.cs
- RightsManagementEncryptionTransform.cs
- QueueProcessor.cs
- SimpleBitVector32.cs
- FontClient.cs
- XsltContext.cs
- XNodeNavigator.cs
- AppLevelCompilationSectionCache.cs
- WebPartCloseVerb.cs
- GlyphRunDrawing.cs
- InfoCardSymmetricAlgorithm.cs
- EventSinkActivity.cs
- ServiceReference.cs
- ContextTokenTypeConverter.cs
- AnnotationResourceChangedEventArgs.cs
- DataGridViewIntLinkedList.cs
- RuntimeArgumentHandle.cs
- CroppedBitmap.cs
- XmlEncoding.cs
- WindowsGrip.cs
- SoapInteropTypes.cs
- AsyncOperation.cs
- SmiEventStream.cs
- LogLogRecordHeader.cs
- ColorPalette.cs
- FilteredDataSetHelper.cs
- _AutoWebProxyScriptWrapper.cs
- CertificateElement.cs
- SafeNativeMethods.cs
- AuthorizationSection.cs
- LineSegment.cs
- BinaryObjectInfo.cs
- BaseTemplatedMobileComponentEditor.cs
- TrackingServices.cs
- ListDictionaryInternal.cs