Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Point4D.cs / 1 / Point4D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
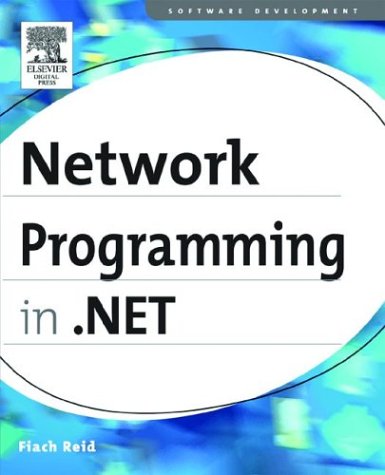
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadOnlyNameValueCollection.cs
- CompModSwitches.cs
- Emitter.cs
- WindowsFormsHost.cs
- SymbolDocumentInfo.cs
- OleDbTransaction.cs
- SQLBytes.cs
- XPathEmptyIterator.cs
- ZipArchive.cs
- Point3DAnimationUsingKeyFrames.cs
- EventWaitHandleSecurity.cs
- TextTabProperties.cs
- SchemaImporterExtensionElementCollection.cs
- securitymgrsite.cs
- ToolboxCategory.cs
- DefaultClaimSet.cs
- GridViewCancelEditEventArgs.cs
- BaseParaClient.cs
- AssociationTypeEmitter.cs
- MeshGeometry3D.cs
- Parser.cs
- SqlConnectionString.cs
- CanExpandCollapseAllConverter.cs
- NumericUpDown.cs
- ScriptBehaviorDescriptor.cs
- TypedMessageConverter.cs
- RepeaterItemCollection.cs
- LocatorGroup.cs
- BooleanKeyFrameCollection.cs
- Pair.cs
- Point3DConverter.cs
- DelegateHelpers.Generated.cs
- MultiBindingExpression.cs
- ValidationError.cs
- ObservableCollection.cs
- InstanceDescriptor.cs
- WebDisplayNameAttribute.cs
- XmlCharCheckingWriter.cs
- ScriptModule.cs
- EpmSyndicationContentSerializer.cs
- XmlDocumentFragment.cs
- TableItemStyle.cs
- Config.cs
- SqlConnectionString.cs
- SqlConnectionString.cs
- GraphicsContainer.cs
- TraceHandlerErrorFormatter.cs
- ToolStripDropDownItem.cs
- EventProvider.cs
- DbExpressionVisitor.cs
- DBCommand.cs
- VirtualDirectoryMapping.cs
- WebControlParameterProxy.cs
- PersonalizablePropertyEntry.cs
- XamlFilter.cs
- InheritanceContextHelper.cs
- SessionIDManager.cs
- CallId.cs
- Stacktrace.cs
- PathHelper.cs
- ThreadStartException.cs
- ListParaClient.cs
- TraceEventCache.cs
- ScrollBarAutomationPeer.cs
- IImplicitResourceProvider.cs
- XmlArrayAttribute.cs
- MenuTracker.cs
- Rules.cs
- StandardBindingElement.cs
- MapPathBasedVirtualPathProvider.cs
- CharUnicodeInfo.cs
- StateWorkerRequest.cs
- GlyphRun.cs
- RadialGradientBrush.cs
- RotationValidation.cs
- ExtendedProperty.cs
- XmlSerializerImportOptions.cs
- DynamicExpression.cs
- VariantWrapper.cs
- NavigationFailedEventArgs.cs
- LocationFactory.cs
- NamedPipeProcessProtocolHandler.cs
- TextRangeProviderWrapper.cs
- errorpatternmatcher.cs
- ToolboxItemCollection.cs
- documentsequencetextcontainer.cs
- ToolTip.cs
- GenericTypeParameterBuilder.cs
- cache.cs
- XpsS0ValidatingLoader.cs
- Pen.cs
- ExpressionPrefixAttribute.cs
- UseLicense.cs
- Script.cs
- LocalizabilityAttribute.cs
- ListQueryResults.cs
- FullTextState.cs
- BCLDebug.cs
- SqlCacheDependencyDatabaseCollection.cs
- AssociationType.cs