Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / DefaultWorkflowTransactionService.cs / 1305376 / DefaultWorkflowTransactionService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Transactions; using System.Collections.Specialized; using System.Diagnostics; using System.Configuration; namespace System.Workflow.Runtime.Hosting { ///A simple TransactionService that creates /// public class DefaultWorkflowCommitWorkBatchService : WorkflowCommitWorkBatchService { private bool _enableRetries = false; private bool _ignoreCommonEnableRetries = false; public DefaultWorkflowCommitWorkBatchService() { } public DefaultWorkflowCommitWorkBatchService(NameValueCollection parameters) { if (parameters == null) throw new ArgumentNullException("parameters", ExecutionStringManager.MissingParameters); if (parameters.Count > 0) { foreach (string key in parameters.Keys) { if (0 == string.Compare("EnableRetries", key, StringComparison.OrdinalIgnoreCase)) { _enableRetries = bool.Parse(parameters[key]); _ignoreCommonEnableRetries = true; } } } } public bool EnableRetries { get { return _enableRetries; } set { _enableRetries = value; _ignoreCommonEnableRetries = true; } } protected internal override void Start() { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "DefaultWorkflowCommitWorkBatchService: Starting"); // // If we didn't find a local value for enable retries // check in the common section if ((!_ignoreCommonEnableRetries)&&(null != base.Runtime)) { NameValueConfigurationCollection commonConfigurationParameters = base.Runtime.CommonParameters; if (commonConfigurationParameters != null) { // Then scan for connection string in the common configuration parameters section foreach (string key in commonConfigurationParameters.AllKeys) { if (string.Compare("EnableRetries", key, StringComparison.OrdinalIgnoreCase) == 0) { _enableRetries = bool.Parse(commonConfigurationParameters[key].Value); break; } } } } base.Start(); } protected override void OnStopped() { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "DefaultWorkflowCommitWorkBatchService: Stopping"); base.OnStopped(); } internal protected override void CommitWorkBatch(CommitWorkBatchCallback commitWorkBatchCallback) { DbRetry dbRetry = new DbRetry(_enableRetries); short retryCounter = 0; while (true) { if (null != Transaction.Current) { // // Can't retry as we don't own the tx // Set the counter to only allow one iteration retryCounter = dbRetry.MaxRetries; } try { base.CommitWorkBatch(commitWorkBatchCallback); break; } catch( Exception e ) { WorkflowTrace.Host.TraceEvent(TraceEventType.Error, 0, "DefaultWorkflowCommitWorkBatchService caught exception from commitWorkBatchCallback: " + e.ToString()); if (dbRetry.TryDoRetry(ref retryCounter)) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "DefaultWorkflowCommitWorkBatchService retrying commitWorkBatchCallback (retry attempt " + retryCounter.ToString(System.Globalization.CultureInfo.InvariantCulture) + ")"); continue; } else throw; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.System.Transactions.CommittableTransaction .
Link Menu
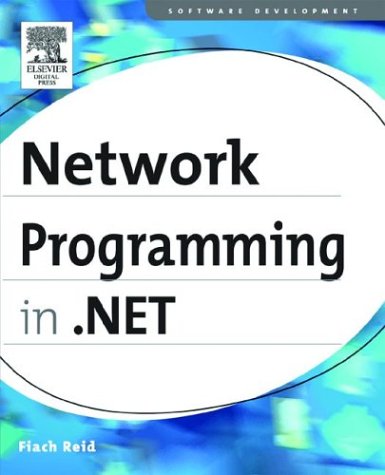
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlStreamStore.cs
- SoapObjectInfo.cs
- AnonymousIdentificationModule.cs
- latinshape.cs
- TdsParserStateObject.cs
- _LazyAsyncResult.cs
- DebugHandleTracker.cs
- WindowHelperService.cs
- InvalidCastException.cs
- HandlerFactoryCache.cs
- KeyConstraint.cs
- ImageSource.cs
- BaseUriHelper.cs
- SerialErrors.cs
- WebHttpElement.cs
- Native.cs
- NativeBuffer.cs
- LoginUtil.cs
- HttpCacheParams.cs
- ExtendedPropertyInfo.cs
- SamlAuthenticationClaimResource.cs
- ZipIOLocalFileDataDescriptor.cs
- ITextView.cs
- TakeOrSkipWhileQueryOperator.cs
- SessionStateContainer.cs
- _RequestLifetimeSetter.cs
- SqlUDTStorage.cs
- GeometryConverter.cs
- CompiledQueryCacheEntry.cs
- SecurityTokenValidationException.cs
- ListMarkerLine.cs
- RotateTransform3D.cs
- Crypto.cs
- RefreshEventArgs.cs
- Triplet.cs
- ManagedWndProcTracker.cs
- DbProviderManifest.cs
- FactoryRecord.cs
- ObjectItemAttributeAssemblyLoader.cs
- Matrix.cs
- RegexBoyerMoore.cs
- UnionCodeGroup.cs
- QueryCorrelationInitializer.cs
- Stroke.cs
- BitmapScalingModeValidation.cs
- X509SubjectKeyIdentifierClause.cs
- Membership.cs
- PointF.cs
- CollectionCodeDomSerializer.cs
- ChangePasswordAutoFormat.cs
- UrlMapping.cs
- HTTPNotFoundHandler.cs
- IBuiltInEvidence.cs
- StickyNote.cs
- TransactionContext.cs
- Dictionary.cs
- ThrowHelper.cs
- TextEffectCollection.cs
- IconBitmapDecoder.cs
- MethodAccessException.cs
- ControlParameter.cs
- ClientSideProviderDescription.cs
- FactoryGenerator.cs
- UIElementHelper.cs
- SortDescriptionCollection.cs
- ArraySortHelper.cs
- XmlCollation.cs
- WmlTextViewAdapter.cs
- ToolStripPanelCell.cs
- ListBox.cs
- RangeValidator.cs
- BStrWrapper.cs
- LifetimeServices.cs
- EnumValidator.cs
- MethodBuilderInstantiation.cs
- DataFormat.cs
- LocalizableAttribute.cs
- DrawingDrawingContext.cs
- BoundColumn.cs
- SessionSwitchEventArgs.cs
- Stack.cs
- XmlHelper.cs
- ApplicationDirectoryMembershipCondition.cs
- Utils.cs
- COM2ColorConverter.cs
- AccessKeyManager.cs
- RoleManagerSection.cs
- WpfGeneratedKnownTypes.cs
- SspiSafeHandles.cs
- ThaiBuddhistCalendar.cs
- Expr.cs
- ButtonStandardAdapter.cs
- FtpRequestCacheValidator.cs
- FlatButtonAppearance.cs
- CodeAttributeArgumentCollection.cs
- printdlgexmarshaler.cs
- SqlDataSourceEnumerator.cs
- HttpCacheParams.cs
- ClassicBorderDecorator.cs
- OdbcCommandBuilder.cs