Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / PtsHost / ListMarkerLine.cs / 1 / ListMarkerLine.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: LineBase.cs // // Description: Text line formatter. // // History: // 02/07/2005 : [....] - Split from Line.cs // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal.Text; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { internal class ListMarkerLine : LineBase { ////// Constructor /// /// /// TextFormatter host /// /// /// Owner of the ListMarker /// internal ListMarkerLine(TextFormatterHost host, ListParaClient paraClient) : base(paraClient) { _host = host; } // ----------------------------------------------------------------- // // TextSource Implementation // // ----------------------------------------------------------------- #region TextSource Implementation ////// Return the text run at specified text source position. /// /// /// Offset of specified position /// internal override TextRun GetTextRun(int dcp) { return new ParagraphBreakRun(1, PTS.FSFLRES.fsflrEndOfParagraph); } ////// Return the text, as CharacterBufferRange, immediately before specified text source position. /// /// /// Offset of specified position /// internal override TextSpanGetPrecedingText(int dcp) { return new TextSpan ( 0, new CultureSpecificCharacterBufferRange(null, CharacterBufferRange.Empty) ); } /// /// Get Text effect index from specified position /// /// /// Offset of specified position /// ///internal override int GetTextEffectCharacterIndexFromTextSourceCharacterIndex(int dcp) { return dcp; } #endregion TextSource Implementation /// /// Create and format text line. /// /// /// DrawingContext for text line. /// /// /// LineProperties of text line /// /// /// Horizontal draw location /// /// /// Vertical baseline draw location /// internal void FormatAndDrawVisual(DrawingContext ctx, LineProperties lineProps, int ur, int vrBaseline) { System.Windows.Media.TextFormatting.TextLine line; bool mirror = (lineProps.FlowDirection == FlowDirection.RightToLeft); _host.Context = this; try { // Create line object line = _host.TextFormatter.FormatLine(_host, 0, 0, lineProps.FirstLineProps, null, new TextRunCache()); Point drawLocation = new Point(TextDpi.FromTextDpi(ur), TextDpi.FromTextDpi(vrBaseline) - line.Baseline); line.Draw(ctx, drawLocation, (mirror ? InvertAxes.Horizontal : InvertAxes.None)); line.Dispose(); } finally { // clear the context _host.Context = null; } } ////// Text formatter host /// private readonly TextFormatterHost _host; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
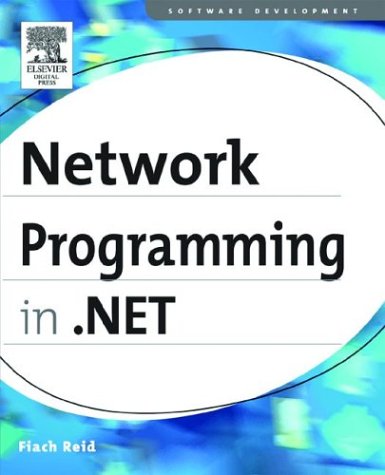
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlXml.cs
- URLString.cs
- XmlHierarchicalEnumerable.cs
- ExceptionHandlers.cs
- LoopExpression.cs
- AttributeQuery.cs
- Operand.cs
- FontFamily.cs
- UnmanagedMemoryStreamWrapper.cs
- IPeerNeighbor.cs
- DispatcherEventArgs.cs
- RegisteredDisposeScript.cs
- ListBoxChrome.cs
- JoinTreeSlot.cs
- PeerNameRecord.cs
- X509InitiatorCertificateServiceElement.cs
- GradientSpreadMethodValidation.cs
- ServiceX509SecurityTokenProvider.cs
- AssociationTypeEmitter.cs
- ImportFileRequest.cs
- SerializationAttributes.cs
- VerticalAlignConverter.cs
- ActivationArguments.cs
- ExpandSegment.cs
- SevenBitStream.cs
- SymmetricKeyWrap.cs
- HtmlElement.cs
- Vector3D.cs
- ReachUIElementCollectionSerializerAsync.cs
- StackSpiller.Temps.cs
- DropDownHolder.cs
- QilFactory.cs
- ByteStreamGeometryContext.cs
- MimeTypeAttribute.cs
- FlowDocument.cs
- BitArray.cs
- ToolStripSplitButton.cs
- XmlSchemaCompilationSettings.cs
- ExpressionNormalizer.cs
- StructureChangedEventArgs.cs
- MenuItemBindingCollection.cs
- ServiceReference.cs
- ArithmeticException.cs
- SharedDp.cs
- HttpRequestCacheValidator.cs
- HostProtectionException.cs
- ScriptReferenceBase.cs
- ObjectConverter.cs
- contentDescriptor.cs
- QueuePropertyVariants.cs
- IisTraceWebEventProvider.cs
- VScrollProperties.cs
- DataGridTextBox.cs
- MarshalByValueComponent.cs
- CodeEventReferenceExpression.cs
- OrderByExpression.cs
- EventHandlerList.cs
- ToolStripItemEventArgs.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- OneOfScalarConst.cs
- _DigestClient.cs
- DataGridViewLinkCell.cs
- DoubleLinkListEnumerator.cs
- SerialReceived.cs
- ConfigXmlWhitespace.cs
- AssemblyInfo.cs
- Transform3DGroup.cs
- ImageAnimator.cs
- DataTableReader.cs
- PromptEventArgs.cs
- PropertyFilter.cs
- CodeCompileUnit.cs
- UnsafeMethods.cs
- SectionXmlInfo.cs
- BamlRecords.cs
- LinkConverter.cs
- SynchronizedPool.cs
- EffectiveValueEntry.cs
- PropertyChangedEventArgs.cs
- GlobalEventManager.cs
- TrackingLocation.cs
- XmlElementAttribute.cs
- CombinedGeometry.cs
- CommonGetThemePartSize.cs
- EncoderBestFitFallback.cs
- CustomErrorCollection.cs
- ComboBox.cs
- LocalizationParserHooks.cs
- OdbcConnectionPoolProviderInfo.cs
- NativeWindow.cs
- RootAction.cs
- XamlTypeMapper.cs
- CodeCompiler.cs
- EncryptRequest.cs
- DiscoveryInnerClientAdhoc11.cs
- XmlSerializationWriter.cs
- EFTableProvider.cs
- IxmlLineInfo.cs
- StyleCollectionEditor.cs
- ValidationErrorEventArgs.cs