Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / MarshalByValueComponent.cs / 1305376 / MarshalByValueComponent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel.Design; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; using System.Runtime.InteropServices; ////// [ ComVisible(true), Designer("System.Windows.Forms.Design.ComponentDocumentDesigner, " + AssemblyRef.SystemDesign, typeof(IRootDesigner)), DesignerCategory("Component"), TypeConverter(typeof(ComponentConverter)) ] public class MarshalByValueComponent : IComponent, IServiceProvider { ///Provides the base implementation for ///, /// which is the base class for all components in Win Forms. /// private static readonly object EventDisposed = new object(); private ISite site; private EventHandlerList events; ///Static hask key for the Disposed event. This field is read-only. ////// public MarshalByValueComponent() { } ~MarshalByValueComponent() { Dispose(false); } ///Initializes a new instance of the ///class. /// public event EventHandler Disposed { add { Events.AddHandler(EventDisposed, value); } remove { Events.RemoveHandler(EventDisposed, value); } } ///Adds a event handler to listen to the Disposed event on the component. ////// protected EventHandlerList Events { get { if (events == null) { events = new EventHandlerList(); } return events; } } ///Gets the list of event handlers that are attached to this component. ////// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual ISite Site { get { return site;} set { site = value;} } ///Gets or sets the site of the component. ////// [SuppressMessage("Microsoft.Usage", "CA2213:DisposableFieldsShouldBeDisposed")] public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///Disposes of the resources (other than memory) used by the component. ////// protected virtual void Dispose(bool disposing) { if (disposing) { lock(this) { if (site != null && site.Container != null) { site.Container.Remove(this); } if (events != null) { EventHandler handler = (EventHandler)events[EventDisposed]; if (handler != null) handler(this, EventArgs.Empty); } } } } ////// Disposes all the resources associated with this component. /// If disposing is false then you must never touch any other /// managed objects, as they may already be finalized. When /// in this state you should dispose any native resources /// that you have a reference to. /// ////// When disposing is true then you should dispose all data /// and objects you have references to. The normal implementation /// of this method would look something like: /// ////// public void Dispose() { /// Dispose(true); /// GC.SuppressFinalize(this); /// } /// /// protected virtual void Dispose(bool disposing) { /// if (disposing) { /// if (myobject != null) { /// myobject.Dispose(); /// myobject = null; /// } /// } /// if (myhandle != IntPtr.Zero) { /// NativeMethods.Release(myhandle); /// myhandle = IntPtr.Zero; /// } /// } /// /// ~MyClass() { /// Dispose(false); /// } ///
////// For base classes, you should never override the Finalier (~Class in C#) /// or the Dispose method that takes no arguments, rather you should /// always override the Dispose method that takes a bool. /// ////// protected override void Dispose(bool disposing) { /// if (disposing) { /// if (myobject != null) { /// myobject.Dispose(); /// myobject = null; /// } /// } /// if (myhandle != IntPtr.Zero) { /// NativeMethods.Release(myhandle); /// myhandle = IntPtr.Zero; /// } /// base.Dispose(disposing); /// } ///
////// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual IContainer Container { get { ISite s = site; return s == null ? null : s.Container; } } ///Gets the container for the component. ////// public virtual object GetService(Type service) { return((site==null)? null : site.GetService(service)); } ///Gets the implementer of the ///. /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual bool DesignMode { get { ISite s = site; return(s == null) ? false : s.DesignMode; } } ///Gets a value indicating whether the component is currently in design mode. ////// /// public override String ToString() { ISite s = site; if (s != null) return s.Name + " [" + GetType().FullName + "]"; else return GetType().FullName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns a ///containing the name of the , if any. This method should not be /// overridden. For /// internal use only. ///
Link Menu
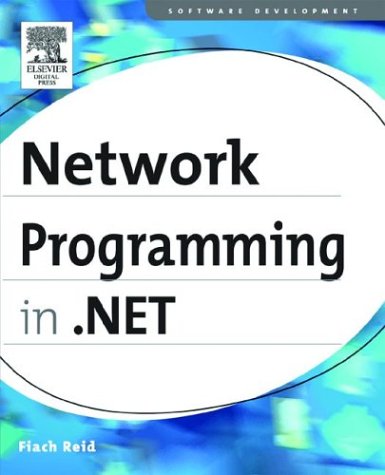
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegionData.cs
- SelectorAutomationPeer.cs
- HttpPostedFileBase.cs
- NativeMethods.cs
- ScrollViewerAutomationPeer.cs
- CodeSnippetStatement.cs
- DataColumnMappingCollection.cs
- ContainsSearchOperator.cs
- WriterOutput.cs
- AutoResizedEvent.cs
- TrackingCondition.cs
- FileDialogCustomPlacesCollection.cs
- DataTransferEventArgs.cs
- CodeTypeParameter.cs
- _NativeSSPI.cs
- ObjectStorage.cs
- VectorAnimationBase.cs
- DataServiceQueryException.cs
- ChannelReliableSession.cs
- ColumnPropertiesGroup.cs
- UnsafeNativeMethodsCLR.cs
- TCEAdapterGenerator.cs
- EnvironmentPermission.cs
- AnonymousIdentificationSection.cs
- CqlLexer.cs
- ObjectViewEntityCollectionData.cs
- XmlSchemaAppInfo.cs
- JpegBitmapDecoder.cs
- GatewayIPAddressInformationCollection.cs
- FixedPageStructure.cs
- RawStylusSystemGestureInputReport.cs
- ApplicationDirectoryMembershipCondition.cs
- QueryCacheKey.cs
- ManualResetEvent.cs
- TabControlDesigner.cs
- PrtCap_Public_Simple.cs
- SingleQueryOperator.cs
- ExtensibleClassFactory.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- ReferenceEqualityComparer.cs
- ObjectCacheHost.cs
- RelatedEnd.cs
- LoginUtil.cs
- GroupByQueryOperator.cs
- HttpCapabilitiesEvaluator.cs
- ExpressionNormalizer.cs
- LocalizableAttribute.cs
- DataObjectPastingEventArgs.cs
- XmlSerializerFactory.cs
- DataErrorValidationRule.cs
- GeneratedContractType.cs
- StrongNameHelpers.cs
- AttributeQuery.cs
- AssemblyBuilderData.cs
- FontStretchConverter.cs
- WindowsScrollBarBits.cs
- WindowInteropHelper.cs
- LinqTreeNodeEvaluator.cs
- DnsPermission.cs
- ToolStripCustomTypeDescriptor.cs
- DataGridViewCellStyle.cs
- safemediahandle.cs
- ListGeneralPage.cs
- TypeContext.cs
- CompModSwitches.cs
- StringArrayConverter.cs
- FrugalList.cs
- XPathEmptyIterator.cs
- DescendantOverDescendantQuery.cs
- TypedAsyncResult.cs
- AsymmetricAlgorithm.cs
- _ContextAwareResult.cs
- ParameterReplacerVisitor.cs
- Point3DCollectionConverter.cs
- ReadOnlyState.cs
- PassportIdentity.cs
- DataProtection.cs
- DataGridViewRowsRemovedEventArgs.cs
- IisTraceListener.cs
- Bookmark.cs
- Transform.cs
- RefExpr.cs
- PageMediaSize.cs
- XmlSchemaDocumentation.cs
- MouseActionValueSerializer.cs
- HandlerBase.cs
- EventLogConfiguration.cs
- MimeXmlReflector.cs
- DataSourceControlBuilder.cs
- EndEvent.cs
- X509ThumbprintKeyIdentifierClause.cs
- Accessible.cs
- BaseComponentEditor.cs
- DurableInstancingOptions.cs
- XsdValidatingReader.cs
- FixedPageAutomationPeer.cs
- MarkupCompilePass1.cs
- RelatedImageListAttribute.cs
- _Win32.cs
- DBParameter.cs