Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ToolStripCustomTypeDescriptor.cs / 1 / ToolStripCustomTypeDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Windows.Forms; ////// ToolStripCustomTypeDescriptor class. /// internal class ToolStripCustomTypeDescriptor : CustomTypeDescriptor { ToolStrip instance = null; PropertyDescriptor propItems = null; PropertyDescriptorCollection collection = null; public ToolStripCustomTypeDescriptor(ToolStrip instance) : base() { this.instance = instance; } ////// /// The GetPropertyOwner method returns an instance of an object that /// owns the given property for the object this type descriptor is representing. /// An optional attribute array may be provided to filter the collection that is /// returned. Returning null from this method causes the TypeDescriptor object /// to use its default type description services. /// public override object GetPropertyOwner(PropertyDescriptor pd) { return instance; } ////// /// The GetProperties method returns a collection of property descriptors /// for the object this type descriptor is representing. An optional /// attribute array may be provided to filter the collection that is returned. /// If no parent is provided,this will return an empty /// property collection. /// public override PropertyDescriptorCollection GetProperties() { if (instance!= null && collection == null) { PropertyDescriptorCollection retColl = TypeDescriptor.GetProperties(instance); PropertyDescriptor[] propArray = new PropertyDescriptor[retColl.Count]; retColl.CopyTo(propArray, 0); collection = new PropertyDescriptorCollection(propArray, false); } if (collection.Count > 0) { propItems = collection["Items"]; if (propItems != null) { collection.Remove(propItems); } } return collection; } ////// /// The GetProperties method returns a collection of property descriptors /// for the object this type descriptor is representing. An optional /// attribute array may be provided to filter the collection that is returned. /// If no parent is provided,this will return an empty /// property collection. /// Here we will pass the "collection without the "items" property. /// public override PropertyDescriptorCollection GetProperties(Attribute[] attributes) { if (instance!= null && collection == null) { PropertyDescriptorCollection retColl = TypeDescriptor.GetProperties(instance); PropertyDescriptor[] propArray = new PropertyDescriptor[retColl.Count]; retColl.CopyTo(propArray, 0); collection = new PropertyDescriptorCollection(propArray, false); } if (collection.Count > 0) { propItems = collection["Items"]; if (propItems != null) { collection.Remove(propItems); } } return collection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
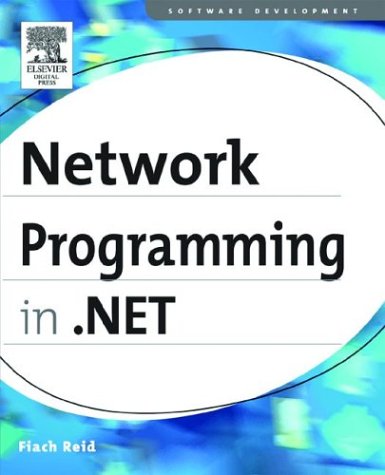
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Array.cs
- RawKeyboardInputReport.cs
- UriTemplateEquivalenceComparer.cs
- ActivityInstanceMap.cs
- ArgumentValueSerializer.cs
- ListView.cs
- BamlReader.cs
- localization.cs
- SqlClientFactory.cs
- _ListenerAsyncResult.cs
- cryptoapiTransform.cs
- EventSetter.cs
- MgmtConfigurationRecord.cs
- ExtractorMetadata.cs
- UrlMapping.cs
- WebEventTraceProvider.cs
- Package.cs
- WizardStepCollectionEditor.cs
- XmlUrlResolver.cs
- OptimalTextSource.cs
- StylusPoint.cs
- WebServiceEnumData.cs
- DataQuery.cs
- EtwTrace.cs
- SequentialUshortCollection.cs
- CompositeFontInfo.cs
- FunctionDescription.cs
- DecoratedNameAttribute.cs
- DataControlHelper.cs
- ImageCodecInfo.cs
- NativeMethods.cs
- PageCodeDomTreeGenerator.cs
- ManagedFilter.cs
- TextSearch.cs
- MouseWheelEventArgs.cs
- SplayTreeNode.cs
- RegexMatchCollection.cs
- LocationSectionRecord.cs
- ToolStripSplitButton.cs
- MediaTimeline.cs
- MergablePropertyAttribute.cs
- XmlObjectSerializerReadContextComplexJson.cs
- ObjectViewQueryResultData.cs
- XmlReaderDelegator.cs
- ArrayExtension.cs
- Lease.cs
- TakeQueryOptionExpression.cs
- SmiConnection.cs
- TdsParserSessionPool.cs
- ProcessStartInfo.cs
- NamespaceList.cs
- WebBrowserUriTypeConverter.cs
- Span.cs
- HebrewCalendar.cs
- PropertyReferenceExtension.cs
- RowParagraph.cs
- TextEditorSelection.cs
- MenuScrollingVisibilityConverter.cs
- SequenceNumber.cs
- XmlImplementation.cs
- BamlResourceContent.cs
- ToolstripProfessionalRenderer.cs
- StringUtil.cs
- TableProvider.cs
- HuffModule.cs
- LinqDataSourceView.cs
- RuleInfoComparer.cs
- WasHttpModulesInstallComponent.cs
- UserControl.cs
- RoleManagerSection.cs
- CodeEventReferenceExpression.cs
- OleDbParameter.cs
- RenderDataDrawingContext.cs
- PrinterUnitConvert.cs
- MouseButton.cs
- StagingAreaInputItem.cs
- UnsafeNativeMethods.cs
- KeyConstraint.cs
- DbConnectionPoolGroup.cs
- FilterFactory.cs
- EmptyStringExpandableObjectConverter.cs
- SafeRegistryHandle.cs
- FixedSOMLineCollection.cs
- NamedObjectList.cs
- ProcessHostConfigUtils.cs
- MapPathBasedVirtualPathProvider.cs
- ScrollBarAutomationPeer.cs
- ToolStripDropDownClosingEventArgs.cs
- DbMetaDataCollectionNames.cs
- PreservationFileReader.cs
- EditorPartChrome.cs
- CheckBoxStandardAdapter.cs
- AddInContractAttribute.cs
- ConsumerConnectionPoint.cs
- ObjectTag.cs
- DataServiceQueryOfT.cs
- MdiWindowListItemConverter.cs
- OleDbError.cs
- PropertyBuilder.cs
- CorrelationResolver.cs