Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Globalization / BamlResourceContent.cs / 1 / BamlResourceContent.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Utility that handles parsing Baml Resource Content // // History: 11/29/2003 garyyang Rewrote // //--------------------------------------------------------------------------- using System; using System.Text; using System.Text.RegularExpressions; using System.Collections; using System.Diagnostics; using System.Collections.Generic; using System.Windows; namespace MS.Internal.Globalization { internal static class BamlResourceContentUtil { //------------------------------------- // Internal methods //------------------------------------- ////// Escape a string /// internal static string EscapeString(string content) { if (content == null) return null; StringBuilder builder = new StringBuilder(); for (int i = 0; i < content.Length; i++) { switch (content[i]) { case BamlConst.ChildStart : case BamlConst.ChildEnd : case BamlConst.EscapeChar : { builder.Append(BamlConst.EscapeChar); builder.Append(content[i]); break; } case '&' : { builder.Append("&"); break; } case '<' : { builder.Append("<"); break; } case '>' : { builder.Append(">"); break; } case '\'': { builder.Append("'"); break; } case '\"': { builder.Append("""); break; } default : { builder.Append(content[i]); break; } } } return builder.ToString(); } ////// Unescape a string. Note: /// Backslash following any character will become that character. /// Backslash by itself will be skipped. /// internal static string UnescapeString(string content) { return UnescapePattern.Replace( content, UnescapeMatchEvaluator ); } // Regular expression // need to use 4 backslash here because it is escaped by compiler and regular expressions private static Regex UnescapePattern = new Regex("(\\\\.?|<|>|"|'|&)", RegexOptions.CultureInvariant | RegexOptions.Compiled); // delegates to escape and unesacpe a matched pattern private static MatchEvaluator UnescapeMatchEvaluator = new MatchEvaluator(UnescapeMatch); ////// the delegate to Unescape the matched pattern /// private static string UnescapeMatch(Match match) { switch (match.Value) { case "<" : return "<"; case ">" : return ">"; case "&" : return "&"; case "'": return "'"; case """: return "\""; default: { // this is a '\' followed by 0 or 1 character Debug.Assert(match.Value.Length > 0 && match.Value[0] == BamlConst.EscapeChar); if (match.Value.Length == 2) { return match.Value[1].ToString(); } else { return string.Empty; } } } } ////// Parse the input string into an array of text/child-placeholder tokens. /// Element placeholders start with '#' and end with ';'. /// In case of error, a null array is returned. /// internal static BamlStringToken[] ParseChildPlaceholder(string input) { if (input == null) return null; Listtokens = new List (8); int tokenStart = 0; bool inPlaceHolder = false; for (int i = 0; i < input.Length; i++) { if (input[i] == BamlConst.ChildStart) { if (i == 0 || input[i - 1]!= BamlConst.EscapeChar) { if (inPlaceHolder) { // All # needs to be escaped in a child place holder return null; // error } inPlaceHolder = true; if (tokenStart < i) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart, i - tokenStart)) ) ); tokenStart = i; } } } else if (input[i] == BamlConst.ChildEnd) { if ( i > 0 && input[i - 1] != BamlConst.EscapeChar && inPlaceHolder) { // It is a valid child placeholder end tokens.Add( new BamlStringToken( BamlStringToken.TokenType.ChildPlaceHolder, UnescapeString(input.Substring(tokenStart + 1, i - tokenStart - 1)) ) ); // Advance the token index tokenStart = i + 1; inPlaceHolder = false; } } } if (inPlaceHolder) { // at the end of the string, all child placeholder must be closed return null; // error } if (tokenStart < input.Length) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart)) ) ); } return tokens.ToArray(); } } internal struct BamlStringToken { internal readonly TokenType Type; internal readonly string Value; internal BamlStringToken(TokenType type, string value) { Type = type; Value = value; } internal enum TokenType { Text, ChildPlaceHolder, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Utility that handles parsing Baml Resource Content // // History: 11/29/2003 garyyang Rewrote // //--------------------------------------------------------------------------- using System; using System.Text; using System.Text.RegularExpressions; using System.Collections; using System.Diagnostics; using System.Collections.Generic; using System.Windows; namespace MS.Internal.Globalization { internal static class BamlResourceContentUtil { //------------------------------------- // Internal methods //------------------------------------- ////// Escape a string /// internal static string EscapeString(string content) { if (content == null) return null; StringBuilder builder = new StringBuilder(); for (int i = 0; i < content.Length; i++) { switch (content[i]) { case BamlConst.ChildStart : case BamlConst.ChildEnd : case BamlConst.EscapeChar : { builder.Append(BamlConst.EscapeChar); builder.Append(content[i]); break; } case '&' : { builder.Append("&"); break; } case '<' : { builder.Append("<"); break; } case '>' : { builder.Append(">"); break; } case '\'': { builder.Append("'"); break; } case '\"': { builder.Append("""); break; } default : { builder.Append(content[i]); break; } } } return builder.ToString(); } ////// Unescape a string. Note: /// Backslash following any character will become that character. /// Backslash by itself will be skipped. /// internal static string UnescapeString(string content) { return UnescapePattern.Replace( content, UnescapeMatchEvaluator ); } // Regular expression // need to use 4 backslash here because it is escaped by compiler and regular expressions private static Regex UnescapePattern = new Regex("(\\\\.?|<|>|"|'|&)", RegexOptions.CultureInvariant | RegexOptions.Compiled); // delegates to escape and unesacpe a matched pattern private static MatchEvaluator UnescapeMatchEvaluator = new MatchEvaluator(UnescapeMatch); ////// the delegate to Unescape the matched pattern /// private static string UnescapeMatch(Match match) { switch (match.Value) { case "<" : return "<"; case ">" : return ">"; case "&" : return "&"; case "'": return "'"; case """: return "\""; default: { // this is a '\' followed by 0 or 1 character Debug.Assert(match.Value.Length > 0 && match.Value[0] == BamlConst.EscapeChar); if (match.Value.Length == 2) { return match.Value[1].ToString(); } else { return string.Empty; } } } } ////// Parse the input string into an array of text/child-placeholder tokens. /// Element placeholders start with '#' and end with ';'. /// In case of error, a null array is returned. /// internal static BamlStringToken[] ParseChildPlaceholder(string input) { if (input == null) return null; Listtokens = new List (8); int tokenStart = 0; bool inPlaceHolder = false; for (int i = 0; i < input.Length; i++) { if (input[i] == BamlConst.ChildStart) { if (i == 0 || input[i - 1]!= BamlConst.EscapeChar) { if (inPlaceHolder) { // All # needs to be escaped in a child place holder return null; // error } inPlaceHolder = true; if (tokenStart < i) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart, i - tokenStart)) ) ); tokenStart = i; } } } else if (input[i] == BamlConst.ChildEnd) { if ( i > 0 && input[i - 1] != BamlConst.EscapeChar && inPlaceHolder) { // It is a valid child placeholder end tokens.Add( new BamlStringToken( BamlStringToken.TokenType.ChildPlaceHolder, UnescapeString(input.Substring(tokenStart + 1, i - tokenStart - 1)) ) ); // Advance the token index tokenStart = i + 1; inPlaceHolder = false; } } } if (inPlaceHolder) { // at the end of the string, all child placeholder must be closed return null; // error } if (tokenStart < input.Length) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart)) ) ); } return tokens.ToArray(); } } internal struct BamlStringToken { internal readonly TokenType Type; internal readonly string Value; internal BamlStringToken(TokenType type, string value) { Type = type; Value = value; } internal enum TokenType { Text, ChildPlaceHolder, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
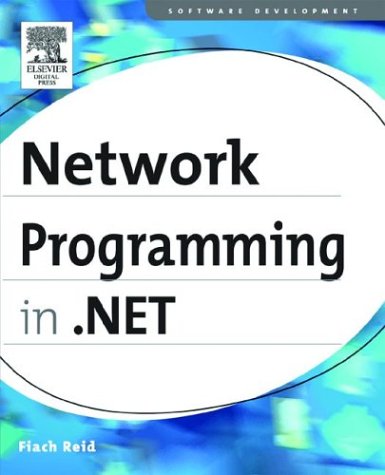
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SplayTreeNode.cs
- RotationValidation.cs
- CellConstantDomain.cs
- EntityDataSourceChangingEventArgs.cs
- QueryCacheEntry.cs
- XsltContext.cs
- FastPropertyAccessor.cs
- MergeLocalizationDirectives.cs
- ConfigXmlSignificantWhitespace.cs
- DataServiceRequestArgs.cs
- SymbolUsageManager.cs
- HMACSHA512.cs
- ImpersonationContext.cs
- ActivityMetadata.cs
- DbConnectionPoolIdentity.cs
- PasswordTextNavigator.cs
- SmtpLoginAuthenticationModule.cs
- GridViewSelectEventArgs.cs
- ReadOnlyHierarchicalDataSource.cs
- StrongNamePublicKeyBlob.cs
- SequenceDesigner.cs
- HostVisual.cs
- XmlDictionary.cs
- PopOutPanel.cs
- StrokeDescriptor.cs
- Timer.cs
- RenderDataDrawingContext.cs
- ObjectDataSourceView.cs
- LineServices.cs
- DbProviderServices.cs
- CreateUserWizardAutoFormat.cs
- Roles.cs
- Int64AnimationBase.cs
- CacheManager.cs
- Walker.cs
- XmlUrlResolver.cs
- XmlAttributes.cs
- QilName.cs
- SqlDataSourceWizardForm.cs
- ServicesUtilities.cs
- EntityDataSourceSelectingEventArgs.cs
- _NetRes.cs
- RtfToXamlLexer.cs
- Relationship.cs
- PresentationTraceSources.cs
- ModifierKeysConverter.cs
- ConfigurationUtility.cs
- DataListItemCollection.cs
- QilDataSource.cs
- AutomationPeer.cs
- VisualStyleTypesAndProperties.cs
- WebBrowser.cs
- EventProxy.cs
- BlurBitmapEffect.cs
- XsltOutput.cs
- FormViewModeEventArgs.cs
- ExceptionValidationRule.cs
- GlyphTypeface.cs
- GenericAuthenticationEventArgs.cs
- EntityContainerEmitter.cs
- Switch.cs
- DateTimePickerDesigner.cs
- EditorPartCollection.cs
- columnmapkeybuilder.cs
- ReachVisualSerializer.cs
- TrackingParameters.cs
- ActivityTypeResolver.xaml.cs
- EmptyEnumerator.cs
- NavigateEvent.cs
- TextServicesDisplayAttribute.cs
- RijndaelManaged.cs
- ImageDesigner.cs
- assertwrapper.cs
- CompositeCollectionView.cs
- IDictionary.cs
- DebugView.cs
- HybridDictionary.cs
- XmlSchemaSimpleTypeList.cs
- Perspective.cs
- UnconditionalPolicy.cs
- Rotation3DKeyFrameCollection.cs
- ISFClipboardData.cs
- BindableAttribute.cs
- PageStatePersister.cs
- DataTableTypeConverter.cs
- BaseWebProxyFinder.cs
- SqlCommandBuilder.cs
- Pen.cs
- Vector3DValueSerializer.cs
- FrameAutomationPeer.cs
- GroupStyle.cs
- NavigationExpr.cs
- EmptyControlCollection.cs
- SizeAnimationUsingKeyFrames.cs
- ToolStripGrip.cs
- HostExecutionContextManager.cs
- MonitoringDescriptionAttribute.cs
- FileDataSourceCache.cs
- SystemThemeKey.cs
- WmlPhoneCallAdapter.cs