Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / propertytag.cs / 1 / propertytag.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.Internal.SrgsParser; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal.SrgsCompiler { ////// Summary description for Rule. /// internal sealed class PropertyTag : ParseElement, IPropertyTag { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal PropertyTag (ParseElement parent, Backend backend) : base (parent._rule) { } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods #pragma warning disable 56507 /// TODOC <_include file='doc\Tag.uex' path='docs/doc[@for="Tag.RepeatProbability"]/*' /> // The probability that this item will be repeated. void IPropertyTag.NameValue (IElement parent, string name, object value) { //Return if the Tag content is empty string sValue = value as string; if (string.IsNullOrEmpty (name) && (value == null || (sValue != null && string.IsNullOrEmpty ((sValue).Trim ())))) { return; } // Build semantic properties to attach to epsilon transition. //Name= pszValue = null vValue = VT_EMPTY //Name="string" pszValue = "string" vValue = VT_EMPTY //Name=true pszValue = null vValue = VT_BOOL //Name=123 pszValue = null vValue = VT_I4 //Name=3.14 pszValue = null vValue = VT_R8 if (!string.IsNullOrEmpty (name)) { // Set property name _propInfo._pszName = name; } else { // If no property, set the name to the anonymous property name _propInfo._pszName = "="; } // Set property value _propInfo._comValue = value; if (value == null) { _propInfo._comType = VarEnum.VT_EMPTY; } else if (sValue != null) { _propInfo._comType = VarEnum.VT_EMPTY; } else if (value is int) { _propInfo._comType = VarEnum.VT_I4; } else if (value is double) { _propInfo._comType = VarEnum.VT_R8; } else if (value is bool) { _propInfo._comType = VarEnum.VT_BOOL; } else { // should never get here System.Diagnostics.Debug.Assert (false); } } void IElement.PostParse (IElement parentElement) { ParseElementCollection parent = (ParseElementCollection) parentElement; _propInfo._ulId = (uint) parent._rule._iSerialize2; // Attach the semantic properties on the parent element. parent.AddSementicPropertyTag (_propInfo); } #pragma warning restore 56507 #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private CfgGrammar.CfgProperty _propInfo = new CfgGrammar.CfgProperty (); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.Internal.SrgsParser; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal.SrgsCompiler { ////// Summary description for Rule. /// internal sealed class PropertyTag : ParseElement, IPropertyTag { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal PropertyTag (ParseElement parent, Backend backend) : base (parent._rule) { } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods #pragma warning disable 56507 /// TODOC <_include file='doc\Tag.uex' path='docs/doc[@for="Tag.RepeatProbability"]/*' /> // The probability that this item will be repeated. void IPropertyTag.NameValue (IElement parent, string name, object value) { //Return if the Tag content is empty string sValue = value as string; if (string.IsNullOrEmpty (name) && (value == null || (sValue != null && string.IsNullOrEmpty ((sValue).Trim ())))) { return; } // Build semantic properties to attach to epsilon transition. //Name= pszValue = null vValue = VT_EMPTY //Name="string" pszValue = "string" vValue = VT_EMPTY //Name=true pszValue = null vValue = VT_BOOL //Name=123 pszValue = null vValue = VT_I4 //Name=3.14 pszValue = null vValue = VT_R8 if (!string.IsNullOrEmpty (name)) { // Set property name _propInfo._pszName = name; } else { // If no property, set the name to the anonymous property name _propInfo._pszName = "="; } // Set property value _propInfo._comValue = value; if (value == null) { _propInfo._comType = VarEnum.VT_EMPTY; } else if (sValue != null) { _propInfo._comType = VarEnum.VT_EMPTY; } else if (value is int) { _propInfo._comType = VarEnum.VT_I4; } else if (value is double) { _propInfo._comType = VarEnum.VT_R8; } else if (value is bool) { _propInfo._comType = VarEnum.VT_BOOL; } else { // should never get here System.Diagnostics.Debug.Assert (false); } } void IElement.PostParse (IElement parentElement) { ParseElementCollection parent = (ParseElementCollection) parentElement; _propInfo._ulId = (uint) parent._rule._iSerialize2; // Attach the semantic properties on the parent element. parent.AddSementicPropertyTag (_propInfo); } #pragma warning restore 56507 #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private CfgGrammar.CfgProperty _propInfo = new CfgGrammar.CfgProperty (); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
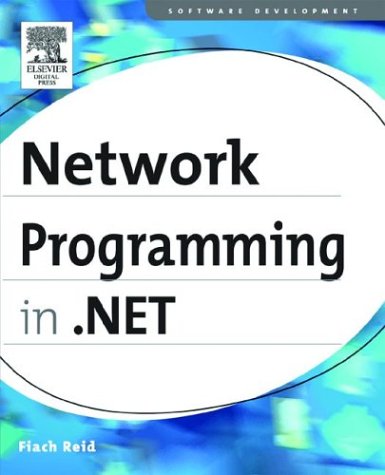
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _NegoState.cs
- XmlAtomicValue.cs
- ToolZoneDesigner.cs
- FontFamilyValueSerializer.cs
- SiteMapSection.cs
- HttpServerVarsCollection.cs
- AssemblyNameUtility.cs
- OracleTimeSpan.cs
- _UncName.cs
- MappedMetaModel.cs
- DocumentXPathNavigator.cs
- Model3DGroup.cs
- TextServicesDisplayAttributePropertyRanges.cs
- TripleDES.cs
- KeyValueConfigurationCollection.cs
- Wizard.cs
- LinkLabel.cs
- WSFederationHttpBindingElement.cs
- ModelUIElement3D.cs
- Pool.cs
- EntityParameterCollection.cs
- XmlReaderSettings.cs
- AnimationStorage.cs
- XmlMapping.cs
- DataGridViewColumnConverter.cs
- MobileFormsAuthentication.cs
- WebServiceReceive.cs
- ManualResetEvent.cs
- ClonableStack.cs
- FileStream.cs
- RuntimeConfig.cs
- WorkflowDesignerColors.cs
- SyndicationFeed.cs
- BrowserDefinitionCollection.cs
- ScriptResourceAttribute.cs
- ProjectionCamera.cs
- ExpressionCopier.cs
- DoubleCollection.cs
- UnsettableComboBox.cs
- Pair.cs
- BindingContext.cs
- DeploymentSectionCache.cs
- ColorConverter.cs
- WindowsNonControl.cs
- Image.cs
- NotSupportedException.cs
- XmlEntityReference.cs
- SplayTreeNode.cs
- C14NUtil.cs
- TextRange.cs
- StatusStrip.cs
- LightweightCodeGenerator.cs
- Propagator.JoinPropagator.cs
- StylusTip.cs
- PageContentCollection.cs
- ArgIterator.cs
- RowBinding.cs
- IxmlLineInfo.cs
- IntellisenseTextBox.designer.cs
- CodeRegionDirective.cs
- TargetException.cs
- HyperlinkAutomationPeer.cs
- BaseValidator.cs
- UnknownBitmapDecoder.cs
- BlurBitmapEffect.cs
- FileSecurity.cs
- UnsafeNativeMethodsMilCoreApi.cs
- AssemblyAssociatedContentFileAttribute.cs
- CompilerLocalReference.cs
- SevenBitStream.cs
- Evidence.cs
- ReachDocumentReferenceCollectionSerializer.cs
- XmlAnyAttributeAttribute.cs
- InternalPermissions.cs
- XmlnsDictionary.cs
- BitmapImage.cs
- ImageBrush.cs
- _HeaderInfo.cs
- DrawingState.cs
- SqlDataReader.cs
- Win32.cs
- XamlBrushSerializer.cs
- securestring.cs
- Enum.cs
- WebPartConnectionsConnectVerb.cs
- InputScope.cs
- RectKeyFrameCollection.cs
- ResourceManagerWrapper.cs
- PartManifestEntry.cs
- BaseParser.cs
- MemberRestriction.cs
- XmlLangPropertyAttribute.cs
- SBCSCodePageEncoding.cs
- cookie.cs
- ProcessHostConfigUtils.cs
- WebPartTracker.cs
- InheritedPropertyDescriptor.cs
- Wildcard.cs
- FocusWithinProperty.cs
- DataService.cs