Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Query / ResultAssembly / BitVec.cs / 1 / BitVec.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
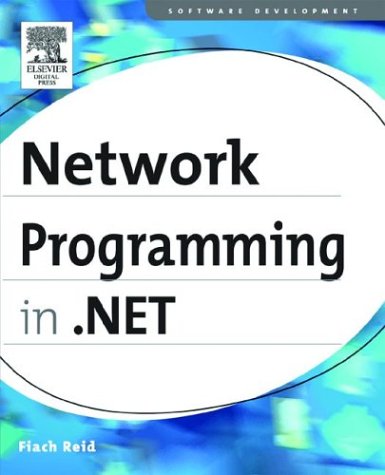
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeriveBytes.cs
- UpnEndpointIdentityExtension.cs
- SymbolType.cs
- ItemsControlAutomationPeer.cs
- ChineseLunisolarCalendar.cs
- GridViewUpdateEventArgs.cs
- MaterializeFromAtom.cs
- MappingModelBuildProvider.cs
- ListParaClient.cs
- FlowDocumentPageViewerAutomationPeer.cs
- precedingsibling.cs
- TokenBasedSetEnumerator.cs
- XsdBuilder.cs
- InvokeAction.cs
- CmsInterop.cs
- IndexerNameAttribute.cs
- XmlElementCollection.cs
- Tuple.cs
- VideoDrawing.cs
- SBCSCodePageEncoding.cs
- Camera.cs
- ButtonFieldBase.cs
- PathFigureCollectionConverter.cs
- DataSetUtil.cs
- FieldNameLookup.cs
- FormatControl.cs
- WebBrowserNavigatedEventHandler.cs
- ReferenceEqualityComparer.cs
- KeyEventArgs.cs
- WmiEventSink.cs
- XPathDocumentNavigator.cs
- EmptyControlCollection.cs
- DataGridViewSelectedColumnCollection.cs
- DataColumnSelectionConverter.cs
- DataGridTextBoxColumn.cs
- DynamicVirtualDiscoSearcher.cs
- IMembershipProvider.cs
- VerbConverter.cs
- Repeater.cs
- TableItemStyle.cs
- ServerIdentity.cs
- SchemaManager.cs
- DesignerSerializationManager.cs
- BaseParaClient.cs
- PathFigureCollectionConverter.cs
- MachineSettingsSection.cs
- StylusPointCollection.cs
- XmlSchemaExternal.cs
- MenuDesigner.cs
- KeyInstance.cs
- FontFamily.cs
- PowerModeChangedEventArgs.cs
- HyperLink.cs
- TypeReference.cs
- CodeCatchClause.cs
- FontCollection.cs
- XmlSchemaAttributeGroup.cs
- DrawingVisualDrawingContext.cs
- ContentElementAutomationPeer.cs
- ControlCollection.cs
- DataSourceCacheDurationConverter.cs
- UrlPath.cs
- WebPartDisplayModeCollection.cs
- xsdvalidator.cs
- HandleExceptionArgs.cs
- ListDataBindEventArgs.cs
- ListViewItemSelectionChangedEvent.cs
- processwaithandle.cs
- ConditionCollection.cs
- UInt32Storage.cs
- NameValueCollection.cs
- _HeaderInfo.cs
- OutputCacheSettingsSection.cs
- OlePropertyStructs.cs
- RequestNavigateEventArgs.cs
- TextParagraphView.cs
- UdpTransportSettingsElement.cs
- TextElementEnumerator.cs
- DataContractSerializerSection.cs
- SystemTcpConnection.cs
- StringValidator.cs
- Image.cs
- EntityDataSourceStatementEditor.cs
- StreamSecurityUpgradeAcceptor.cs
- RSACryptoServiceProvider.cs
- BitmapEffect.cs
- SqlCommandBuilder.cs
- WorkflowIdleBehavior.cs
- OpenTypeLayout.cs
- AccessKeyManager.cs
- NullableIntSumAggregationOperator.cs
- ADMembershipProvider.cs
- DoubleStorage.cs
- NameValuePermission.cs
- NamespaceEmitter.cs
- WebReferencesBuildProvider.cs
- MainMenu.cs
- RenderDataDrawingContext.cs
- SynchronizedCollection.cs
- TextSchema.cs