Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / precedingsibling.cs / 1 / precedingsibling.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
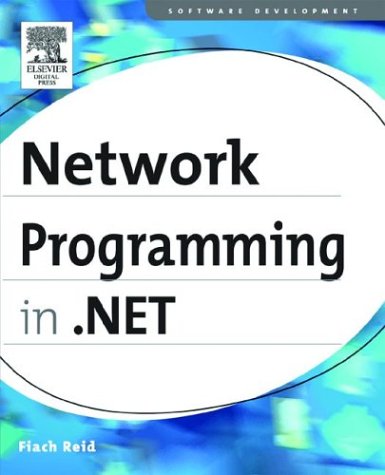
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthenticationException.cs
- XmlSchemaSimpleType.cs
- VectorAnimation.cs
- _LoggingObject.cs
- SiteMapSection.cs
- ToolStripSeparatorRenderEventArgs.cs
- baseaxisquery.cs
- CryptoApi.cs
- ClientTargetSection.cs
- SchemaCollectionCompiler.cs
- ImageSource.cs
- QueryContinueDragEvent.cs
- HMACSHA256.cs
- GrammarBuilderRuleRef.cs
- FormViewModeEventArgs.cs
- EndpointIdentityConverter.cs
- Figure.cs
- Int32.cs
- FontFamilyIdentifier.cs
- ProgressBarBrushConverter.cs
- BooleanToVisibilityConverter.cs
- DocumentAutomationPeer.cs
- TextParentUndoUnit.cs
- SqlException.cs
- TabItem.cs
- ResXFileRef.cs
- SiteMap.cs
- BackgroundFormatInfo.cs
- EventLogPermissionEntryCollection.cs
- ModifierKeysValueSerializer.cs
- SoapAttributeAttribute.cs
- BevelBitmapEffect.cs
- DialogResultConverter.cs
- DesigntimeLicenseContext.cs
- MultiBindingExpression.cs
- RuntimeConfig.cs
- AdministrationHelpers.cs
- Utils.cs
- Page.cs
- XmlSchemaObject.cs
- Figure.cs
- ExecutionTracker.cs
- LocationUpdates.cs
- UnsafeNativeMethods.cs
- MouseEvent.cs
- CharacterMetricsDictionary.cs
- ToolStripDropDownClosedEventArgs.cs
- StatusBarAutomationPeer.cs
- ArgumentValue.cs
- entityreference_tresulttype.cs
- DiscoveryOperationContextExtension.cs
- InternalSafeNativeMethods.cs
- StreamUpgradeAcceptor.cs
- HotSpotCollectionEditor.cs
- MergePropertyDescriptor.cs
- CacheForPrimitiveTypes.cs
- ClientSettingsStore.cs
- TempEnvironment.cs
- EntityTransaction.cs
- CodeRegionDirective.cs
- SafeCryptoHandles.cs
- ConfigurationManagerInternalFactory.cs
- Misc.cs
- EncoderExceptionFallback.cs
- DateTimeValueSerializerContext.cs
- XmlExpressionDumper.cs
- OleDbConnectionInternal.cs
- DataBinding.cs
- CallSiteHelpers.cs
- DataStreams.cs
- WindowPattern.cs
- CompiledAction.cs
- ToolBarTray.cs
- EtwTrackingBehaviorElement.cs
- FileLogRecordEnumerator.cs
- OLEDB_Enum.cs
- _IPv6Address.cs
- HtmlInputSubmit.cs
- Translator.cs
- FieldAccessException.cs
- AssociatedControlConverter.cs
- RtfControls.cs
- xdrvalidator.cs
- StopRoutingHandler.cs
- Icon.cs
- FieldDescriptor.cs
- RoleService.cs
- FunctionNode.cs
- HtmlAnchor.cs
- CellParaClient.cs
- AnnotationResource.cs
- SmtpLoginAuthenticationModule.cs
- XmlSchemaComplexContentExtension.cs
- WeakEventTable.cs
- DataListItemCollection.cs
- PropertyChangingEventArgs.cs
- ActivityInfo.cs
- FixedDocumentPaginator.cs
- InternalSafeNativeMethods.cs
- WorkflowFileItem.cs