Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / WindowPattern.cs / 1305600 / WindowPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Window Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { // Disable warning for obsolete types. These are scheduled to be removed in M8.2 so // only need the warning to come out for components outside of APT. #pragma warning disable 0618 ///wrapper class for Window pattern #if (INTERNAL_COMPILE) internal class WindowPattern: BasePattern #else public class WindowPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private WindowPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Returns the Window pattern identifier public static readonly AutomationPattern Pattern = WindowPatternIdentifiers.Pattern; ///Property ID: CanMaximize - public static readonly AutomationProperty CanMaximizeProperty = WindowPatternIdentifiers.CanMaximizeProperty; ///Property ID: CanMinimize - public static readonly AutomationProperty CanMinimizeProperty = WindowPatternIdentifiers.CanMinimizeProperty; ///Property ID: IsModal - Is this is a modal window public static readonly AutomationProperty IsModalProperty = WindowPatternIdentifiers.IsModalProperty; ///Property ID: WindowVisualState - Is the Window Maximized, Minimized, or Normal (aka restored) public static readonly AutomationProperty WindowVisualStateProperty = WindowPatternIdentifiers.WindowVisualStateProperty; ///Property ID: WindowInteractionState - Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. public static readonly AutomationProperty WindowInteractionStateProperty = WindowPatternIdentifiers.WindowInteractionStateProperty; ///Property ID: - This window is always on top public static readonly AutomationProperty IsTopmostProperty = WindowPatternIdentifiers.IsTopmostProperty; ///Event ID: WindowOpened - Immediately after opening the window - ApplicationWindows or Window Status is not guarantee to be: ReadyForUserInteraction public static readonly AutomationEvent WindowOpenedEvent = WindowPatternIdentifiers.WindowOpenedEvent; ///Event ID: WindowClosed - Immediately after closing the window public static readonly AutomationEvent WindowClosedEvent = WindowPatternIdentifiers.WindowClosedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Changes the State of the window based on the passed enum. /// /// The requested state of the window. /// ////// This API does not work inside the secure execution environment. /// public void SetWindowVisualState( WindowVisualState state ) { UiaCoreApi.WindowPattern_SetWindowVisualState(_hPattern, state); } ////// /// Non-blocking call to close this non-application window. /// When called on a split pane, it will close the pane (thereby removing a /// split), it may or may not also close all other panes related to the /// document/content/etc. This behavior is application dependent. /// /// ////// This API does not work inside the secure execution environment. /// public void Close() { UiaCoreApi.WindowPattern_Close(_hPattern); } ////// /// Causes the calling code to block, waiting the specified number of milliseconds, for the /// associated window to enter an idle state. /// ////// The implementation is dependent on the underlying application framework therefore this /// call may return sometime after the window is ready for user input. The calling code /// should not rely on this call to understand exactly when the window has become idle. /// /// For now this method works reliably for both WinFx and Win32 Windows that are starting /// up. However, if called at other times on WinFx Windows (e.g. during a long layout) /// WaitForInputIdle may return true before the Window is actually idle. Additional work /// needs to be done to detect when WinFx Windows are idle. /// /// The amount of time, in milliseconds, to wait for the /// associated process to become idle. The maximum is the largest possible value of a /// 32-bit integer, which represents infinity to the operating system /// ////// returns true if the window has reached the idle state and false if the timeout occurred. /// public bool WaitForInputIdle( int milliseconds ) { return UiaCoreApi.WindowPattern_WaitForInputIdle(_hPattern, milliseconds); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public WindowPatternInformation Cached { get { Misc.ValidateCached(_cached); return new WindowPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public WindowPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new WindowPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new WindowPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct WindowPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal WindowPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///Is this window Maximizable /// ////// This API does not work inside the secure execution environment. /// public bool CanMaximize { get { return (bool)_el.GetPatternPropertyValue(CanMaximizeProperty, _useCache); } } ////// Is this window Minimizable /// ////// This API does not work inside the secure execution environment. /// public bool CanMinimize { get { return (bool)_el.GetPatternPropertyValue(CanMinimizeProperty, _useCache); } } ////// Is this is a modal window. /// ////// This API does not work inside the secure execution environment. /// public bool IsModal { get { return (bool)_el.GetPatternPropertyValue(IsModalProperty, _useCache); } } ////// Is the Window Maximized, Minimized, or Normal (aka restored) /// ////// This API does not work inside the secure execution environment. /// public WindowVisualState WindowVisualState { get { return (WindowVisualState)_el.GetPatternPropertyValue(WindowVisualStateProperty, _useCache); } } ////// Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. /// ////// This API does not work inside the secure execution environment. /// public WindowInteractionState WindowInteractionState { get { return (WindowInteractionState)_el.GetPatternPropertyValue(WindowInteractionStateProperty, _useCache); } } ////// Is this window is always on top /// ////// This API does not work inside the secure execution environment. /// public bool IsTopmost { get { return (bool)_el.GetPatternPropertyValue(IsTopmostProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Window Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { // Disable warning for obsolete types. These are scheduled to be removed in M8.2 so // only need the warning to come out for components outside of APT. #pragma warning disable 0618 ///wrapper class for Window pattern #if (INTERNAL_COMPILE) internal class WindowPattern: BasePattern #else public class WindowPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private WindowPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Returns the Window pattern identifier public static readonly AutomationPattern Pattern = WindowPatternIdentifiers.Pattern; ///Property ID: CanMaximize - public static readonly AutomationProperty CanMaximizeProperty = WindowPatternIdentifiers.CanMaximizeProperty; ///Property ID: CanMinimize - public static readonly AutomationProperty CanMinimizeProperty = WindowPatternIdentifiers.CanMinimizeProperty; ///Property ID: IsModal - Is this is a modal window public static readonly AutomationProperty IsModalProperty = WindowPatternIdentifiers.IsModalProperty; ///Property ID: WindowVisualState - Is the Window Maximized, Minimized, or Normal (aka restored) public static readonly AutomationProperty WindowVisualStateProperty = WindowPatternIdentifiers.WindowVisualStateProperty; ///Property ID: WindowInteractionState - Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. public static readonly AutomationProperty WindowInteractionStateProperty = WindowPatternIdentifiers.WindowInteractionStateProperty; ///Property ID: - This window is always on top public static readonly AutomationProperty IsTopmostProperty = WindowPatternIdentifiers.IsTopmostProperty; ///Event ID: WindowOpened - Immediately after opening the window - ApplicationWindows or Window Status is not guarantee to be: ReadyForUserInteraction public static readonly AutomationEvent WindowOpenedEvent = WindowPatternIdentifiers.WindowOpenedEvent; ///Event ID: WindowClosed - Immediately after closing the window public static readonly AutomationEvent WindowClosedEvent = WindowPatternIdentifiers.WindowClosedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Changes the State of the window based on the passed enum. /// /// The requested state of the window. /// ////// This API does not work inside the secure execution environment. /// public void SetWindowVisualState( WindowVisualState state ) { UiaCoreApi.WindowPattern_SetWindowVisualState(_hPattern, state); } ////// /// Non-blocking call to close this non-application window. /// When called on a split pane, it will close the pane (thereby removing a /// split), it may or may not also close all other panes related to the /// document/content/etc. This behavior is application dependent. /// /// ////// This API does not work inside the secure execution environment. /// public void Close() { UiaCoreApi.WindowPattern_Close(_hPattern); } ////// /// Causes the calling code to block, waiting the specified number of milliseconds, for the /// associated window to enter an idle state. /// ////// The implementation is dependent on the underlying application framework therefore this /// call may return sometime after the window is ready for user input. The calling code /// should not rely on this call to understand exactly when the window has become idle. /// /// For now this method works reliably for both WinFx and Win32 Windows that are starting /// up. However, if called at other times on WinFx Windows (e.g. during a long layout) /// WaitForInputIdle may return true before the Window is actually idle. Additional work /// needs to be done to detect when WinFx Windows are idle. /// /// The amount of time, in milliseconds, to wait for the /// associated process to become idle. The maximum is the largest possible value of a /// 32-bit integer, which represents infinity to the operating system /// ////// returns true if the window has reached the idle state and false if the timeout occurred. /// public bool WaitForInputIdle( int milliseconds ) { return UiaCoreApi.WindowPattern_WaitForInputIdle(_hPattern, milliseconds); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public WindowPatternInformation Cached { get { Misc.ValidateCached(_cached); return new WindowPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public WindowPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new WindowPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new WindowPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct WindowPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal WindowPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///Is this window Maximizable /// ////// This API does not work inside the secure execution environment. /// public bool CanMaximize { get { return (bool)_el.GetPatternPropertyValue(CanMaximizeProperty, _useCache); } } ////// Is this window Minimizable /// ////// This API does not work inside the secure execution environment. /// public bool CanMinimize { get { return (bool)_el.GetPatternPropertyValue(CanMinimizeProperty, _useCache); } } ////// Is this is a modal window. /// ////// This API does not work inside the secure execution environment. /// public bool IsModal { get { return (bool)_el.GetPatternPropertyValue(IsModalProperty, _useCache); } } ////// Is the Window Maximized, Minimized, or Normal (aka restored) /// ////// This API does not work inside the secure execution environment. /// public WindowVisualState WindowVisualState { get { return (WindowVisualState)_el.GetPatternPropertyValue(WindowVisualStateProperty, _useCache); } } ////// Is the Window Closing, ReadyForUserInteraction, BlockedByModalWindow or NotResponding. /// ////// This API does not work inside the secure execution environment. /// public WindowInteractionState WindowInteractionState { get { return (WindowInteractionState)_el.GetPatternPropertyValue(WindowInteractionStateProperty, _useCache); } } ////// Is this window is always on top /// ////// This API does not work inside the secure execution environment. /// public bool IsTopmost { get { return (bool)_el.GetPatternPropertyValue(IsTopmostProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
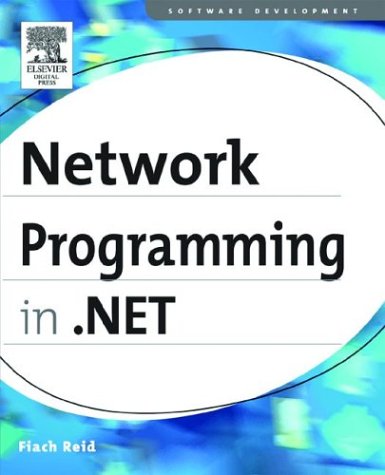
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Attributes.cs
- OdbcError.cs
- TypeConverterValueSerializer.cs
- DescendantOverDescendantQuery.cs
- MeshGeometry3D.cs
- XmlTextReaderImpl.cs
- HttpModuleAction.cs
- ParameterElementCollection.cs
- StringSorter.cs
- DataGridItem.cs
- ImageField.cs
- CompiledQueryCacheKey.cs
- ObjectListDataBindEventArgs.cs
- Accessors.cs
- ScrollViewerAutomationPeer.cs
- RIPEMD160Managed.cs
- MailDefinition.cs
- NoneExcludedImageIndexConverter.cs
- GridViewUpdatedEventArgs.cs
- COM2PictureConverter.cs
- DesignTimeVisibleAttribute.cs
- ListViewTableCell.cs
- DataGridViewComboBoxCell.cs
- HeaderCollection.cs
- FixUpCollection.cs
- isolationinterop.cs
- TdsParserStateObject.cs
- _UriSyntax.cs
- XmlSchemaImport.cs
- Asn1IntegerConverter.cs
- NGCSerializer.cs
- DurableInstanceManager.cs
- EntryWrittenEventArgs.cs
- Emitter.cs
- HtmlCalendarAdapter.cs
- RestHandler.cs
- HasCopySemanticsAttribute.cs
- CodeTypeReferenceCollection.cs
- DesignerDeviceConfig.cs
- XmlExceptionHelper.cs
- ArgumentNullException.cs
- MatrixUtil.cs
- OptimalBreakSession.cs
- DataGridPreparingCellForEditEventArgs.cs
- CompleteWizardStep.cs
- HwndAppCommandInputProvider.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- Point3DCollection.cs
- NetNamedPipeBinding.cs
- EndpointPerformanceCounters.cs
- ImportContext.cs
- ToolboxBitmapAttribute.cs
- MethodCallConverter.cs
- ThreadWorkerController.cs
- DbSourceCommand.cs
- ProviderConnectionPoint.cs
- PenContext.cs
- TogglePatternIdentifiers.cs
- SecurityTokenProvider.cs
- FontSizeConverter.cs
- MediaContextNotificationWindow.cs
- MessageHeaderDescription.cs
- EntityParameterCollection.cs
- Label.cs
- PipelineComponent.cs
- SoapFault.cs
- TCPClient.cs
- JsonClassDataContract.cs
- Transform.cs
- ReadOnlyCollection.cs
- AttributedMetaModel.cs
- TreeViewImageIndexConverter.cs
- BatchParser.cs
- ExpressionEditorAttribute.cs
- MaskedTextBoxTextEditor.cs
- RefExpr.cs
- WinFormsSecurity.cs
- CryptoKeySecurity.cs
- ZipIOExtraField.cs
- CardSpacePolicyElement.cs
- DataGridViewSelectedRowCollection.cs
- HitTestFilterBehavior.cs
- SurrogateSelector.cs
- GlobalProxySelection.cs
- EastAsianLunisolarCalendar.cs
- ColumnMapProcessor.cs
- EncodingNLS.cs
- EntityContainerRelationshipSetEnd.cs
- DataQuery.cs
- TimerEventSubscriptionCollection.cs
- HttpCachePolicy.cs
- EdmFunction.cs
- HitTestParameters3D.cs
- RoleManagerEventArgs.cs
- BitmapEffectDrawingContextState.cs
- MatrixCamera.cs
- ListComponentEditorPage.cs
- GPRECT.cs
- Enlistment.cs
- XamlPathDataSerializer.cs