Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Diagnostics / EndpointPerformanceCounters.cs / 1 / EndpointPerformanceCounters.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System.Collections.Generic; using System.ServiceModel.Channels; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.ServiceModel.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; internal class EndpointPerformanceCounters : PerformanceCountersBase { string instanceName; PerformanceCounter[] counters = null; enum PerfCounters : int { Calls = 0, CallsPerSecond, CallsOutstanding, CallsFailed, CallsFailedPerSecond, CallsFaulted, CallsFaultedPerSecond, CallDuration, CallDurationBase, SecurityValidationAuthenticationFailures, SecurityValidationAuthenticationFailuresPerSecond, CallsNotAuthorized, CallsNotAuthorizedPerSecond, RMSessionsFaulted, RMSessionsFaultedPerSecond, RMMessagesDropped, RMMessagesDroppedPerSecond, TxFlowed, TxFlowedPerSecond, TotalCounters = TxFlowedPerSecond + 1 } string[] perfCounterNames = { PerformanceCounterStrings.SERVICEMODELENDPOINT.ECalls, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsOutstanding, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsFailed, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsFailedPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsFaulted, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallsFaultedPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallDuration, PerformanceCounterStrings.SERVICEMODELENDPOINT.ECallDurationBase, PerformanceCounterStrings.SERVICEMODELENDPOINT.ESecurityValidationAuthenticationFailures, PerformanceCounterStrings.SERVICEMODELENDPOINT.ESecurityValidationAuthenticationFailuresPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ESecurityCallsNotAuthorized, PerformanceCounterStrings.SERVICEMODELENDPOINT.ESecurityCallsNotAuthorizedPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ERMSessionsFaulted, PerformanceCounterStrings.SERVICEMODELENDPOINT.ERMSessionsFaultedPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ERMMessagesDropped, PerformanceCounterStrings.SERVICEMODELENDPOINT.ERMMessagesDroppedPerSecond, PerformanceCounterStrings.SERVICEMODELENDPOINT.ETxFlowed, PerformanceCounterStrings.SERVICEMODELENDPOINT.ETxFlowedPerSecond, }; const int maxCounterLength = 64; const int hashLength = 2; [Flags] enum truncOptions : uint { NoBits = 0, service15 = 0x01, contract16 = 0x02, uri31 = 0x04 } internal EndpointPerformanceCounters(string service, string contract, string uri) { this.instanceName = EndpointPerformanceCounters.CreateFriendlyInstanceName(service, contract, uri); this.counters = new PerformanceCounter[(int)PerfCounters.TotalCounters]; for (int i = 0; i < (int)PerfCounters.TotalCounters; i++) { PerformanceCounter counter = PerformanceCounters.GetEndpointPerformanceCounter(this.perfCounterNames[i], this.instanceName); if (counter != null) { try { counter.RawValue = 0; this.counters[i] = counter; } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (ExceptionUtility.IsFatal(e)) throw; if (DiagnosticUtility.ShouldTraceError) TraceUtility.TraceEvent(TraceEventType.Error, TraceCode.PerformanceCounterFailedToLoad, null, e); break; } } else { break; } } } internal static string CreateFriendlyInstanceName(string service, string contract, string uri) { // instance name is: serviceName.interfaceName.operationName@uri int length = service.Length + contract.Length + uri.Length + 2; if (length > maxCounterLength) { int count = 0; truncOptions tasks = EndpointPerformanceCounters.GetCompressionTasks( length, service.Length, contract.Length, uri.Length); //if necessary, compress service name to 13 chars with a 2 char hash code if ((tasks & truncOptions.service15) > 0) { count = 15; service = GetHashedString(service, count - hashLength, service.Length - count + hashLength, true); } //if necessary, compress contract name to 14 chars with a 2 char hash code if ((tasks & truncOptions.contract16) > 0) { count = 16; contract = GetHashedString(contract, count - hashLength, contract.Length - count + hashLength, true); } //if necessary, compress uri to 29 chars with a 2 char hash code if ((tasks & truncOptions.uri31) > 0) { count = 31; uri = GetHashedString(uri, 0, uri.Length - count + hashLength, false); } } // replace '/' with '|' because perfmon fails when '/' is in perfcounter instance name return service + "." + contract + "@" + uri.Replace('/', '|'); } private static truncOptions GetCompressionTasks(int totalLen, int serviceLen, int contractLen, int uriLen) { truncOptions bitmask = 0; if (totalLen > maxCounterLength) { int workingLen = totalLen; //note: order of if statements important (see spec)! if (workingLen > maxCounterLength && serviceLen > 15) { bitmask |= truncOptions.service15; //compress service name to 16 chars workingLen -= serviceLen - 15; } if (workingLen > maxCounterLength && contractLen > 16) { bitmask |= truncOptions.contract16; //compress contract name to 8 chars workingLen -= contractLen - 16; } if (workingLen > maxCounterLength && uriLen > 31) { bitmask |= truncOptions.uri31; //compress uri to 31 chars } } return bitmask; } internal override PerformanceCounter[] Counters { get { return this.counters; } set { this.counters = value; } } internal override string InstanceName { get { return this.instanceName; } } internal override string[] CounterNames { get { return this.perfCounterNames; } } internal override int PerfCounterStart { get { return (int)PerfCounters.Calls; } } internal override int PerfCounterEnd { get { return (int)PerfCounters.TotalCounters; } } internal void MethodCalled() { Increment((int)PerfCounters.Calls); Increment((int)PerfCounters.CallsPerSecond); Increment((int)PerfCounters.CallsOutstanding); } internal void MethodReturnedSuccess() { Decrement((int)PerfCounters.CallsOutstanding); } internal void MethodReturnedError() { Increment((int)PerfCounters.CallsFailed); Increment((int)PerfCounters.CallsFailedPerSecond); Decrement((int)PerfCounters.CallsOutstanding); } internal void MethodReturnedFault() { Increment((int)PerfCounters.CallsFaulted); Increment((int)PerfCounters.CallsFaultedPerSecond); Decrement((int)PerfCounters.CallsOutstanding); } internal void SaveCallDuration(long time) { IncrementBy((int)PerfCounters.CallDuration, time); Increment((int)PerfCounters.CallDurationBase); } internal void AuthenticationFailed() { Increment((int)PerfCounters.SecurityValidationAuthenticationFailures); Increment((int)PerfCounters.SecurityValidationAuthenticationFailuresPerSecond); } internal void AuthorizationFailed() { Increment((int)PerfCounters.CallsNotAuthorized); Increment((int)PerfCounters.CallsNotAuthorizedPerSecond); } internal void SessionFaulted() { Increment((int)PerfCounters.RMSessionsFaulted); Increment((int)PerfCounters.RMSessionsFaultedPerSecond); } internal void MessageDropped() { Increment((int)PerfCounters.RMMessagesDropped); Increment((int)PerfCounters.RMMessagesDroppedPerSecond); } internal void TxFlowed() { Increment((int)PerfCounters.TxFlowed); Increment((int)PerfCounters.TxFlowedPerSecond); } internal bool Initialized { get { return this.counters != null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
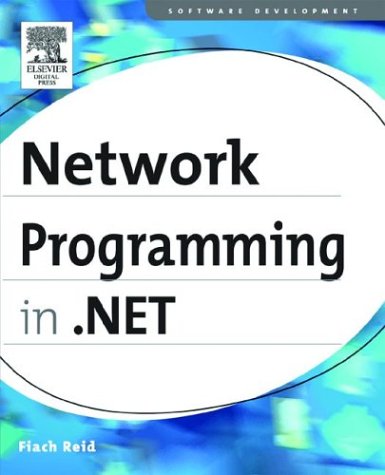
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BasicSecurityProfileVersion.cs
- StringDictionaryCodeDomSerializer.cs
- DebugHandleTracker.cs
- ClientSettings.cs
- datacache.cs
- SrgsToken.cs
- WS2007FederationHttpBinding.cs
- UnorderedHashRepartitionStream.cs
- ConfigurationManagerHelper.cs
- ParallelDesigner.xaml.cs
- KnownTypeDataContractResolver.cs
- ApplicationGesture.cs
- ObjectConverter.cs
- ValueExpressions.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- DataGridViewAccessibleObject.cs
- ArraySegment.cs
- Array.cs
- mediaeventargs.cs
- SqlRowUpdatingEvent.cs
- XmlBinaryReader.cs
- DataGridViewHeaderCell.cs
- DoubleStorage.cs
- DtdParser.cs
- UniqueEventHelper.cs
- AbstractDataSvcMapFileLoader.cs
- RewritingValidator.cs
- DetailsView.cs
- MasterPageBuildProvider.cs
- ErrorProvider.cs
- BaseDataBoundControl.cs
- XmlWhitespace.cs
- SQLInt32Storage.cs
- _BasicClient.cs
- ConnectionManagementElementCollection.cs
- MetafileHeaderWmf.cs
- TypeContext.cs
- ToolStripItemImageRenderEventArgs.cs
- Crc32Helper.cs
- NetMsmqSecurityElement.cs
- OleDbConnection.cs
- BuildDependencySet.cs
- XmlSerializationWriter.cs
- AppSettingsSection.cs
- GACMembershipCondition.cs
- UIPropertyMetadata.cs
- AnonymousIdentificationModule.cs
- EventHandlingScope.cs
- AutomationPropertyInfo.cs
- ImageMap.cs
- LinkedResource.cs
- BackEase.cs
- handlecollector.cs
- ThreadExceptionEvent.cs
- EndpointDiscoveryMetadata.cs
- MeasureData.cs
- D3DImage.cs
- EditorPartChrome.cs
- SystemDiagnosticsSection.cs
- SerializableAttribute.cs
- HierarchicalDataBoundControl.cs
- AliasGenerator.cs
- EnumerableRowCollection.cs
- _CommandStream.cs
- GlyphInfoList.cs
- Vector3DConverter.cs
- HatchBrush.cs
- DesignerHost.cs
- WindowsGraphics.cs
- SelectionHighlightInfo.cs
- DataGridColumnsPage.cs
- SectionUpdates.cs
- DataServiceQueryException.cs
- WebPartAddingEventArgs.cs
- XmlTextEncoder.cs
- HtmlEmptyTagControlBuilder.cs
- RuntimeCompatibilityAttribute.cs
- Attributes.cs
- Label.cs
- COM2EnumConverter.cs
- SafeWaitHandle.cs
- SqlClientPermission.cs
- TrailingSpaceComparer.cs
- XmlSerializerVersionAttribute.cs
- FactorySettingsElement.cs
- SqlNodeTypeOperators.cs
- WebPartEventArgs.cs
- BamlRecordReader.cs
- WebPartConnectVerb.cs
- Activator.cs
- QuaternionConverter.cs
- ColumnMapProcessor.cs
- PixelShader.cs
- SqlDataReader.cs
- NativeMethods.cs
- NullableLongSumAggregationOperator.cs
- PrintControllerWithStatusDialog.cs
- RemotingAttributes.cs
- UnknownBitmapDecoder.cs
- QuaternionAnimationBase.cs