Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Dom / XmlWhitespace.cs / 1305376 / XmlWhitespace.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Xml.XPath; using System.Text; using System.Diagnostics; // Represents the text content of an element or attribute. public class XmlWhitespace : XmlCharacterData { protected internal XmlWhitespace( string strData, XmlDocument doc ) : base( strData, doc ) { if ( !doc.IsLoading && !base.CheckOnData( strData ) ) throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } // Gets the name of the node. public override String Name { get { return OwnerDocument.strNonSignificantWhitespaceName; } } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strNonSignificantWhitespaceName; } } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.Whitespace; } } public override XmlNode ParentNode { get { switch (parentNode.NodeType) { case XmlNodeType.Document: return base.ParentNode; case XmlNodeType.Text: case XmlNodeType.CDATA: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: XmlNode parent = parentNode.parentNode; while (parent.IsText) { parent = parent.parentNode; } return parent; default: return parentNode; } } } public override String Value { get { return Data; } set { if ( CheckOnData( value ) ) Data = value; else throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateWhitespace( Data ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteWhitespace(Data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override XPathNodeType XPNodeType { get { XPathNodeType xnt = XPathNodeType.Whitespace; DecideXPNodeTypeForTextNodes(this, ref xnt); return xnt; } } internal override bool IsText { get { return true; } } internal override XmlNode PreviousText { get { if (parentNode.IsText) { return parentNode; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Xml.XPath; using System.Text; using System.Diagnostics; // Represents the text content of an element or attribute. public class XmlWhitespace : XmlCharacterData { protected internal XmlWhitespace( string strData, XmlDocument doc ) : base( strData, doc ) { if ( !doc.IsLoading && !base.CheckOnData( strData ) ) throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } // Gets the name of the node. public override String Name { get { return OwnerDocument.strNonSignificantWhitespaceName; } } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strNonSignificantWhitespaceName; } } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.Whitespace; } } public override XmlNode ParentNode { get { switch (parentNode.NodeType) { case XmlNodeType.Document: return base.ParentNode; case XmlNodeType.Text: case XmlNodeType.CDATA: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: XmlNode parent = parentNode.parentNode; while (parent.IsText) { parent = parent.parentNode; } return parent; default: return parentNode; } } } public override String Value { get { return Data; } set { if ( CheckOnData( value ) ) Data = value; else throw new ArgumentException(Res.GetString(Res.Xdom_WS_Char)); } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateWhitespace( Data ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteWhitespace(Data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override XPathNodeType XPNodeType { get { XPathNodeType xnt = XPathNodeType.Whitespace; DecideXPNodeTypeForTextNodes(this, ref xnt); return xnt; } } internal override bool IsText { get { return true; } } internal override XmlNode PreviousText { get { if (parentNode.IsText) { return parentNode; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
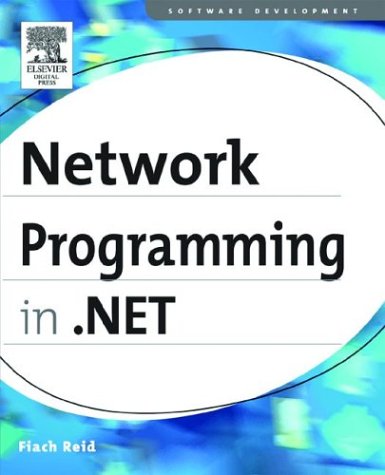
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MSAANativeProvider.cs
- PrivilegedConfigurationManager.cs
- Registry.cs
- HybridDictionary.cs
- IsolatedStoragePermission.cs
- DataColumnMappingCollection.cs
- ContentAlignmentEditor.cs
- ParserContext.cs
- XmlSchemaSimpleContent.cs
- sqlcontext.cs
- DesignerSerializerAttribute.cs
- ClientBuildManagerCallback.cs
- CompModSwitches.cs
- LocalizationCodeDomSerializer.cs
- HandlerFactoryWrapper.cs
- Point3DKeyFrameCollection.cs
- SqlConnectionStringBuilder.cs
- TrustSection.cs
- DSGeneratorProblem.cs
- XmlAnyElementAttribute.cs
- ListDictionary.cs
- StringExpressionSet.cs
- PageCatalogPart.cs
- XamlReader.cs
- Missing.cs
- ScrollableControl.cs
- HttpInputStream.cs
- IndicCharClassifier.cs
- StrongNamePublicKeyBlob.cs
- NamedElement.cs
- BufferedReadStream.cs
- BaseConfigurationRecord.cs
- BoolExpression.cs
- ScriptResourceAttribute.cs
- XsdBuilder.cs
- GraphicsPath.cs
- infer.cs
- COM2PictureConverter.cs
- RegexWorker.cs
- ServiceNameCollection.cs
- WorkflowClientDeliverMessageWrapper.cs
- ColorDialog.cs
- ProxyAttribute.cs
- wgx_render.cs
- SubtreeProcessor.cs
- ToolboxItemImageConverter.cs
- InternalDispatchObject.cs
- PerformanceCounterLib.cs
- StylusDownEventArgs.cs
- CollectionChange.cs
- WebPartZoneBase.cs
- DataTableMappingCollection.cs
- TraversalRequest.cs
- FillErrorEventArgs.cs
- AppDomainProtocolHandler.cs
- WindowsAuthenticationEventArgs.cs
- SelectionRangeConverter.cs
- SafePipeHandle.cs
- MsmqUri.cs
- ResourceCategoryAttribute.cs
- WebPartConnectionsCancelVerb.cs
- LinqMaximalSubtreeNominator.cs
- SpeechSynthesizer.cs
- WorkflowFormatterBehavior.cs
- IgnoreFileBuildProvider.cs
- BindingCompleteEventArgs.cs
- WmlPageAdapter.cs
- AvtEvent.cs
- NodeLabelEditEvent.cs
- Debugger.cs
- SafeRightsManagementSessionHandle.cs
- BitmapEffectDrawing.cs
- HttpStreamXmlDictionaryWriter.cs
- JournalNavigationScope.cs
- OdbcConnection.cs
- TemplateNameScope.cs
- CodeCompiler.cs
- bidPrivateBase.cs
- XmlName.cs
- WebInvokeAttribute.cs
- MSAAWinEventWrap.cs
- DifferencingCollection.cs
- TableHeaderCell.cs
- BitmapEffectDrawingContextWalker.cs
- ObjectSpanRewriter.cs
- DeferredRunTextReference.cs
- IsolatedStorageFileStream.cs
- JsonWriter.cs
- CommandLibraryHelper.cs
- CompositionAdorner.cs
- _NegoState.cs
- WorkflowInstance.cs
- WbmpConverter.cs
- ParagraphVisual.cs
- FixedSOMTextRun.cs
- ScriptModule.cs
- PerformanceCounterNameAttribute.cs
- WSFederationHttpSecurity.cs
- CaseInsensitiveHashCodeProvider.cs
- CrossAppDomainChannel.cs