Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / LinearGradientBrush.cs / 1 / LinearGradientBrush.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of LinearGradientBrush. // The LinearGradientBrush is a GradientBrush which defines its // Gradient as a linear interpolation between two parallel lines. // // History: // 05/07/2003 : adsmith - Created it. // 09/21/2004 : timothyc - Added GradientStopCollection constructors. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Runtime.InteropServices; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// LinearGradientBrush - This GradientBrush defines its Gradient as an interpolation /// between two parallel lines. /// public sealed partial class LinearGradientBrush : GradientBrush { #region Constructors ////// Default constructor for LinearGradientBrush. The resulting brush has no content. /// public LinearGradientBrush() : base() { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(Color startColor, Color endColor, double angle) : base() { EndPoint = EndPointFromAngle(angle); GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with two colors at the specified start and end points. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The start point /// The end point public LinearGradientBrush(Color startColor, Color endColor, Point startPoint, Point endPoint) : base() { StartPoint = startPoint; EndPoint = endPoint; GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// /// GradientStopCollection to set on this brush. public LinearGradientBrush(GradientStopCollection gradientStopCollection) : base (gradientStopCollection) { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// GradientStopCollection to set on this brush. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(GradientStopCollection gradientStopCollection, double angle) : base (gradientStopCollection) { EndPoint = EndPointFromAngle(angle); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// The StartPoint and EndPoint are set to the specified startPoint and endPoint. /// /// GradientStopCollection to set on this brush. /// The start point /// The end point public LinearGradientBrush(GradientStopCollection gradientStopCollection, Point startPoint, Point endPoint) : base (gradientStopCollection) { StartPoint = startPoint; EndPoint = endPoint; } #endregion Constructors ////// Critical: This code accesses unsafe code blocks /// TreatAsSafe: This code does is safe to call but needs to be verified for correctness /// [SecurityCritical, SecurityTreatAsSafe] private void ManualUpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; GradientStopCollection vGradientStops = GradientStops; DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hStartPointAnimations = GetAnimationResourceHandle(StartPointProperty, channel); DUCE.ResourceHandle hEndPointAnimations = GetAnimationResourceHandle(EndPointProperty, channel); unsafe { DUCE.MILCMD_LINEARGRADIENTBRUSH data; data.Type = MILCMD.MilCmdLinearGradientBrush; data.Handle = _duceResource.GetHandle(channel); double tempOpacity = Opacity; DUCE.CopyBytes((byte*)&data.Opacity, (byte*)&tempOpacity, 8); data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; data.ColorInterpolationMode = ColorInterpolationMode; data.MappingMode = MappingMode; data.SpreadMethod = SpreadMethod; Point tempStartPoint = StartPoint; DUCE.CopyBytes((byte*)&data.StartPoint, (byte*)&tempStartPoint, 16); data.hStartPointAnimations = hStartPointAnimations; Point tempEndPoint = EndPoint; DUCE.CopyBytes((byte*)&data.EndPoint, (byte*)&tempEndPoint, 16); data.hEndPointAnimations = hEndPointAnimations; // NTRAID#Longhorn-1011154-2004/8/12-asecchia GradientStopCollection: Need to enforce upper-limit of gradient stop capacity int count = (vGradientStops == null) ? 0 : vGradientStops.Count; data.GradientStopsSize = (UInt32)(sizeof(DUCE.MIL_GRADIENTSTOP)*count); channel.BeginCommand( (byte*)&data, sizeof(DUCE.MILCMD_LINEARGRADIENTBRUSH), sizeof(DUCE.MIL_GRADIENTSTOP)*count ); for (int i=0; i/// LinearGradientBrush - This GradientBrush defines its Gradient as an interpolation /// between two parallel lines. /// public sealed partial class LinearGradientBrush : GradientBrush { #region Constructors /// /// Default constructor for LinearGradientBrush. The resulting brush has no content. /// public LinearGradientBrush() : base() { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(Color startColor, Color endColor, double angle) : base() { EndPoint = EndPointFromAngle(angle); GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with two colors at the specified start and end points. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The start point /// The end point public LinearGradientBrush(Color startColor, Color endColor, Point startPoint, Point endPoint) : base() { StartPoint = startPoint; EndPoint = endPoint; GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// /// GradientStopCollection to set on this brush. public LinearGradientBrush(GradientStopCollection gradientStopCollection) : base (gradientStopCollection) { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// GradientStopCollection to set on this brush. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(GradientStopCollection gradientStopCollection, double angle) : base (gradientStopCollection) { EndPoint = EndPointFromAngle(angle); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// The StartPoint and EndPoint are set to the specified startPoint and endPoint. /// /// GradientStopCollection to set on this brush. /// The start point /// The end point public LinearGradientBrush(GradientStopCollection gradientStopCollection, Point startPoint, Point endPoint) : base (gradientStopCollection) { StartPoint = startPoint; EndPoint = endPoint; } #endregion Constructors ////// Critical: This code accesses unsafe code blocks /// TreatAsSafe: This code does is safe to call but needs to be verified for correctness /// [SecurityCritical, SecurityTreatAsSafe] private void ManualUpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; GradientStopCollection vGradientStops = GradientStops; DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hStartPointAnimations = GetAnimationResourceHandle(StartPointProperty, channel); DUCE.ResourceHandle hEndPointAnimations = GetAnimationResourceHandle(EndPointProperty, channel); unsafe { DUCE.MILCMD_LINEARGRADIENTBRUSH data; data.Type = MILCMD.MilCmdLinearGradientBrush; data.Handle = _duceResource.GetHandle(channel); double tempOpacity = Opacity; DUCE.CopyBytes((byte*)&data.Opacity, (byte*)&tempOpacity, 8); data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; data.ColorInterpolationMode = ColorInterpolationMode; data.MappingMode = MappingMode; data.SpreadMethod = SpreadMethod; Point tempStartPoint = StartPoint; DUCE.CopyBytes((byte*)&data.StartPoint, (byte*)&tempStartPoint, 16); data.hStartPointAnimations = hStartPointAnimations; Point tempEndPoint = EndPoint; DUCE.CopyBytes((byte*)&data.EndPoint, (byte*)&tempEndPoint, 16); data.hEndPointAnimations = hEndPointAnimations; // NTRAID#Longhorn-1011154-2004/8/12-asecchia GradientStopCollection: Need to enforce upper-limit of gradient stop capacity int count = (vGradientStops == null) ? 0 : vGradientStops.Count; data.GradientStopsSize = (UInt32)(sizeof(DUCE.MIL_GRADIENTSTOP)*count); channel.BeginCommand( (byte*)&data, sizeof(DUCE.MILCMD_LINEARGRADIENTBRUSH), sizeof(DUCE.MIL_GRADIENTSTOP)*count ); for (int i=0; i
Link Menu
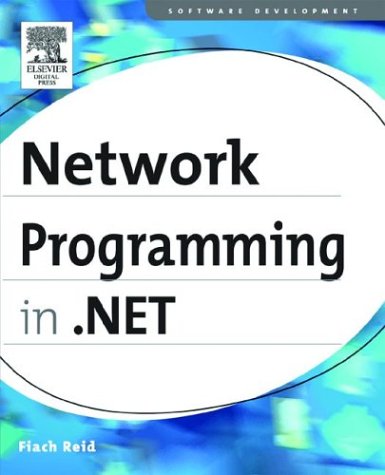
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HintTextMaxWidthConverter.cs
- AutomationPeer.cs
- Image.cs
- ImageListUtils.cs
- CodeCastExpression.cs
- XslCompiledTransform.cs
- XsltSettings.cs
- Panel.cs
- SharedDp.cs
- Matrix3D.cs
- DataFormats.cs
- Transform3DCollection.cs
- MimeWriter.cs
- ClipboardProcessor.cs
- ObjectConverter.cs
- DnsPermission.cs
- ProfilePropertySettings.cs
- RichTextBoxConstants.cs
- ToolStripGripRenderEventArgs.cs
- OdbcCommand.cs
- TypeConverterHelper.cs
- DataGridViewColumnDesigner.cs
- DataGridTemplateColumn.cs
- FileLevelControlBuilderAttribute.cs
- ComponentEditorForm.cs
- PageSetupDialog.cs
- AssemblyBuilderData.cs
- RemoteWebConfigurationHostStream.cs
- ServiceHostFactory.cs
- ClientCredentials.cs
- EntityParameter.cs
- DataPagerFieldCollection.cs
- PropertyDescriptors.cs
- UnmanagedMemoryStream.cs
- PathFigure.cs
- CheckBoxFlatAdapter.cs
- RequestQueue.cs
- FormsAuthenticationModule.cs
- ObjectMaterializedEventArgs.cs
- TraceContextEventArgs.cs
- DetailsViewDeleteEventArgs.cs
- Geometry3D.cs
- TypeRestriction.cs
- DrawingGroup.cs
- ImpersonationOption.cs
- GcSettings.cs
- WindowPattern.cs
- RegistryPermission.cs
- EventProviderTraceListener.cs
- DiscriminatorMap.cs
- UnitySerializationHolder.cs
- MatrixValueSerializer.cs
- FormCollection.cs
- AttributeCollection.cs
- RangeContentEnumerator.cs
- SettingsBase.cs
- EpmCustomContentWriterNodeData.cs
- IpcChannel.cs
- SessionStateModule.cs
- Utils.cs
- ItemAutomationPeer.cs
- _NetRes.cs
- WindowInteractionStateTracker.cs
- DrawingContextWalker.cs
- SurrogateSelector.cs
- JoinElimination.cs
- OutputCacheProfileCollection.cs
- WorkflowServiceOperationListItem.cs
- FileLogRecordStream.cs
- ProcessModelSection.cs
- PtsContext.cs
- GenericNameHandler.cs
- Function.cs
- CookieProtection.cs
- Gdiplus.cs
- ServiceSecurityAuditElement.cs
- ExpressionBuilderContext.cs
- SqlConnectionFactory.cs
- PictureBox.cs
- CompositeCollection.cs
- AdRotator.cs
- HyperLinkField.cs
- MiniAssembly.cs
- ConfigXmlWhitespace.cs
- HandledEventArgs.cs
- StrokeNodeEnumerator.cs
- SqlDataSourceEnumerator.cs
- UnmanagedHandle.cs
- ClientFormsAuthenticationCredentials.cs
- CapabilitiesUse.cs
- TreeNodeStyle.cs
- UIElement3D.cs
- ParseHttpDate.cs
- EventListenerClientSide.cs
- BindingExpressionUncommonField.cs
- SafeViewOfFileHandle.cs
- HighlightVisual.cs
- RangeBaseAutomationPeer.cs
- Function.cs
- BulletedList.cs