Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Markup / TypeContext.cs / 1 / TypeContext.cs
//---------------------------------------------------------------------------- // // File: typeContext.cs // // Description: // class for the main TypeConverterContext object passed to type converters // // // History: // 8/02/01: rogerg Created // 05/23/03: peterost Ported to wcp // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Xml; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ///TypeConverterContext class used for parsing Attributes. internal class TypeConvertContext : ITypeDescriptorContext { #region Public #region Methods ////// OnComponentChange /// ////// member is public only because base class has /// this public member declared /// ////// void /// public void OnComponentChanged() { } ////// OnComponentChanging /// ////// member is public only because base class has /// this public member declared /// ////// void /// public bool OnComponentChanging() { return false; } ////// IServiceProvider GetService implementation /// /// /// Type of Service to be returned /// ////// member is public only because base class has /// this public member declared /// ////// Service object or null if service is not found /// virtual public object GetService(Type serviceType) { if (serviceType == typeof(IUriContext)) { return _parserContext as IUriContext; } // temporary code to optimize Paints.White etc, until this is done // in a more generic fashion in SolidPaint ctor else if (serviceType == typeof(string)) { return _attribStringValue; } #if PBTCOMPILER return null; #else // Check for the other provided services ProvideValueServiceProvider serviceProvider = _parserContext.ProvideValueProvider; return serviceProvider.GetService( serviceType ); #endif } #endregion Methods #region Properties ///Container property ////// property is public only because base class has /// this public property declared /// public IContainer Container { get {return null;} } ///Instance property ////// property is public only because base class has /// this public property declared /// public object Instance { get { return null; } } ///Propert Descriptor ////// property is public only because base class has /// this public property declared /// public PropertyDescriptor PropertyDescriptor { get { return null;} } #if !PBTCOMPILER // Make the ParserContext available internally as an optimization. public ParserContext ParserContext { get { return _parserContext; } } #endif #endregion Properties #endregion Public #region Internal #region Contructors #if !PBTCOMPILER ////// /// /// public TypeConvertContext(ParserContext parserContext) { _parserContext = parserContext; } #endif // temporary code to optimize Paints.White etc, until this is done // in a more generic fashion in SolidPaint ctor #if PBTCOMPILER ////// /// /// /// public TypeConvertContext(ParserContext parserContext, string originalAttributeValue) { _parserContext = parserContext; _attribStringValue = originalAttributeValue; } #endif #endregion Constructors #endregion internal #region Private #region Data ParserContext _parserContext; // _attribStringValue is never set when !PBTCOMPILER #pragma warning disable 0649 string _attribStringValue; #pragma warning restore 0649 #endregion Data #endregion Private } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: typeContext.cs // // Description: // class for the main TypeConverterContext object passed to type converters // // // History: // 8/02/01: rogerg Created // 05/23/03: peterost Ported to wcp // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Xml; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ///TypeConverterContext class used for parsing Attributes. internal class TypeConvertContext : ITypeDescriptorContext { #region Public #region Methods ////// OnComponentChange /// ////// member is public only because base class has /// this public member declared /// ////// void /// public void OnComponentChanged() { } ////// OnComponentChanging /// ////// member is public only because base class has /// this public member declared /// ////// void /// public bool OnComponentChanging() { return false; } ////// IServiceProvider GetService implementation /// /// /// Type of Service to be returned /// ////// member is public only because base class has /// this public member declared /// ////// Service object or null if service is not found /// virtual public object GetService(Type serviceType) { if (serviceType == typeof(IUriContext)) { return _parserContext as IUriContext; } // temporary code to optimize Paints.White etc, until this is done // in a more generic fashion in SolidPaint ctor else if (serviceType == typeof(string)) { return _attribStringValue; } #if PBTCOMPILER return null; #else // Check for the other provided services ProvideValueServiceProvider serviceProvider = _parserContext.ProvideValueProvider; return serviceProvider.GetService( serviceType ); #endif } #endregion Methods #region Properties ///Container property ////// property is public only because base class has /// this public property declared /// public IContainer Container { get {return null;} } ///Instance property ////// property is public only because base class has /// this public property declared /// public object Instance { get { return null; } } ///Propert Descriptor ////// property is public only because base class has /// this public property declared /// public PropertyDescriptor PropertyDescriptor { get { return null;} } #if !PBTCOMPILER // Make the ParserContext available internally as an optimization. public ParserContext ParserContext { get { return _parserContext; } } #endif #endregion Properties #endregion Public #region Internal #region Contructors #if !PBTCOMPILER ////// /// /// public TypeConvertContext(ParserContext parserContext) { _parserContext = parserContext; } #endif // temporary code to optimize Paints.White etc, until this is done // in a more generic fashion in SolidPaint ctor #if PBTCOMPILER ////// /// /// /// public TypeConvertContext(ParserContext parserContext, string originalAttributeValue) { _parserContext = parserContext; _attribStringValue = originalAttributeValue; } #endif #endregion Constructors #endregion internal #region Private #region Data ParserContext _parserContext; // _attribStringValue is never set when !PBTCOMPILER #pragma warning disable 0649 string _attribStringValue; #pragma warning restore 0649 #endregion Data #endregion Private } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
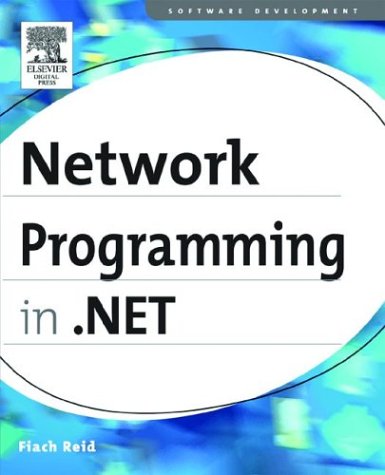
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripLabel.cs
- mda.cs
- SwitchElementsCollection.cs
- XPathQueryGenerator.cs
- DBBindings.cs
- AdRotatorDesigner.cs
- PropertyPath.cs
- PositiveTimeSpanValidatorAttribute.cs
- RecognizerStateChangedEventArgs.cs
- PerformanceCounterLib.cs
- GrammarBuilder.cs
- LocalizableAttribute.cs
- StatusBarDrawItemEvent.cs
- TextTreeRootTextBlock.cs
- RootAction.cs
- GestureRecognitionResult.cs
- CqlGenerator.cs
- WebContext.cs
- MessageContractImporter.cs
- ModifyActivitiesPropertyDescriptor.cs
- selecteditemcollection.cs
- FileDetails.cs
- IndependentlyAnimatedPropertyMetadata.cs
- WebPartDisplayModeCollection.cs
- ActivityCodeGenerator.cs
- XmlSchemaSimpleContentExtension.cs
- PeerToPeerException.cs
- DataGridTableStyleMappingNameEditor.cs
- AnnotationResourceChangedEventArgs.cs
- LifetimeServices.cs
- ConstraintManager.cs
- XmlUnspecifiedAttribute.cs
- TreeBuilderXamlTranslator.cs
- TemplateManager.cs
- SqlUDTStorage.cs
- ListBindableAttribute.cs
- CodeConstructor.cs
- WebPartMenuStyle.cs
- WaitHandleCannotBeOpenedException.cs
- BufferBuilder.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- XamlGridLengthSerializer.cs
- SoapExtensionTypeElement.cs
- OpCodes.cs
- OrderingQueryOperator.cs
- ObjectAssociationEndMapping.cs
- UInt32Storage.cs
- DependencySource.cs
- ObjectQuery.cs
- HostProtectionException.cs
- WindowsTab.cs
- MouseCaptureWithinProperty.cs
- Not.cs
- OleDbRowUpdatingEvent.cs
- VersionedStream.cs
- ProfilePropertySettingsCollection.cs
- FieldMetadata.cs
- SimplePropertyEntry.cs
- SystemWebSectionGroup.cs
- ClientRoleProvider.cs
- _AutoWebProxyScriptEngine.cs
- EventLogRecord.cs
- DbgUtil.cs
- BlurBitmapEffect.cs
- MexTcpBindingElement.cs
- AllMembershipCondition.cs
- ColumnTypeConverter.cs
- WebPartDisplayMode.cs
- DelayedRegex.cs
- RenderDataDrawingContext.cs
- SystemFonts.cs
- GeometryCombineModeValidation.cs
- SchemeSettingElement.cs
- EncodedStreamFactory.cs
- PatternMatchRules.cs
- AsnEncodedData.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- DesignerForm.cs
- TextServicesDisplayAttributePropertyRanges.cs
- FileDialog.cs
- CompensatableTransactionScopeActivityDesigner.cs
- ObjectAnimationBase.cs
- CalendarDataBindingHandler.cs
- NamespaceList.cs
- FloaterBaseParaClient.cs
- CallTemplateAction.cs
- ConnectionPointGlyph.cs
- _NetworkingPerfCounters.cs
- HttpResponseHeader.cs
- ScriptRef.cs
- NavigatorInput.cs
- MembershipAdapter.cs
- ServiceInstanceProvider.cs
- CaseInsensitiveHashCodeProvider.cs
- Scripts.cs
- NumericPagerField.cs
- MessageDesigner.cs
- FactorySettingsElement.cs
- ListBoxItemAutomationPeer.cs
- MessageLoggingElement.cs