Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / TemplateManager.cs / 1 / TemplateManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class TemplateManager { private XmlQualifiedName mode; internal ArrayList templates; private Stylesheet stylesheet; // Owning stylesheet private class TemplateComparer : IComparer { public int Compare(object x, object y) { Debug.Assert(x != null && x is TemplateAction); Debug.Assert(y != null && y is TemplateAction); TemplateAction tx = (TemplateAction) x; TemplateAction ty = (TemplateAction) y; Debug.Assert(! Double.IsNaN(tx.Priority)); Debug.Assert(! Double.IsNaN(ty.Priority)); if (tx.Priority == ty.Priority) { Debug.Assert(tx.TemplateId != ty.TemplateId || tx == ty); return tx.TemplateId - ty.TemplateId; } else { return tx.Priority > ty.Priority ? 1 : -1; } } } private static TemplateComparer s_TemplateComparer = new TemplateComparer(); internal XmlQualifiedName Mode { get { return this.mode; } } internal TemplateManager(Stylesheet stylesheet, XmlQualifiedName mode) { this.mode = mode; this.stylesheet = stylesheet; } internal void AddTemplate(TemplateAction template) { Debug.Assert(template != null); Debug.Assert( ((object) this.mode == (object) template.Mode) || (template.Mode == null && this.mode.Equals(XmlQualifiedName.Empty)) || this.mode.Equals(template.Mode) ); if (this.templates == null) { this.templates = new ArrayList(); } this.templates.Add(template); } internal void ProcessTemplates() { if (this.templates != null) { this.templates.Sort(s_TemplateComparer); } } internal TemplateAction FindTemplate(Processor processor, XPathNavigator navigator) { if (this.templates == null) { return null; } Debug.Assert(this.templates != null); for (int templateIndex = this.templates.Count - 1; templateIndex >= 0 ; templateIndex --) { TemplateAction action = (TemplateAction) this.templates[templateIndex]; int matchKey = action.MatchKey; if (matchKey != Compiler.InvalidQueryKey) { if (processor.Matches(navigator, matchKey)) { return action; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class TemplateManager { private XmlQualifiedName mode; internal ArrayList templates; private Stylesheet stylesheet; // Owning stylesheet private class TemplateComparer : IComparer { public int Compare(object x, object y) { Debug.Assert(x != null && x is TemplateAction); Debug.Assert(y != null && y is TemplateAction); TemplateAction tx = (TemplateAction) x; TemplateAction ty = (TemplateAction) y; Debug.Assert(! Double.IsNaN(tx.Priority)); Debug.Assert(! Double.IsNaN(ty.Priority)); if (tx.Priority == ty.Priority) { Debug.Assert(tx.TemplateId != ty.TemplateId || tx == ty); return tx.TemplateId - ty.TemplateId; } else { return tx.Priority > ty.Priority ? 1 : -1; } } } private static TemplateComparer s_TemplateComparer = new TemplateComparer(); internal XmlQualifiedName Mode { get { return this.mode; } } internal TemplateManager(Stylesheet stylesheet, XmlQualifiedName mode) { this.mode = mode; this.stylesheet = stylesheet; } internal void AddTemplate(TemplateAction template) { Debug.Assert(template != null); Debug.Assert( ((object) this.mode == (object) template.Mode) || (template.Mode == null && this.mode.Equals(XmlQualifiedName.Empty)) || this.mode.Equals(template.Mode) ); if (this.templates == null) { this.templates = new ArrayList(); } this.templates.Add(template); } internal void ProcessTemplates() { if (this.templates != null) { this.templates.Sort(s_TemplateComparer); } } internal TemplateAction FindTemplate(Processor processor, XPathNavigator navigator) { if (this.templates == null) { return null; } Debug.Assert(this.templates != null); for (int templateIndex = this.templates.Count - 1; templateIndex >= 0 ; templateIndex --) { TemplateAction action = (TemplateAction) this.templates[templateIndex]; int matchKey = action.MatchKey; if (matchKey != Compiler.InvalidQueryKey) { if (processor.Matches(navigator, matchKey)) { return action; } } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
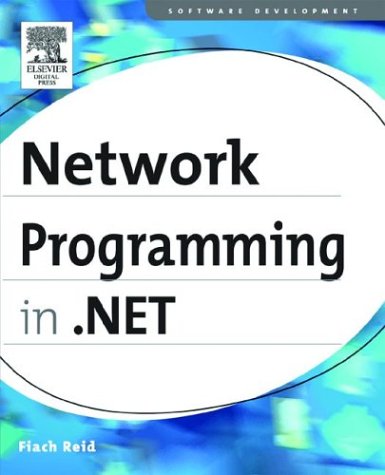
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GregorianCalendar.cs
- EventData.cs
- WebPartConnectionsDisconnectVerb.cs
- TextServicesCompartmentContext.cs
- GenericUI.cs
- FlowLayout.cs
- ComponentEvent.cs
- PointCollectionValueSerializer.cs
- AccessText.cs
- ArraySubsetEnumerator.cs
- FlowDocumentPaginator.cs
- CallContext.cs
- CredentialSelector.cs
- BufferedReadStream.cs
- Tokenizer.cs
- IntranetCredentialPolicy.cs
- PropertyNames.cs
- PassportAuthenticationModule.cs
- XmlQuerySequence.cs
- SecurityElement.cs
- ErrorLog.cs
- MetadataWorkspace.cs
- FormattedTextSymbols.cs
- Compress.cs
- ScrollChrome.cs
- PageAsyncTask.cs
- TrustLevelCollection.cs
- ProcessMessagesAsyncResult.cs
- Matrix3DValueSerializer.cs
- FileIOPermission.cs
- FactoryGenerator.cs
- ScaleTransform3D.cs
- XmlReader.cs
- DataReceivedEventArgs.cs
- EntityUtil.cs
- Stack.cs
- COAUTHIDENTITY.cs
- EventsTab.cs
- IsolatedStorageFileStream.cs
- MetaType.cs
- ListBoxItemWrapperAutomationPeer.cs
- Input.cs
- errorpatternmatcher.cs
- SoapObjectInfo.cs
- _ContextAwareResult.cs
- DoubleLink.cs
- WindowsUpDown.cs
- StringPropertyBuilder.cs
- HostedImpersonationContext.cs
- StylusDownEventArgs.cs
- Dictionary.cs
- FormatConvertedBitmap.cs
- DataGridColumnCollection.cs
- BaseTemplateBuildProvider.cs
- DelayDesigner.cs
- DataProtection.cs
- PolicyStatement.cs
- SystemInfo.cs
- HtmlInputRadioButton.cs
- FillErrorEventArgs.cs
- CopyNodeSetAction.cs
- HeaderedItemsControl.cs
- IdentitySection.cs
- DecoderBestFitFallback.cs
- DesignerObjectListAdapter.cs
- QilInvokeLateBound.cs
- ObjectSet.cs
- RoleService.cs
- ProviderConnectionPointCollection.cs
- Roles.cs
- IndexedEnumerable.cs
- DataGridTableCollection.cs
- TemplateBindingExtension.cs
- RtfToXamlReader.cs
- SelectionEditingBehavior.cs
- IndentTextWriter.cs
- WebHttpBinding.cs
- NullableConverter.cs
- ItemContainerGenerator.cs
- BasePattern.cs
- TreeSet.cs
- InputLanguageEventArgs.cs
- WorkflowOperationErrorHandler.cs
- TypeConverterHelper.cs
- UIAgentCrashedException.cs
- TextParagraphView.cs
- EntityProviderFactory.cs
- ProviderUtil.cs
- SqlTypeSystemProvider.cs
- Win32SafeHandles.cs
- ErrorFormatterPage.cs
- DecoderFallback.cs
- RectValueSerializer.cs
- XmlTextAttribute.cs
- ControlCachePolicy.cs
- SimpleType.cs
- TargetException.cs
- WebReferencesBuildProvider.cs
- LayoutTable.cs
- InvalidComObjectException.cs