Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / HostedImpersonationContext.cs / 1 / HostedImpersonationContext.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.ServiceModel; using System.ServiceModel.Diagnostics; using System.Security.Principal; using System.ServiceModel.ComIntegration; using System.ServiceModel.Security; using System.Web.Hosting; using System.Runtime.InteropServices; using System.ComponentModel; using System.Threading; using System.Security; using SafeCloseHandle = System.IdentityModel.SafeCloseHandleCritical; using SMD = System.ServiceModel.Diagnostics; ////// RequiresReview - All member methods are security critical. The class might be used outside of the restricted SecurityContext. /// Ensure they do not accidentally satisfy any demands /// [SecurityRequiresReview] class HostedImpersonationContext { ////// Critical - stores the impersonation token handle. since we're allowing "safe" Impersonation of the token we got from asp.net /// we need to protect this value /// [SecurityCritical] SafeCloseHandle tokenHandle; ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] int refCount = 0; ////// Critical - a security critical field, caller must use care /// [SecurityCritical] bool isImpersonated; ////// Critical - calls two safe native methods: GetCurrentThread and OpenCurrentThreadToken; and Marshal.GetLastWin32Error /// captures current thread token in a SecurityCritical field /// [SecurityCritical] public HostedImpersonationContext() { if (ServiceHostingEnvironment.AspNetCompatibilityEnabled) { bool isSuccess = SafeNativeMethods.OpenCurrentThreadTokenCritical(SafeNativeMethods.GetCurrentThread(), TokenAccessLevels.Query | TokenAccessLevels.Impersonate, true, out tokenHandle); int error = Marshal.GetLastWin32Error(); if (isSuccess) { isImpersonated = true; Interlocked.Increment(ref refCount); } else { SMD.Utility.CloseInvalidOutSafeHandleCritical(tokenHandle); tokenHandle = null; if (error != (int)Win32Error.ERROR_NO_TOKEN) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(error, SR.GetString(SR.Hosting_ImpersonationFailed))); } } } } public bool IsImpersonated { ////// Critical - accesses SecurityCritical isImpersonated field /// Safe - does not leak control or mutable/harmful data, no potential for harm /// [SecurityCritical, SecurityTreatAsSafe] get { return isImpersonated; } } ////// Critical - accesses SecurityCritical tokenHandle field and uses LinkDemanded DangerousGetHandle method as well as UnsafeCreate /// caller should use with care, must take responsibility for reverting impersonation /// [SecurityCritical] public IDisposable Impersonate() { if (!isImpersonated) return null; DiagnosticUtility.DebugAssert(tokenHandle != null, "The token handle was incorrectly released earlier."); HostedInnerImpersonationContext context = null; lock(tokenHandle) { context = HostedInnerImpersonationContext.UnsafeCreate(tokenHandle.DangerousGetHandle()); GC.KeepAlive(tokenHandle); } return context; } ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] public void AddRef() { if (IsImpersonated) { Interlocked.Increment(ref refCount); } } ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] public void Release() { if (IsImpersonated) { DiagnosticUtility.DebugAssert(tokenHandle != null, "The token handle is incorrectly released earlier."); int currentCount = Interlocked.Decrement(ref refCount); if (currentCount == 0) { lock(tokenHandle) { tokenHandle.Close(); tokenHandle = null; } } } } ////// Critical - keeps track of impersonated user, caller must use with care and call Dispose at the appropriate time /// [SecurityCritical(SecurityCriticalScope.Everything)] class HostedInnerImpersonationContext : IDisposable { IDisposable impersonatedContext; HostedInnerImpersonationContext(IDisposable impersonatedContext) { if (impersonatedContext == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.Hosting_ImpersonationFailed))); } this.impersonatedContext = impersonatedContext; } public static HostedInnerImpersonationContext UnsafeCreate(IntPtr token) { return new HostedInnerImpersonationContext(HostingEnvironmentWrapper.UnsafeImpersonate(token)); } public void Dispose() { if (impersonatedContext != null) { impersonatedContext.Dispose(); impersonatedContext = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
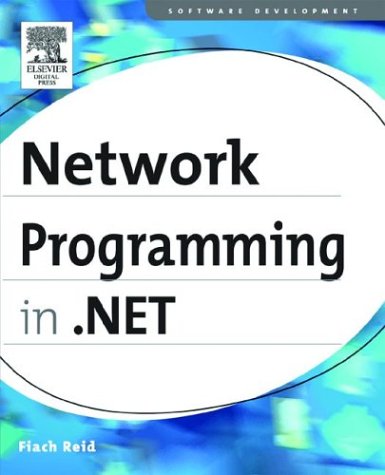
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CalendarModeChangedEventArgs.cs
- NativeMethodsCLR.cs
- HighlightVisual.cs
- HtmlContainerControl.cs
- ListBindableAttribute.cs
- ThicknessKeyFrameCollection.cs
- CodeLabeledStatement.cs
- CatalogZoneBase.cs
- RelationshipEndMember.cs
- ParenExpr.cs
- Utils.cs
- RegexMatchCollection.cs
- BitmapCodecInfo.cs
- LayoutTableCell.cs
- GlobalizationSection.cs
- Psha1DerivedKeyGeneratorHelper.cs
- IDQuery.cs
- UnsafeNativeMethods.cs
- TogglePatternIdentifiers.cs
- TextEditorParagraphs.cs
- WindowsFormsSectionHandler.cs
- DependencyObject.cs
- SerializationObjectManager.cs
- CompleteWizardStep.cs
- DelegateHelpers.cs
- VSWCFServiceContractGenerator.cs
- UpdatePanelControlTrigger.cs
- TypeGeneratedEventArgs.cs
- AsynchronousChannel.cs
- DiscoveryDocumentSerializer.cs
- SQLDateTimeStorage.cs
- PeerPresenceInfo.cs
- EngineSite.cs
- ConstructorNeedsTagAttribute.cs
- DispatcherExceptionEventArgs.cs
- PrivateFontCollection.cs
- HtmlString.cs
- FormViewInsertedEventArgs.cs
- GeneratedCodeAttribute.cs
- TraceSection.cs
- SoapFault.cs
- ObjectItemCollection.cs
- XmlName.cs
- URL.cs
- unitconverter.cs
- TextRangeBase.cs
- Utils.cs
- AffineTransform3D.cs
- OracleDataReader.cs
- WindowVisualStateTracker.cs
- InvokeMemberBinder.cs
- ValueChangedEventManager.cs
- SpellCheck.cs
- MissingMemberException.cs
- DocobjHost.cs
- EndpointAddress10.cs
- PackageRelationshipCollection.cs
- SqlBulkCopyColumnMappingCollection.cs
- RefreshPropertiesAttribute.cs
- XpsFilter.cs
- RenderContext.cs
- RunInstallerAttribute.cs
- InvalidOperationException.cs
- PeerNearMe.cs
- XpsFixedDocumentSequenceReaderWriter.cs
- GeometryValueSerializer.cs
- CardSpaceException.cs
- ExpandCollapseProviderWrapper.cs
- Block.cs
- CodeCatchClause.cs
- OneOfConst.cs
- ObjectSet.cs
- StrongName.cs
- ObjectDisposedException.cs
- ZoomingMessageFilter.cs
- NotConverter.cs
- StoryFragments.cs
- BamlResourceSerializer.cs
- WebResponse.cs
- CollectionsUtil.cs
- PrintDocument.cs
- TypeSystemHelpers.cs
- XmlAttributeProperties.cs
- ResourceAttributes.cs
- SspiSafeHandles.cs
- XMLDiffLoader.cs
- FlowchartDesigner.Helpers.cs
- BindingWorker.cs
- TypographyProperties.cs
- Models.cs
- SqlClientPermission.cs
- PrivilegeNotHeldException.cs
- KeyGesture.cs
- XXXOnTypeBuilderInstantiation.cs
- TypeFieldSchema.cs
- WinCategoryAttribute.cs
- ImplicitInputBrush.cs
- SiteMapNodeItem.cs
- ChannelManager.cs
- PeerInvitationResponse.cs