Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Policy / URL.cs / 1 / URL.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Url.cs // // Url is an IIdentity representing url internet sites. // namespace System.Security.Policy { using System.IO; using System.Security.Util; using UrlIdentityPermission = System.Security.Permissions.UrlIdentityPermission; using System.Runtime.Serialization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Url : IIdentityPermissionFactory, IBuiltInEvidence { private URLString m_url; internal Url() { m_url = null; } internal Url( SerializationInfo info, StreamingContext context ) { m_url = new URLString( (String)info.GetValue( "Url", typeof( String ) ) ); } internal Url( String name, bool parsed ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name, parsed ); } public Url( String name ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name ); } public String Value { get { if (m_url == null) return null; return m_url.ToString(); } } internal URLString GetURLString() { return m_url; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new UrlIdentityPermission( m_url ); } public override bool Equals(Object o) { if (o == null) return false; if (o is Url) { Url url = (Url) o; if (this.m_url == null) { return url.m_url == null; } else if (url.m_url == null) { return false; } else { return this.m_url.Equals( url.m_url ); } } return false; } public override int GetHashCode() { if (this.m_url == null) return 0; else return this.m_url.GetHashCode(); } public Object Copy() { Url url = new Url(); url.m_url = this.m_url; return url; } internal SecurityElement ToXml() { SecurityElement root = new SecurityElement( "System.Security.Policy.Url" ); // If you hit this assertt then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assertt below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Url" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_url != null) root.AddChild( new SecurityElement( "Url", m_url.ToString() ) ); return root; } public override String ToString() { return ToXml().ToString(); } ///int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position++] = BuiltInEvidenceHelper.idUrl; String value = this.Value; int length = value.Length; if (verbose) { BuiltInEvidenceHelper.CopyIntToCharArray(length, buffer, position); position += 2; } value.CopyTo( 0, buffer, position, length ); return length + position; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose ) { if (verbose) return this.Value.Length + 3; else return this.Value.Length + 1; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position) { int length = BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); position += 2; m_url = new URLString( new String(buffer, position, length )); return position + length; } #if false public void GetObjectData(SerializationInfo info, StreamingContext context) { String normalizedUrl = m_url.NormalizeUrl(); info.AddValue( "Url", normalizedUrl ); } #endif // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_url.NormalizeUrl(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
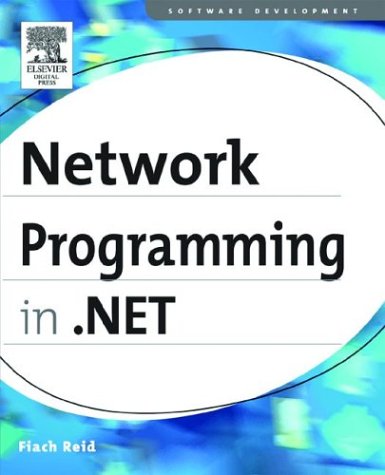
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutSettingsTypeConverter.cs
- DBDataPermission.cs
- WpfXamlType.cs
- PageWrapper.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- Stack.cs
- UpdatePanelTriggerCollection.cs
- CompilationSection.cs
- ItemCollection.cs
- WebHttpEndpoint.cs
- HttpResponseHeader.cs
- Context.cs
- WindowPattern.cs
- PersonalizationProvider.cs
- WebPartMenuStyle.cs
- TypeDelegator.cs
- TextTreeDeleteContentUndoUnit.cs
- ScaleTransform3D.cs
- LocationSectionRecord.cs
- ItemsPresenter.cs
- Mapping.cs
- DigitShape.cs
- RotationValidation.cs
- VirtualPathProvider.cs
- LinkedResource.cs
- FixedTextContainer.cs
- WinEventHandler.cs
- HttpConfigurationSystem.cs
- ConnectionManagementElementCollection.cs
- EUCJPEncoding.cs
- DesignerVerbCollection.cs
- Timer.cs
- RootBrowserWindowProxy.cs
- XsdBuilder.cs
- PointIndependentAnimationStorage.cs
- PointCollectionValueSerializer.cs
- ProviderConnectionPointCollection.cs
- UnsafeMethods.cs
- HttpCachePolicyWrapper.cs
- ListBoxDesigner.cs
- HtmlShim.cs
- HtmlEmptyTagControlBuilder.cs
- SecurityHelper.cs
- OverrideMode.cs
- DocumentViewerHelper.cs
- PointAnimationBase.cs
- PreloadedPackages.cs
- LineProperties.cs
- SettingsBindableAttribute.cs
- cookieexception.cs
- ProgressChangedEventArgs.cs
- EntityClassGenerator.cs
- ManipulationStartingEventArgs.cs
- ParenthesizePropertyNameAttribute.cs
- ModelService.cs
- AutomationElement.cs
- SmiMetaData.cs
- StrongTypingException.cs
- Debugger.cs
- GeometryCollection.cs
- FunctionMappingTranslator.cs
- ListItemCollection.cs
- VoiceChangeEventArgs.cs
- DataGridViewHitTestInfo.cs
- AsyncCompletedEventArgs.cs
- XPathCompileException.cs
- ITreeGenerator.cs
- CodeSnippetCompileUnit.cs
- XmlNodeComparer.cs
- GuidTagList.cs
- ToolStripSettings.cs
- LineInfo.cs
- WebPartMovingEventArgs.cs
- BitmapEffectGeneralTransform.cs
- BamlReader.cs
- HostedElements.cs
- DataGridViewRowCancelEventArgs.cs
- SystemUnicastIPAddressInformation.cs
- SystemColorTracker.cs
- StyleCollectionEditor.cs
- DataAdapter.cs
- ExtenderControl.cs
- ProbeMatchesCD1.cs
- NonVisualControlAttribute.cs
- ICspAsymmetricAlgorithm.cs
- HttpCacheParams.cs
- sqlmetadatafactory.cs
- DesignerActionHeaderItem.cs
- _BaseOverlappedAsyncResult.cs
- ImageKeyConverter.cs
- ILGenerator.cs
- MergablePropertyAttribute.cs
- PageTheme.cs
- PerspectiveCamera.cs
- SelectionEditingBehavior.cs
- Boolean.cs
- BufferedWebEventProvider.cs
- HtmlInputHidden.cs
- InvalidCastException.cs
- WsatServiceCertificate.cs