Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Tools / WSATConfig / Configuration / WsatServiceCertificate.cs / 1305376 / WsatServiceCertificate.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel.WsatConfig { using System; using System.Net; using System.Runtime.InteropServices; using System.Security.Cryptography.X509Certificates; class WsatServiceCertificate { X509Certificate2 cert; uint port; string certificateStore = "MY"; internal WsatServiceCertificate(X509Certificate2 cert, uint port) { this.cert = cert; this.port = port; } internal void BindSSLCertificate() { if (Utilities.IsHttpApiLibAvailable) { BindSSL(); } } internal void UnbindSSLCertificate() { if (Utilities.IsHttpApiLibAvailable) { this.UnbindSSL(); } } void BindSSL() { int retVal = SafeNativeMethods.NoError; WinsockSockAddr sockAddr = null; try { retVal = SafeNativeMethods.HttpInitialize(HttpWrapper.HttpApiVersion1, SafeNativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); if (SafeNativeMethods.NoError == retVal) { IntPtr pOverlapped = IntPtr.Zero; sockAddr = new WinsockSockAddr(new IPAddress(0), (short)this.port); HttpServiceConfigSslSet sslConf = new HttpServiceConfigSslSet(); sslConf.KeyDesc.pIpPort = sockAddr.PinnedSockAddr; sslConf.ParamDesc.DefaultCertCheckMode = 0; sslConf.ParamDesc.DefaultFlags = SafeNativeMethods.HTTP_SERVICE_CONFIG_SSL_FLAG_NEGOTIATE_CLIENT_CERT; sslConf.ParamDesc.DefaultRevocationFreshnessTime = 0; sslConf.ParamDesc.pSslCertStoreName = certificateStore; byte[] sslHash = this.cert.GetCertHash(); sslConf.ParamDesc.pSslHash = new SafeLocalAllocation(sslHash.Length); sslConf.ParamDesc.pSslHash.Copy(sslHash, 0, sslHash.Length); sslConf.ParamDesc.SslHashLength = sslHash.Length; int configInformationLength = Marshal.SizeOf(sslConf); retVal = SafeNativeMethods.HttpSetServiceConfiguration_Ssl(IntPtr.Zero, HttpServiceConfigId.HttpServiceConfigSSLCertInfo, ref sslConf, configInformationLength, pOverlapped); if (SafeNativeMethods.ErrorAlreadyExists == retVal) { retVal = SafeNativeMethods.HttpDeleteServiceConfiguration_Ssl(IntPtr.Zero, HttpServiceConfigId.HttpServiceConfigSSLCertInfo, ref sslConf, configInformationLength, IntPtr.Zero); if (SafeNativeMethods.NoError == retVal) { retVal = SafeNativeMethods.HttpSetServiceConfiguration_Ssl(IntPtr.Zero, HttpServiceConfigId.HttpServiceConfigSSLCertInfo, ref sslConf, configInformationLength, pOverlapped); } } GC.KeepAlive(sockAddr); sslConf.ParamDesc.pSslHash.Close(); } } finally { if (sockAddr != null) { sockAddr.Dispose(); } SafeNativeMethods.HttpTerminate(SafeNativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); } if (SafeNativeMethods.NoError != retVal) { if (SafeNativeMethods.ErrorAlreadyExists == retVal) { throw new WsatAdminException(WsatAdminErrorCode.HTTPS_PORT_SSL_CERT_BINDING_ALREADYEXISTS, SR.GetString(SR.ErrorHttpsPortSSLBindingAlreadyExists)); } else { throw new WsatAdminException(WsatAdminErrorCode.HTTPS_PORT_SSL_CERT_BINDING, SR.GetString(SR.ErrorHttpsPortSSLBinding, retVal)); } } } void UnbindSSL() { int retVal = SafeNativeMethods.NoError; WinsockSockAddr sockAddr = null; try { retVal = SafeNativeMethods.HttpInitialize(HttpWrapper.HttpApiVersion1, SafeNativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); if (SafeNativeMethods.NoError == retVal) { IntPtr pOverlapped = IntPtr.Zero; sockAddr = new WinsockSockAddr(new IPAddress(0), (short)this.port); HttpServiceConfigSslSet sslConf = new HttpServiceConfigSslSet(); sslConf.KeyDesc.pIpPort = sockAddr.PinnedSockAddr; sslConf.ParamDesc.DefaultCertCheckMode = 0; sslConf.ParamDesc.DefaultFlags = SafeNativeMethods.HTTP_SERVICE_CONFIG_SSL_FLAG_NEGOTIATE_CLIENT_CERT; sslConf.ParamDesc.DefaultRevocationFreshnessTime = 0; sslConf.ParamDesc.pSslCertStoreName = certificateStore; byte[] sslHash = this.cert.GetCertHash(); sslConf.ParamDesc.pSslHash = new SafeLocalAllocation(sslHash.Length); sslConf.ParamDesc.pSslHash.Copy(sslHash, 0, sslHash.Length); sslConf.ParamDesc.SslHashLength = sslHash.Length; int configInformationLength = System.Runtime.InteropServices.Marshal.SizeOf(sslConf); retVal = SafeNativeMethods.HttpDeleteServiceConfiguration_Ssl(IntPtr.Zero, HttpServiceConfigId.HttpServiceConfigSSLCertInfo, ref sslConf, configInformationLength, pOverlapped); sslConf.ParamDesc.pSslHash.Close(); GC.KeepAlive(sockAddr); } } finally { if (sockAddr != null) { sockAddr.Dispose(); } SafeNativeMethods.HttpTerminate(SafeNativeMethods.HTTP_INITIALIZE_CONFIG, IntPtr.Zero); } if (retVal != SafeNativeMethods.NoError && retVal != SafeNativeMethods.FileNotFound && retVal != SafeNativeMethods.ErrorInvalidParameter) { throw new WsatAdminException(WsatAdminErrorCode.HTTPS_PORT_SSL_CERT_UNBINDING, SR.GetString(SR.ErrorHttpsPortSSLUnbinding, retVal)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
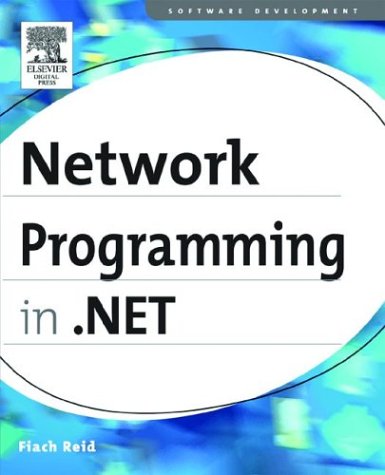
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOCentralDirectoryFileHeader.cs
- CapabilitiesUse.cs
- ArgumentsParser.cs
- SoapFormatExtensions.cs
- RepeaterDataBoundAdapter.cs
- DecimalAnimationBase.cs
- LOSFormatter.cs
- AutoResizedEvent.cs
- UpdateManifestForBrowserApplication.cs
- DragAssistanceManager.cs
- Rotation3DKeyFrameCollection.cs
- KeyValueConfigurationElement.cs
- SqlDesignerDataSourceView.cs
- ProfileInfo.cs
- _FtpDataStream.cs
- LockedHandleGlyph.cs
- SortDescriptionCollection.cs
- BitmapImage.cs
- ZipIOLocalFileHeader.cs
- Gdiplus.cs
- ChildChangedEventArgs.cs
- DependencyPropertyChangedEventArgs.cs
- SqlParameterCollection.cs
- FormParameter.cs
- BookmarkWorkItem.cs
- input.cs
- AuthorizationBehavior.cs
- MessagingDescriptionAttribute.cs
- ProcessThread.cs
- XmlBinaryReader.cs
- DesignerOptionService.cs
- ServiceModelActivity.cs
- CodeAttachEventStatement.cs
- _FixedSizeReader.cs
- BatchServiceHost.cs
- SelectionEditingBehavior.cs
- TypedServiceOperationListItem.cs
- QilParameter.cs
- PropertySourceInfo.cs
- SizeKeyFrameCollection.cs
- HostingEnvironment.cs
- ListSurrogate.cs
- Exception.cs
- WebBaseEventKeyComparer.cs
- StrokeCollectionConverter.cs
- CharacterMetrics.cs
- ToolboxItemImageConverter.cs
- XmlBindingWorker.cs
- AuthorizationRuleCollection.cs
- RSAOAEPKeyExchangeDeformatter.cs
- XmlIterators.cs
- SoapSchemaExporter.cs
- Rotation3DKeyFrameCollection.cs
- UnicodeEncoding.cs
- DataControlFieldHeaderCell.cs
- DataBinding.cs
- NotifyInputEventArgs.cs
- ActivityMarkupSerializer.cs
- RotateTransform.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- IdleTimeoutMonitor.cs
- PageTextBox.cs
- CLSCompliantAttribute.cs
- TemplateControlParser.cs
- MailDefinition.cs
- CompiledQuery.cs
- ResourcesBuildProvider.cs
- DataGridViewAutoSizeModeEventArgs.cs
- HtmlControl.cs
- ReadOnlyHierarchicalDataSourceView.cs
- SQlBooleanStorage.cs
- Transform3D.cs
- DataRelationPropertyDescriptor.cs
- MultipleViewPattern.cs
- ConfigurationLockCollection.cs
- CompilerCollection.cs
- MobileControlPersister.cs
- FixUpCollection.cs
- ListViewEditEventArgs.cs
- glyphs.cs
- SqlTypesSchemaImporter.cs
- UIPropertyMetadata.cs
- ToolStripCustomTypeDescriptor.cs
- VectorAnimationBase.cs
- ZipPackage.cs
- DataGridViewHitTestInfo.cs
- List.cs
- DependentList.cs
- WebServiceHandlerFactory.cs
- Thread.cs
- ContentWrapperAttribute.cs
- MouseEvent.cs
- CompModSwitches.cs
- ControlValuePropertyAttribute.cs
- ProxyFragment.cs
- CipherData.cs
- UserThread.cs
- Win32MouseDevice.cs
- XmlILStorageConverter.cs
- WebPartEditorOkVerb.cs