Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / NetworkInformation / SystemUnicastIPAddressInformation.cs / 1 / SystemUnicastIPAddressInformation.cs
////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; /// /// Provides support for ip configuation information and statistics. /// /// Specifies the unicast addresses for an interface. ///OS < XP ////// internal class SystemUnicastIPAddressInformation:UnicastIPAddressInformation { private IpAdapterUnicastAddress adapterAddress; private long dhcpLeaseLifetime; private SystemIPAddressInformation innerInfo; internal IPAddress ipv4Mask; private SystemUnicastIPAddressInformation() { } internal SystemUnicastIPAddressInformation(IpAdapterInfo ipAdapterInfo, IPExtendedAddress address){ innerInfo = new SystemIPAddressInformation(address.address); DateTime tempdate = new DateTime(1970,1,1); tempdate = tempdate.AddSeconds(ipAdapterInfo.leaseExpires); dhcpLeaseLifetime = (long)((tempdate - DateTime.UtcNow).TotalSeconds); ipv4Mask = address.mask; } internal SystemUnicastIPAddressInformation(IpAdapterUnicastAddress adapterAddress, IPAddress ipAddress){ innerInfo = new SystemIPAddressInformation(adapterAddress,ipAddress); this.adapterAddress = adapterAddress; dhcpLeaseLifetime = adapterAddress.leaseLifetime; } ///public override IPAddress Address{get {return innerInfo.Address;}} public override IPAddress IPv4Mask{ get { if(Address.AddressFamily != AddressFamily.InterNetwork){ return new IPAddress(0); } return ipv4Mask; } } /// /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (innerInfo.IsTransient); } } ////// This address can be used for DNS. public override bool IsDnsEligible{ get { return (innerInfo.IsDnsEligible); } } ///public override PrefixOrigin PrefixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.prefixOrigin; } } /// public override SuffixOrigin SuffixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.suffixOrigin; } } /// /// IPv6 only. Specifies the duplicate address detection state. Only supported /// for IPv6. If called on an IPv4 address, will throw a "not supported" exception. public override DuplicateAddressDetectionState DuplicateAddressDetectionState{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.dadState; } } ////// Specifies the valid lifetime of the address in seconds. public override long AddressValidLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.validLifetime; } } ////// Specifies the prefered lifetime of the address in seconds. public override long AddressPreferredLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.preferredLifetime; } } ////// /// Specifies the prefered lifetime of the address in seconds. public override long DhcpLeaseLifetime{ get { return dhcpLeaseLifetime; } } //helper method that marshals the addressinformation into the classes internal static UnicastIPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. UnicastIPAddressInformationCollection addressList = new UnicastIPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterUnicastAddress addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); } return addressList; } } }
Link Menu
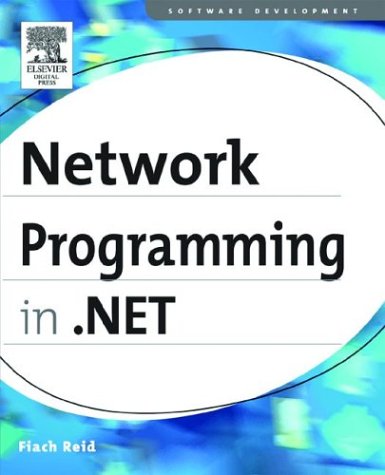
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PenContext.cs
- dbdatarecord.cs
- MULTI_QI.cs
- DataServiceQueryException.cs
- PreDigestedSignedInfo.cs
- OletxTransactionHeader.cs
- TextServicesHost.cs
- RankException.cs
- HMACSHA256.cs
- ModuleElement.cs
- DependencyPropertyAttribute.cs
- _ConnectOverlappedAsyncResult.cs
- SafeFileMappingHandle.cs
- EncryptedType.cs
- StringAnimationUsingKeyFrames.cs
- TextChange.cs
- EpmContentDeSerializer.cs
- BooleanAnimationBase.cs
- AnnotationDocumentPaginator.cs
- StylusButtonEventArgs.cs
- FileDataSourceCache.cs
- SqlDataSourceSelectingEventArgs.cs
- IDictionary.cs
- StandardOleMarshalObject.cs
- ObjectDataSourceMethodEventArgs.cs
- Parser.cs
- FeatureSupport.cs
- RuleInfoComparer.cs
- SharedUtils.cs
- XmlDocumentSerializer.cs
- CompilerCollection.cs
- WorkflowQueue.cs
- Thread.cs
- WebServiceReceive.cs
- DecoderNLS.cs
- EUCJPEncoding.cs
- TextBox.cs
- SqlFactory.cs
- EntityKey.cs
- FunctionImportMapping.cs
- CodeTypeOfExpression.cs
- DataServiceQueryOfT.cs
- cookieexception.cs
- SessionStateItemCollection.cs
- SimpleWebHandlerParser.cs
- SByte.cs
- XmlHierarchicalEnumerable.cs
- ControllableStoryboardAction.cs
- CellConstantDomain.cs
- XmlSchemaType.cs
- _NegoState.cs
- ControlCachePolicy.cs
- ProfileSettings.cs
- OletxTransactionFormatter.cs
- Stack.cs
- MimeTypePropertyAttribute.cs
- DataObjectCopyingEventArgs.cs
- LostFocusEventManager.cs
- AttributeInfo.cs
- VersionConverter.cs
- LinkLabelLinkClickedEvent.cs
- DataGridViewCellCollection.cs
- MaskedTextBox.cs
- DictionaryEntry.cs
- EventProviderWriter.cs
- DeviceSpecificDialogCachedState.cs
- StrongName.cs
- ServiceMetadataExtension.cs
- ElementMarkupObject.cs
- DiscreteKeyFrames.cs
- HtmlSelect.cs
- ApplicationSecurityInfo.cs
- Journaling.cs
- PeerPresenceInfo.cs
- OrderPreservingPipeliningMergeHelper.cs
- DataGrid.cs
- DataGridViewComboBoxEditingControl.cs
- FileStream.cs
- TdsParserStaticMethods.cs
- COM2ICategorizePropertiesHandler.cs
- WindowsGraphics2.cs
- FrameAutomationPeer.cs
- AssemblyCache.cs
- HostingEnvironmentException.cs
- ImmComposition.cs
- TextElementCollectionHelper.cs
- XmlNamespaceMapping.cs
- CheckBoxAutomationPeer.cs
- ExpressionBuilder.cs
- XmlDataCollection.cs
- HttpServerUtilityBase.cs
- RtfToken.cs
- SplineKeyFrames.cs
- TranslateTransform.cs
- ApplicationId.cs
- ThreadPool.cs
- HyperLinkField.cs
- GeometryGroup.cs
- Int64.cs
- OperationResponse.cs