Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / SqlClient / TdsParserStaticMethods.cs / 1 / TdsParserStaticMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.Data.Common; using System.Data.ProviderBase; using System.Data.Sql; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Threading; internal sealed class TdsParserStaticMethods { private TdsParserStaticMethods() { /* prevent utility class from being insantiated*/ } // // Static methods // static internal void AliasRegistryLookup(ref string host, ref string protocol) { if (!ADP.IsEmpty(host)) { const String folder = "SOFTWARE\\Microsoft\\MSSQLServer\\Client\\ConnectTo"; // Put a try...catch... around this so we don't abort ANY connection if we can't read the registry. string aliasLookup = (string) ADP.LocalMachineRegistryValue(folder, host); if (!ADP.IsEmpty(aliasLookup)) { /* Result will be in the form of: "DBNMPNTW,\\blained1\pipe\sql\query". or Result will be in the form of: "DBNETLIB, via:\\blained1\pipe\sql\query". supported formats: tcp - DBMSSOCN,[server|server\instance][,port] np - DBNMPNTW,[\\server\pipe\sql\query | \\server\pipe\MSSQL$instance\sql\query] where \sql\query is the pipename and can be replaced with any other pipe name via - [DBMSGNET,server,port | DBNETLIB, via:server, port] sm - DBMSLPCN,server unsupported formats: rpc - DBMSRPCN,server,[parameters] where parameters could be "username,password" bv - DBMSVINN,service@group@organization appletalk - DBMSADSN,objectname@zone spx - DBMSSPXN,[service | address,port,network] */ // We must parse into the two component pieces, then map the first protocol piece to the // appropriate value. int index = aliasLookup.IndexOf(','); // If we found the key, but there was no "," in the string, it is a bad Alias so return. if (-1 != index) { string parsedProtocol = aliasLookup.Substring(0, index).ToLower(CultureInfo.InvariantCulture); // If index+1 >= length, Alias consisted of "FOO," which is a bad alias so return. if (index+1 < aliasLookup.Length) { string parsedAliasName = aliasLookup.Substring(index+1); // Fix bug 298286 if ("dbnetlib" == parsedProtocol) { index = parsedAliasName.IndexOf(':'); if (-1 != index && index + 1 < parsedAliasName.Length) { parsedProtocol = parsedAliasName.Substring (0, index); if (SqlConnectionString.ValidProtocal (parsedProtocol)) { protocol = parsedProtocol; host = parsedAliasName.Substring(index + 1); } } } else { protocol = (string)SqlConnectionString.NetlibMapping()[parsedProtocol]; if (null != protocol) { host = parsedAliasName; } } } } } } } static internal Byte[] EncryptPassword(string password) { Byte[] bEnc = new Byte[password.Length << 1]; int s; byte bLo; byte bHi; for (int i = 0; i < password.Length; i ++) { s = (int) password[i]; bLo = (byte) (s & 0xff); bHi = (byte) ((s >> 8) & 0xff); bEnc[i<<1] = (Byte) ( (((bLo & 0x0f) << 4) | (bLo >> 4)) ^ 0xa5 ); bEnc[(i<<1)+1] = (Byte) ( (((bHi & 0x0f) << 4) | (bHi >> 4)) ^ 0xa5); } return bEnc; } static internal int GetCurrentProcessId() { return SafeNativeMethods.GetCurrentProcessId(); } static internal byte[] GetNIC() { // NIC address is stored in NetworkAddress key. However, if NetworkAddressLocal key // has a value that is not zero, then we cannot use the NetworkAddress key and must // instead generate a random one. I do not fully understand why, this is simply what // the native providers do. As for generation, I use a random number generator, which // means that different processes on the same machine will have different NIC address // values on the server. It is not ideal, but native does not have the same value for // different processes either. const string key = "NetworkAddress"; const string localKey = "NetworkAddressLocal"; const string folder = "SOFTWARE\\Description\\Microsoft\\Rpc\\UuidTemporaryData"; int result = 0; byte[] nicAddress = null; object temp = ADP.LocalMachineRegistryValue(folder, localKey); if (temp is int) { result = (int) temp; } if (result <= 0) { temp = ADP.LocalMachineRegistryValue(folder, key); if (temp is byte[]) { nicAddress = (byte[]) temp; } } if (null == nicAddress) { nicAddress = new byte[TdsEnums.MAX_NIC_SIZE]; Random random = new Random(); random.NextBytes(nicAddress); } return nicAddress; } // translates remaining time in stateObj (from user specified timeout) to timout value for SNI static internal Int32 GetTimeoutMilliseconds(long timeoutTime) { // User provided timeout t | timeout value for SNI | meaning // ------------------------+-----------------------+------------------------------ // t == long.MaxValue | -1 | infinite timeout (no timeout) // t>0 && tint.MaxValue | int.MaxValue | must not exceed int.MaxValue if (Int64.MaxValue == timeoutTime) { return -1; // infinite timeout } long msecRemaining = ADP.TimerRemainingMilliseconds(timeoutTime); if (msecRemaining < 0) { return 0; } if (msecRemaining > (long)Int32.MaxValue) { return Int32.MaxValue; } return (Int32)msecRemaining; } static internal long GetTimeoutSeconds(int timeoutSeconds) { long result; if (0 == timeoutSeconds) { result = Int64.MaxValue; // no timeout... } else { long ticksNow = ADP.TimerCurrent(); result = ticksNow + ADP.TimerFromSeconds(timeoutSeconds); } return result; } static internal bool TimeoutHasExpired(long timeoutTime) { bool result = false; if (0 != timeoutTime && Int64.MaxValue != timeoutTime) { result = ADP.TimerHasExpired(timeoutTime); } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.Data.Common; using System.Data.ProviderBase; using System.Data.Sql; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Threading; internal sealed class TdsParserStaticMethods { private TdsParserStaticMethods() { /* prevent utility class from being insantiated*/ } // // Static methods // static internal void AliasRegistryLookup(ref string host, ref string protocol) { if (!ADP.IsEmpty(host)) { const String folder = "SOFTWARE\\Microsoft\\MSSQLServer\\Client\\ConnectTo"; // Put a try...catch... around this so we don't abort ANY connection if we can't read the registry. string aliasLookup = (string) ADP.LocalMachineRegistryValue(folder, host); if (!ADP.IsEmpty(aliasLookup)) { /* Result will be in the form of: "DBNMPNTW,\\blained1\pipe\sql\query". or Result will be in the form of: "DBNETLIB, via:\\blained1\pipe\sql\query". supported formats: tcp - DBMSSOCN,[server|server\instance][,port] np - DBNMPNTW,[\\server\pipe\sql\query | \\server\pipe\MSSQL$instance\sql\query] where \sql\query is the pipename and can be replaced with any other pipe name via - [DBMSGNET,server,port | DBNETLIB, via:server, port] sm - DBMSLPCN,server unsupported formats: rpc - DBMSRPCN,server,[parameters] where parameters could be "username,password" bv - DBMSVINN,service@group@organization appletalk - DBMSADSN,objectname@zone spx - DBMSSPXN,[service | address,port,network] */ // We must parse into the two component pieces, then map the first protocol piece to the // appropriate value. int index = aliasLookup.IndexOf(','); // If we found the key, but there was no "," in the string, it is a bad Alias so return. if (-1 != index) { string parsedProtocol = aliasLookup.Substring(0, index).ToLower(CultureInfo.InvariantCulture); // If index+1 >= length, Alias consisted of "FOO," which is a bad alias so return. if (index+1 < aliasLookup.Length) { string parsedAliasName = aliasLookup.Substring(index+1); // Fix bug 298286 if ("dbnetlib" == parsedProtocol) { index = parsedAliasName.IndexOf(':'); if (-1 != index && index + 1 < parsedAliasName.Length) { parsedProtocol = parsedAliasName.Substring (0, index); if (SqlConnectionString.ValidProtocal (parsedProtocol)) { protocol = parsedProtocol; host = parsedAliasName.Substring(index + 1); } } } else { protocol = (string)SqlConnectionString.NetlibMapping()[parsedProtocol]; if (null != protocol) { host = parsedAliasName; } } } } } } } static internal Byte[] EncryptPassword(string password) { Byte[] bEnc = new Byte[password.Length << 1]; int s; byte bLo; byte bHi; for (int i = 0; i < password.Length; i ++) { s = (int) password[i]; bLo = (byte) (s & 0xff); bHi = (byte) ((s >> 8) & 0xff); bEnc[i<<1] = (Byte) ( (((bLo & 0x0f) << 4) | (bLo >> 4)) ^ 0xa5 ); bEnc[(i<<1)+1] = (Byte) ( (((bHi & 0x0f) << 4) | (bHi >> 4)) ^ 0xa5); } return bEnc; } static internal int GetCurrentProcessId() { return SafeNativeMethods.GetCurrentProcessId(); } static internal byte[] GetNIC() { // NIC address is stored in NetworkAddress key. However, if NetworkAddressLocal key // has a value that is not zero, then we cannot use the NetworkAddress key and must // instead generate a random one. I do not fully understand why, this is simply what // the native providers do. As for generation, I use a random number generator, which // means that different processes on the same machine will have different NIC address // values on the server. It is not ideal, but native does not have the same value for // different processes either. const string key = "NetworkAddress"; const string localKey = "NetworkAddressLocal"; const string folder = "SOFTWARE\\Description\\Microsoft\\Rpc\\UuidTemporaryData"; int result = 0; byte[] nicAddress = null; object temp = ADP.LocalMachineRegistryValue(folder, localKey); if (temp is int) { result = (int) temp; } if (result <= 0) { temp = ADP.LocalMachineRegistryValue(folder, key); if (temp is byte[]) { nicAddress = (byte[]) temp; } } if (null == nicAddress) { nicAddress = new byte[TdsEnums.MAX_NIC_SIZE]; Random random = new Random(); random.NextBytes(nicAddress); } return nicAddress; } // translates remaining time in stateObj (from user specified timeout) to timout value for SNI static internal Int32 GetTimeoutMilliseconds(long timeoutTime) { // User provided timeout t | timeout value for SNI | meaning // ------------------------+-----------------------+------------------------------ // t == long.MaxValue | -1 | infinite timeout (no timeout) // t>0 && tint.MaxValue | int.MaxValue | must not exceed int.MaxValue if (Int64.MaxValue == timeoutTime) { return -1; // infinite timeout } long msecRemaining = ADP.TimerRemainingMilliseconds(timeoutTime); if (msecRemaining < 0) { return 0; } if (msecRemaining > (long)Int32.MaxValue) { return Int32.MaxValue; } return (Int32)msecRemaining; } static internal long GetTimeoutSeconds(int timeoutSeconds) { long result; if (0 == timeoutSeconds) { result = Int64.MaxValue; // no timeout... } else { long ticksNow = ADP.TimerCurrent(); result = ticksNow + ADP.TimerFromSeconds(timeoutSeconds); } return result; } static internal bool TimeoutHasExpired(long timeoutTime) { bool result = false; if (0 != timeoutTime && Int64.MaxValue != timeoutTime) { result = ADP.TimerHasExpired(timeoutTime); } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
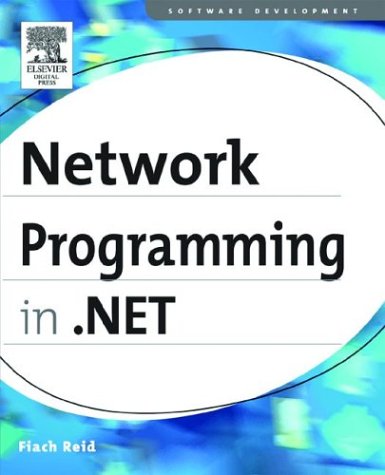
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompilerError.cs
- DecoderFallbackWithFailureFlag.cs
- MetabaseServerConfig.cs
- SqlDataSourceView.cs
- CallContext.cs
- ContentTextAutomationPeer.cs
- OleAutBinder.cs
- DropShadowBitmapEffect.cs
- StylusSystemGestureEventArgs.cs
- SymLanguageType.cs
- ProviderException.cs
- UIntPtr.cs
- CatalogPartChrome.cs
- AutoGeneratedFieldProperties.cs
- ColorPalette.cs
- PostBackOptions.cs
- Bold.cs
- NativeMethods.cs
- EventItfInfo.cs
- EntityContainer.cs
- WebRequest.cs
- UserControl.cs
- PageThemeCodeDomTreeGenerator.cs
- SiteMembershipCondition.cs
- UiaCoreTypesApi.cs
- ReachPrintTicketSerializerAsync.cs
- TypeTypeConverter.cs
- SoapExtensionTypeElement.cs
- PhoneCall.cs
- SoapIncludeAttribute.cs
- ComplexLine.cs
- DataComponentGenerator.cs
- WmlLabelAdapter.cs
- XhtmlBasicCommandAdapter.cs
- UnicodeEncoding.cs
- TextSegment.cs
- ObjectDataProvider.cs
- RenderDataDrawingContext.cs
- TextContainer.cs
- InternalTypeHelper.cs
- SelectionProviderWrapper.cs
- ResourceDisplayNameAttribute.cs
- ModuleBuilder.cs
- PackWebRequest.cs
- ZipQueryOperator.cs
- DataGridCellsPresenter.cs
- RelationshipEndMember.cs
- ConstNode.cs
- LocalFileSettingsProvider.cs
- HttpInputStream.cs
- AppDomainAttributes.cs
- AlternateViewCollection.cs
- XmlJsonWriter.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- PaperSize.cs
- InitializerFacet.cs
- PointIndependentAnimationStorage.cs
- XmlDictionaryWriter.cs
- DesigntimeLicenseContext.cs
- DataBoundLiteralControl.cs
- MessageLoggingFilterTraceRecord.cs
- WindowsToolbarItemAsMenuItem.cs
- CancellationTokenRegistration.cs
- ImageField.cs
- UnknownBitmapDecoder.cs
- PointLightBase.cs
- DetailsViewCommandEventArgs.cs
- EUCJPEncoding.cs
- XmlTextReader.cs
- ArgIterator.cs
- SvcMapFile.cs
- MsmqVerifier.cs
- EntityTransaction.cs
- Internal.cs
- CompressedStack.cs
- DataComponentGenerator.cs
- WinEventHandler.cs
- DataGridViewControlCollection.cs
- DataObjectMethodAttribute.cs
- XamlBrushSerializer.cs
- HtmlInputControl.cs
- CompareInfo.cs
- DocumentProperties.cs
- DurableEnlistmentState.cs
- FormParameter.cs
- ReflectEventDescriptor.cs
- DataGrid.cs
- TranslateTransform3D.cs
- DbDataSourceEnumerator.cs
- ManagementOptions.cs
- Msec.cs
- FileDialog.cs
- IriParsingElement.cs
- PageThemeParser.cs
- GrammarBuilder.cs
- Decoder.cs
- GraphicsState.cs
- AuthenticationModuleElement.cs
- ZoomingMessageFilter.cs
- LoginDesigner.cs